The "if not exist" statement in PowerShell checks whether a specified item, such as a file or directory, does not exist before executing a command.
Here’s a code snippet demonstrating this:
if (-not (Test-Path 'C:\path\to\your\file.txt')) {
Write-Host 'File does not exist.'
}
Understanding the Basics of PowerShell Conditional Statements
What are Conditional Statements?
Conditional statements are fundamental programming constructs that allow you to make decisions in your code based on specific conditions. In many programming languages, including PowerShell, these statements guide the flow of execution based on whether a given condition is true or false.
In PowerShell, the `if` statement is the backbone of conditional logic. It allows you to execute specific code blocks based on conditions that evaluate to true or false.
Importance of Checking for Existence
In scripting and automation, it’s often crucial to check whether an object, file, or service exists before performing certain actions. This checking mechanism helps avoid unnecessary errors, ensures smooth execution, and enhances script efficiency.
For example, attempting to create a file that already exists will throw an error. By checking for existence first, you can gracefully handle these situations, leading to more efficient and error-free scripts.
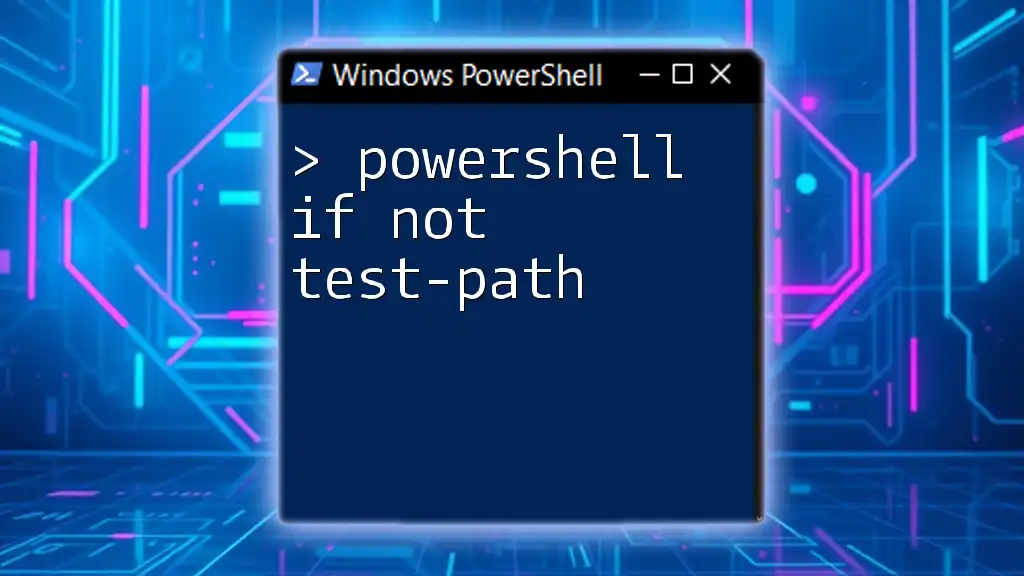
The "If Not Exist" Concept in PowerShell
What Does "If Not Exist" Mean?
The phrase "if not exist" refers to a logical condition where you want to carry out a command only if a specified item does not exist. This is integral when designing scripts that need to ensure certain prerequisites are met before proceeding.
Syntax of the "If Not Exist" Statement
The primary way to check for existence in PowerShell is through the `Test-Path` cmdlet. The basic syntax for using "if not exist" can be represented as follows:
if (-not (Test-Path "C:\path\to\file.txt")) {
# Actions to take if the file does not exist
}
In this statement:
- `Test-Path` checks whether the specified path exists.
- `-not` negates the condition, allowing you to execute the enclosed block only if the path does not exist.
