The `Invoke-Command` cmdlet in PowerShell allows you to run commands or scripts on local or remote computers, enabling efficient task execution across multiple environments.
Here’s a basic example of how to use it:
Invoke-Command -ScriptBlock { Get-Process }
Understanding `PowerShell.Invoke`
`PowerShell.Invoke` encompasses two primary commands in PowerShell: `Invoke-Expression` and `Invoke-Command`. Each serves distinct yet powerful roles within the PowerShell environment, making automation smoother and more efficient.
-
Definition and Basic Functionality:
- The `Invoke` commands facilitate the execution of other commands and scripts, either locally or remotely. They allow you to take complex command strings and run them as if they were directly typed into the PowerShell console.
-
Primary Use Cases:
- Quickly executing strings as code.
- Running complex command blocks remotely against computers in a network.
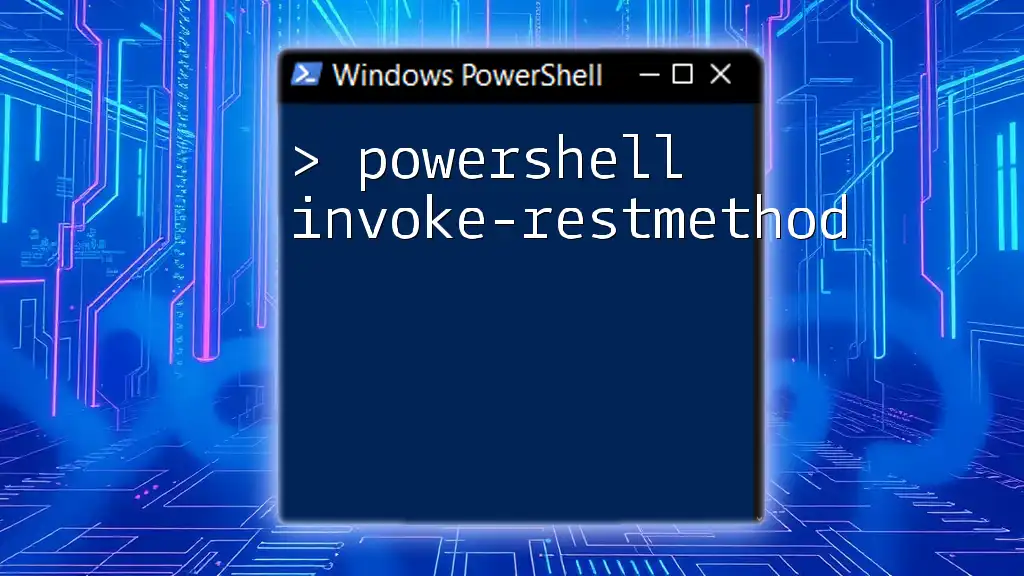
Key Components of PowerShell Invoke
Cmdlets: Understanding the Core Commands
Cmdlets are built-in functions in PowerShell, representing small, single-function commands that can be utilized in scripts. Some common cmdlets used with `Invoke` include:
- `Get-Process`: Retrieves a list of processes running on the system.
- `Get-Service`: Retrieves the status of services on the system.
Arguments & Parameters: How They Work with Invoke
Arguments and parameters allow you to customize the behavior of a command. You can pass parameters to `Invoke-Command` to interact with systems or processes effectively.
For instance, when executing a command using `Invoke-Command`, you can pass parameters like so:
Invoke-Command -ScriptBlock { param($name) Write-Host "Hello, $name!" } -ArgumentList "Alice"
Here, the `param` block within `ScriptBlock` accepts the argument and expands its usefulness.
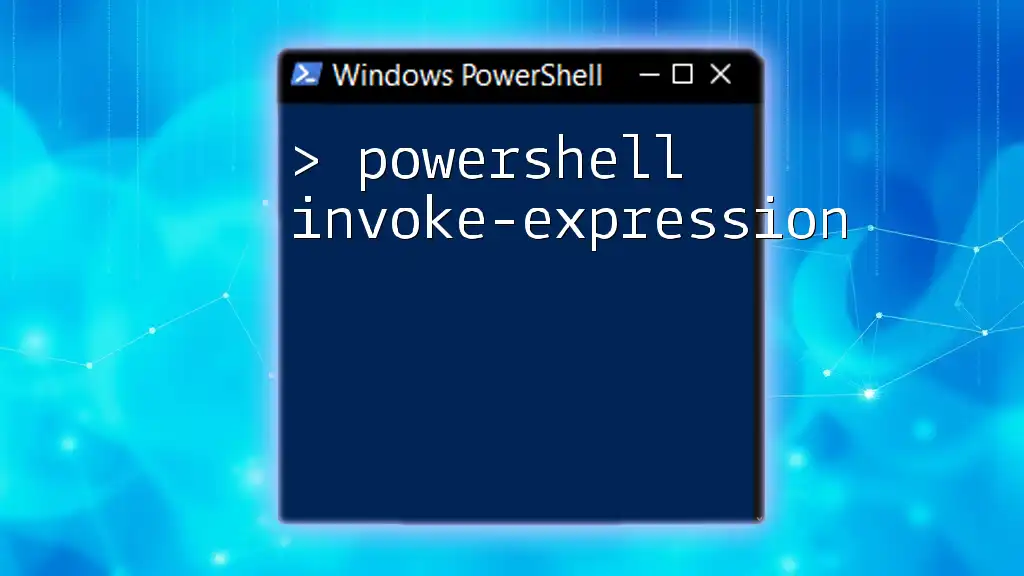
Working with the Invoke Command
Using `Invoke-Expression`
Definition and Use Cases: `Invoke-Expression` evaluates a string as a command that can be executed. This is particularly useful when you have built a command as a string concatenation that you want to run.
