A PowerShell script template provides a structured framework that simplifies the creation of scripts, allowing users to efficiently implement commands and automate tasks.
Here's a basic example of a PowerShell script template:
# Script Name: ExampleScript.ps1
# Description: A brief description of what the script does
# Author: Your Name
# Date: Today's Date
# Begin script
Write-Host 'Hello, World!'
# Add your commands below
# Example command
# Get-Process
What is a PowerShell Script Template?
A PowerShell Script Template serves as a foundational blueprint for creating PowerShell scripts efficiently. It helps standardize scripting practices and ensures your code is organized, reusable, and maintainable. By using a template, you can quickly generate scripts that adhere to best practices without having to start from scratch each time.
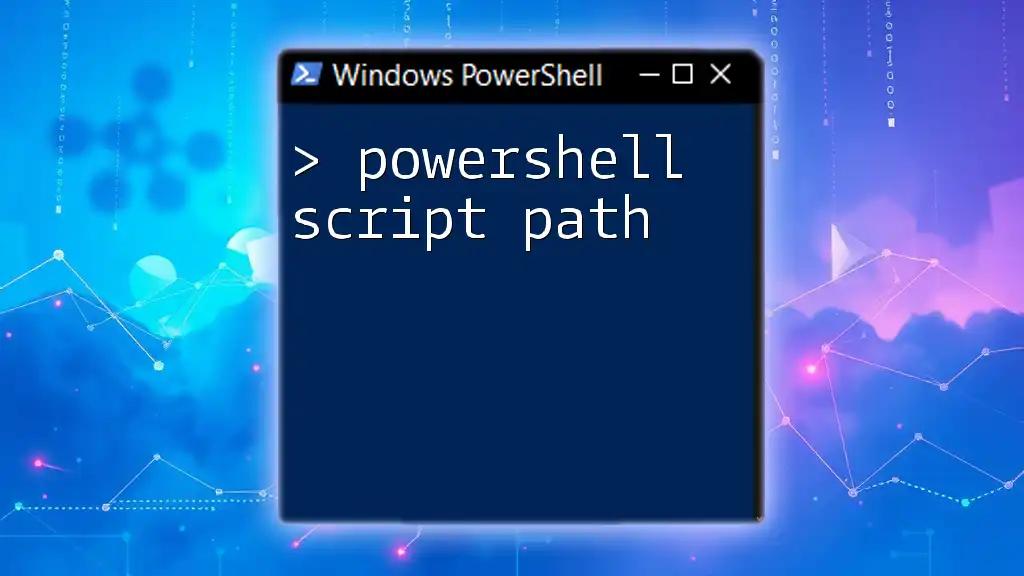
Benefits of Using PowerShell Templates
Consistency in Coding
One of the foremost advantages of using PowerShell templates is consistency. Whether you're working on a small project or across a large team, adhering to a standardized structure fosters uniformity. This consistency not only enhances readability but also makes it easier for others (and your future self) to understand and collaborate on the code.
Time Efficiency
Using a template can significantly boost time efficiency. For example, instead of recalibrating your code structure for every new task, a well-designed template provides a ready-made framework. This can save you hours and promote faster script development.
Error Reduction
Another essential benefit is the reduction of errors. By utilizing predefined structures and best practices within your template, you minimize the chances of making common mistakes. This leads to more robust scripts that perform effectively upon execution.
