The `Test-Path` cmdlet in PowerShell checks whether a specified path exists, allowing you to conditionally execute commands based on the result of that test.
Here’s a code snippet demonstrating its use:
if (Test-Path "C:\example\file.txt") {
Write-Host "The file exists."
} else {
Write-Host "The file does not exist."
}
Understanding Test-Path
What is Test-Path?
`Test-Path` is a cmdlet in PowerShell that checks if a specified path exists. It evaluates whether the path represents a valid file or directory. This cmdlet is essential for scripts that require validation of paths prior to performing operations, ensuring that actions are only taken if the necessary files or directories are present.
Syntax of Test-Path
The general syntax for using `Test-Path` is straightforward:
Test-Path -Path <String> [-PathType <Container | Leaf>]
Here, the `-Path` parameter specifies the path to check, while the optional `-PathType` parameter allows you to set the expected type of the path — either a container (directory) or a leaf (file).
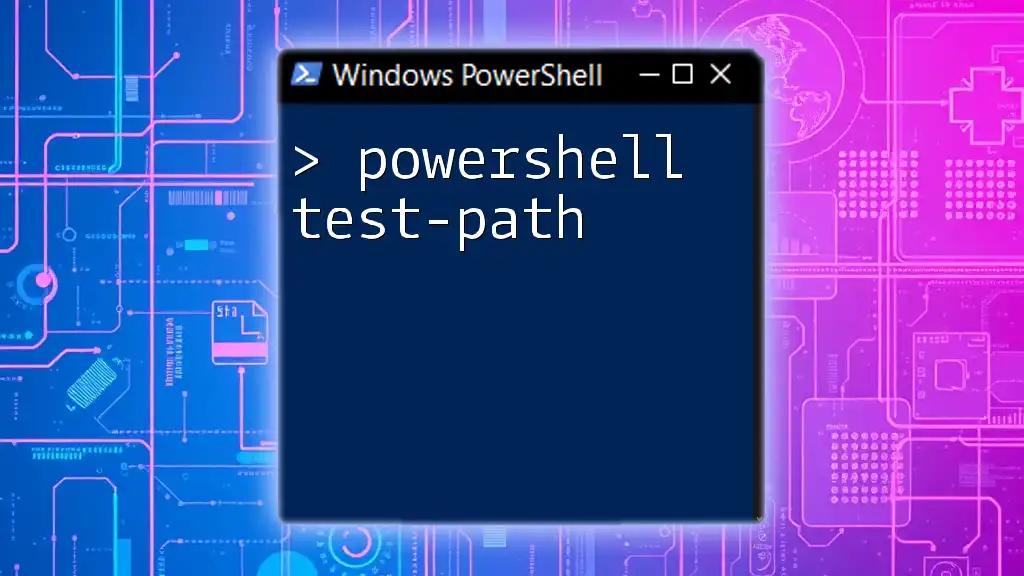
The Power of Conditional Statements
Introduction to If Statements
In PowerShell, if statements are used to define a block of code that executes only when certain conditions are met. This functionality allows for dynamic decision-making in scripts, which is crucial for automating tasks efficiently.
Structure of If Statements
The basic structure of an if statement in PowerShell looks like this:
If (<condition>) {
<code to execute if condition is true>
} Else {
<code to execute if condition is false>
}
Using `Test-Path` within an if statement enables scripts to respond to the existence (or absence) of specific files or directories.
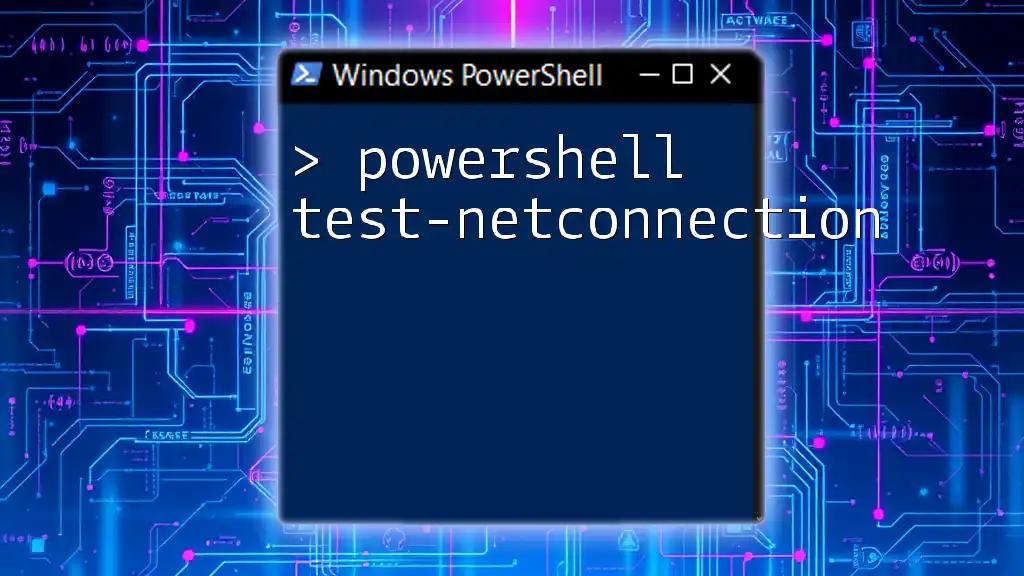
Using Test-Path with If Statements
Checking for Files
One of the most common use cases of `Test-Path` is checking whether a specific file exists. The following example demonstrates this functionality:
If (Test-Path -Path "C:\example\file.txt") {
Write-Host "File exists."
