The `Invoke-RestMethod` cmdlet in PowerShell is used to send HTTP requests to a RESTful API and can include a JSON body for creating or updating resources.
Here’s a code snippet demonstrating how to use `Invoke-RestMethod` with a JSON body:
$body = @{ name = "John Doe"; email = "john.doe@example.com" } | ConvertTo-Json
$response = Invoke-RestMethod -Uri 'https://api.example.com/users' -Method Post -Body $body -ContentType 'application/json'
Understanding PowerShell's Invoke-RestMethod
What is Invoke-RestMethod?
`Invoke-RestMethod` is a versatile cmdlet in PowerShell used to interact with REST APIs. It simplifies the process of sending requests to web services and processing responses. Unlike `Invoke-WebRequest`, which is more suited for downloading web pages and files, `Invoke-RestMethod` is specifically designed for working with structured data formats like JSON.
When you work with REST APIs, you might need to perform a variety of HTTP methods, such as GET, POST, PUT, and DELETE. `Invoke-RestMethod` caters to these needs, making it an essential tool for developers and system administrators alike.
Why Use JSON with REST APIs?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. Its simplicity and compactness make it a preferred choice for transmitting data between a client and a server, especially in web applications.
APIs that utilize JSON are prevalent across services like GitHub, Twitter, and many more. Using JSON allows for streamlined communication, reducing the overhead involved in data transmission. Here are some of the key benefits of using JSON for REST APIs:
- Readability: The structure of JSON is straightforward and intuitive, which makes it easy to understand.
- Data Types: JSON supports various data types like strings, numbers, arrays, and objects, allowing for complex data representations.
- Language Agnostic: JSON is supported by virtually all modern programming languages, facilitating compatibility.
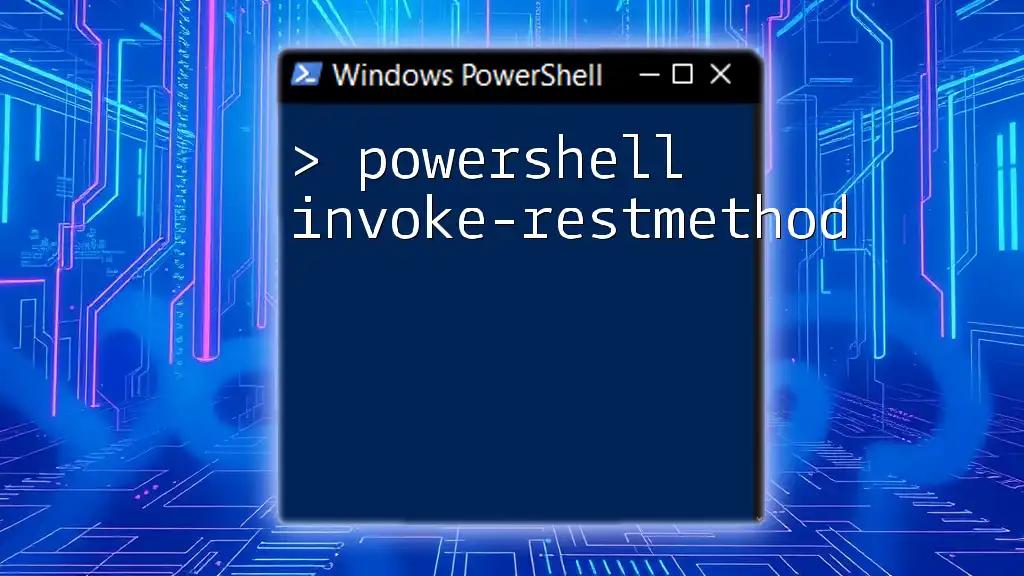
Setting Up Your PowerShell Environment
Installing PowerShell (if necessary)
Before you can make use of `Invoke-RestMethod`, ensure that your PowerShell environment is set up correctly. You can check your current version of PowerShell using the following command:
$PSVersionTable.PSVersion
If necessary, you can download the latest version from the official PowerShell GitHub page and follow the installation instructions provided there.
Required Modules and Permissions
In most cases, `Invoke-RestMethod` is available by default in Windows PowerShell 3.0 and later. However, it's important to ensure that your execution policy allows for script running. You can check the execution policy with:
Get-ExecutionPolicy
You may need to set it to `RemoteSigned` or `Unrestricted` if you plan on running scripts that include REST API calls:
Set-ExecutionPolicy RemoteSigned
Ensure you have the necessary permissions to access the APIs you intend to work with, particularly for POST and other methods that affect server data.
