In PowerShell, the `-or` operator is used to combine multiple conditions into a single Boolean expression, evaluating to true if at least one condition is true.
if ($a -eq 1 -or $b -eq 2) {
Write-Host 'One of the conditions is true!'
}
Understanding the PowerShell Or Operator
What is the PowerShell Or Operator?
The PowerShell Or Operator, denoted as `-or`, is a fundamental logical operator that evaluates two Boolean expressions and returns `True` if at least one of the conditions is true. It’s essential for scripting as it allows for the simplification of complex conditional checks, leading to cleaner and more efficient code.
The truthiness concept in PowerShell is crucial here: if at least one condition evaluates to true, the entire expression is regarded as true, allowing for versatile and flexible decision-making in scripts.
How to Use the Or Operator
Using the `-or` operator is straightforward. Its syntax typically involves two conditions that you want to test. The basic usage can be represented as follows:
$condition1 -or $condition2
As you can see, the conditions on either side of the `-or` operator should be valid Boolean expressions, meaning they can resolve to either true or false. Let's delve into a simple example:
$value1 = $true
$value2 = $false
$result = $value1 -or $value2
Write-Output $result # Outputs: True
In this example, although one of the conditions is false, the overall result is true because `$value1` is true. This characteristic is what makes the `-or` operator particularly powerful.
The Logical Flow: When to Use the Or Operator
Appropriate Scenarios for Using `-or`
The `-or` operator shines when you need to check multiple conditions that may independently satisfy the requirements of your script. For example, if you want to verify whether a user is permitted to access a system, you might want to check against multiple usernames or roles.
Real-world applications include configuration checks or filtering actions based on various criteria, making your scripts more adaptable and efficient.
Nested Conditions and Or Operator
One of the strengths of the `-or` operator is its capability to be combined with other logical operators, such as `-and`. This allows for nested conditions that can check multiple criteria simultaneously.
For example:
$color = "Red"
$size = "Small"
if ($color -eq "Blue" -or $size -eq "Small") {
Write-Output "Criteria met."
}
In this scenario, the `-or` operator helps determine if either of the provided conditions is true. As `$size` is indeed "Small," the output here would confirm that the criteria have been met.
Exploring Logical Operators in PowerShell
Overview of PowerShell Logical Operators
PowerShell provides several logical operators, each serving its purpose within scripts. The primary operators are:
- `-and`: Returns true only if both expressions are true.
- `-or`: Returns true if at least one expression is true.
- `-not`: Reverses the Boolean value of an expression.
Understanding the differences between these operators is critical when constructing complex conditions that drive your scripts.
The Power of Combining Operators
Combining logical operators can lead to intricate conditions that yield specific outcomes based on multi-faceted criteria. For an even clearer understanding, consider a visual chart to represent the combination of various logical operators. This can help illustrate how the outputs will vary based on the combinations provided in your condition statements.
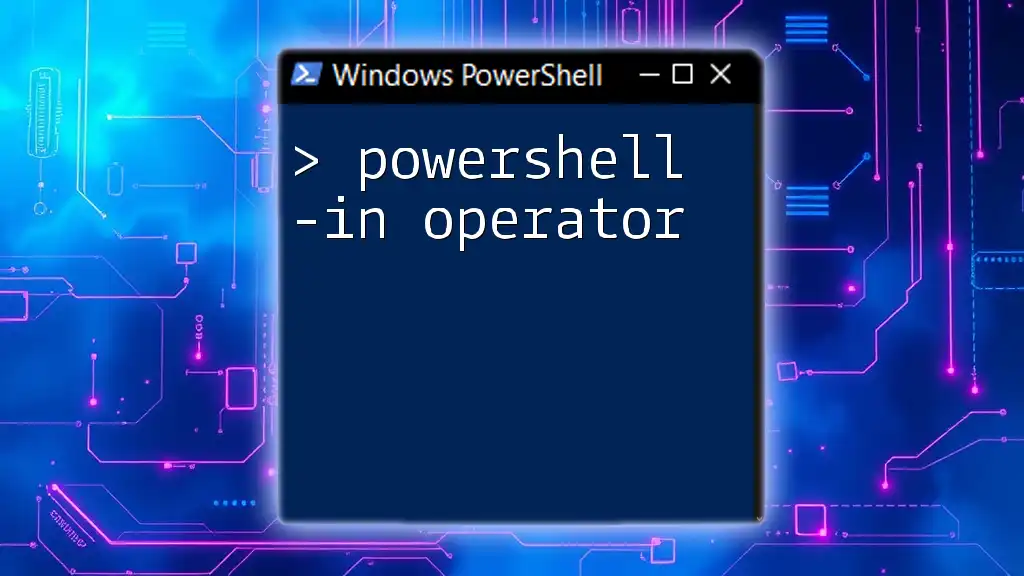
Practical Use Cases for PowerShell Or Operator
System Administration Scripts
The `-or` operator is frequently employed in system management tasks. For instance, when monitoring system configurations or performing batch operations based on user roles or statuses, the `-or` operator becomes indispensable.