Scenarios where `Invoke-Expression` shines:
- Executing dynamically generated PowerShell commands if you need to build your command at runtime.
Code Example:
$command = "Get-Process"
Invoke-Expression $command
This line will execute `Get-Process` as if it were typed directly into the console.
Using `Invoke-Command`
Definition and Use Cases: `Invoke-Command` is designed to run scripts or commands on remote computers. It is essential for scenarios where you need to manage multiple machines without manually logging into each one.
Differences between `Invoke-Expression` and `Invoke-Command`:
- `Invoke-Expression` executes commands in the local session.
- `Invoke-Command` can leverage remoting capabilities to execute commands across several machines in a network.
Remote Execution:
You can invoke commands on a remote machine by specifying the `-ComputerName` parameter. Here’s how it works:
Invoke-Command -ComputerName Server01 -ScriptBlock { Get-Service }
This example retrieves the list of running services from `Server01` without needing to log into the machine directly.
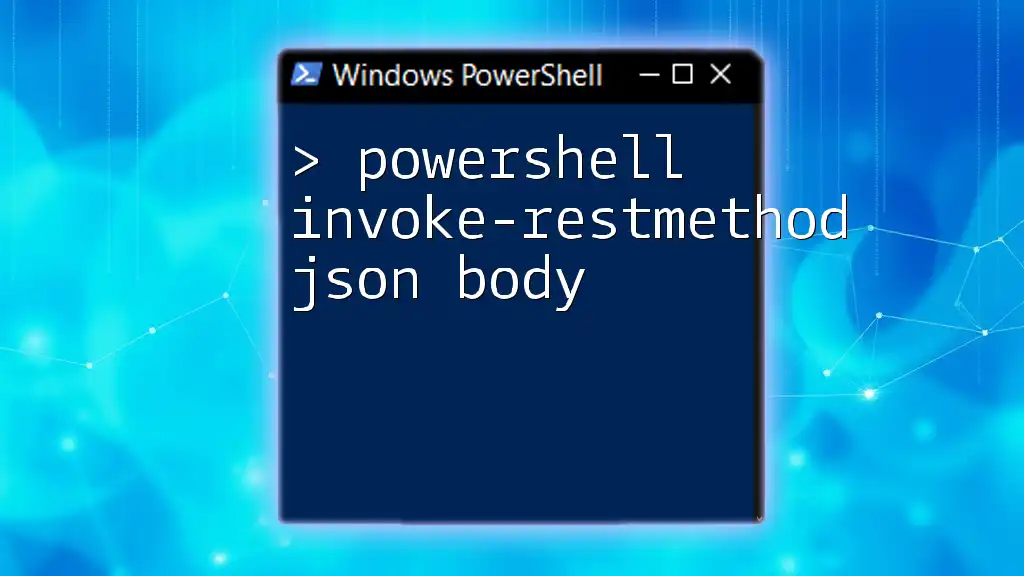
Practical Applications of PowerShell Invoke
Automating Administrative Tasks
`PowerShell.Invoke` can automate a variety of administrative tasks such as monitoring services, gathering system information, or applying configurations across multiple systems. This enhances efficiency and reduces manual errors significantly.
Script Development
`Invoke` commands allow for streamlined script writing. Instead of having cumbersome inline script blocks, you can design scripts that dynamically generate commands and execute them using `Invoke`.
Error Handling with Invoke
Best Practices for Error Catching: Always implement error handling to make your scripts robust. PowerShell’s try-catch-finally construct helps manage exceptions elegantly.