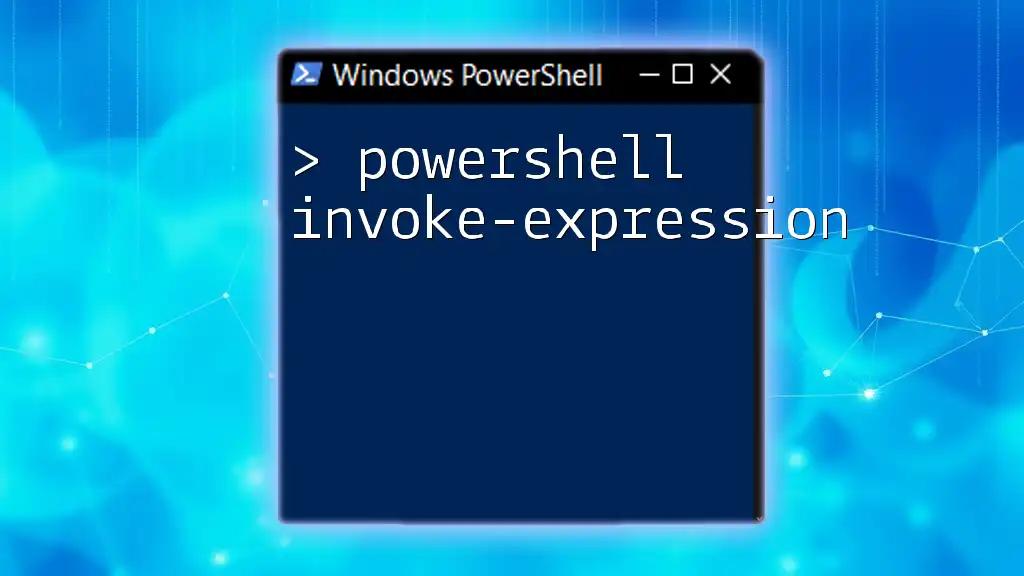
Basics of Using Invoke-RestMethod
Syntax of Invoke-RestMethod
The syntax of `Invoke-RestMethod` is straightforward yet flexible. Here’s the foundational command structure:
Invoke-RestMethod -Uri <URI> -Method <HTTP_Method> -Body <Body> -Headers <Headers>
The key parameters include:
- `-Uri`: The endpoint you wish to interact with.
- `-Method`: The HTTP method (`GET`, `POST`, etc.).
- `-Body`: The data you are sending (for POST/PUT requests).
- `-Headers`: Any custom headers required by the API.
Performing a GET Request
Let’s explore a simple GET request. A GET request is used to retrieve data from a specified resource. Here’s an example of how to fetch data from a public API:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method GET
$response
In this command, `Invoke-RestMethod` fetches data from the provided URI. The JSON response is automatically parsed into PowerShell objects, allowing you to access properties easily.
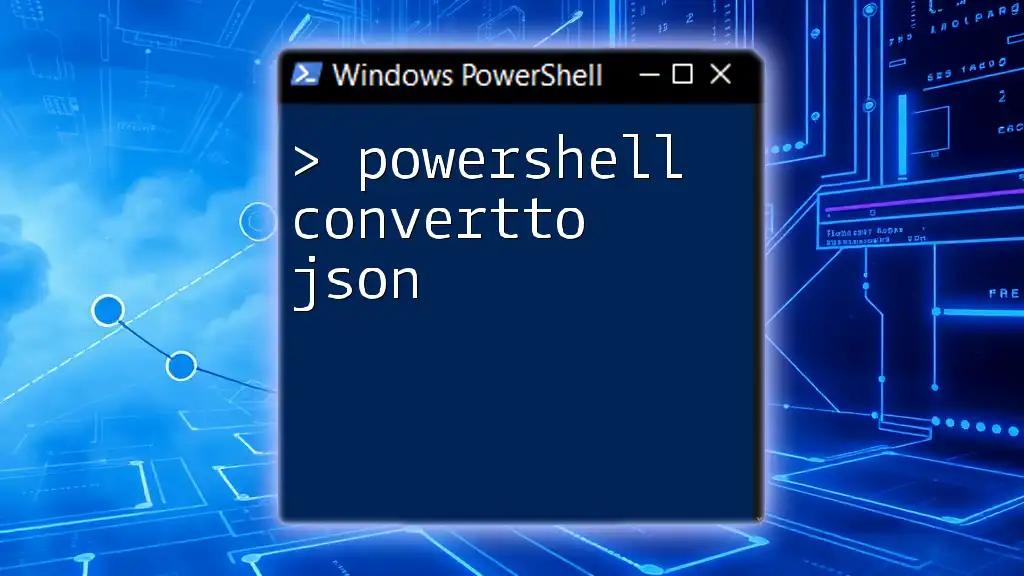
Working with JSON Bodies in PowerShell
Creating JSON Data
When sending data to an API, particularly with POST requests, it's common to structure that data in JSON format. You can create a JSON object in PowerShell using a hashtable and the `ConvertTo-Json` cmdlet.