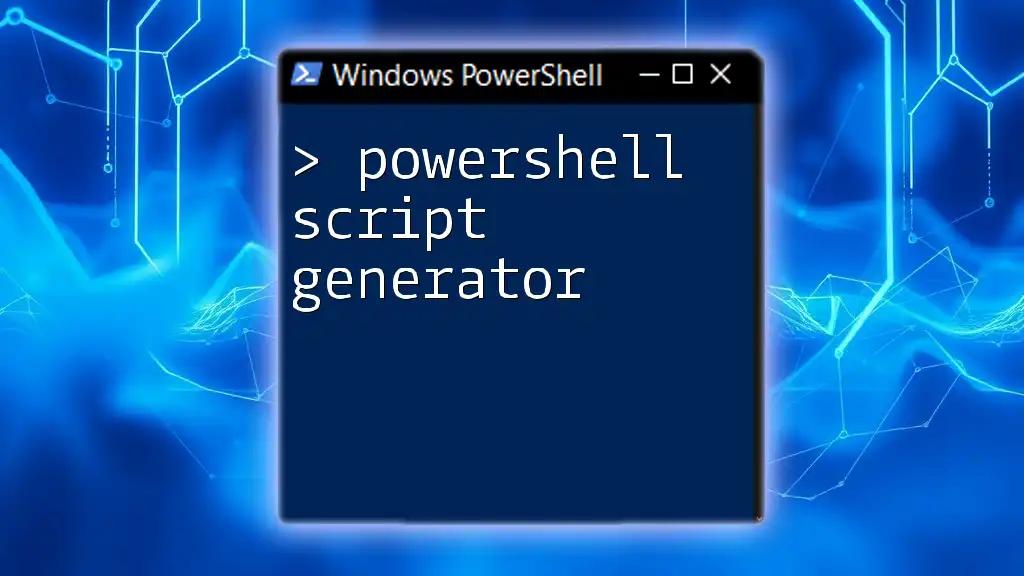
Basic Structure of a PowerShell Script Template
Key Components
Header Comments
Header comments are critical in providing context about a script. They help document its purpose, parameters, and usage examples. A well-crafted header improves the script's readability and usability. A typical header comment block may look like this:
<#
.SYNOPSIS
Brief description of the script
.DESCRIPTION
Detailed explanation of the script functionality
.PARAMETER Parameter1
Description of parameter 1
.EXAMPLE
Example of how to use the script
#>
Parameter Declaration
Declaring parameters allows users to customize the script's input, making it versatile. By using the `param` keyword, you define the data your script will accept. Here’s an example of how to declare parameters:
param (
[string]$Parameter1,
[int]$Parameter2 = 10 # Default value
)
In this example, Parameter1 is a string input, while Parameter2: is an integer with a default value of 10. This gives users flexibility since they can override default values when needed.
Function Definitions
Modularizing code through function definitions is crucial for maintainability. Functions encapsulate specific tasks, making it easier to debug and reuse code segments. Here’s an example of how a simple function can be structured:
function Process-Data {
param (
[string]$InputData
)
# Processing logic here
}
In this instance, Process-Data takes a single string parameter called InputData. This function can be invoked throughout the script as needed.
Main Execution Block
The main execution block dictates how the entire script runs. It typically includes checks, calls to functions, or any other required logic. Here’s a straightforward illustration:
if ($PSCmdlet.MyInvocation.BoundParameters.Count -eq 0) {
Write-Host "No parameters were supplied."
} else {
Process-Data -InputData $Parameter1
}
In this block, the script checks if any parameters were supplied. If not, it provides user feedback, and if parameters exist, it executes Process-Data with the specified input.
