In PowerShell, the "if not" condition can be expressed using the `-not` operator to execute code when a specified condition evaluates to false.
if (-not $condition) {
Write-Host 'Condition is false!'
}
What is PowerShell Conditional Logic?
Definition of Conditional Statements
Conditional statements are fundamental to programming, allowing scripts to execute different actions based on specified conditions. They form the backbone of decision-making in scripts, enabling automation and control flow. In PowerShell, conditional statements are primarily handled by the if, else, and switch constructs.
Importance of the "If" Statement
The if statement provides the basic mechanism for controlling flow based on logical conditions. It evaluates a condition and executes a block of code if the condition is true. Understanding this concept is crucial for effective scripting in PowerShell. The general syntax for an if statement is as follows:
if (condition) {
# Code to execute if condition is true
}
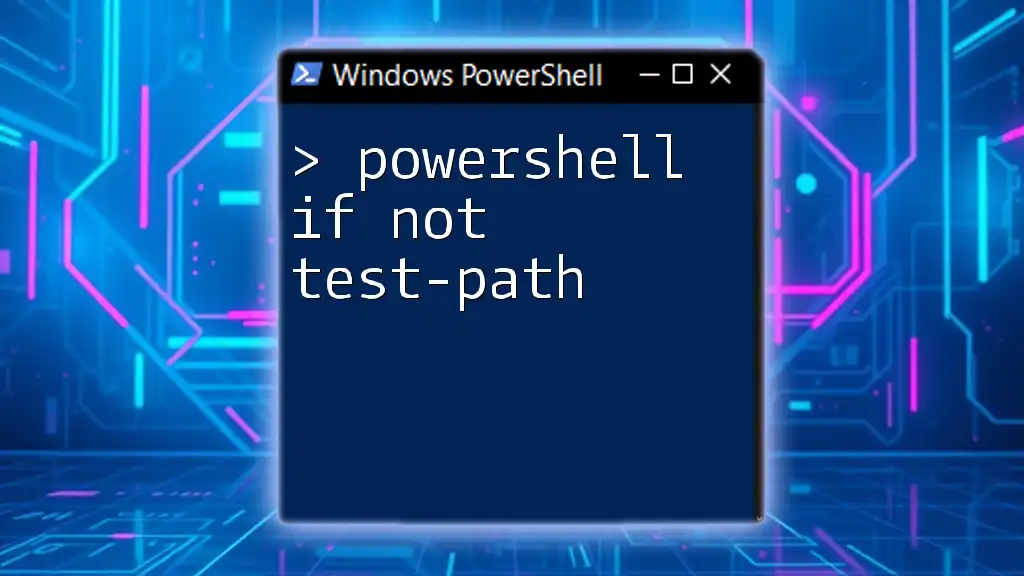
The Power of "If Not" in PowerShell
What Does "If Not" Mean?
The "if not" logic provides a means to execute code when certain conditions are false. This can prove essential when validating states or checking for the non-existence of elements. Using if not allows for cleaner and more readable scripts, focusing on scenarios where something should not happen.
Syntax of "If Not" in PowerShell
The basic syntax when utilizing "if not" in PowerShell is crafted using the `-not` operator. This operator inverts the outcome of a boolean condition. Here’s an example demonstrating this concept:
if (-not (Test-Path "C:\example.txt")) {
Write-Host "The file does not exist."
}
In this example, the script checks whether a specific file exists. If it does not, the message "The file does not exist" is displayed. This syntax is straightforward and effective for checking negative conditions.
