In PowerShell, the `if` statement combined with the `-and` operator allows you to execute a block of code only when multiple conditions are true. Here's a simple example:
if ($a -gt 10 -and $b -lt 20) {
Write-Host 'Both conditions are met!'
}
Understanding PowerShell Conditional Statements
What are Conditional Statements?
Conditional statements are an essential aspect of programming, allowing scripts to execute different blocks of code based on specific conditions. In PowerShell, the primary conditional statement is the `if` statement.
These statements help control the flow of execution, determining which actions to perform as the script runs. Mastering these conditions empowers you to write flexible and dynamic scripts that adapt to varying situations.
The Basics of PowerShell If Statement
The syntax of the `if` statement in PowerShell is straightforward:
if (condition) {
# Code to execute if condition is true
}
Key Points:
- Condition: This is an expression that evaluates to either true (`$true`) or false (`$false`).
- Execution Block: The code inside the braces executes only if the condition evaluates to true.
For instance, a basic `if` statement checking whether a variable is equal to a value might look like this:
$temperature = 30
if ($temperature -gt 25) {
Write-Host "It's warm outside."
}
In this example, the message "It's warm outside." only appears if the temperature exceeds 25 degrees, demonstrating the simplicity and power of conditional statements in PowerShell.
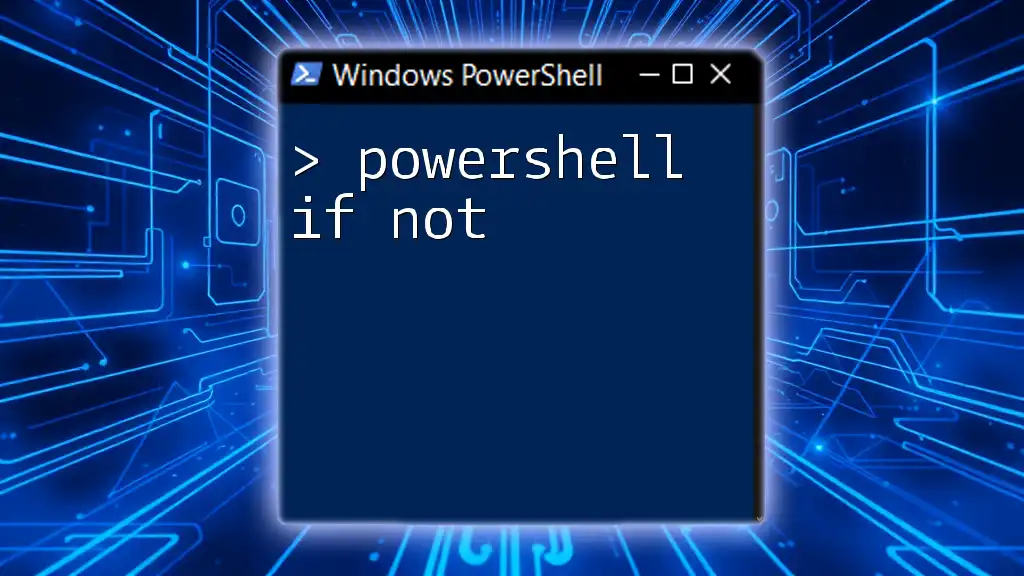
Exploring the If and Operator in PowerShell
What is the If and Operator?
The `and` logical operator allows you to evaluate multiple conditions, ensuring that all specified conditions must be true for the execution block to run. This operator is vital when you need to apply multiple checks simultaneously.
Syntax and Structure
The proper syntax to use the `if` statement combined with the `and` operator is as follows:
if ($condition1 -and $condition2) {
# Code to execute if both conditions are true
}
This structure allows for a powerful combination of checks.
Practical Examples of If and
Consider the following example where we check if a person is both an adult and a student:
$age = 20
$isStudent = $true
if ($age -ge 18 -and $isStudent) {
Write-Host "You are an adult and a student."
}
In this code snippet:
- We check if the person is at least 18 years old and also a student.
- Both conditions must evaluate as true for the message to be displayed.
Another real-world example is user authentication:
$username = "admin"
$password = "password123"
if ($username -eq "admin" -and $password -eq "password123") {
Write-Host "Access granted."
}
This demonstrates how the `and` operator ensures that both the username and password must match for access to be granted, emphasizing security in scripts.