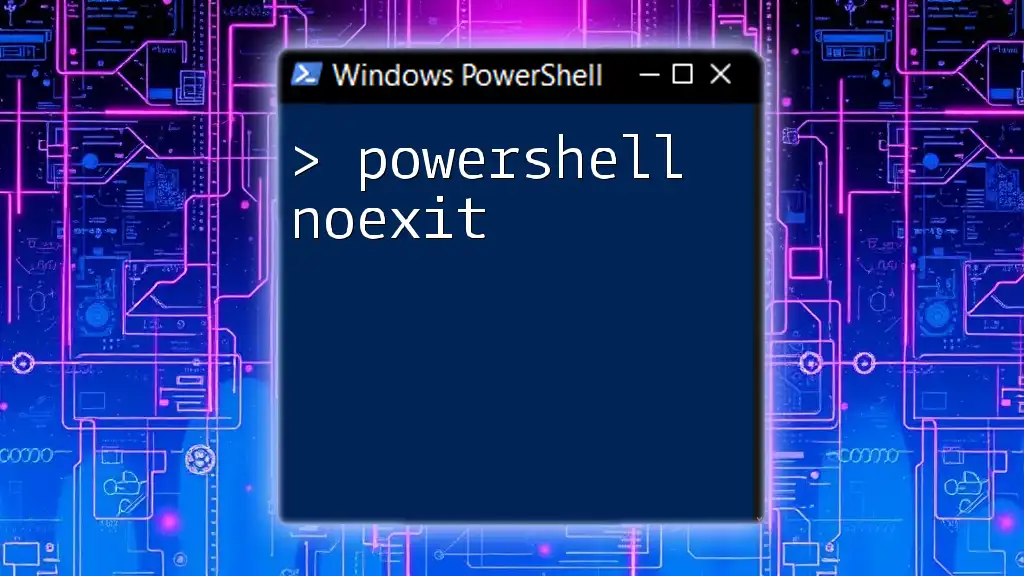
Practical Examples of "If Not Exist" in PowerShell
Example 1: Checking for a File
One common scenario is checking if a specific file exists before creating it. Consider the following script:
$filePath = "C:\path\to\file.txt"
if (-not (Test-Path $filePath)) {
New-Item -Path $filePath -ItemType File
Write-Host "File created: $filePath"
} else {
Write-Host "File already exists."
}
In this example, if `file.txt` does not exist in the specified path, it will be created, and the user will be informed. If it does exist, the script will simply notify the user that the file is already present.
Example 2: Ensuring a Directory Exists
Another practical use of the "if not exist" construct is to ensure that a directory is created only if it doesn't already exist. Here’s how you can implement this:
$directoryPath = "C:\path\to\directory"
if (-not (Test-Path $directoryPath)) {
New-Item -Path $directoryPath -ItemType Directory
Write-Host "Directory created: $directoryPath"
} else {
Write-Host "Directory already exists."
}
This script checks if the specified directory exists at the given path. If absent, a new directory will be created, and the user will receive a confirmation message.
Example 3: Checking for a Running Service
Sometimes, you may need to ensure that a service is running. This can be handled by checking whether the service exists, as shown in the following example:
$serviceName = "YourServiceName"
if (-not (Get-Service -Name $serviceName -ErrorAction SilentlyContinue)) {
Start-Service -Name $serviceName
Write-Host "Service started: $serviceName"
} else {
Write-Host "Service is already running."
}
In this scenario, the script checks for the existence of a specific service. If the service is not found (i.e., it does not exist), it will attempt to start it and notify the user about the action taken.
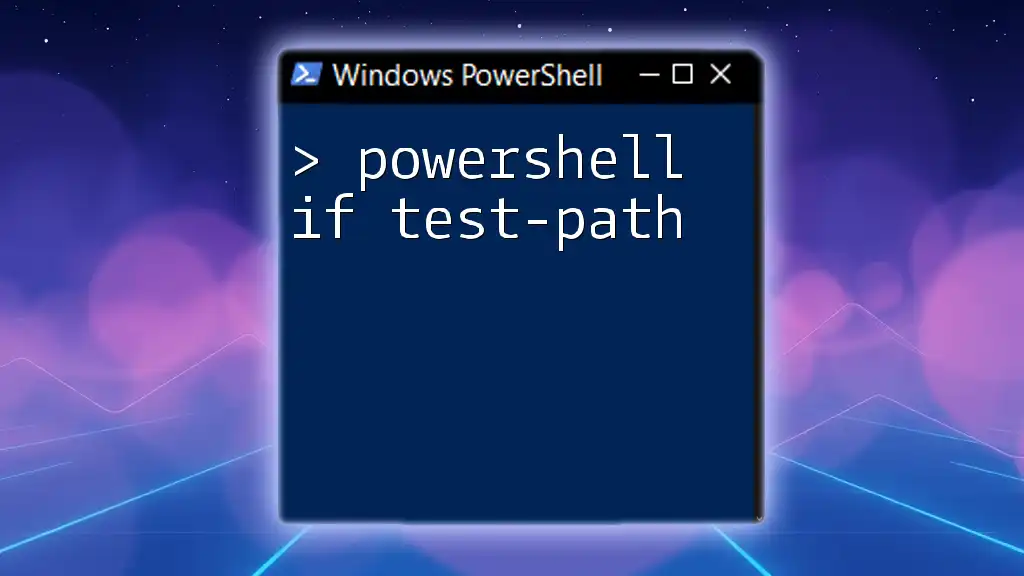
Advanced Use Cases
Combining "If Not Exist" with Loops
You can also extend the "if not exist" construct by using loops for cases where you need to create multiple files dynamically. Here’s a practical example:
$files = @("file1.txt", "file2.txt", "file3.txt")
foreach ($file in $files) {
if (-not (Test-Path $file)) {
New-Item -Path $file -ItemType File
Write-Host "Created: $file"
} else {
Write-Host "Exists: $file"
}
}
In this script, a collection of files is processed. Each file is checked for existence, and only non-existing files are created, providing immediate feedback about each action.
