To convert a string to Base64 in PowerShell, you can use the following command which encodes the input string and outputs its Base64 representation:
[Convert]::ToBase64String([System.Text.Encoding]::UTF8.GetBytes("Hello, World!"))
Understanding PowerShell
PowerShell is a powerful scripting language and shell designed for system administration. It enables users to automate tasks and manage system configurations efficiently. It provides a wide range of commands (known as cmdlets) that can be combined and leveraged for complex operations.
In the context of data encoding, particularly with Base64, PowerShell offers convenient functionalities to convert strings and files seamlessly.
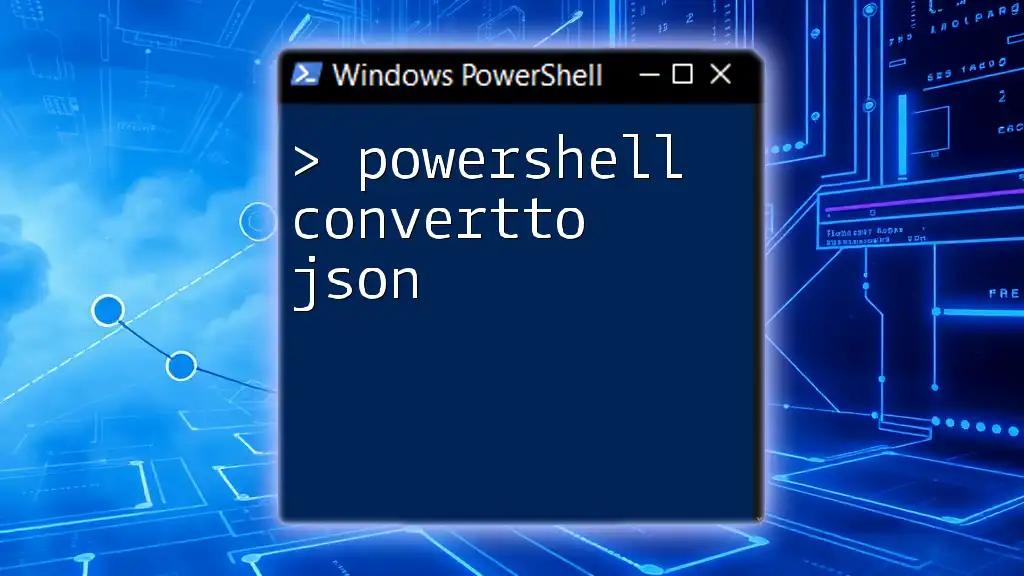
The Concept of Encoding
What Is Encoding?
Encoding is the process of converting data from one form to another. It's not the same as encryption; rather, it’s a way to transform data into a format that can be easily and consistently transmitted or stored. Base64 is a popular encoding scheme used primarily to encode binary data into ASCII characters. This is particularly useful for transmitting data over media designed to handle textual data.
Why Base64 Is Used for Data Representation
Base64 encoding encodes binary data into a string of ASCII characters. This is valuable when:
- Emailing attachments.
- Embedding image data directly into HTML or CSS.
- Storing complex data objects in JSON or XML formats.
Using Base64 ensures that data remains intact without modification during transmission.
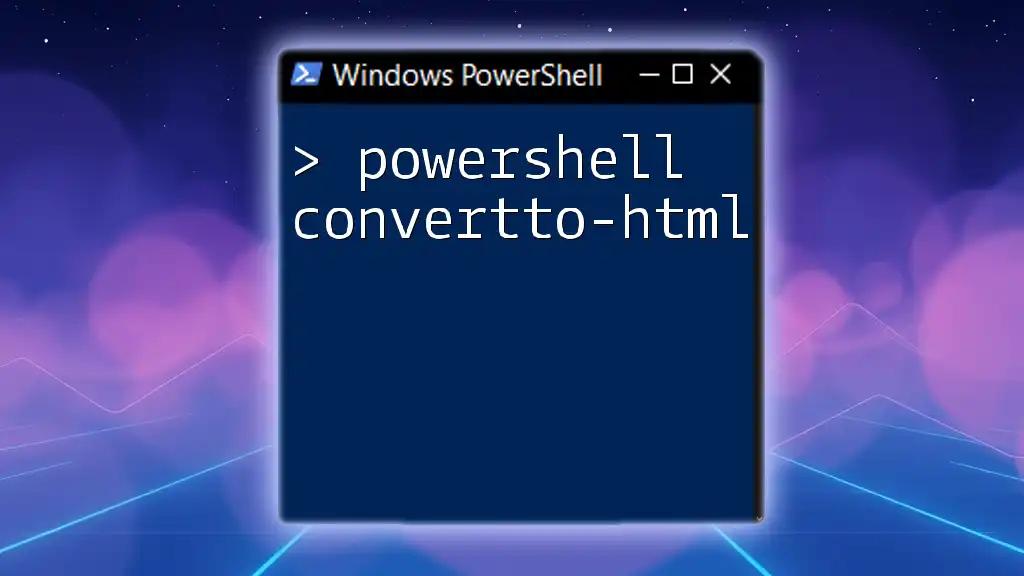
PowerShell and Base64
Why Use PowerShell for Base64 Conversion?