Example of using try-catch with `Invoke` commands:
try {
Invoke-Command -ComputerName Server01 -ScriptBlock { Get-Service }
} catch {
Write-Host "An error occurred: $_"
}
In this snippet, should a command fail, the error message will be displayed without crashing the script, allowing you to log or respond to the issue accordingly.
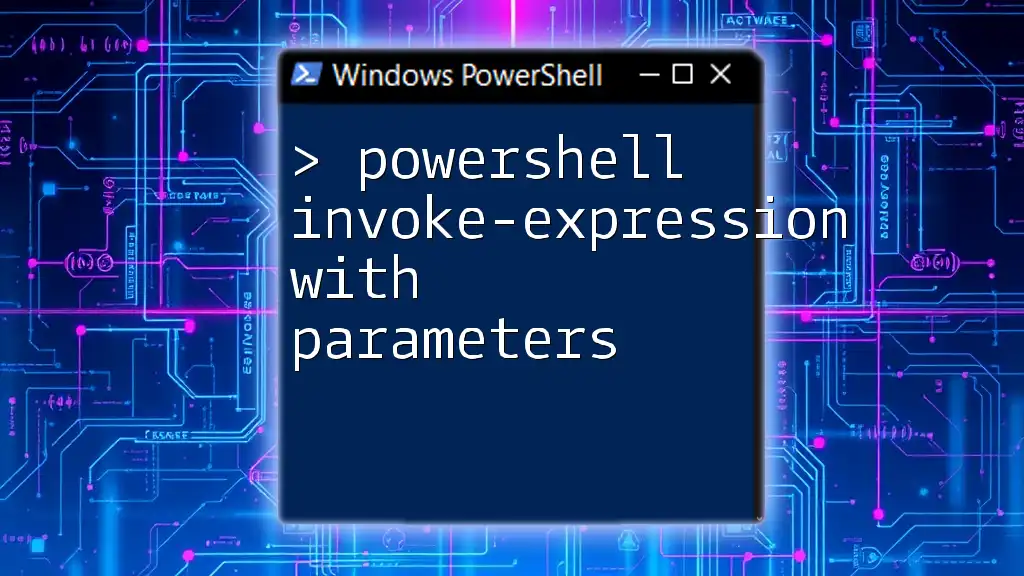
Advanced Use of PowerShell Invoke
Combining Tools and Technologies
PowerShell is versatile and integrates exceptionally well with other tools. With `Invoke`, you can execute commands against APIs using `Invoke-RestMethod`. It allows your scripts to interact with web services smoothly.
An example of calling a web API using `Invoke-RestMethod`:
$response = Invoke-RestMethod -Uri 'https://api.example.com/data'
This command fetches data from the specified API, enabling you to automate workflows involving external systems easily.
Performance Considerations
When using `Invoke`, ensure you're leveraging it effectively for performance. Use it when commands require flexible execution or when running scripts across several servers. Be mindful of network latency and load on remote systems to avoid bottlenecks.
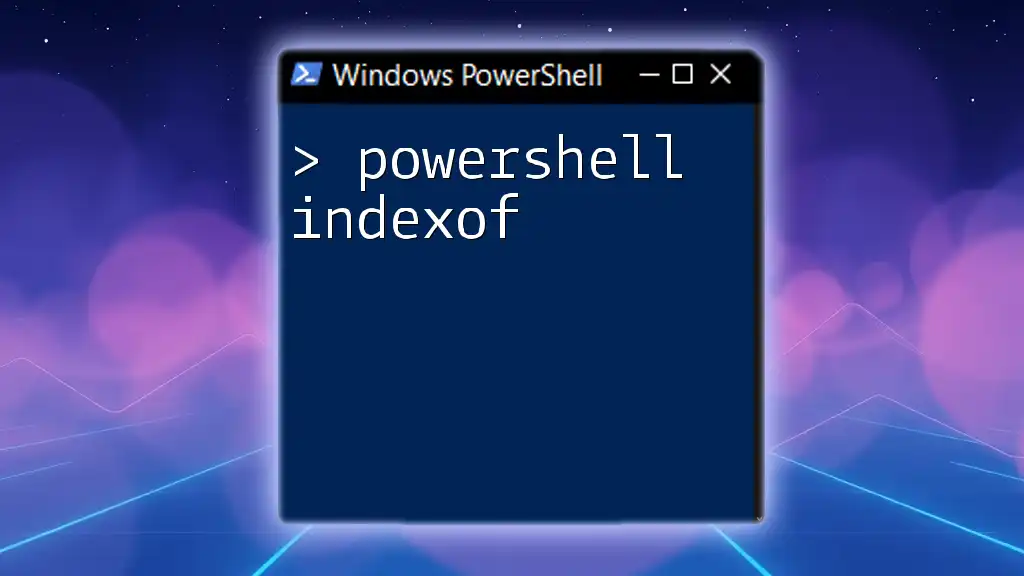
Conclusion
Understanding how to use `PowerShell.Invoke` effectively can streamline your workflows, enhance automation capabilities, and improve overall efficiency in managing systems. Experiment with these commands in a test environment to deeply grasp their power and potential.