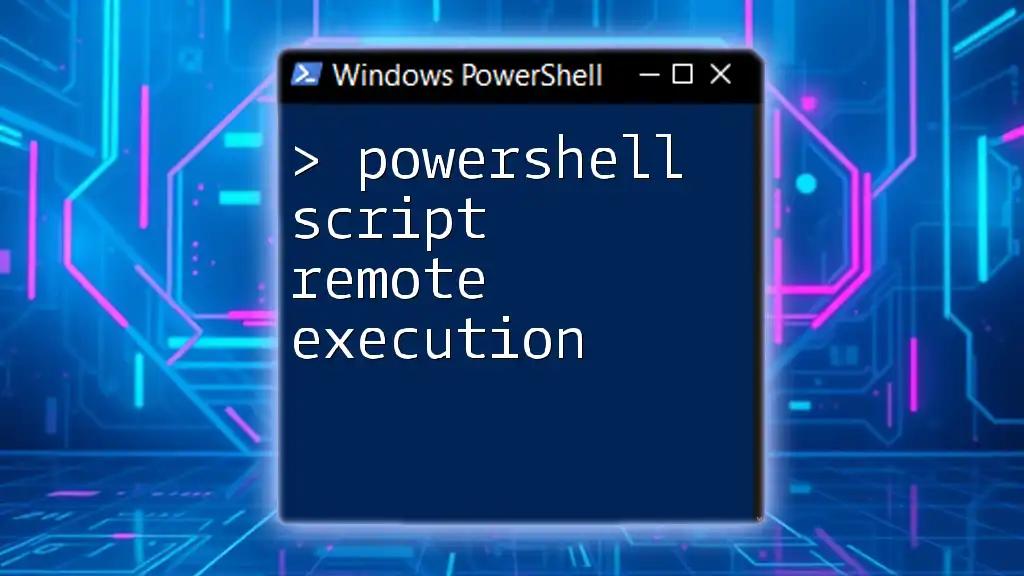
Creating Your Own PowerShell Script Template
Steps to Develop a Personal Template
Identify Common Tasks
The first step in creating a personal PowerShell script template is to identify commonly performed tasks you encounter regularly. Doing so enables you to address the most significant pain points in your scripting.
Outline Basic Structure
Once you understand your needs, outline the basic structure using the components discussed earlier. This becomes the skeleton of your customized PowerShell script template.
Example of a Simple PowerShell Script Template
Combining all elements, here’s a complete example that demonstrates how these components come together in a PowerShell script template:
<#
.SYNOPSIS
A simple template script for demonstration
.DESCRIPTION
This script is a basic template for PowerShell scripts.
.PARAMETER Name
The name to process
.EXAMPLE
.\MyScript.ps1 -Name "John"
#>
param (
[string]$Name
)
function Greet-User {
param (
[string]$UserName
)
Write-Host "Hello, $UserName!"
}
Greet-User -UserName $Name
In this template, users can run the script by providing a specific name to greet. The function encapsulates greeting logic, illustrating modular design.
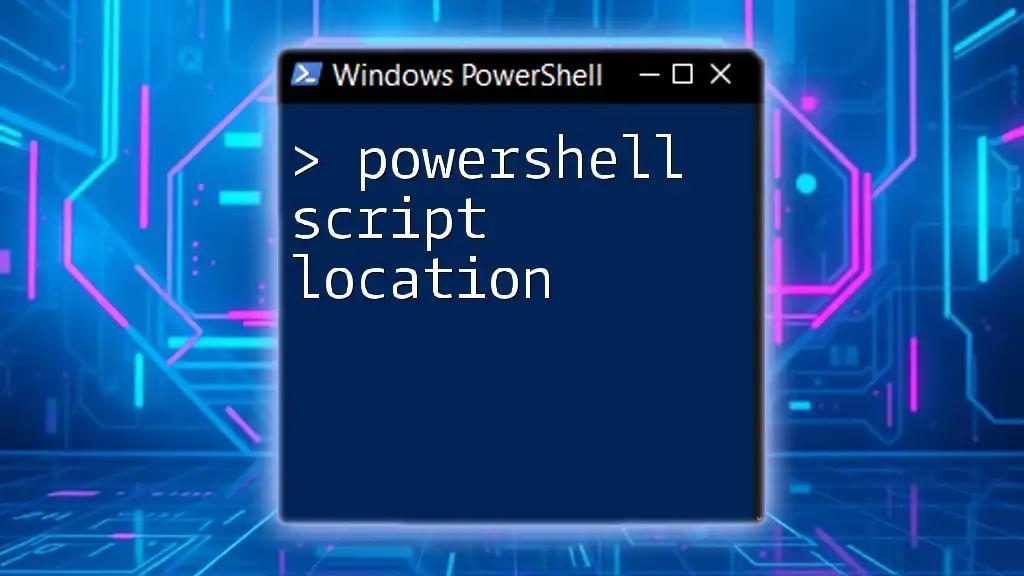
Tips for Optimizing Your PowerShell Templates
Best Practices
Commenting and Documentation
Effective commenting and thorough documentation cannot be overstated. Well-commented scripts allow not just others, but also your future self to revisit the code with greater ease. Consider including details on each parameter, function, and logic employed in your scripts.