Example 1: User Authentication Checks
In authentication scenarios, you often need to validate multiple credentials. The `-or` operator can be efficiently leveraged for this purpose:
$username = "Admin"
$isLocal = $true
if ($username -eq "Admin" -or $isLocal) {
Write-Output "User has admin access."
}
In the above script, the condition checks whether the username matches "Admin" or if the user is a local user. If either condition is satisfied, the user is granted access.
Example 2: File Existence Checks
Another practical use is in verifying the existence of files:
$file1 = "C:\example1.txt"
$file2 = "C:\example2.txt"
if (Test-Path $file1 -or Test-Path $file2) {
Write-Output "At least one file exists."
}
This snippet checks if at least one of the specified files exists on the filesystem, which can be crucial for operations that depend on those files being available.
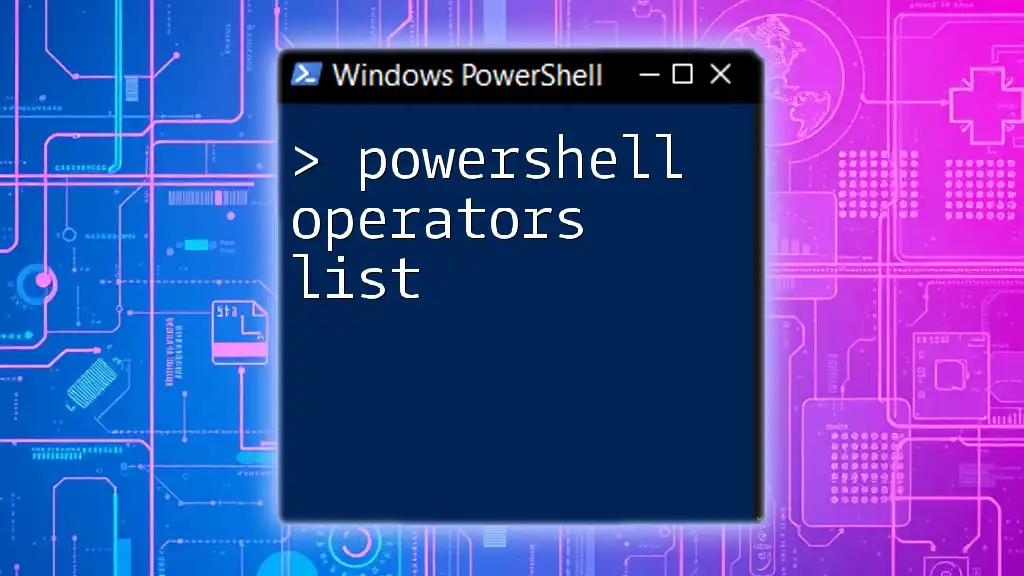
Best Practices for Using the Or Operator
Structuring Conditions for Readability
To ensure that your scripts remain easy to read and maintain, structure your logical conditions appropriately. Using the `-or` operator should not complicate your conditions excessively. Instead, aim for clarity and conciseness. Consider breaking down complex conditions into smaller, more manageable parts.
Performance Considerations
While using `-or` can simplify your conditions, it can also impact script performance if overused or nested excessively. When constructing scripts that may execute repeatedly, ensure your conditions are necessary and optimized to avoid detrimental effects on performance.
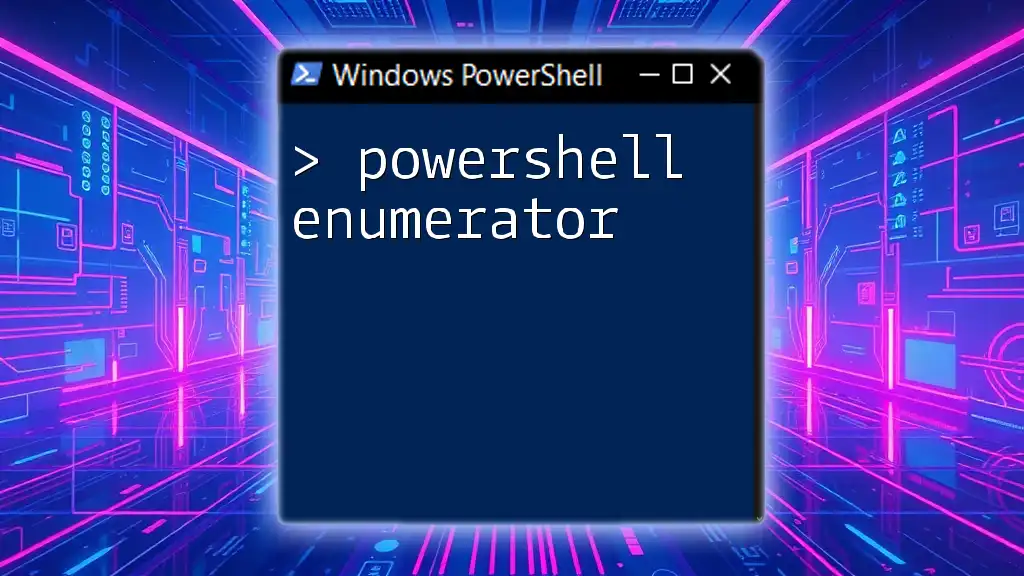
Debugging and Troubleshooting Logical Conditions
Common Pitfalls with the Or Operator
One common mistake is an incorrect assumption of how `-or` evaluates conditions. Remember that if multiple conditions are used, the first true condition will dictate the output, which might lead to unintended results.