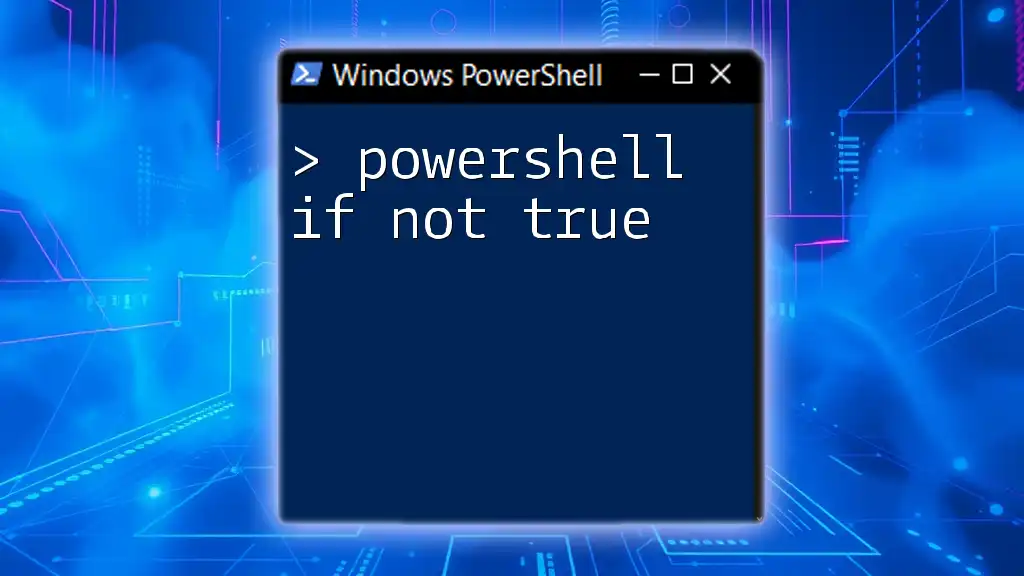
How to Implement "If Not" in Your Scripts
Using "If Not" with Boolean Conditions
Boolean conditions play a critical role in conditional logic. In PowerShell, you can easily evaluate true or false states. The following snippet shows how to leverage if not with a boolean parameter:
$condition = $false
if (-not $condition) {
Write-Host "Condition is false."
}
Here, since `$condition` evaluates to false, the message "Condition is false" will be printed. This is particularly useful in scenarios involving validation checks where the expected outcomes may cause further actions.
Incorporating "If Not" with PowerShell Cmdlets
Employing "if not" alongside common cmdlets allows more granular control over your scripts. For example, if you want to check if a service is not running, you can use:
if (-not (Get-Service -Name "wuauserv" -ErrorAction SilentlyContinue)) {
Write-Host "Windows Update service is not running."
}
In this snippet, the script checks the status of the Windows Update service. If the service is not found or is not running, it will print the corresponding message. This creates robust checks within your automation tasks.
Complex "If Not" Statements
To handle multiple conditions with "if not," you may need to combine logical operators such as `-and` and `-or`. This allows the script to manage complex decision trees effectively. For instance:
if (-not ($condition1 -and $condition2)) {
Write-Host "At least one of the conditions is false."
}
This example evaluates two conditions. If either `condition1` or `condition2` is false, the output will indicate one or both of the conditions have failed.
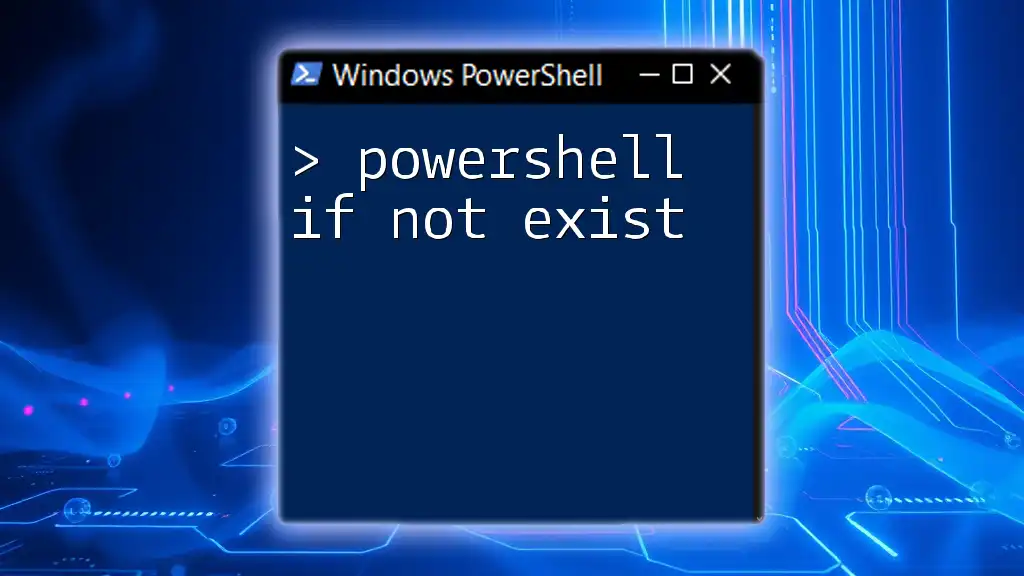
Error Handling with "If Not"
Using "If Not" for Error Checking
Error handling is a crucial aspect of scripting to manage unexpected behavior. PowerShell provides mechanisms for capturing errors through try/catch blocks. Using "if not" with error checks allows for more resilient scripts. Consider the following example:
try {
# Code that may throw an error
Get-Content "C:\nonexistentfile.txt"
} catch {
if (-not $_.Exception) {
Write-Host "An error occurred, but it's critically handled."
