The `Invoke-RestMethod` cmdlet in PowerShell is used to send HTTP and HTTPS requests to RESTful web services and receive responses in a simple way, making it easier to interact with APIs.
Here’s a code snippet demonstrating its usage:
$response = Invoke-RestMethod -Uri 'https://api.example.com/data' -Method Get
Write-Host $response
Understanding Invoke-RestMethod
What is Invoke-RestMethod?
At its core, Invoke-RestMethod is a PowerShell cmdlet that facilitates making HTTP requests to RESTful APIs. REST (Representational State Transfer) is an architectural style that allows interaction with web services in a stateless manner. As PowerShell has gained popularity in automation and script-based environments, Invoke-RestMethod provides a straightforward way to tap into web services and retrieve or send data.
Why Use Invoke-RestMethod?
Using Invoke-RestMethod streamlines the process of calling APIs within PowerShell scripts. The key benefits include:
- Automation: It allows for the automation of tasks such as data retrieval and manipulation.
- Integration: Seamlessly integrate various web services into your workflows.
- Ease of Use: The cmdlet is designed with simplicity in mind, making it user-friendly even for those new to PowerShell scripting.

Getting Started with Invoke-RestMethod
Pre-requisites
Before you dive into using Invoke-RestMethod, it's essential to have a basic understanding of PowerShell. Familiarity with the command line, as well as JSON (JavaScript Object Notation), can enhance your experience, especially when dealing with APIs that return data in this format.
Installing PowerShell (if applicable)
If PowerShell is not already installed on your system, follow these steps based on your operating system:
- Windows: PowerShell comes pre-installed on most Windows systems. If you need the latest version, visit the [PowerShell GitHub repository](https://github.com/PowerShell/PowerShell) for installation instructions.
- macOS: You can install PowerShell via Homebrew:
brew install --cask powershell
- Linux: Use the package manager specific to your distribution. For example, on Ubuntu:
sudo apt-get install -y powershell
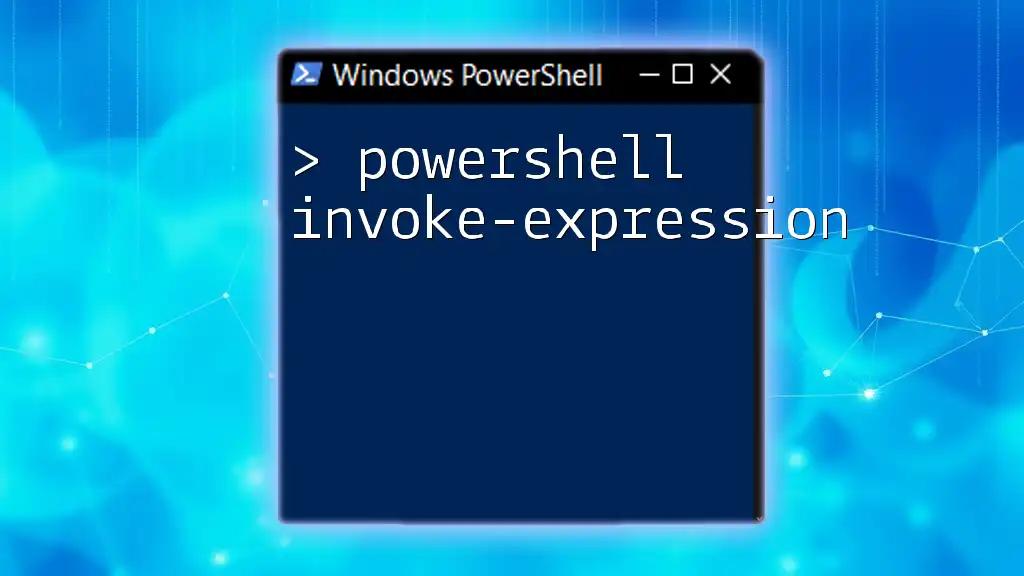
Basic Syntax of Invoke-RestMethod
The Command Structure
The fundamental structure of the Invoke-RestMethod cmdlet is simple:
Invoke-RestMethod -Uri "<URL>" -Method <HTTPMethod>
Here, `<URL>` represents the endpoint you are calling, and `<HTTPMethod>` can be one of several types, such as GET, POST, PUT, or DELETE.
Specifying Parameters
While the basic syntax is straightforward, there are additional parameters that enhance the functionality of the cmdlet:
- -Headers: Use this parameter to send custom headers, often needed for authentication.
