To retrieve the CPU temperature using PowerShell, you can utilize the `Get-WmiObject` command to query the thermal zone data from the Win32_TemperatureProbe class. Here's a simple code snippet to get you started:
Get-WmiObject -Namespace root\wmi -Class MSAcpi_ThermalZone | Select-Object -Property CurrentTemperature
This command will return the current temperature of your CPU in tenths of degrees Kelvin.
Understanding CPU Temperature
What is CPU Temperature?
CPU temperature refers to the heat generated by the processor during operation. Understanding this temperature is crucial because it directly impacts the performance of your computer. Most CPUs have an optimal operating temperature range. Operating outside this range can lead to performance throttling or even permanent damage.
Impact of High CPU Temperature
When the CPU operates at elevated temperatures, several adverse effects can occur.
- Performance Issues: High temperatures can cause the CPU to throttle its performance, meaning it runs slower to prevent damage.
- Risk of Overheating: Prolonged high temperatures may lead to hardware malfunctions or failure.
- System Instability: Crashes and unexpected behavior become more prevalent in systems where the CPU is consistently overheating.
Therefore, regular monitoring of CPU temperature is essential for maintaining system health and performance.
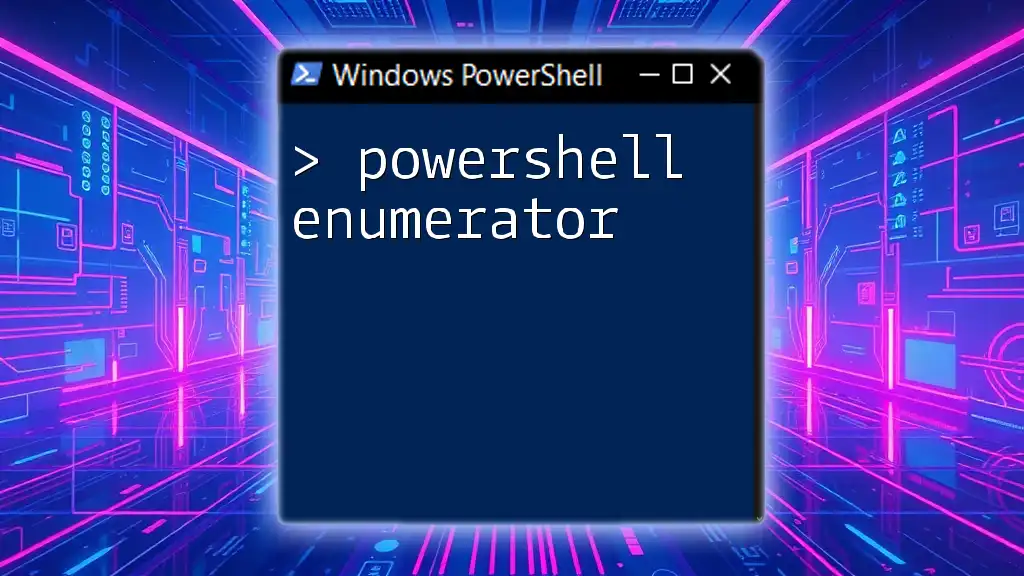
Setting Up PowerShell for CPU Monitoring
Prerequisites
Before you can monitor CPU temperature, ensure that PowerShell is installed on your system. Additionally, you may need administrative permissions to access certain system information.
Installing Necessary Tools
Using PowerShell Modules
PowerShell supports various modules that facilitate obtaining hardware information, including CPU temperature measurements. One such recommended module is OpenHardwareMonitor.
Example: Installing OpenHardwareMonitor Module
To install the module, run the following command in PowerShell:
Install-Module -Name OpenHardwareMonitor
This simple command will enable you to access the necessary functionalities for monitoring hardware metrics including CPU temperature.
