The `-like` operator in PowerShell allows you to compare a string against a pattern using wildcard characters, facilitating flexible string matching.
# Sample usage of the -like operator
$greeting = "Hello, World!"
if ($greeting -like "Hello*") {
Write-Host 'The greeting starts with "Hello".'
}
What is the -like Operator?
The -like operator in PowerShell serves as a powerful tool for matching strings. This operator is widely used when you need to compare a string to a pattern that may include wildcard characters, making it versatile for various scripting scenarios.
Understanding how to effectively utilize the -like operator can significantly enhance your scripting efficiency and data querying capabilities within PowerShell.

Use Cases of the -like Operator
The -like operator is particularly valuable in several scenarios, such as:
- Filtering Data: You can use it to filter object properties in cmdlets like `Get-ChildItem`, `Get-Command`, and more.
- Dynamic String Matching: It allows you to match patterns dynamically within scripts, enabling conditions that dictate flow based on string content.

Understanding Wildcards in PowerShell
What are Wildcards?
Wildcards are special characters that represent one or more characters, providing more flexible pattern matching. In PowerShell, two primary wildcards are prevalent:
- `*` (Asterisk): Matches zero or more characters. For example, `abc*` will match any string starting with `abc` and can be followed by anything (or nothing).
- `?` (Question Mark): Matches exactly one character. For instance, `file?.txt` would match `file1.txt` but not `file11.txt`.
Common Wildcard Examples
Using wildcards effectively allows you to pattern-match strings intuitively:
- `report` would match any string containing the word "report".
- `test?` could match `test1` or `testA`, but not `test` or `test12`.

Syntax of the -like Operator
Basic Structure
The basic syntax for the -like operator is straightforward:
string -like "pattern"
Case Sensitivity
One critical aspect of the -like operator is its case sensitivity. By default, the -like operator is not case-sensitive, contrasting with the -eq (equals) operator, which is case-sensitive. This can lead to unexpected results if you expect a case-sensitive match.
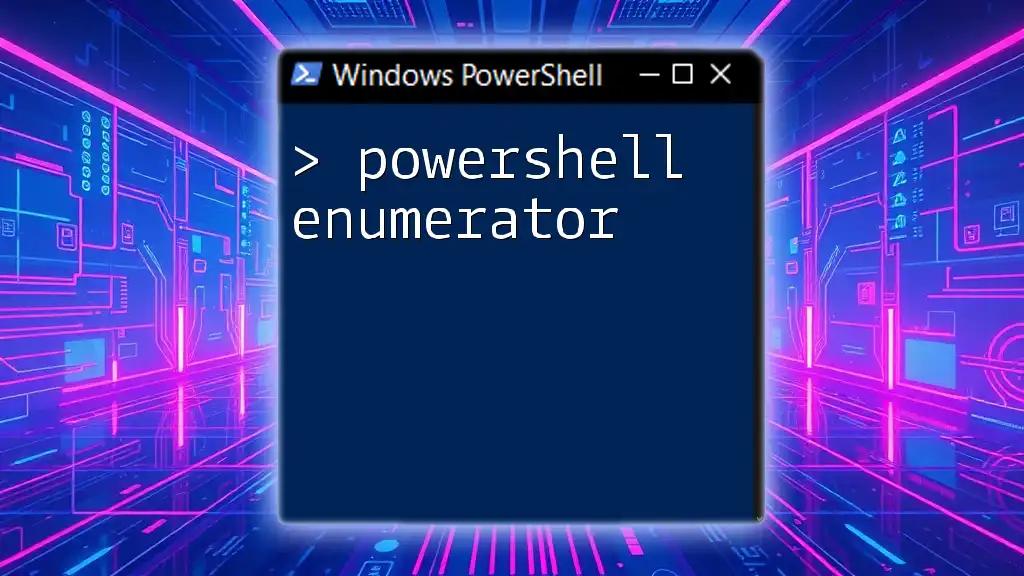
Practical Examples of Using -like
Simple String Matching
A fundamental usage of the -like operator is matching simple strings. For instance, if you want to check if a string starts with "Hello":
$string = "Hello World"
if ($string -like "Hello*") {
"Match found!"
}
In this example, the returned output would be "Match found!" because the string starts with "Hello".
Using -like with Variables
The -like operator can also be employed with variables for more dynamic checks. For instance:
$name = "JohnDoe"
if ($name -like "John*") {
"Matched name!"
}
Here, since `$name` starts with "John", you would see the output "Matched name!".
Multiple Conditions with -like
You can create more complex queries by combining the -like operator with logical operators like -and and -or. For example:
$userName = "JaneDoe"
if ($userName -like "Jane*" -or $userName -like "*Doe") {
"User matches!"
}
In this combination, if either condition is true, the output would read "User matches!".