- -Body: This is where you specify the data you want to send with your request, particularly in POST calls.
- -ContentType: Specify the format of the data you're sending (e.g., `application/json`).
- -OutFile: This parameter allows you to save the output directly to a file.

Making Your First API Call
Setting Up a Simple GET Request
A GET request is designed to retrieve data from the specified resource. Here’s how to make a simple GET request with Invoke-RestMethod:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
Write-Output $response
Once you run this command, the response will typically be a JSON object that you can manipulate directly in PowerShell.
Making a POST Request
To send data to an API, a POST request is appropriate. Here's a step-by-step example using the `-Body` parameter:
$body = @{ name = "Example"; value = "Sample" }
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Post -Body $body -ContentType "application/json"
Write-Output $response
In this example, we create a hashtable that represents the data to be sent in the POST request. Make sure you set the correct `ContentType` to avoid any errors.
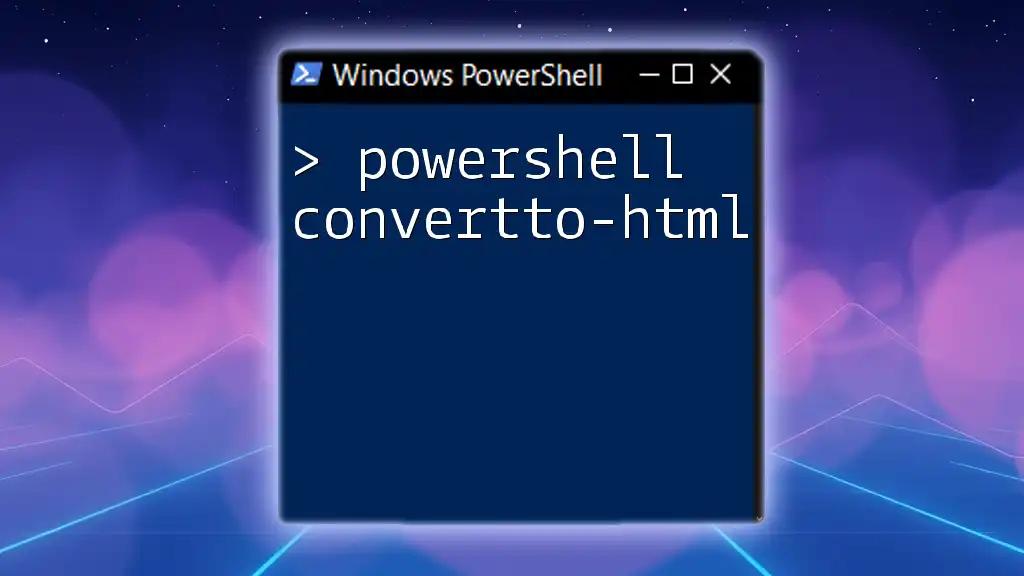
Advanced Invocation Techniques
Using Headers for Authentication
Many APIs require authentication to ensure secure access. To include authentication tokens, you can specify headers as follows:
$headers = @{ Authorization = "Bearer <Your-Token>" }
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get -Headers $headers
In this instance, the `Authorization` header is utilized to include the bearer token necessary for the API access.
Customizing Request Body for Complex Data
When dealing with complex data structures, you may need to send JSON strings. Here’s how to do that:
$jsonBody = '{"name": "Example", "value": "Complex"}'
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Post -Body $jsonBody -ContentType "application/json"
This approach is generally used when your data model is more intricate, requiring a structured JSON format.