}
}
In this scenario, if the `Get-Content` command fails due to the file not being found, the catch block executes, and the message is printed. Using if not here ensures the error is caught and managed gracefully.
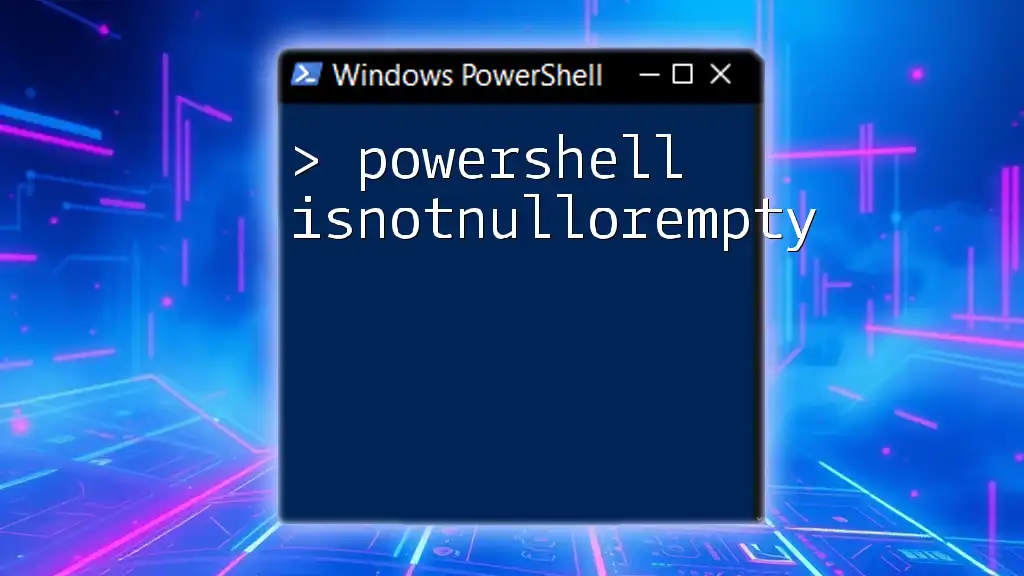
Best Practices for Using "If Not" in PowerShell
Keep It Simple
Simplicity is key when writing scripts. As scripts grow in complexity, ensuring that your conditional statements remain clear can help with maintainability. Focus on using straightforward logic that is easy to read and understand. Overly complicated conditions can lead to errors and confusion.
Consistent Messaging
Providing clear and user-friendly messaging within your "if not" statements enhances the usability of your scripts. Consider the following example:
$filePath = "C:\example.txt"
if (-not (Test-Path $filePath)) {
Write-Host "File not found: $filePath"
}
Here, the output directly informs the user about the specific file's status, which is far more helpful than simply stating whether the file exists or not.
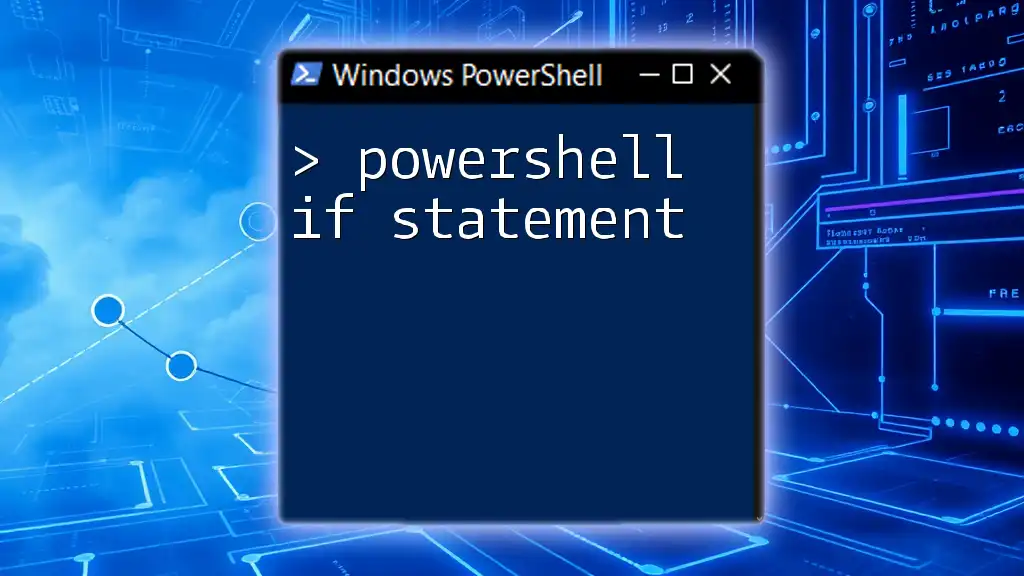
Common Mistakes to Avoid
Forgetting the Parentheses
One prevalent mistake in PowerShell scripting involves neglecting to use parentheses in conditional statements. This can lead to unexpected outcomes. Always ensure proper syntax, like this:
if (-not $condition) {
# Correct usage
}
Overcomplicating Conditions
Avoid constructing overly nested or convoluted conditions. Such complexity makes scripts difficult to understand and maintain. Strive for a balance between comprehensive logic and clarity.