Filtering Data with Get-Command
Basic Filtering Example
You often want to filter commands based on patterns. The -like operator shines here with `Get-Command`:
Get-Command -Name "*Item*"
This command will return all commands whose names contain "Item", demonstrating how to leverage the -like operator for effective filtering.
Advanced Filtering Techniques
Using -like in Arrays
Another practical use is filtering arrays. For example:
$users = @("John", "JaneDoe", "Alice")
$filteredUsers = $users | Where-Object { $_ -like "*Doe" }
In this example, the filtered output would include only the name "JaneDoe" because it matches the condition specified.

Performance Considerations
Speed of -like Operator
While the -like operator is versatile, it can be less efficient than certain other operators when dealing with large datasets. It would be beneficial to assess your scenario and consider if a more specific or optimized approach would yield better performance.
When to Avoid -like
There are specific situations where you might want to avoid using the -like operator. If your string comparisons require exact matches, it is often better to use the -eq or -ieq (case-insensitive) operators as they can provide faster, clearer results without the ambiguity of wildcards.

Troubleshooting Common Issues
Common Errors When Using -like
A typical pitfall with the -like operator is misunderstanding how wildcards work. Users may expect that a wildcard should behave in a specific, possibly intuitive way, leading to confusion over matching results.
Debugging Tips
If your script isn’t returning expected results, follow these practices:
- Check your Wildcards: Ensure that you are using wildcards correctly. Try changing your pattern and re-test.
- Look at Case Sensitivity: Remember that -like is case-insensitive, while other comparison operators are not.
- Utilize Write-Host for Debugging: Print out variables and matches as you go to help clarify what’s happening within your conditions.
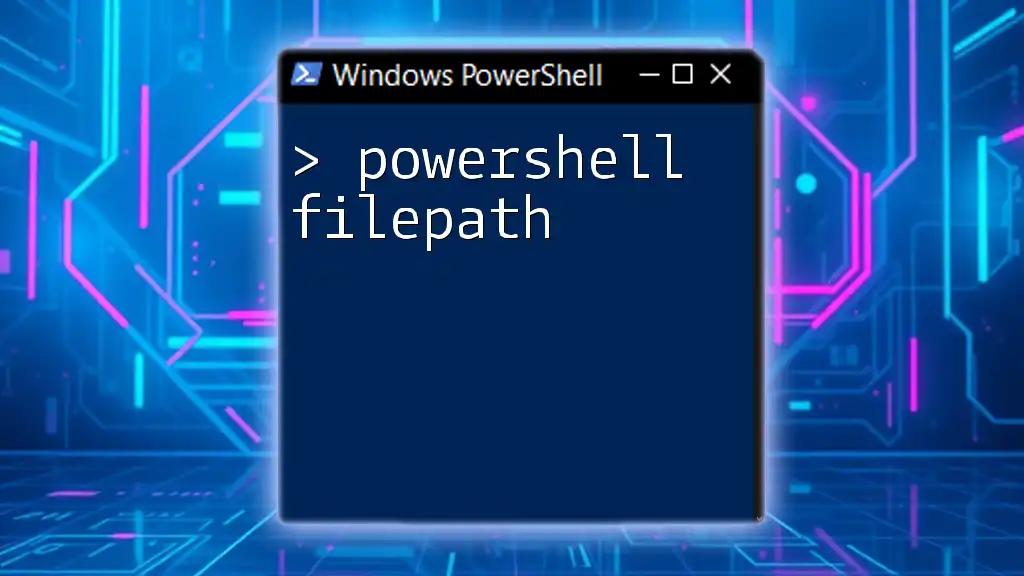
Conclusion
In summary, the PowerShell -like operator is an invaluable tool for string matching and data filtering. Its ability to incorporate wildcards provides a robust method for dynamic scripting. Understanding when and how to use it effectively can significantly enhance your PowerShell scripting capabilities.
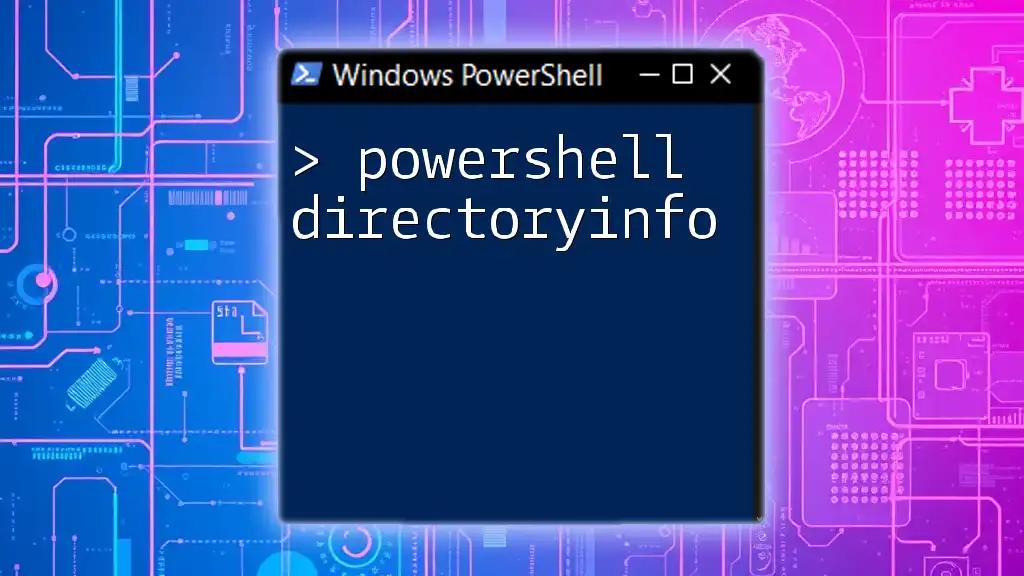
Further Reading and Resources
For those keen on deepening their knowledge of the PowerShell -like operator and string manipulation, consider exploring advanced resources such as books on PowerShell scripting or reputable online courses that focus on practical PowerShell applications.
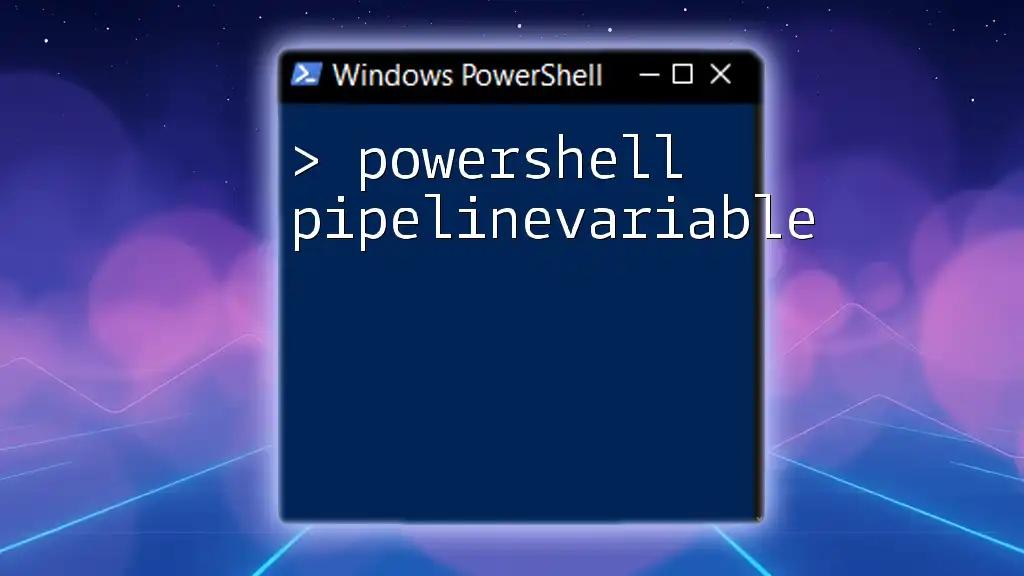
Join Our Community
Don’t forget to join our community for more tips, tricks, and comprehensive guides on using PowerShell effectively in your daily tasks. Embrace the power of automation with mastery over your commands!