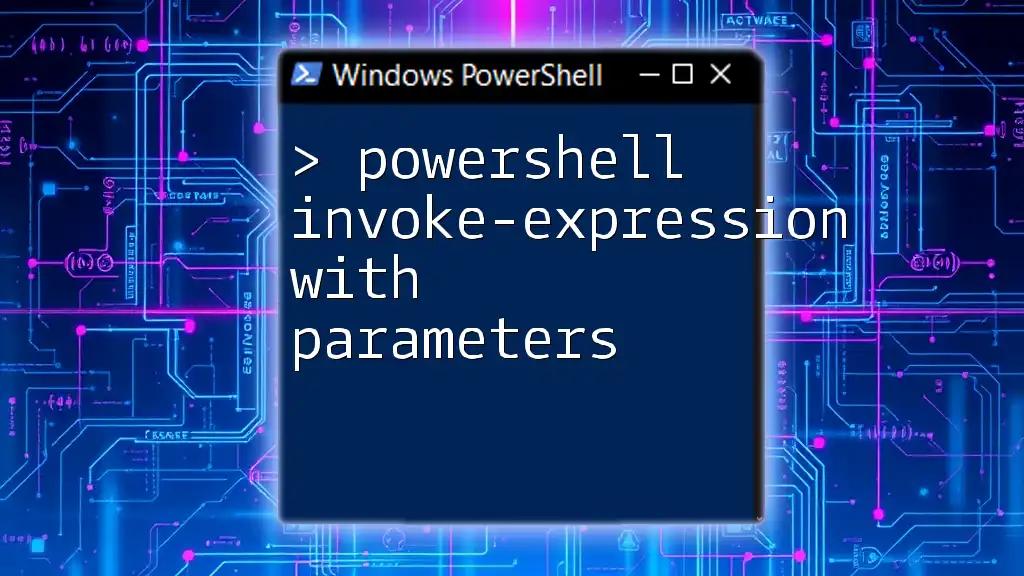
Handling Responses from Invoke-RestMethod
Parsing JSON Responses
Upon receiving a response from an API, it is often in JSON format. Here’s how to parse it effectively:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
$value = $response.value
Write-Output $value
With PowerShell’s inherent capabilities, you can access properties directly from the parsed JSON object with ease.
Error Handling
Errors can occur at any stage when dealing with APIs. Utilize a try-catch block to handle exceptions gracefully:
try {
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
} catch {
Write-Output "Error: $_"
}
This structure allows you to capture and respond to exceptions without terminating your entire script.
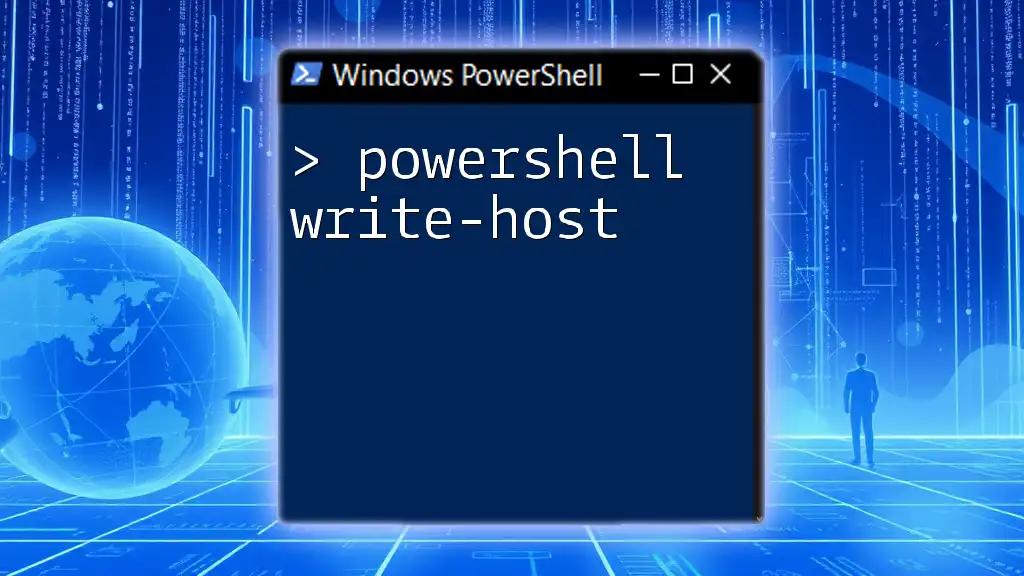
Real-World Applications of Invoke-RestMethod
Fetching Data from Public APIs
Many public APIs are available that provide data for various domains, such as weather or finance. For instance, you can fetch weather data using:
$weatherData = Invoke-RestMethod -Uri "https://api.openweathermap.org/data/2.5/weather?q=London&appid=<Your-API-Key>" -Method Get
Write-Output $weatherData
In this example, make sure to replace `<Your-API-Key>` with your actual API key.
Automating Tasks with PowerShell and APIs
Imagine automating a monthly report generation by fetching different data points from multiple APIs. You can create a script that runs on a schedule to query several endpoints, aggregate the data, and store it for later use.