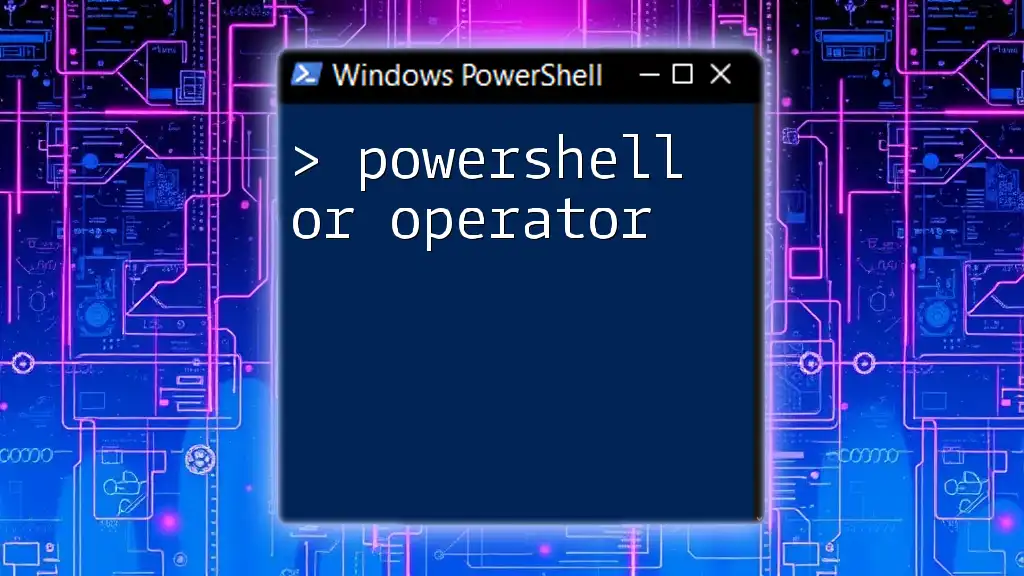
Accessing CPU Temperature Data
Using WMI (Windows Management Instrumentation)
WMI Overview
WMI is a core component of Windows that allows scripts and management applications to interact with various system components. It can provide detailed information on hardware, including CPU temperature.
Retrieving CPU Temperature with WMI
To get the CPU temperature using WMI, you can execute the following command:
Get-WmiObject -Namespace root\wmi -Class MSAcpi_ThermalZone
This command retrieves temperature readings from the thermal zone. The output you get will show temperature values, typically in tenths of degrees Kelvin, which you can convert to Celsius for better understanding.
Using OpenHardwareMonitor
Accessing OpenHardwareMonitor Data
To use OpenHardwareMonitor for CPU temperature readings, first make sure to download and run OpenHardwareMonitor. This tool provides detailed insights and the necessary libraries to gather real-time data.
Example: Using OpenHardwareMonitor with PowerShell
To retrieve CPU temperature via OpenHardwareMonitor, use the following script:
# Load OpenHardwareMonitor assembly
Add-Type -Path "C:\Path\To\OpenHardwareMonitorLib.dll"
# Create object and retrieve temperatures
$monitor = New-Object OpenHardwareMonitor.Hardware.Computer
$monitor.Open()
$monitor.CPUEnabled = $true
$monitor.Activate()
# Display CPU temperatures
$monitor.Hardware | Where-Object { $_.HardwareType -eq 'Processor' } | ForEach-Object { $_.Sensors | Where-Object { $_.SensorType -eq 'Temperature' } }
This example loads the OpenHardwareMonitor library and activates the CPU data collection, providing clear temperature data that can be further processed or logged.