Error Handling with "If Not Exist"
Best practices in scripting include robust error handling. Sometimes, you may want to combine "if not exist" checks with try/catch blocks to deal with potential errors gracefully:
$path = "C:\path\to\importantFile.txt"
try {
if (-not (Test-Path $path)) {
New-Item -Path $path -ItemType File
Write-Host "Important file created: $path"
} else {
Write-Host "Important file already exists."
}
} catch {
Write-Host "An error occurred: $_"
}
In this example, if an error occurs during the file creation process, the catch block captures the error and prints an informative message without stopping the script unexpectedly.
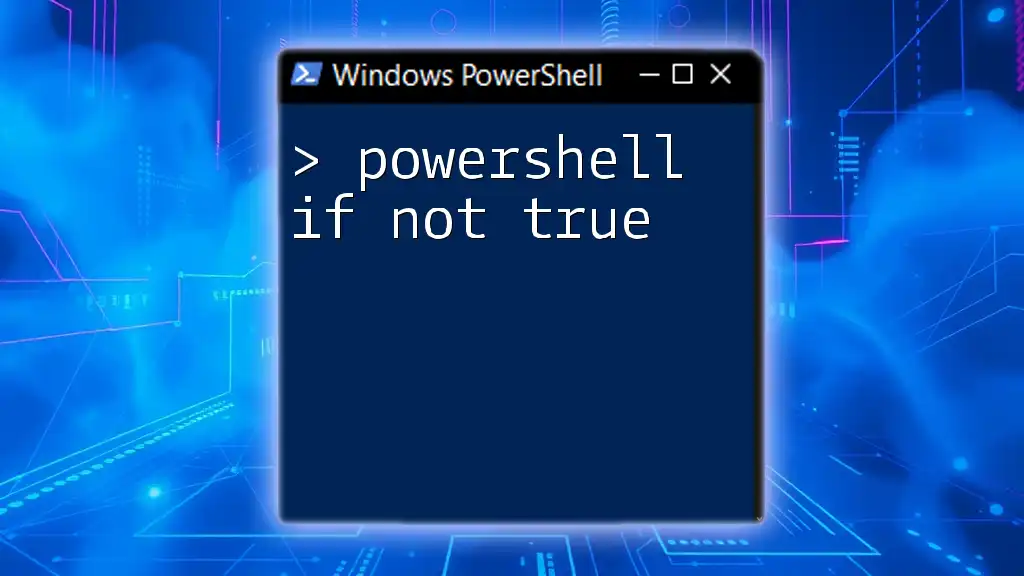
Best Practices for Using "If Not Exist" in PowerShell
Keep Your Scripts Clean
Maintaining clean and readable scripts is key in scripting. Proper use of indentation, naming conventions, and clear logic flow plays a fundamental role in making your code easier to read and maintain.
Using Comments
Incorporating comments within your script can elucidate the purpose of sections of your code, making it easier for other users (or your future self) to understand the code logic quickly.
Performance Considerations
It is wise to minimize redundant existence checks as this can lead to a drop in performance, especially in scripts that run frequently or handle large datasets. Always evaluate the necessity of each test being performed.
Testing Your Scripts
Engage in thorough testing of your scripts before deploying them in a production environment. PowerShell offers various debugging tools and modes that assist in pinpointing issues, ensuring that your scripts run smoothly as intended.