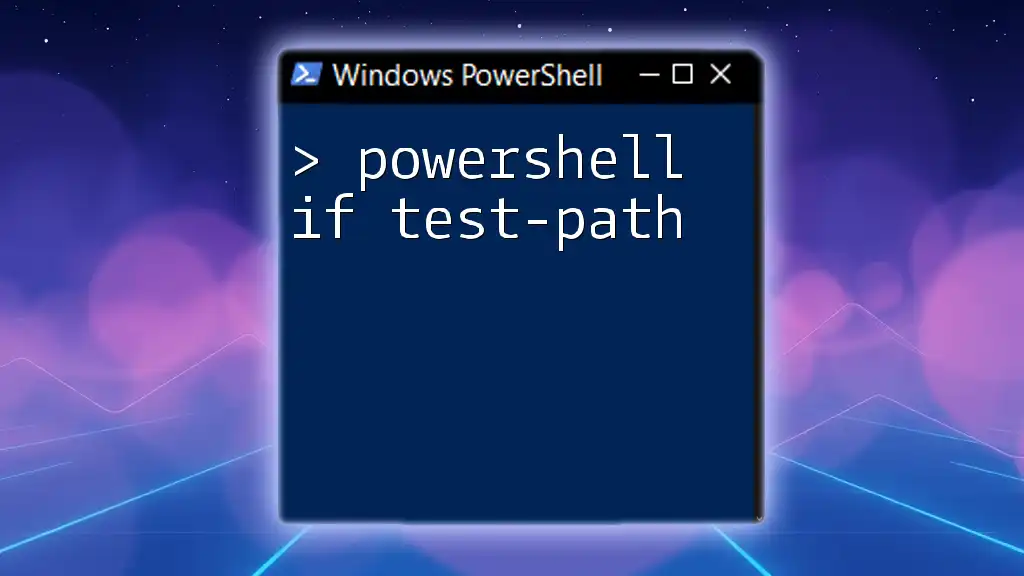
Conclusion
The concept of "powershell if not" serves as a powerful tool for managing conditional logic in PowerShell scripting. By utilizing if not, you can craft scripts that respond to negative conditions effectively and enhance the readability of your scripts. As you grow in your scripting journey, continue to experiment with the if not logic to develop more efficient and maintainable code.
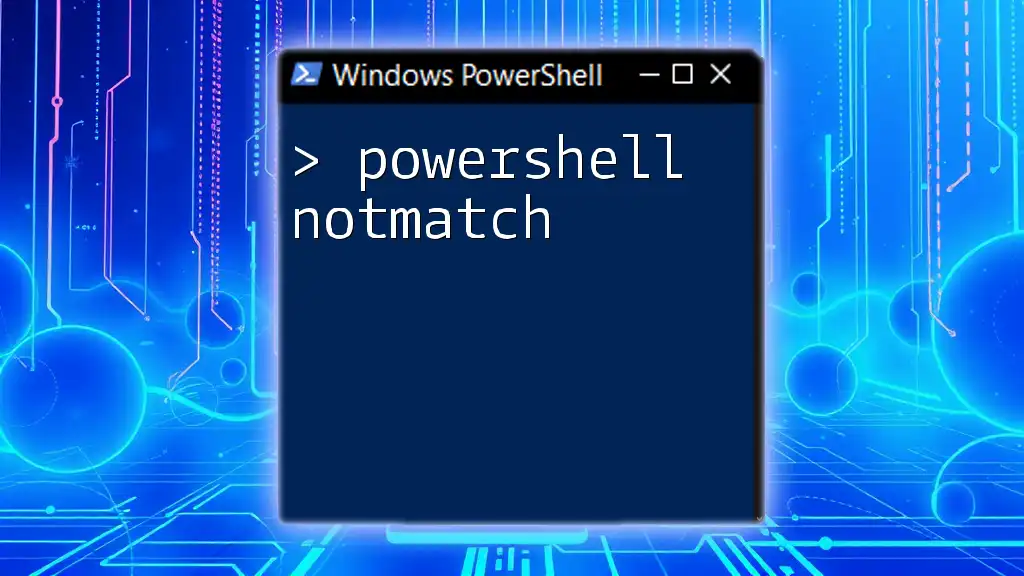
Call to Action
Practice writing your own "if not" scenarios in PowerShell to solidify your understanding. Explore more of what PowerShell can do by engaging with your company’s courses and additional resources aimed at enhancing your scripting skills.