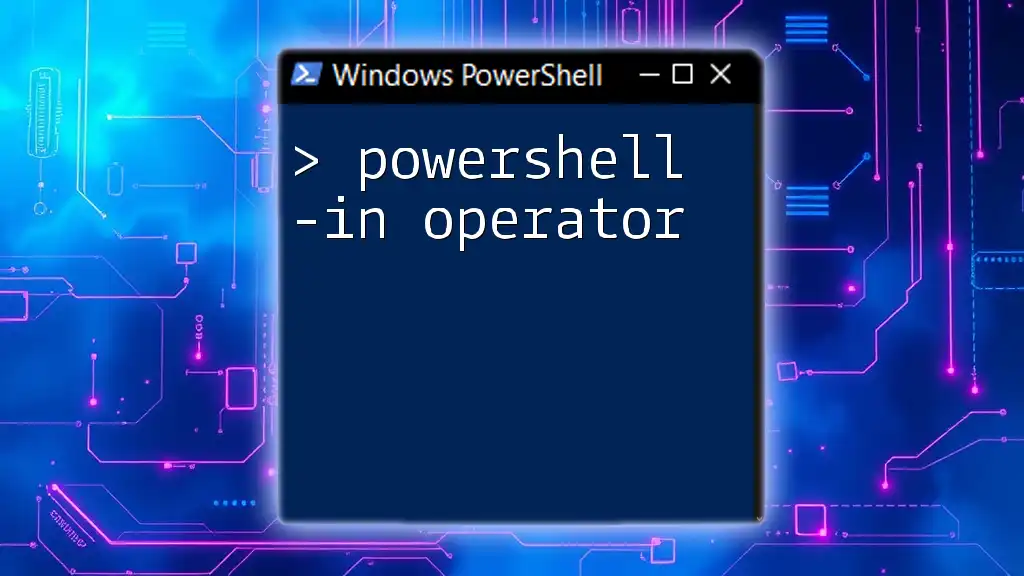
Setting Up Alerts for High Temperatures
Creating Alert Scripts
Setting up alerts is crucial, as it allows you to take action before overheating becomes a problem.
Example: Simple CPU Temperature Alert Script
Consider the following script to create an alert if CPU temperature breaches a specified threshold (e.g., 75°C):
$tempLimit = 75 # Set temperature threshold
$currentTemp = (Get-WmiObject -Namespace root\wmi -Class MSAcpi_ThermalZone).Temperature / 10 - 273.15
if ($currentTemp -gt $tempLimit) {
Write-Host "Alert: CPU temperature is above limit!" -ForegroundColor Red
}
This script fetches the current CPU temperature and displays a warning in red if it exceeds the set limit. You can adjust the `$tempLimit` variable to any temperature that you deem acceptable for your system.
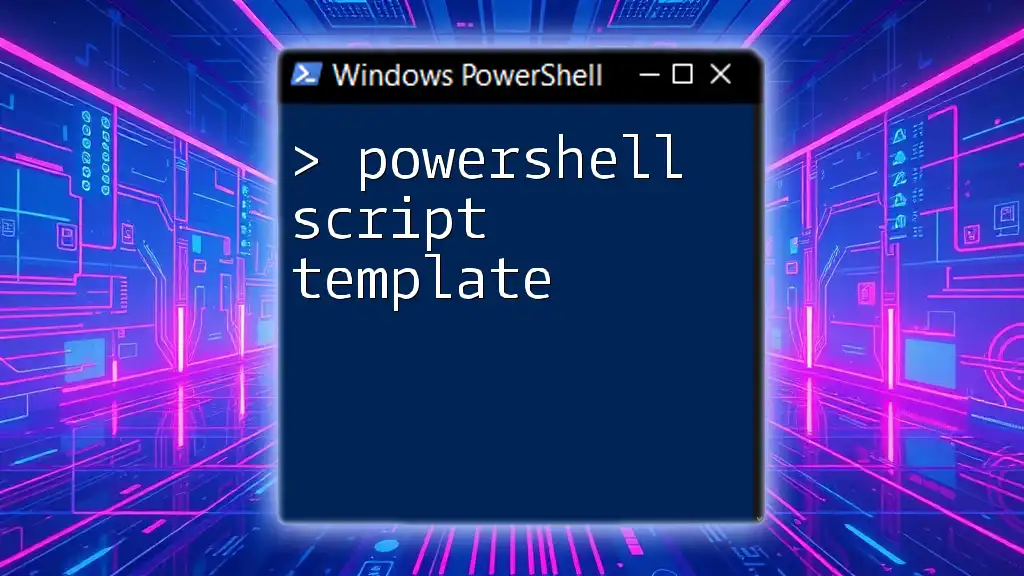
Regular Monitoring and Logging Procedures
Automating Temperature Checks
Regular monitoring can help you stay ahead of any potential issues related to CPU temperature. PowerShell scripts can be scheduled to run periodically.