Tools and Techniques for Debugging
Using `Write-Output` strategically can significantly aid in debugging your scripts. This function helps you verify what values are being returned at each condition and locate where errors may arise. Testing each part of your conditions at runtime can clarify misunderstandings or correctness.
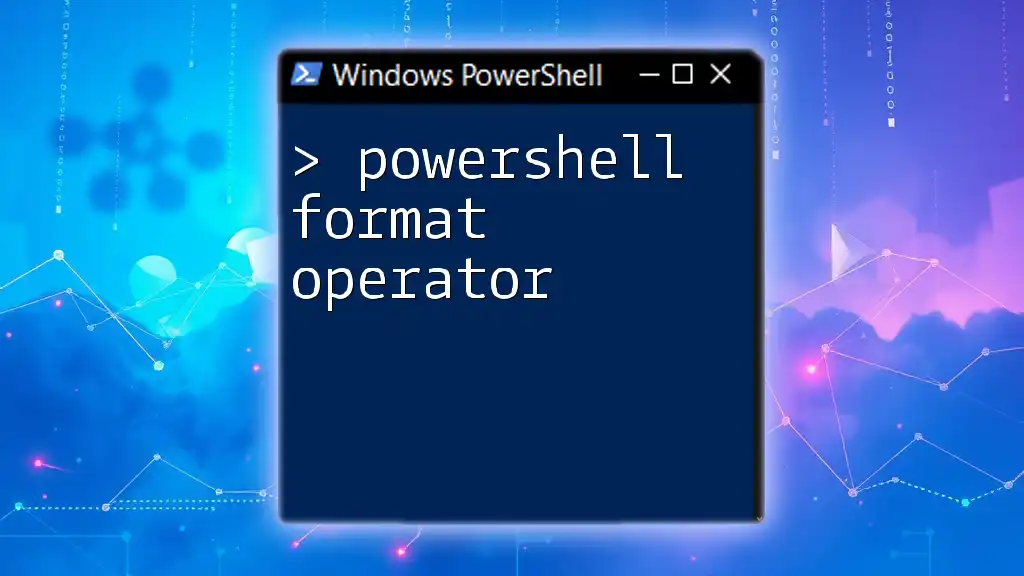
Conclusion
In conclusion, mastering the PowerShell Or Operator equips you with a powerful tool for scripting efficiency and effectiveness. Understanding its usage, as well as the scenarios where it applies, allows for more dynamic and responsive scripts. As you refine your scripting abilities, practice using the `-or` operator in various conditions to uncover its full potential. Embrace the opportunity to delve deeper into PowerShell and explore the many facets that enhance your skills in automation and system management.
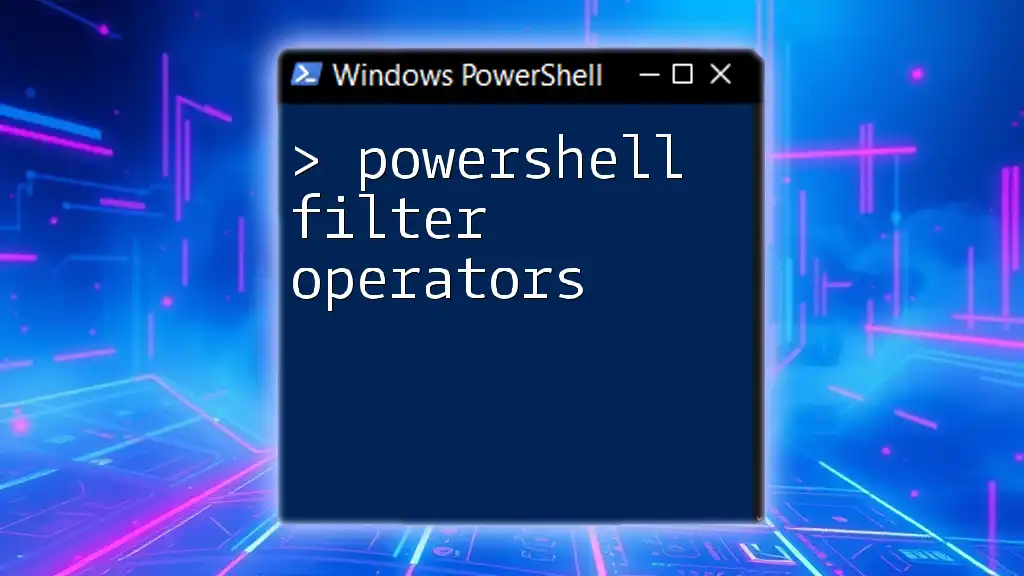
Additional Resources
For further reading on PowerShell operators and best practices, be sure to check out official PowerShell documentation and explore online courses that cover advanced scripting techniques. Continuous learning will enhance your proficiency and enable you to tackle increasingly complex tasks within PowerShell.