In PowerShell, the "if not true" statement allows you to execute code when a specified condition evaluates to false, providing an effective way to handle logical conditions.
Here's a code snippet to illustrate this:
if (-not $condition) {
Write-Host 'The condition is not true!'
}
Understanding Conditional Statements in PowerShell
What are Conditional Statements?
Conditional statements are fundamental constructs in programming that allow you to execute certain blocks of code based on specific conditions. They enhance script functionality by enabling it to make decisions, leading to more dynamic and responsive scripts.
Overview of `if` Statement
In PowerShell, the `if` statement evaluates a condition and executes a block of code only if that condition is true. The basic structure of an `if` statement looks like this:
if (<condition>) {
# Code to execute if the condition is true
}
An if statement is crucial for controlling the flow of scripts, making them adaptable to different scenarios and inputs.
The Role of Boolean Logic
Boolean logic is the backbone of conditional statements in PowerShell. It uses two values—True and False—to determine the outcome of a condition. Understanding how to work with Boolean values is essential for writing efficient scripts, as the evaluation of conditions dictates the flow of execution.

The Concept of "If Not True"
Understanding "Not" Operator
The `-not` operator in PowerShell is used to negate Boolean conditions. When you apply the `-not` operator, it flips the value of the condition from True to False or vice versa. This is particularly useful when you want to execute a block of code when a certain condition is not met.
Using "if not true" in PowerShell
The structure of an `if not` statement can be succinctly described as follows:
if (-not <condition>) {
# Code to execute if the condition is false
}
This allows you to succinctly express conditions where you want to take action when something does not hold true.

Practical Examples of “If Not True”
Example 1: Checking for User Presence
Consider a scenario where you need to check if a specific user does not exist on a system. You can achieve this using the following script:
$username = "testuser"
if (-not (Get-LocalUser -Name $username -ErrorAction SilentlyContinue)) {
Write-Host "$username does not exist."
}
Explanation
In this example, `Get-LocalUser` attempts to find a local user by the name specified in `$username`. The `-ErrorAction SilentlyContinue` parameter suppresses any error messages if the user is not found. If the user does not exist, the script will output that the user does not exist.
Example 2: Validating Input Parameters
Validating user input is a crucial step in ensuring that scripts run without errors. Here’s how you can check if a user has provided an input value:
param (
[string]$inputValue
)
if (-not $inputValue) {
Write-Host "Input value is empty. Please provide a valid value."
}
Explanation
In this case, we define a parameter called `$inputValue`. The script checks whether this parameter is empty. If it is, it prompts the user to provide a valid value. This practice not only improves the user experience but also enhances the robustness of your scripts.
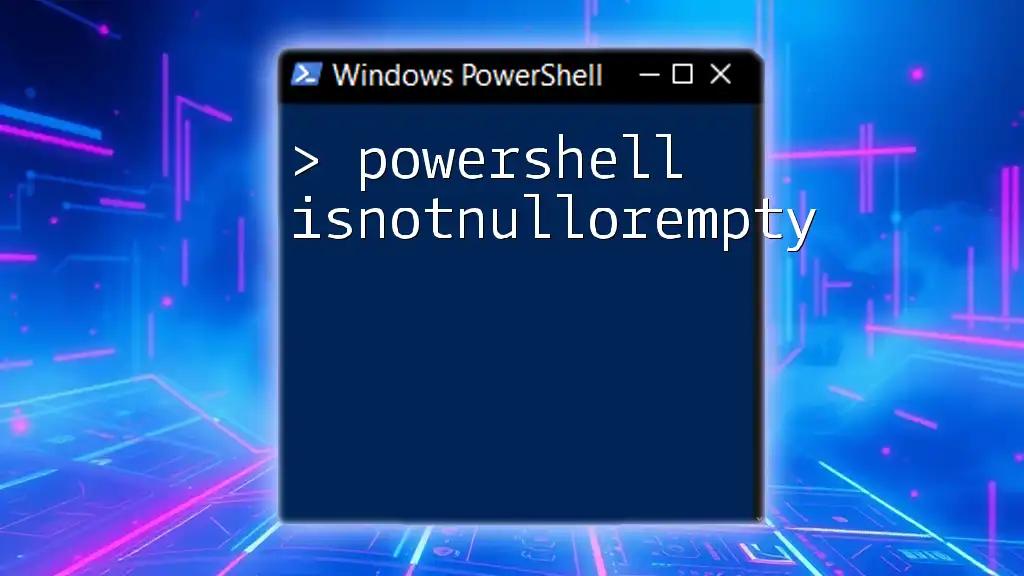
Advanced Scenarios Using "If Not True"
Nested Conditions
Sometimes you may need to perform multiple checks in your scripts. This is where nested `if` statements come into play. Here is an example:
if (-not $condition1) {
if (-not $condition2) {
Write-Host "Both conditions are false."
}
}
Explanation
In this example, the script first checks if `condition1` is false. If so, it then checks `condition2`. If both are false, a message is displayed. Nested conditions allow for more complex decision-making within scripts.
Combining with Logical Operators
PowerShell supports logical operators such as `-and` and `-or`. By combining these operators with `if not true`, you can create powerful conditions. Here’s an example:
if (-not ($condition1 -and $condition2)) {
Write-Host "At least one of the conditions is false."
}
Explanation
In this scenario, the script evaluates whether at least one of the conditions is false. If either `condition1` or `condition2` evaluates to false, the message will be displayed. This approach allows for sophisticated logic in your scripts.

