In PowerShell, the `If (Not (Test-Path <path>))` command checks whether a specified path does not exist, allowing you to execute a block of code only if the condition is true.
Here's an example code snippet:
$path = "C:\example\file.txt"
If (-Not (Test-Path $path)) {
Write-Host 'The file does not exist!'
}
Understanding Test-Path in PowerShell
Definition of Test-Path
Test-Path is a built-in PowerShell cmdlet that checks for the existence of a specified path, which can be a file, a directory, or even a registry path. This command returns a Boolean value: `True` if the path exists and `False` if it does not. This fundamental capability is crucial for scripts that need to conditionally create or modify files and directories based on their existence.
Syntax of Test-Path
The basic syntax of the Test-Path cmdlet is straightforward:
Test-Path "C:\MyFolder"
This command checks whether the directory at "C:\MyFolder" exists. You can also use Test-Path to check for files, like so:
Test-Path "C:\MyFile.txt"
Common Use Cases for Test-Path
Test-Path is often used in a variety of scenarios, including:
- Checking if a required configuration file exists before proceeding with a script.
- Verifying that a directory structure is in place before saving outputs.
- Ensuring that a specific registry key is available before making changes.
By embedding these checks into your scripts, you can avoid runtime errors and ensure that the script behaves as expected.

Using If Not with Test-Path
Basic Structure of If-Not Statements
PowerShell supports conditional statements, allowing you to execute code based on specific conditions. The If-Not structure combined with Test-Path is particularly useful. The typical syntax looks like this:
if (-not (Test-Path "C:\MyFolder")) {
# Commands here
}
This syntax reads as "if the path does not exist, then execute the following commands."
Implementing If Not Test-Path
Example: Creating a Directory If It Doesn't Exist
When creating a directory only if it isn't already present, you can simplify your script like this:
if (-not (Test-Path "C:\MyFolder")) {
New-Item -ItemType Directory -Path "C:\MyFolder"
}
In this example:
- The command checks for "C:\MyFolder".
- If it doesn't exist, `New-Item` is used to create it.
Example: Handling Files More Effectively
You can also use If-Not Test-Path for file management. Here’s an example showing how to create a file only if it does not already exist:
if (-not (Test-Path "C:\MyFile.txt")) {
New-Item -ItemType File -Path "C:\MyFile.txt"
}
This code snippet efficiently ensures that the specified file is created only when it is not present, saving unnecessary error handling later.
Practical Application in Scripting
The If-Not Test-Path construct plays a significant role in automation scripts. Here’s an illustrative example that creates a backup directory:
$path = "C:\Backup"
if (-not (Test-Path $path)) {
New-Item -ItemType Directory -Path $path
Write-Host "Backup directory created."
} else {
Write-Host "Backup directory already exists."
}
In this script:
- A variable `$path` stores the backup directory's path.
- The script checks whether the directory exists. If it does not, it creates the directory and informs the user about its creation.
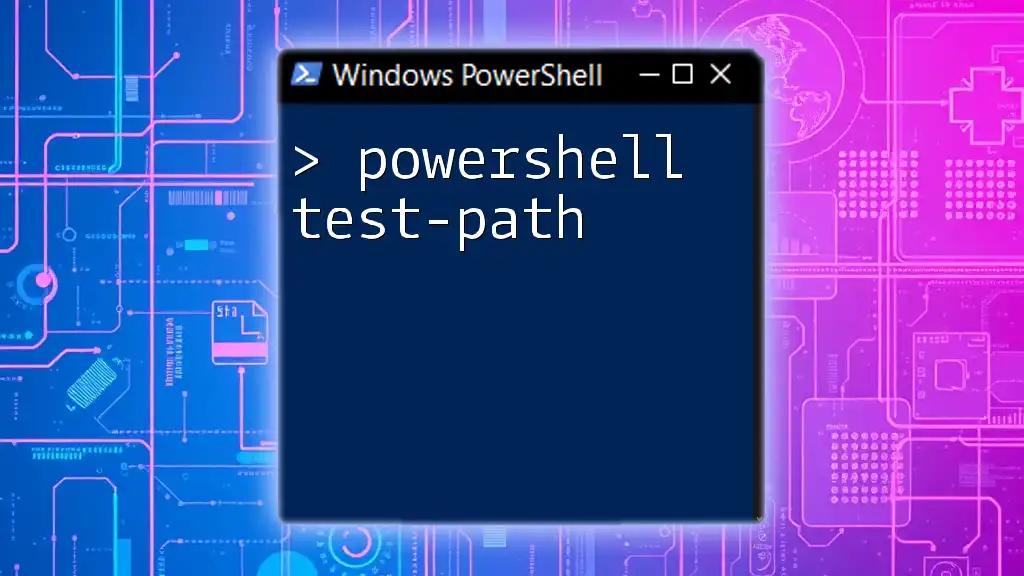
Best Practices for Using Test-Path in PowerShell
Error Handling with Try-Catch
Implementing strong error handling is essential for robust scripting. Utilizing Try-Catch blocks with Test-Path can ensure your scripts handle unexpected scenarios gracefully. Here’s how you can do it:
try {
if (-not (Test-Path "C:\TestPath")) {
New-Item -ItemType Directory -Path "C:\TestPath"
}
} catch {
Write-Error "An error occurred: $_"
}
In this example:
- The Try block attempts to create a directory if it does not exist.