} Else {
Write-Host "File does not exist."
}
In this snippet, if `file.txt` is present in the specified directory, PowerShell will output "File exists." If not, it will respond with "File does not exist." This simple approach can prevent errors in later script executions.
Checking for Directories
Similarly, you may want to check if a directory exists. Here’s how to implement that:
If (Test-Path -Path "C:\example\directory") {
Write-Host "Directory exists."
} Else {
Write-Host "Directory does not exist."
}
This code checks for the existence of the `directory` path and returns appropriate messages based on the outcome. Recognizing the existence of directories is crucial for processes that depend on folder structures or when creating new files.
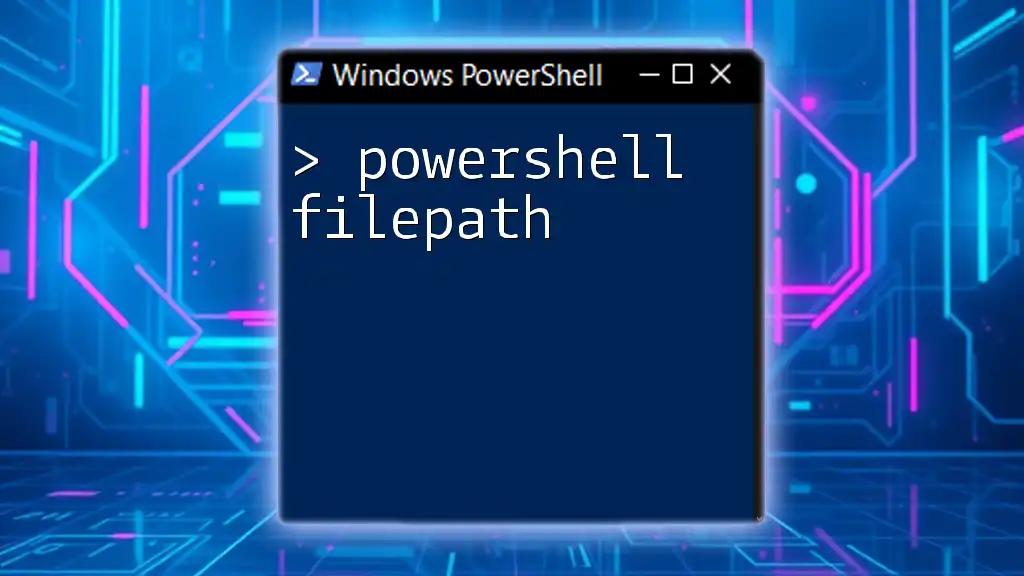
Advanced Uses of Test-Path
Using PathType Parameter
The `-PathType` parameter allows for more specificity when checking paths. It provides two options: `Container` for directories and `Leaf` for files. Here’s an example that uses both:
If (Test-Path -Path "C:\example" -PathType Container) {
Write-Host "This is a directory."
} ElseIf (Test-Path -Path "C:\example\file.txt" -PathType Leaf) {
Write-Host "This is a file."
}
In this example, the script first checks if the path is a directory; if not, it checks if it’s a file. This approach increases accuracy in path verification and helps avoid executing incorrect commands on the wrong types of paths.
Combining Test-Path with Other Cmdlets
Combining `Test-Path` with other cmdlets can enhance script functionality. For instance, here’s how to safely delete a file only if it exists:
If (Test-Path -Path "C:\example\file.txt") {
Remove-Item "C:\example\file.txt"
Write-Host "File deleted."
} Else {
Write-Host "No file to delete."
}
In this scenario, the script first checks for the existence of `file.txt`. If found, it proceeds to delete the file; otherwise, it notifies that there’s no file to delete. This method effectively prevents runtime errors that would occur if you tried to delete a non-existent file.

Best Practices
Error Handling and Testing
Implementing error handling is essential for robust scripts. Using `Test-Path` allows you to validate paths before executing commands, thereby reducing the risk of errors and unintended consequences. Logging outcomes can also aid in troubleshooting; it's a good practice to record success and failure messages.
Performance Considerations
Using `Test-Path` multi-facetedly is typically efficient and optimized within PowerShell. However, in larger scripts with many calls, careful placement of these checks can help avoid bottlenecks and enhance performance. Always consider the frequency of checks and aim to minimize unnecessary validations.

Conclusion
In summary, the `PowerShell if test-path` construct is a critical component of effective scripting and automation. Understanding and implementing `Test-Path` enables you to create robust, error-free scripts that dynamically respond to the state of your files and directories. As you practice and experiment with these concepts, be sure to explore additional resources to deepen your understanding of PowerShell scripting.