$jsonBody = @{ name = "John Doe"; age = 30 } | ConvertTo-Json
This command creates a hashtable with user details and converts it to a JSON string. It's essential to ensure that the JSON structure is valid, as incorrect formatting can result in errors.
Sending a POST Request with JSON Body
One of the common uses of `Invoke-RestMethod` is to send data to a server using a POST request. Here’s how to do it:
$response = Invoke-RestMethod -Uri "https://api.example.com/create" -Method POST -Body $jsonBody -ContentType "application/json"
In this example, the `-Body` parameter sends the `jsonBody` created earlier, and the `-ContentType` specifies that the data format is JSON. The server’s response can also be processed easily, as it is returned as a PowerShell object.
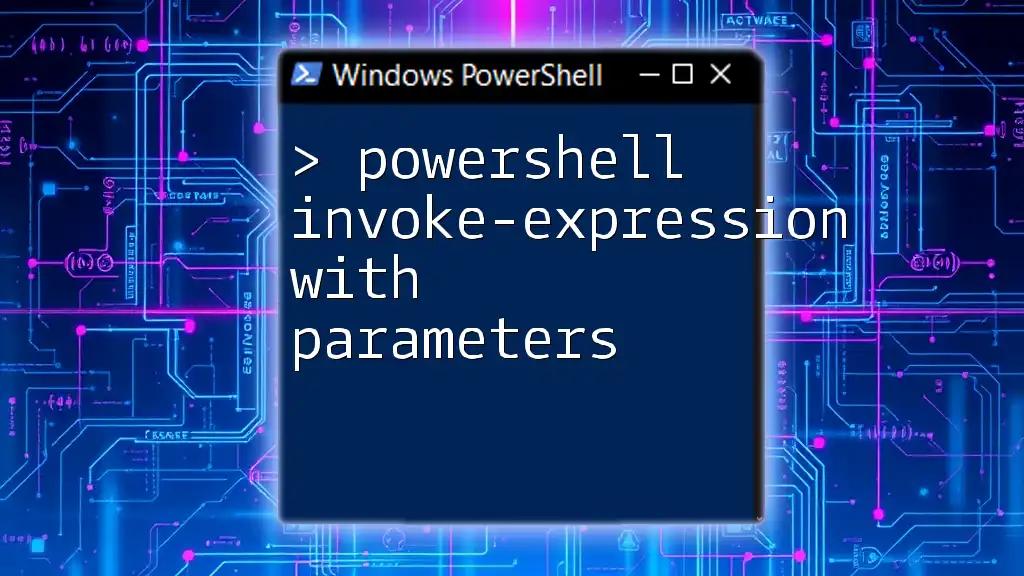
Handling Responses and Errors
Understanding Response Codes
When you interact with REST APIs, understanding the response codes is crucial. Here are some common HTTP response codes you might encounter:
- 200 OK: The request has succeeded.
- 201 Created: The request has been fulfilled and a new resource has been created.
- 400 Bad Request: The server could not understand the request due to invalid syntax.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: The server encountered an error while processing the request.
To check the response status in PowerShell, you can implement a simple conditional:
if ($response.StatusCode -eq 200) {
"Success!"
} else {
"Error: $($response.StatusCode)"
}
This will help you determine if your request was processed successfully.
Error Handling in PowerShell
Good practices in PowerShell involve handling potential errors gracefully. You can use the Try-Catch block to manage exceptions when calling `Invoke-RestMethod`.
try {
$response = Invoke-RestMethod -Uri "https://api.example.com/create" -Method POST -Body $jsonBody -ContentType "application/json"
}
catch {
Write-Host "An error occurred: $_"
}
This approach allows for better debugging and user feedback in case of issues during API calls.

Advanced Usage of Invoke-RestMethod
Customizing Headers
Often, REST APIs require specific headers, such as authorization tokens. Adding custom headers enhances the capabilities of your requests.
$headers = @{ "Authorization" = "Bearer YOUR_TOKEN" }
$response = Invoke-RestMethod -Uri "https://api.example.com/protected" -Method GET -Headers $headers
In this scenario, the `Authorization` header is critical for accessing protected resources, demonstrating how headers can be essential in secure API interactions.
Handling Complex JSON
When dealing with complex JSON structures or nested objects, you can still easily parse and manipulate the response data. For structured responses, simply access properties directly using dots.
Using Query Parameters with Invoke-RestMethod
To enhance your requests, you can append query parameters directly to the URI. This is commonly utilized in GET requests to filter results.
$response = Invoke-RestMethod -Uri "https://api.example.com/items?search=example" -Method GET
By appending `?search=example`, this command fetches specific data based on the provided search criteria.