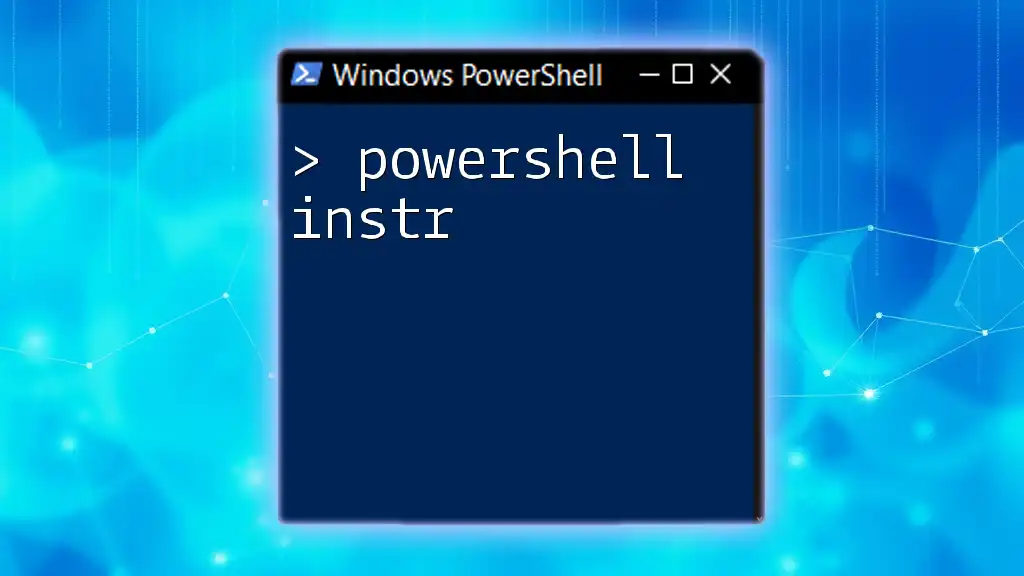
Additional Resources
For further information, consider consulting the official Microsoft PowerShell documentation or joining PowerShell communities and forums to connect with fellow learners and experts.
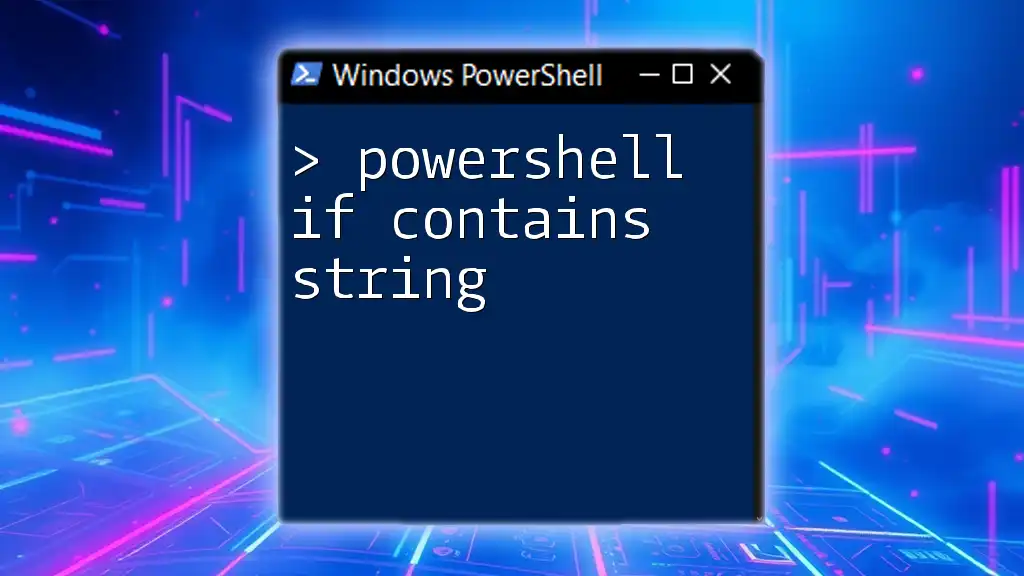
FAQs
This section may address some common inquiries regarding the use of "If Not" in PowerShell scripting, helping readers clarify their doubts and enrich their understanding of the topic.