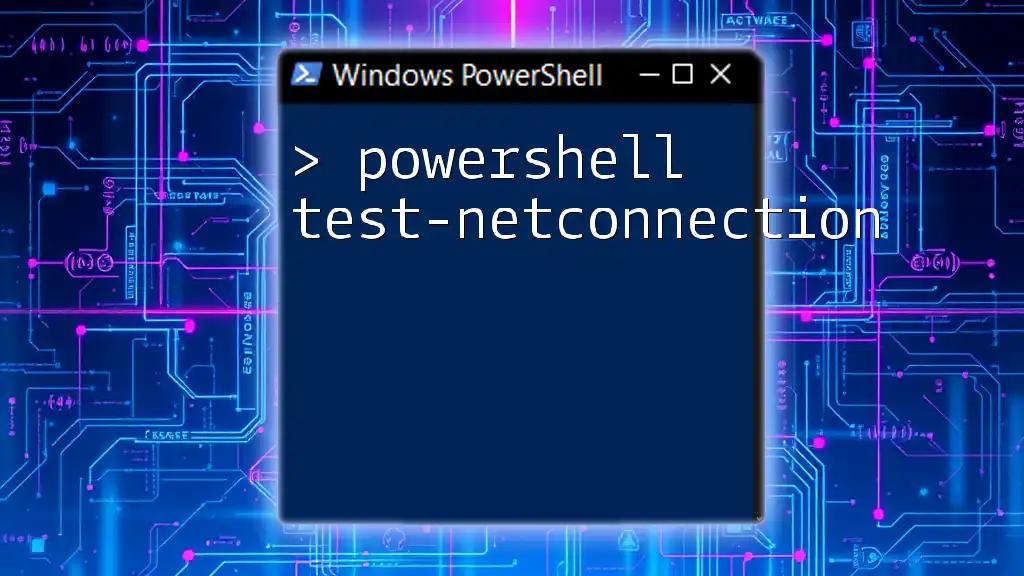
Tips and Best Practices
Debugging API Calls
When debugging API calls, consider using the `-Verbose` switch for additional details about the execution of your command. This can illuminate various factors contributing to potential issues.
Optimizing Performance
For better performance, be mindful of the number of API calls your script makes, as excessive requests may trigger rate limiting. Plan your script logic to minimize calls while still achieving your objectives.
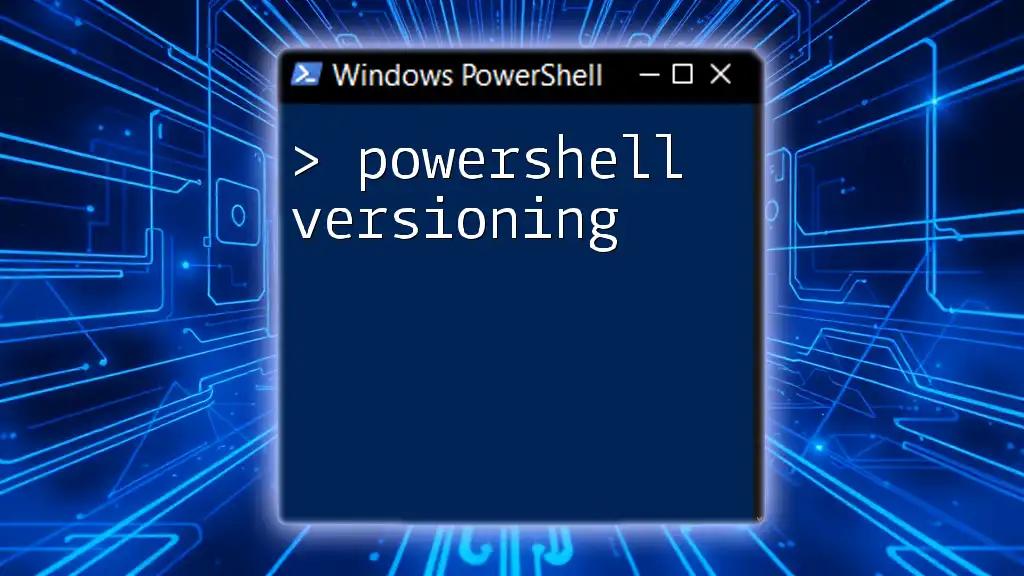
Conclusion
In summary, PowerShell Invoke-RestMethod is a powerful tool for interacting with RESTful APIs. By understanding its syntax and capabilities, you can efficiently retrieve and manipulate data in your workflows. Experiment, practice, and integrate this cmdlet into your automation tasks to unlock the power of web services in your PowerShell scripts.