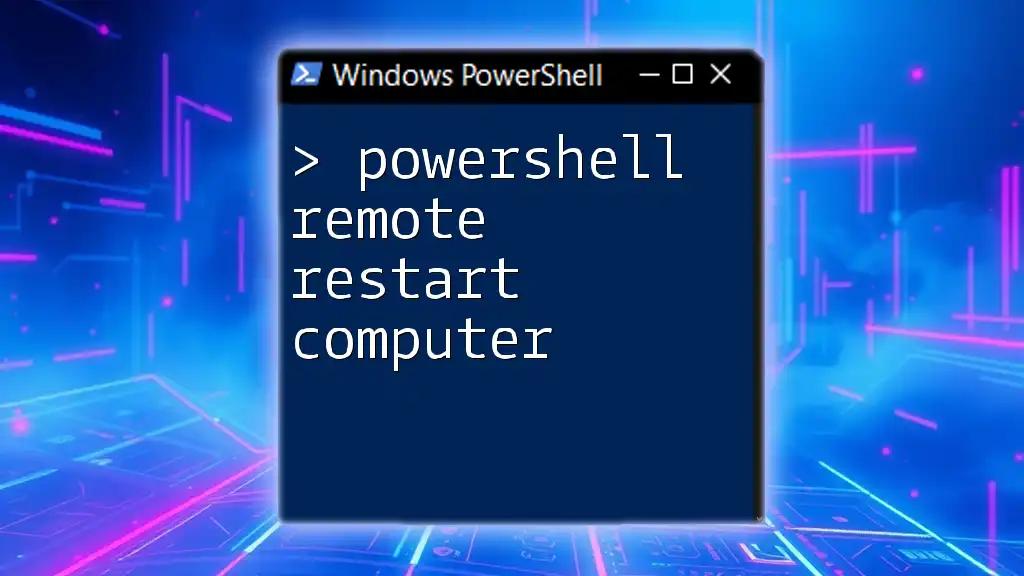
Best Practices for Using Invoke-RestMethod
Security Considerations
Security should always be a priority when working with APIs. Ensure to:
- Use HTTPS wherever possible to encrypt data in transit.
- Protect sensitive data such as API keys and tokens, storing them securely.
Performance Optimization
When interacting with large APIs or making multiple calls, performance can become an issue. Consider using `-AsJob` to run requests asynchronously, allowing your script to continue executing while waiting for responses:
$job = Invoke-RestMethod -Uri "https://api.example.com/data" -Method GET -AsJob
This helps reduce total execution time when working with multiple resources.
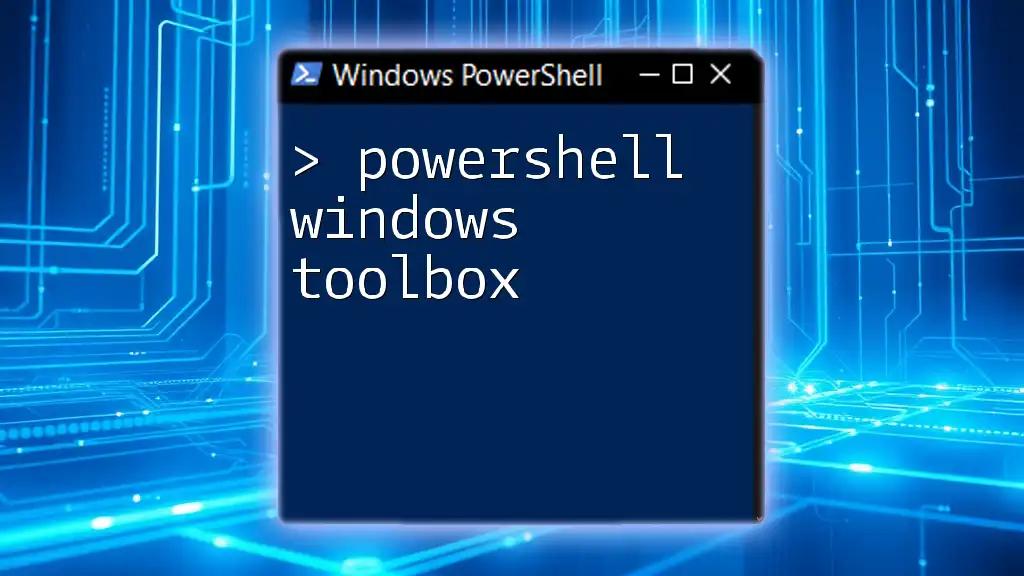
Conclusion
In this guide, we have covered the fundamentals of using `Invoke-RestMethod` in PowerShell to interact with JSON REST APIs. With a strong understanding of how to send requests, handle responses, and manage errors, you can efficiently integrate various web services into your PowerShell scripts.
Welcome to a powerful journey with PowerShell! With the skills you've gained, you are now ready to tackle even more complex API integrations effectively.