- If an error occurs, the Catch block captures it and provides an error message, making troubleshooting more manageable.
Combining Test-Path with Other Commands
The versatility of Test-Path shines when you combine it with other cmdlets. For instance, you can chain commands to manage files more efficiently. Consider the following example:
if (-not (Test-Path "C:\MyFolder")) {
New-Item -ItemType Directory -Path "C:\MyFolder"
} else {
Get-ChildItem "C:\MyFolder" | Remove-Item -Force
}
In this script:
- The code checks for "C:\MyFolder." If it exists, it retrieves all items within the folder and removes them forcefully. This chaining demonstrates a more complex operational logic based on the existence of a path.
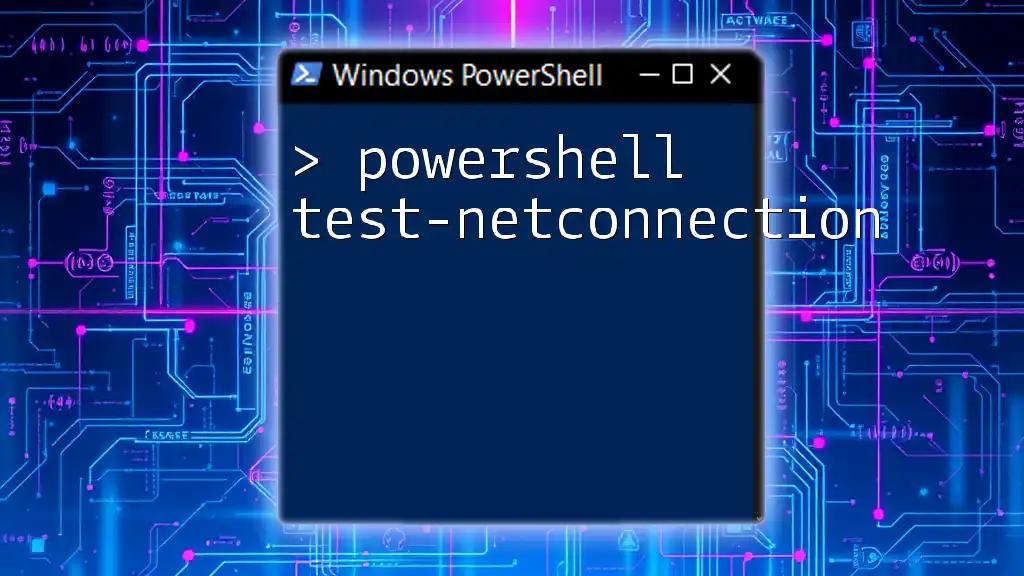
Troubleshooting Common Issues with Test-Path
Common Mistakes to Avoid
To use Test-Path effectively, be cautious of common errors:
- Forgetting to use quotes around paths can lead to unexpected results. Always wrap your paths in quotes.
- Misunderstanding the result of Test-Path — ensure you know it returns `True` or `False` and handle those outcomes in your conditional logic accordingly.
Debugging If Not Test-Path Statements
If you encounter issues with your If-Not Test-Path statements, utilize `Write-Host` for debugging:
if (-not (Test-Path "C:\SomePath")) {
Write-Host "Path does not exist. Proceeding to create..."
} else {
Write-Host "Path exists. Skipping creation."
}
This practice allows you to track the flow of your script execution, identifying where things might be going wrong.