Common Mistakes to Avoid
When using `powershell if not true`, it’s essential to be aware of common pitfalls:
-
Misunderstanding Boolean Logic: Misinterpretations of how PowerShell evaluates true and false can lead to unexpected results. Always be clear about the true/false nature of your conditions.
-
Forgetting Braces: Omitting braces `{}` can lead to errors in your scripts, especially when you intend to execute multiple lines of code based on a condition.
-
Using Incorrect Operator Syntax: Ensure you use the proper syntax when applying logical operators and negations. Typos can often lead to runtime errors or unintended behavior.

Best Practices When Using "If Not True"
To ensure your scripts are efficient and maintainable, keep these best practices in mind:
-
Simplicity and Readability: Strive to keep conditions straightforward and easy to read. The more readable your scripts are, the easier they will be to maintain and debug.
-
Descriptive Variable Names: Use meaningful variable names to convey their purpose, improving code readability and maintainability.
-
Comment Your Code: Adding comments to explain complex portions of code can be immensely beneficial for the future, especially when revisiting your scripts after some time.
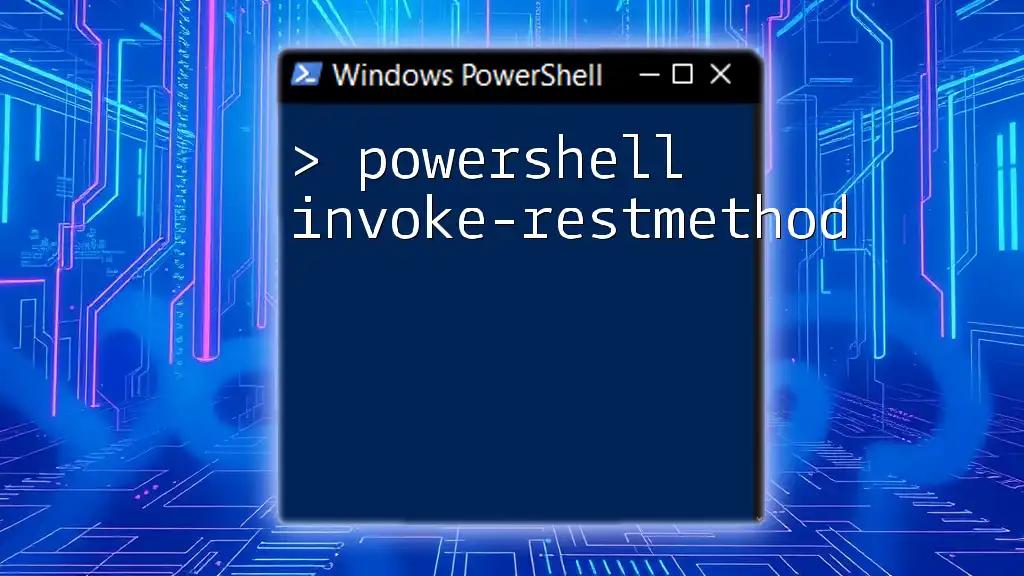
Conclusion
Using `powershell if not true` effectively can enhance your scripting capabilities significantly. It allows for the construction of robust scripts that can make logical decisions based on varying conditions. By practicing with the examples and guidelines provided, you'll become more proficient with PowerShell scripting.

Call to Action
To take your PowerShell skills to the next level, consider signing up for our newsletters, courses, and workshops dedicated to more advanced PowerShell concepts. Explore additional resources and continue your journey towards mastering PowerShell scripting!

Additional Resources
For further reading and exploration, consider visiting the official PowerShell documentation, and check out some recommended books and online courses that dive deeper into PowerShell scripting for more advanced learning.