Error Handling Techniques
Implementing error handling techniques significantly improves script reliability. By using `Try/Catch` blocks, you can manage exceptions gracefully. Here’s an example:
try {
# Code that may cause an error
} catch {
Write-Host "An error occurred: $_"
}
This structure helps capture errors, providing feedback and preventing script failures from occurring unnoticed.
Testing Your Templates
Always test your templates in a controlled environment. This allows you to iron out any bugs and confirm that your templates work correctly under various scenarios.
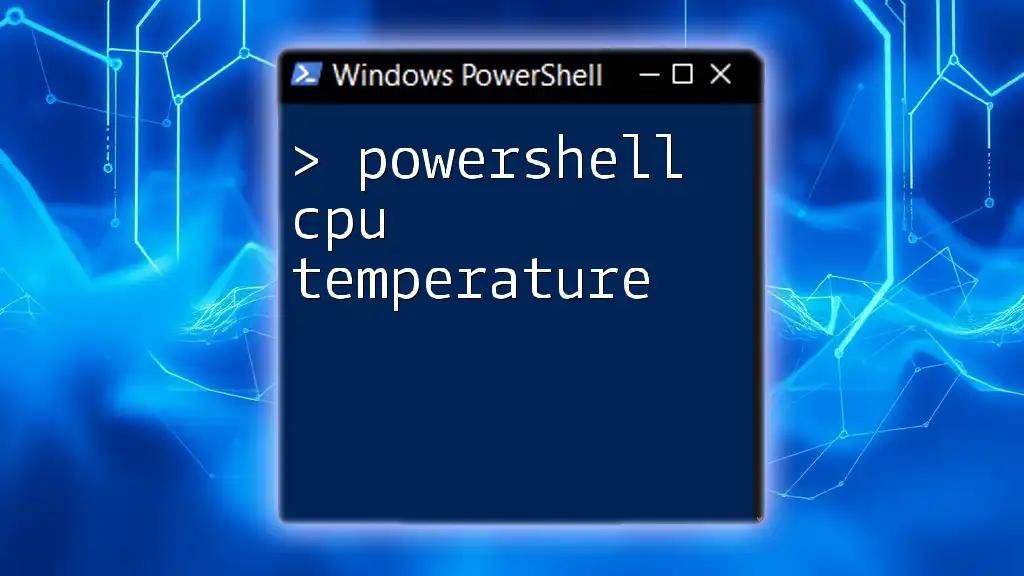
Expanding Your Templates for Advanced Use
Leveraging Modules and Functions
To truly maximize the utility of your PowerShell script template, consider creating reusable functions. By structuring functions for reuse across multiple scripts, you not only save time but also bolster the consistency and reliability of your code.
Using modules is also a strategic way to organize your scripts. Modules allow you to package related functions together, providing a convenient way to manage and distribute your code.