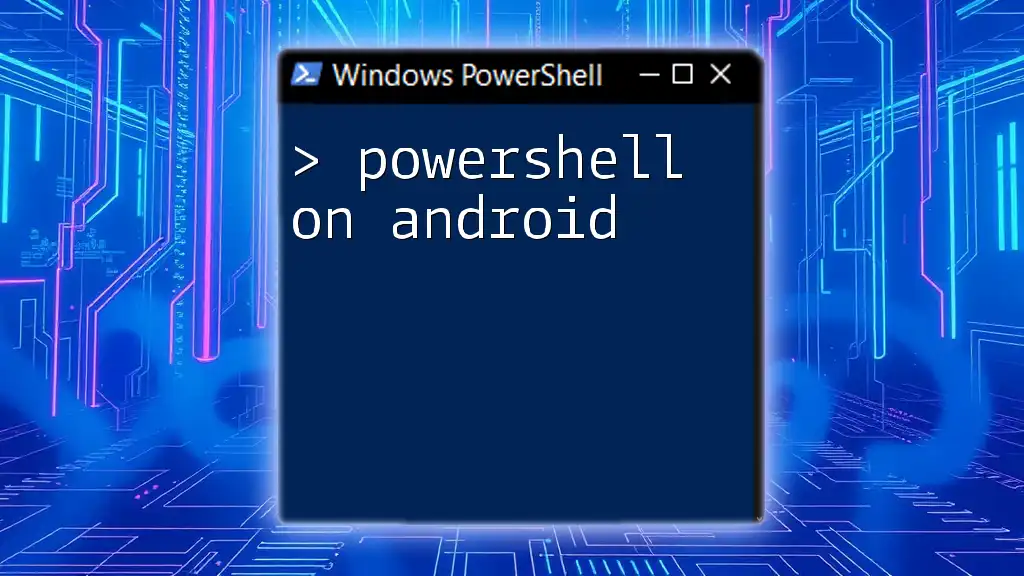
Combining If and Or in PowerShell
Introduction to If Or Operator
The `or` logical operator is another powerful tool, allowing execution to occur if at least one of the specified conditions is true. It is especially useful in situations where multiple possibilities can lead to the same outcome.
Syntax and Structure
The syntax for combining the `if` statement with the `or` operator is similar to that of `and`:
if ($condition1 -or $condition2) {
# Code to execute if at least one condition is true
}
This allows for flexible scripting possibilities.
Combining If with And and Or
To further illustrate, you can combine both the `and` and `or` operators in a single statement. Here’s an example:
$isAdmin = $false
$isGuest = $true
if ($isAdmin -or $isGuest -and $age -lt 30) {
Write-Host "Welcome, you have limited access."
}
In this scenario, access is granted if the user is either an admin or a guest under 30 years old, providing a nuanced approach to condition evaluation.
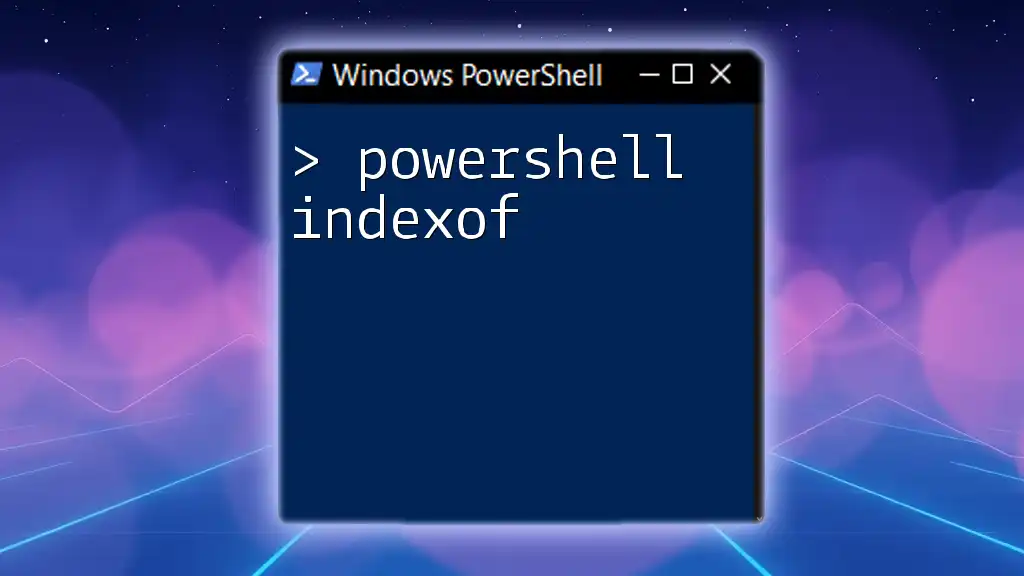
Use Cases for If and, If or, and their Combinations
When to Use If and
Utilizing `if and` is critical in scenarios where it is essential for both conditions to be met. For example, when validating input data or triggering functions based on multiple criteria.
When to Use If or
In contrast, using `if or` is beneficial when any of the provided conditions can lead to a successful outcome. This is particularly useful in processes like user input verification, where multiple valid inputs can exist.
Combining Both for Complex Conditions
A well-designed script may require complex conditions combining both `and` and `or`. For instance, this could apply to permission checks in a software application, enabling various user roles access based on specific conditions.
