In PowerShell, the `-like` operator is used for pattern matching with wildcard characters, allowing you to filter strings, while the `-and` operator combines multiple conditions to evaluate to true only if both conditions are true.
Here's a code snippet demonstrating how to use both operators:
$names = @("Alice", "Bob", "Charlie", "David")
$filteredNames = $names | Where-Object { $_ -like "A*" -and $_ -ne "Alice" }
Write-Host $filteredNames
Understanding the `-like` Operator
What is the `-like` Operator?
The `-like` operator in PowerShell is used for performing pattern matching on string values. It allows you to compare a string with a wildcard pattern, making it extremely useful for filtering data. Unlike the `-eq` (equals) operator that checks for exact matches, `-like` can match patterns with flexibility.
Wildcards in PowerShell
In PowerShell, wildcards are special characters that enable you to define more general search criteria:
- `*` (Asterisk): Represents zero or more characters. For example, `Hello*` will match any string that starts with "Hello".
- `?` (Question Mark): Represents a single character. For instance, `H?llo` would match "Hello", "Hallo", or "Jello".
Example:
"Hello World" -like "Hello*" # Returns True
"Hello World" -like "H?llo*" # Returns True
Practical Applications of the `-like` Operator
Filtering Objects in a Collection
One of the most common uses of the `-like` operator is filtering properties of objects in a collection. You can easily filter processes, services, or files based on their names.
Example:
Get-Process | Where-Object { $_.ProcessName -like "s*" }
This command will list all processes where the name begins with "s".
Searching Text
The `-like` operator can also be useful for searching text within strings. If you want to check if a certain word or phrase exists in a string, `-like` can accomplish this task effectively.
Example:
"The quick brown fox" -like "*brown*" # Returns True
In this case, the check confirms that the string contains the substring "brown".

Understanding the `-and` Operator
What is the `-and` Operator?
The `-and` operator in PowerShell is used to evaluate multiple conditions and requires all conditions to be true for the combined statement to return true. This logical operator plays a crucial role when you need to filter results based on multiple criteria.
Logical Operations in PowerShell
PowerShell supports various logical operators, including `-or` and `-not`, providing flexibility in forming conditional statements. Understanding how to combine these operators allows for nuanced logical operations in your scripts.
Practical Applications of the `-and` Operator
Combining Conditions in Filtering
When filtering data, the `-and` operator enables you to specify multiple conditions that must be satisfied. This is particularly useful when querying datasets that require precision.
Example:
Get-Process | Where-Object { $_.CPU -gt 10 -and $_.ProcessName -like "w*" }
In this command, the script filters processes that are using more than 10 CPU and whose names start with "w".
Complex Conditional Statements
You may often find yourself needing to create complex logical statements that require multiple evaluations. The `-and` operator helps ensure that all specified conditions must be true before actions are executed.
Example:
if ($a -gt 5 -and $b -lt 10) {
"Condition met."
}
Here, the message "Condition met." will only display if both `$a` is greater than 5 and `$b` is less than 10.