Example: Create a Scheduled Task for CPU Monitoring
To automate the monitoring process, you can create a scheduled task with the following commands:
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "C:\Path\To\YourScript.ps1"
$trigger = New-ScheduledTaskTrigger -AtStartup
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "CPUTemperatureMonitor"
This command setup ensures your script runs at startup, continually keeping track of your CPU temperature every time your system boots up.
Logging CPU Temperature Data
Setting Up a Log File
Keeping a log of temperature readings can provide valuable insights over time. It allows you to observe trends and identify potential problems based on historical data.
Example: Logging Data to a CSV
You can log CPU temperatures to a CSV file for later analysis with the following script:
$currentTemp = (Get-WmiObject -Namespace root\wmi -Class MSAcpi_ThermalZone).Temperature / 10 - 273.15
$currentTime = Get-Date
$currentTemp | Out-File -FilePath "C:\Path\To\CPU_Temperature_Log.csv" -Append
This script appends the current temperature along with a timestamp to the specified CSV file, enabling easy tracking and analysis of your CPU's temperature history.
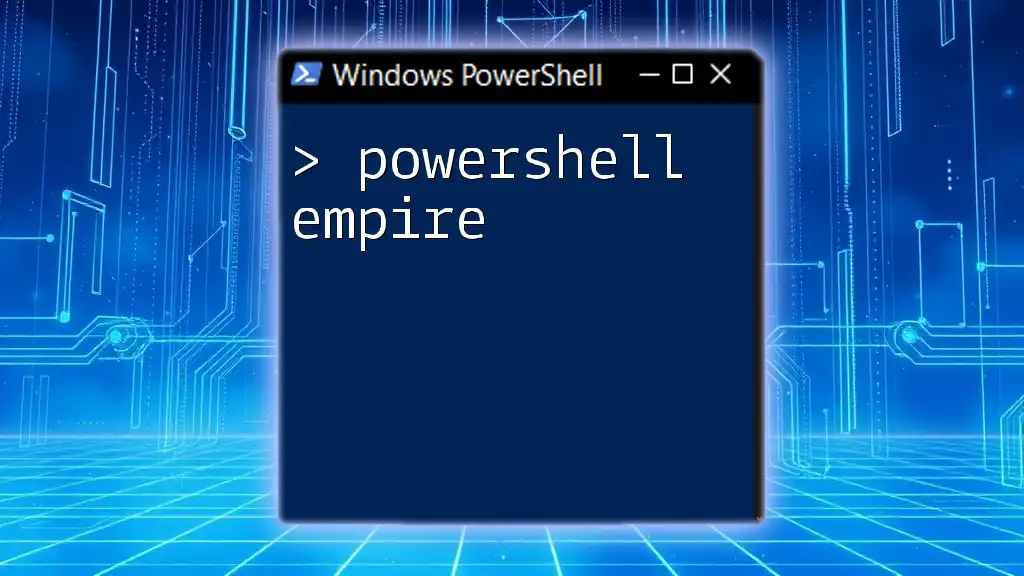
Troubleshooting Common Issues
Common Errors and Solutions
While accessing CPU temperature data with PowerShell, you may encounter several common issues. Some of these include:
- Access Denied: Ensure you have the necessary permissions to run scripts and access WMI.
- Module Not Found: Verify that the required module is installed correctly and that the path to OpenHardwareMonitor is accurate.
- No Data Returned: If no temperature data is returned, check whether OpenHardwareMonitor is running while executing the script.