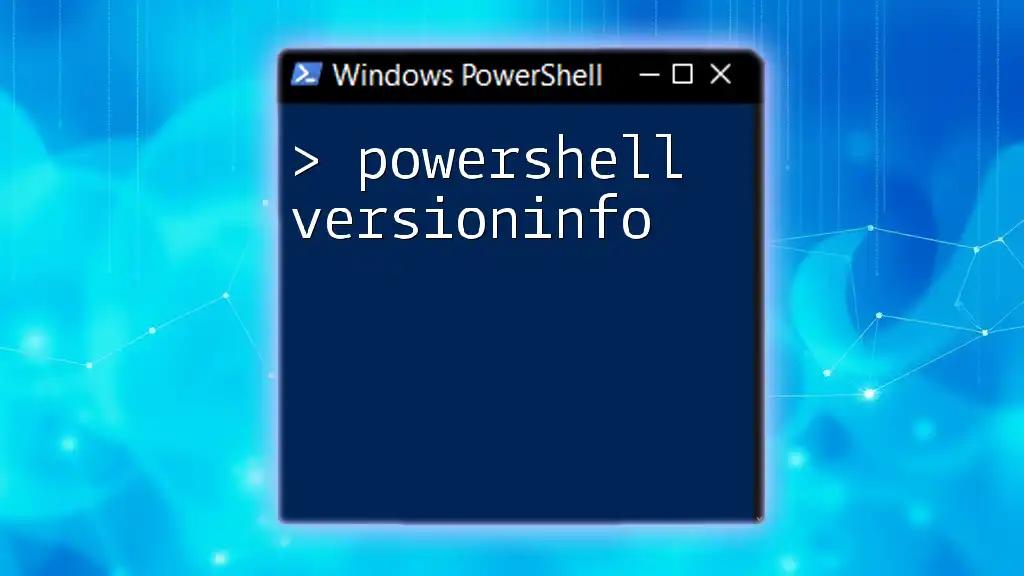
Further Learning Resources
For those eager to expand their knowledge, exploring the official PowerShell documentation and diving into specific API documentation are excellent ways to continue your learning journey.
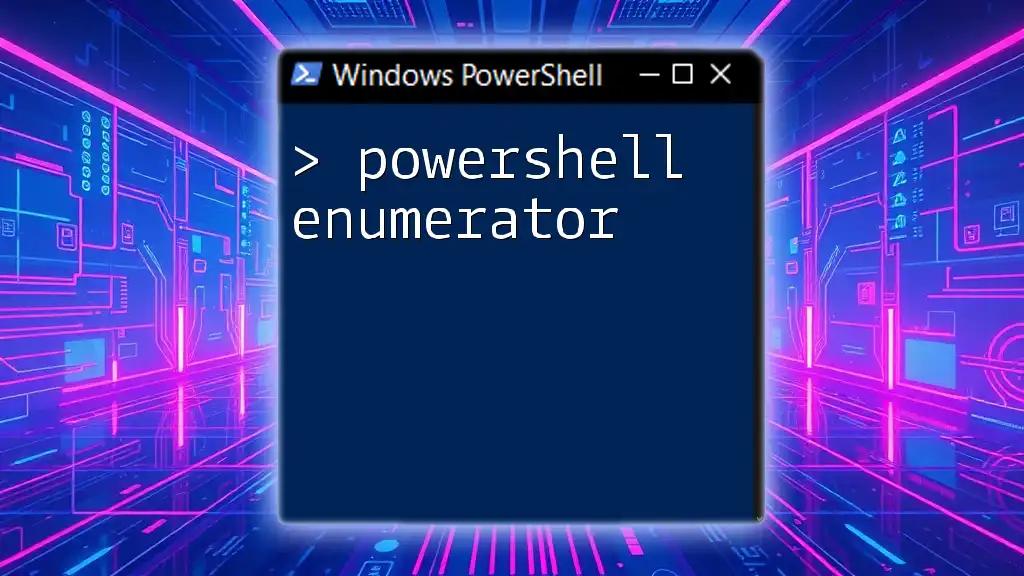
Call to Action
Now that you have a comprehensive understanding of how Invoke-RestMethod operates, it’s time to put that knowledge into action. Start experimenting with web services today, and don’t hesitate to share your experiences, questions, or discoveries in the comments section!