Combining `-like` and `-and` in PowerShell Scripts
Creating Complex Queries
Combining the `-like` and `-and` operators can greatly enhance your ability to filter data based on multiple string patterns and logical conditions.
Example:
Get-Service | Where-Object { $_.Status -eq "Running" -and $_.Name -like "*Firewall*" }
This command retrieves services that are both running and have "Firewall" in their names. The power of the combination provides a refined focus on the desired data.
Real-World Use Case
In a real-world scenario like querying Active Directory, the combination of `-like` and `-and` elevates your querying capabilities, allowing for specific and relevant results.
Example:
Get-ADUser -Filter { Enabled -eq $true -and Name -like "J*" }
This command fetches all Active Directory users who are enabled and have names starting with "J". This combination is particularly valuable in environments with numerous users.
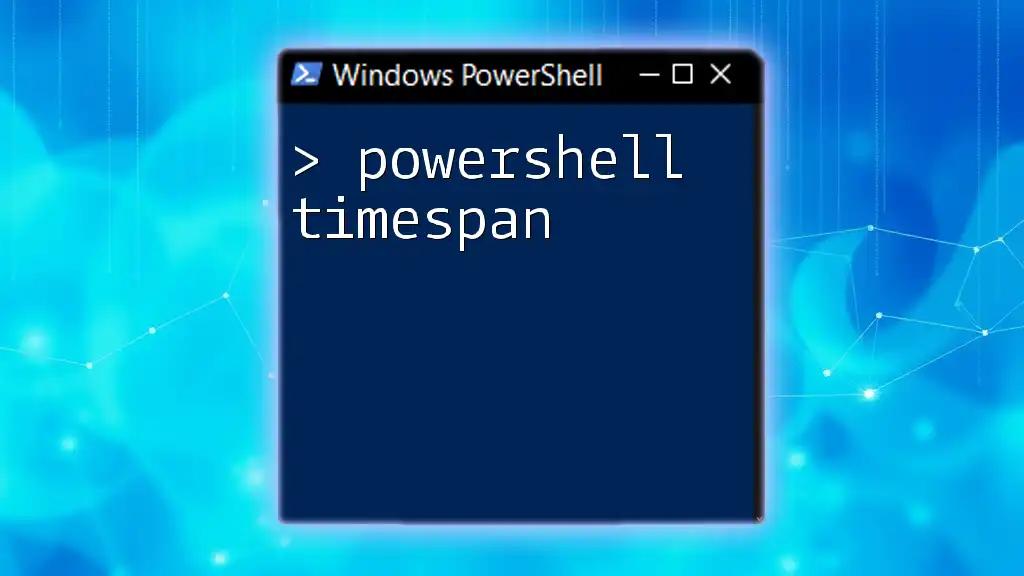
Best Practices for Using `-like` and `-and`
Clarity and Readability
When writing scripts that involve `-like` and `-and`, clarity is essential. Ensure that your commands are legible and easily understandable. Use descriptive variable names and break long expressions into smaller parts, utilizing whitespace and comments to improve readability.
Performance Considerations
While combining conditions can provide precise results, it’s vital to be mindful of performance, especially in large datasets. Optimize your queries where possible and test your scripts on smaller datasets to gauge performance before scaling up.
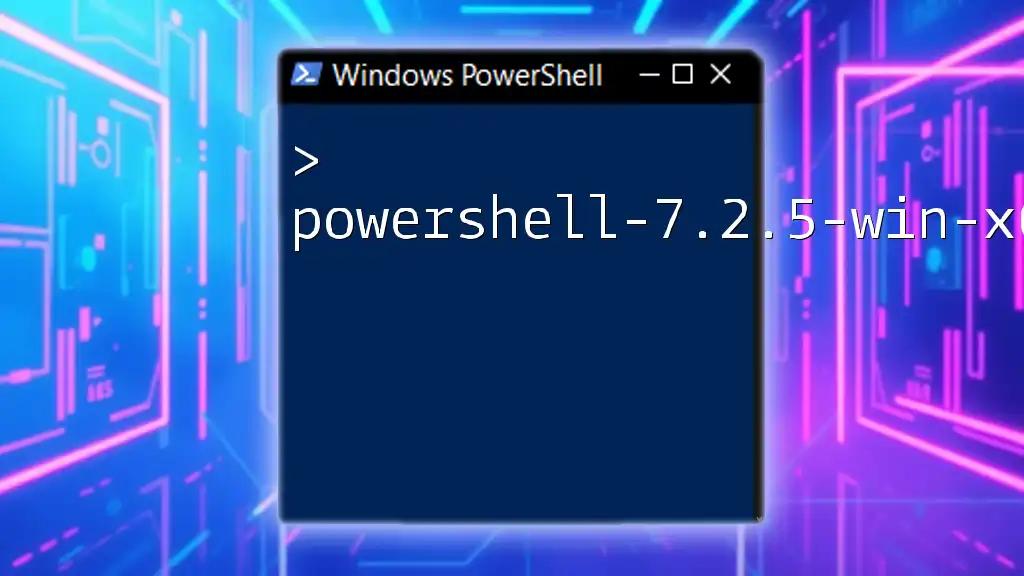
Troubleshooting Common Issues
Common Mistakes
When first using `-like` and `-and`, beginners may run into pitfalls, such as incorrectly escaping special characters in wildcards or misapplying logical evaluations. These mistakes can lead to unexpected results or errors in script execution.
Debugging Techniques
Validating your queries and debugging scripts can save you from hours of frustration. Use `Write-Host` or similar commands to output messages for verification, providing clarity on what conditions are being met in your code.
Example:
Write-Host "Checking conditions: a= $a, b= $b"
This simple output can help you understand variable states during execution.

Conclusion
To sum up, understanding and effectively using the `-like` and `-and` operators in PowerShell is a significant skill. These operators enhance your capability to create filters and write logical expressions that are both powerful and precise. Practice using the examples provided, and you will become proficient in deploying these operators in your PowerShell scripts.
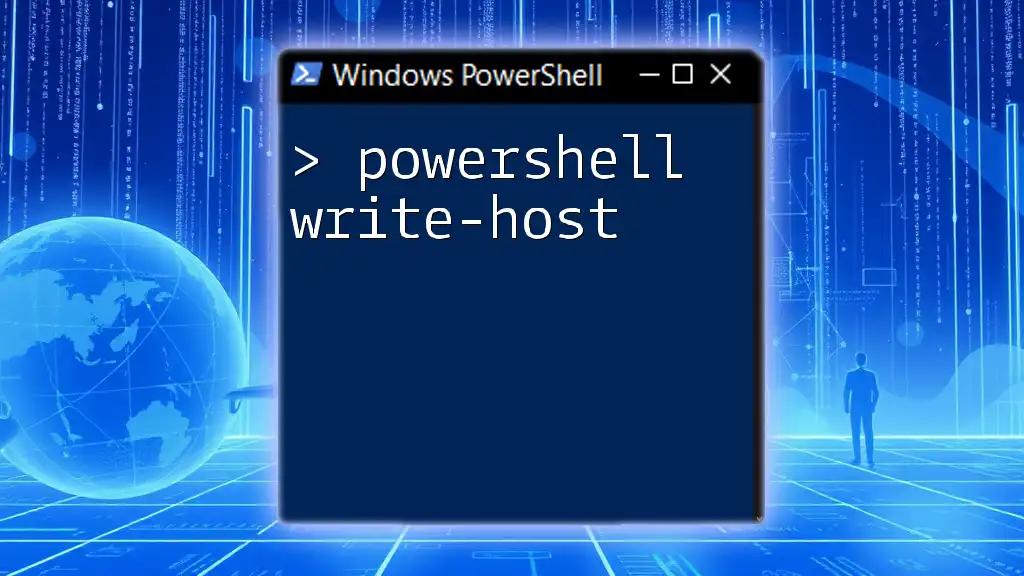
Call-to-Action
For more insights and advanced PowerShell techniques, don’t hesitate to subscribe for upcoming courses and webinars designed to elevate your scripting skills. Your journey towards mastering PowerShell begins here!