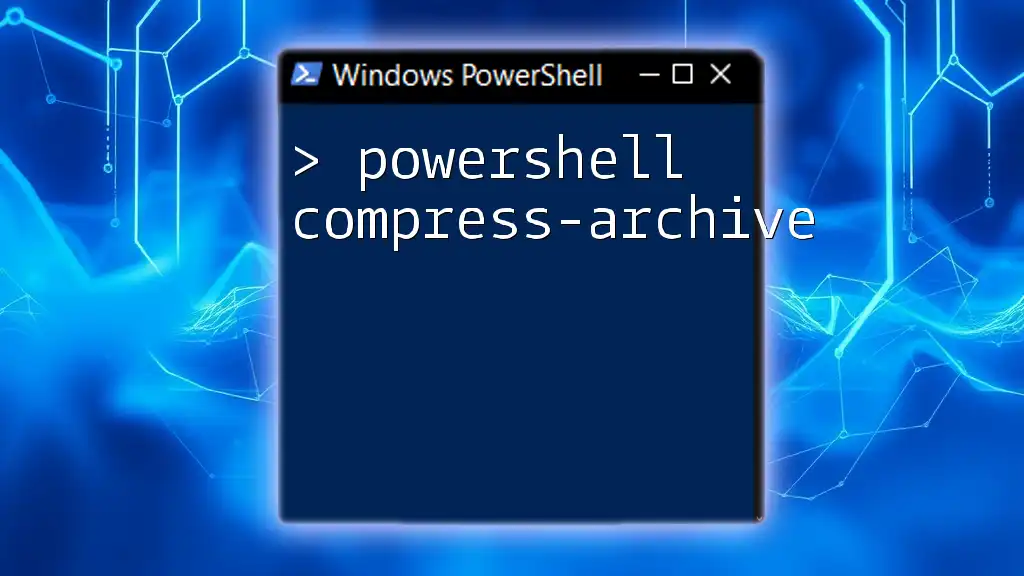
Conclusion
Monitoring CPU temperature with PowerShell not only helps you maintain optimal system performance but also protects your hardware. By regularly utilizing scripts to check CPU temperatures, setting up alerts, and logging data, you can ensure your system runs smoothly and efficiently.
Engaging with your system's health through such proactive measures can contribute to an extended lifespan for your hardware and a more stable computing experience. Embrace the power of PowerShell to take control of your CPU's thermal health.
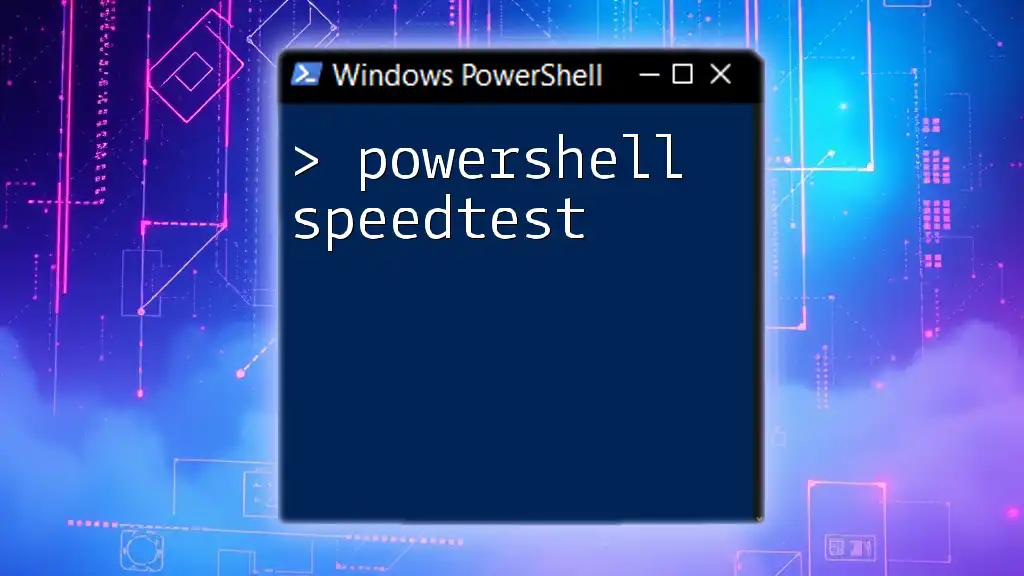
Additional Resources
Recommended Reading
Further your knowledge through various blogs, official documentation, and video tutorials dedicated to mastering PowerShell and hardware monitoring techniques.
Community Engagement
We encourage you to share your experiences, questions, and insights in the comments below. Your contributions could help fellow users enhance their understanding and application of PowerShell for monitoring CPU temperature.