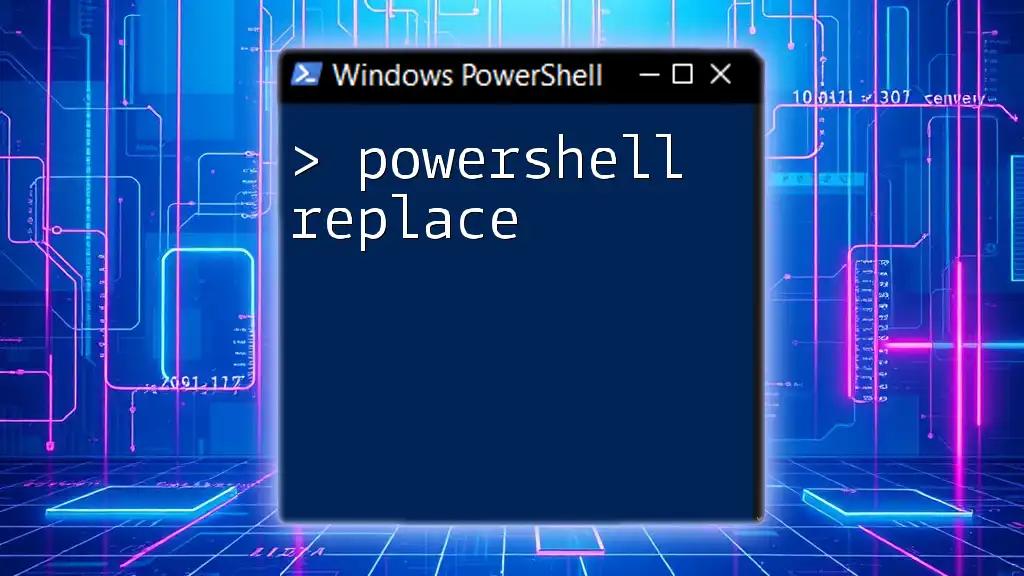
Conclusion
In summary, PowerShell script templates are invaluable tools that promote coding efficiency, consistency, and error reduction. By taking the time to create and refine your own templates, you can streamline your scripting tasks significantly. Remember, as you grow familiar with creating templates, consider customizing and sharing your work with others in the community to foster collaboration and improvement.
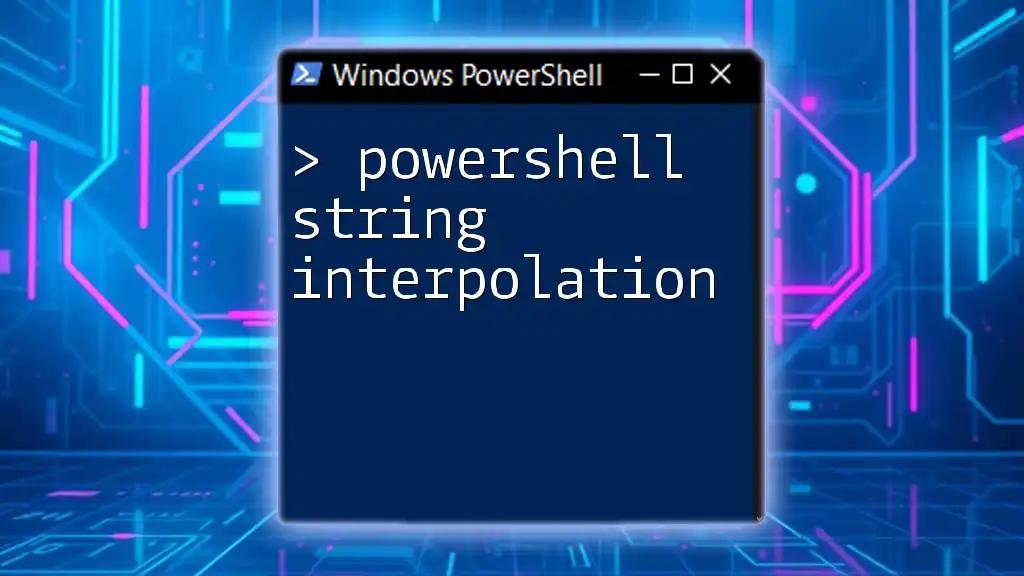
Additional Resources
For those eager to dive deeper into PowerShell scripting, consider exploring the official PowerShell documentation or participating in community forums where you can find scripts shared by other enthusiasts. There are many valuable courses and tutorials available that will enhance your PowerShell skills further.