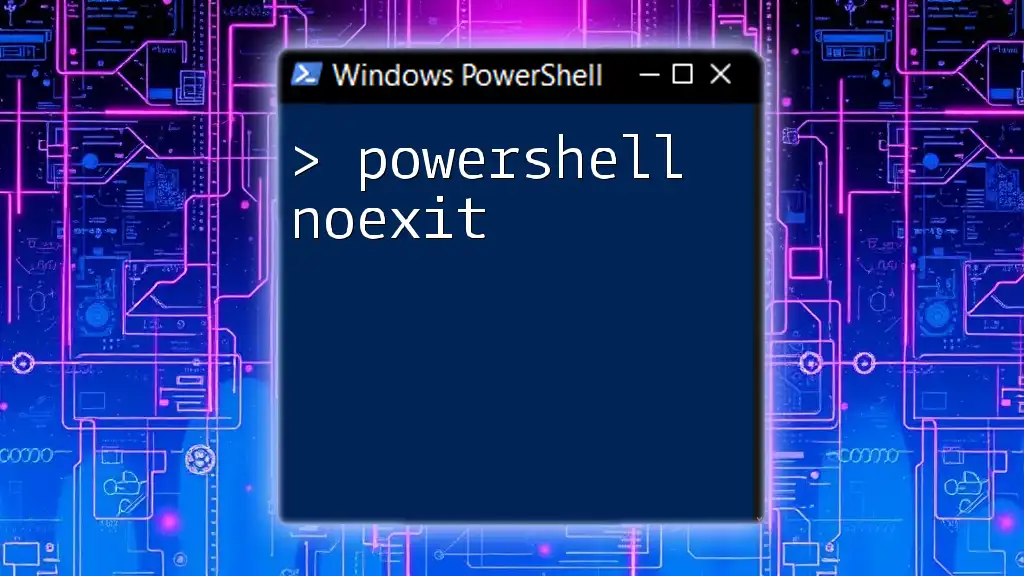
Additional Resources
For further learning, refer to the official PowerShell documentation, explore tutorials, and engage in communities dedicated to mastering PowerShell.
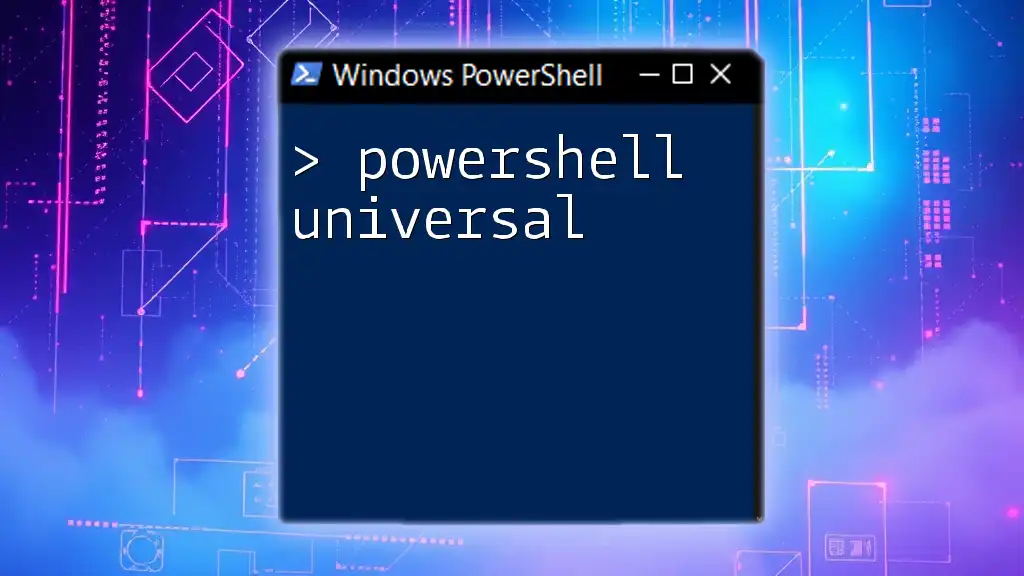
Frequently Asked Questions
You may find common inquiries regarding `Invoke`, addressing specific scenarios or troubleshooting steps invaluable as you deepen your understanding.
Remember, practice makes perfect; continually expand your skill set with `PowerShell.Invoke` through real-world applications, and consider enrolling in specialized training to further enhance your expertise.