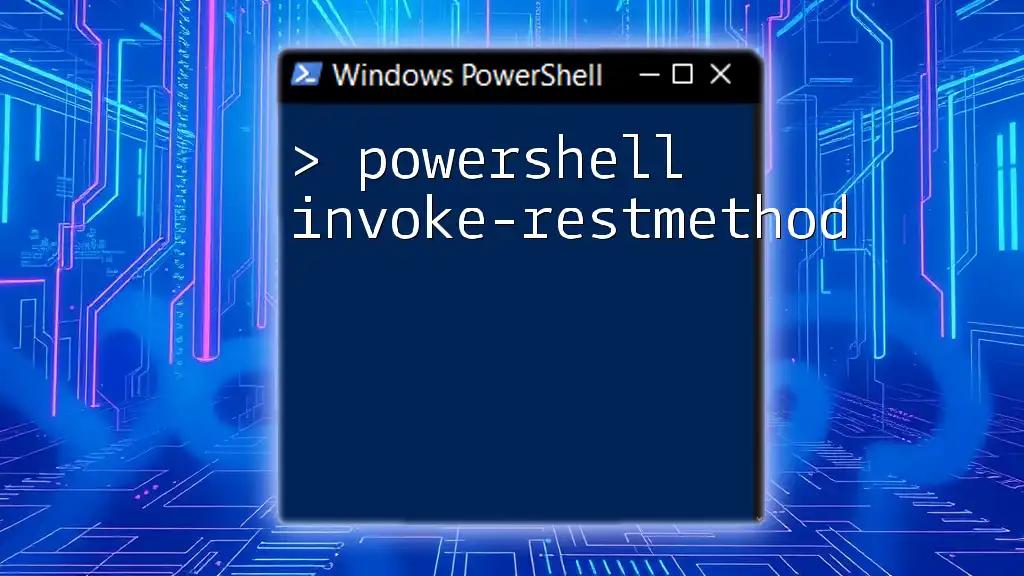
Conclusion
Using PowerShell If Not Test-Path provides an elegant and efficient method to manage the existence of files, directories, and registry paths. This command-line technique empowers you to create robust scripts that automate routine tasks while minimizing errors. Embracing these PowerShell constructs will significantly enhance your scripting capabilities, allowing you to build more advanced and user-friendly automation solutions.
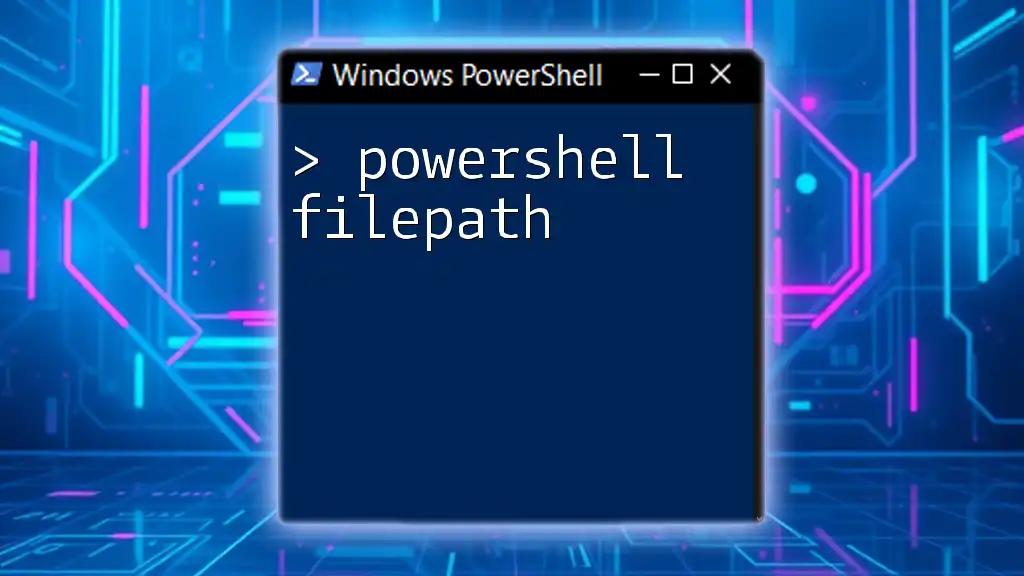
Additional Resources
For further learning, refer to the official PowerShell documentation, which offers comprehensive insights into cmdlets and scripting techniques. Online courses and books can also enhance your understanding and practical skills in PowerShell usage.