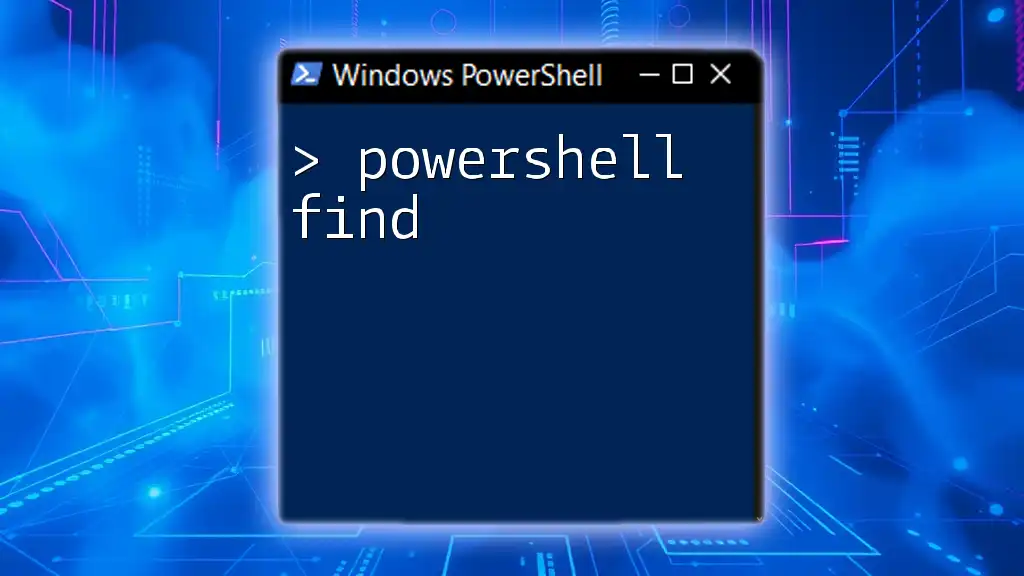
Best Practices for Writing If Statements in PowerShell
Keep It Simple
Simplicity is key to effective scripting. Avoid excessive complexity in your conditional logic, which may confuse readers (including future you). Each condition should have a specific purpose that’s clear and easy to follow.
Commenting Your Code
Using comments effectively can significantly enhance the readability of your scripts. By briefly explaining what each condition checks for, you help others who might read your script, including yourself in the future, understand the intention behind your logic.
Testing and Debugging Conditional Statements
Testing your conditional statements is crucial. Ensure you cover all possible outcomes for your conditions, checking both true and false scenarios. Use tools like `Write-Host` to output intermediary results during execution, which can assist in debugging:
if ($username -eq "admin") {
Write-Host "Username is admin."
} else {
Write-Host "Username is not admin."
}
This practice helps you ensure that your conditions behave as expected, simplifying the troubleshooting process.
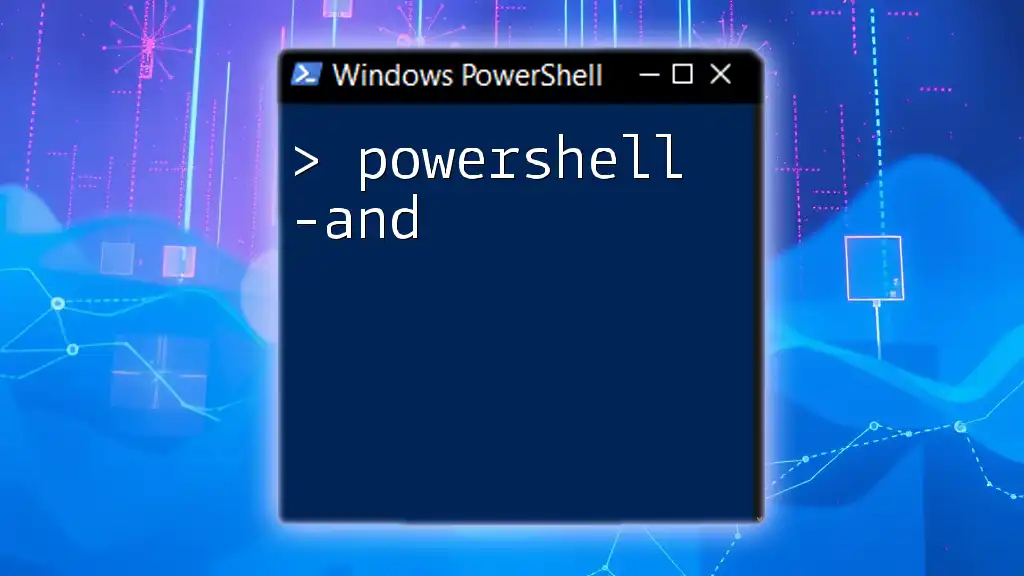
Conclusion
In summary, PowerShell's `if`, `and`, and `or` operators are foundational components that allow for powerful and flexible scripting. By mastering these conditional statements, you can create dynamic scripts that respond intelligently to various situations, greatly enhancing your scripting capabilities.
With practice and the concepts explored in this article, you’ll be well on your way to writing efficient and effective PowerShell scripts that can handle complex conditions seamlessly.