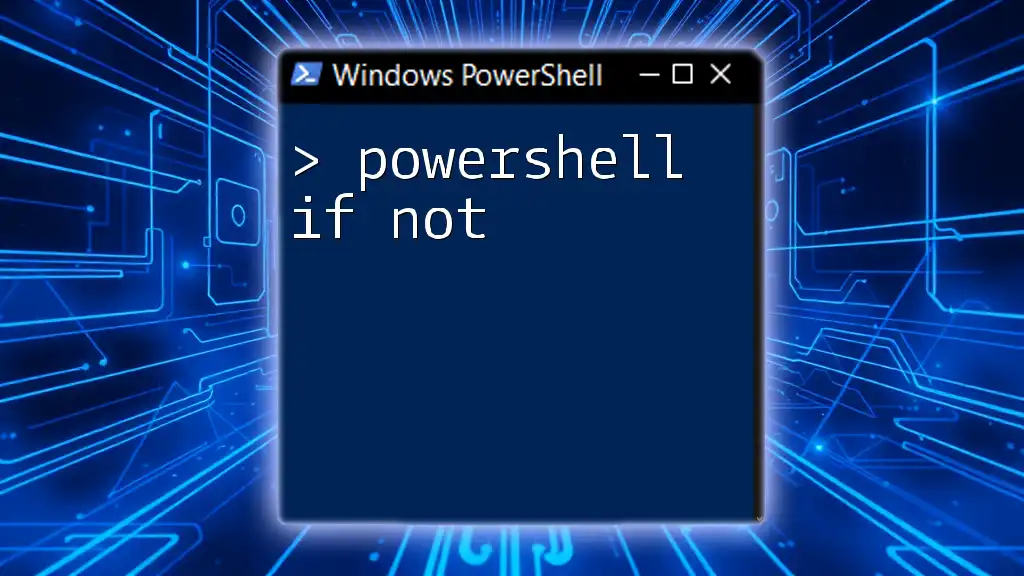
Conclusion
The concept of "PowerShell if not exist" is vital for creating robust scripts that perform checks before executing commands. With the insights shared in this article, you are well-equipped to leverage these constructs in your PowerShell automation tasks.
Be sure to practice using these techniques in your scripts, as hands-on experience will solidify your learning. For continuous professional growth, consider subscribing to our content, and do not hesitate to reach out for personalized PowerShell training.
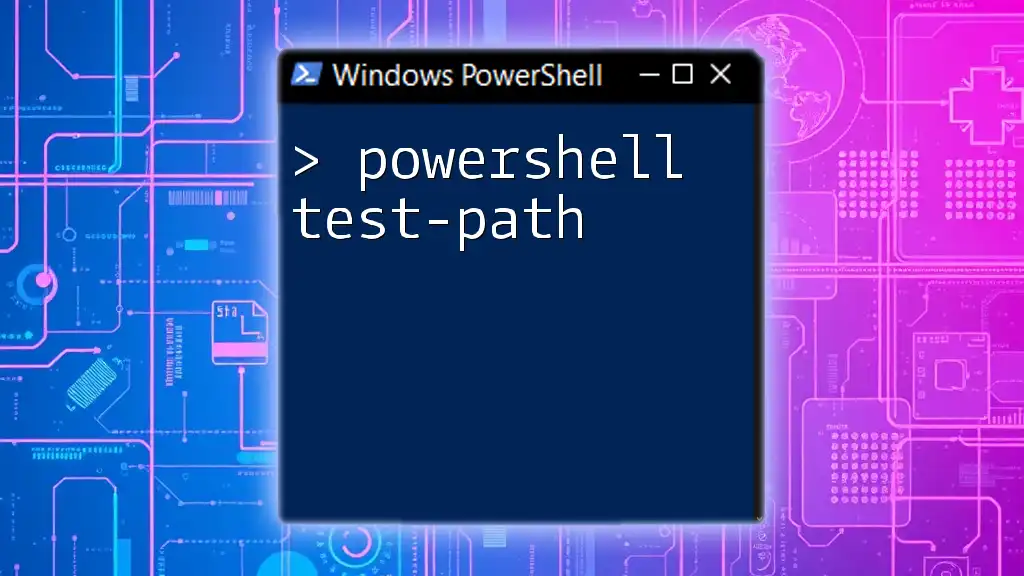
Further Reading and Resources
To deepen your understanding of PowerShell, consider exploring the official PowerShell documentation, which provides comprehensive details about cmdlets and scripting techniques. You might also want to check out specialized PowerShell books tailored for different experience levels.