To convert a SecureString to plain text in PowerShell, you can use the following command that decrypts the SecureString and outputs it as readable text:
$secureString = ConvertTo-SecureString "YourSecurePassword" -AsPlainText -Force
$plainText = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto([System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureString))
Write-Host $plainText
Understanding SecureStrings
What Makes SecureStrings Secure?
SecureStrings are a datatype in PowerShell designed to handle sensitive information, like passwords, with enhanced security. Unlike normal strings, which can be exposed in memory, SecureStrings use encryption to protect their content.
When stored in memory, data represented as SecureStrings is encrypted and only accessible to authorized users. This prevents malicious actors from reading sensitive data during a memory dump or through external software tools.
To emphasize the difference, consider this: when you handle a plain text password, it exists unprotected in memory, making it susceptible to unauthorized access. SecureStrings mitigate this risk by keeping the data encrypted until it is specifically retrieved for a valid function.
Common Use Cases for SecureStrings
SecureStrings are frequently used in various scenarios that involve secure handling of data. Here are a few notable instances:
- Storing Passwords: When automating processes that require a password, using SecureStrings prevents exposing the actual password during operation.
- Handling Sensitive Information: Apart from passwords, SecureStrings can securely store API keys, encryption keys, and other sensitive data that require confidentiality.
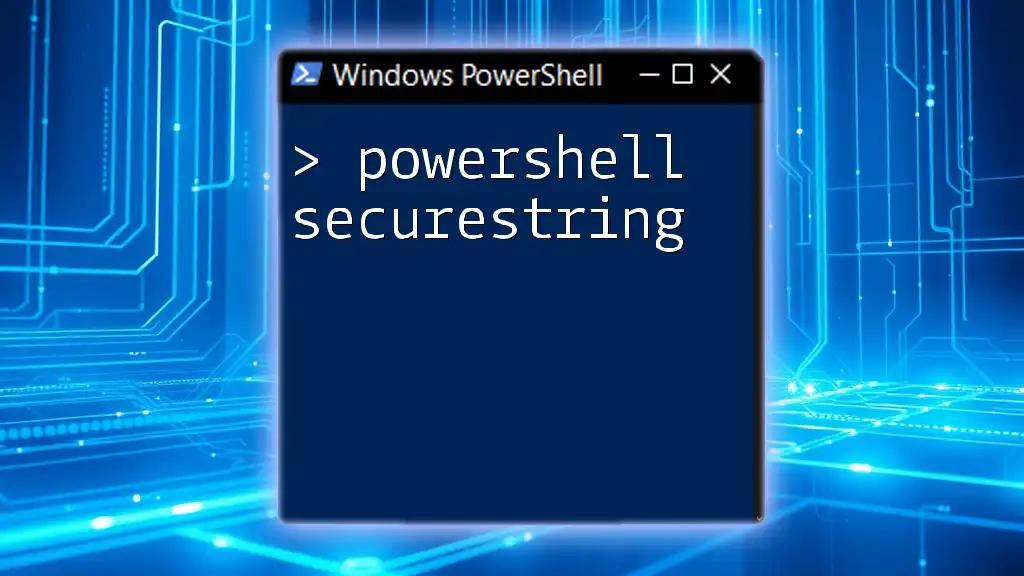
Converting SecureString to Plain Text
Why Convert SecureString to Plain Text?
With all the security measures in place, you might wonder why anyone would want to convert a SecureString back to plain text. The primary reason is compatibility with older applications or scripts that do not accept SecureStrings. In these situations, you’ll need to retrieve the original plain text for processes that cannot handle SecureStrings.
Step-by-Step Guide to Conversion
Using PowerShell Cmdlets
To convert a SecureString back to plain text, PowerShell provides a couple of cmdlets that you can use effectively. Let’s break down the conversion process using these cmdlets.
Convert a Plain Text String to SecureString
First, let’s create a SecureString from a plain text password:
$secureString = ConvertTo-SecureString "YourPassword" -AsPlainText -Force
In this example, `ConvertTo-SecureString` takes the plain text password "YourPassword" and converts it into a SecureString, with the `-AsPlainText` and `-Force` parameters indicating that we are explicitly instructing PowerShell to treat the input as plain text.
Convert SecureString to Plain Text
Once we have our SecureString, we can convert it back to plain text:
$plainText = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto(
[System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureString))
In this code snippet:
- The `SecureStringToBSTR` method converts our SecureString to a binary string (BSTR).
- The `PtrToStringAuto` method then retrieves the plain text version from this binary representation.
Exploring the Code Snippets
Let’s take a closer look at the breakdown of this conversion process:
- Creation of SecureString: By converting a simple password string to a SecureString, we implement a layer of security over sensitive data in memory.
- Conversion to Plain Text: This step involves two method calls from the `System.Runtime.InteropServices.Marshal` namespace, which is required for safely handling secure data within .NET.
While using these methods is straightforward, it is crucial to remember that the converted plain text can be exposed to various threats as it exists unencrypted in memory during processing.

Security Considerations
Risks of Converting SecureStrings
Converting a SecureString to plain text introduces potential data exposure risks. While it may be necessary, there are implications regarding where and how the plain text gets used.
When a SecureString is converted, it is processed wholly in memory, which can be temporarily captured or mishandled by other running processes or tools. Mishandling could allow sensitive information to be logged, shared, or exposed.
Best Practices for Handling Plain Text
To ensure that your plain text remains secure after conversion, consider the following practices:
- Limit Duration in Memory: Keep plain text in memory for the shortest duration necessary. Clear variables holding sensitive data as soon as you are done using them.
- Avoid Logging Sensitive Information: Never log plain text passwords or sensitive data during script execution.
Alternatives to Consider
Using SecureStrings Instead
Whenever possible, prefer to use SecureStrings throughout your scripts to minimize exposure. Keeping sensitive data in a SecureString format reduces the temptation to convert and exposes it to risk at multiple points.
External Password Management Solutions
For situations where you consistently need access to sensitive data, consider using external password management solutions. These tools allow you to manage sensitive information securely without needing to handle plain text directly in your scripts.

Conclusion
In summary, understanding how to work with PowerShell SecureStrings is an invaluable skill for anyone dealing with sensitive information in scripts. While converting a SecureString to plain text can be necessary for compatibility reasons, it is essential to recognize the security implications involved with this action.
Learning to effectively manage $secureString and employing best practices will empower you to improve the security of your scripts significantly. With the power of PowerShell at your fingertips, always strive to maintain a high security standard while automating tasks involving sensitive information.

Additional Resources
For further reading, refer to the official PowerShell documentation on SecureStrings and security best practices. Engage with the PowerShell community through forums and user groups to continually enhance your understanding and skills in secure scripting practices.
Call to Action
Join the community to stay updated on the latest developments in PowerShell! Your journey in mastering PowerShell and secure scripting will not only enhance your productivity but also ensure that you are well-equipped to handle sensitive information with confidence.