Using PowerShell for Base64 conversion is advantageous due to its straightforward cmdlets and rich scripting capabilities. PowerShell provides the necessary tools to handle Base64 encoding and decoding efficiently, making it an ideal choice for administrators and developers alike.
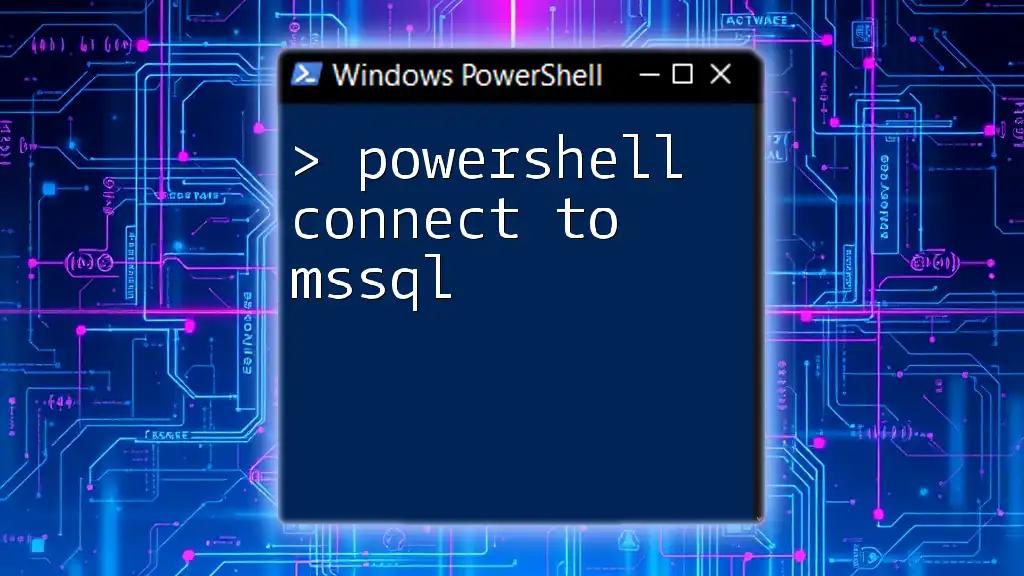
Converting Strings to Base64
Using `ConvertTo-Base64` Function
To perform Base64 conversion in PowerShell, you can create your own function. This allows for a concise and reusable method to convert strings to Base64.
Here’s a simple example of creating a function to convert a string to Base64:
function Convert-ToBase64 {
param (
[string]$inputString
)
[Convert]::ToBase64String([System.Text.Encoding]::UTF8.GetBytes($inputString))
}
Example Usage of the Function
You can apply the function as follows to convert a string to Base64:
$base64String = Convert-ToBase64 -inputString "Hello, World!"
Write-Output $base64String
This command will output the Base64 encoded string of "Hello, World!". Understanding how this works under the hood is crucial—the string is converted into bytes using UTF-8 encoding, and these bytes are transformed into a Base64 representation.
Explanation of the Code
The function takes an input string, converts it into an array of bytes using UTF-8 encoding, and then applies the `ToBase64String` method from the `[Convert]` class. Each of these pieces is essential for achieving the desired encoding.

Converting Files to Base64
Reading File Content
PowerShell can also be used to read the content of files and convert them into Base64 format. This is particularly useful for encoding files for transmission over systems that only accept text inputs.
Here’s how to read a file and convert its content into Base64:
$filePath = "C:\path\to\your\file.txt"
$fileContent = Get-Content -Path $filePath -Raw
$base64File = Convert-ToBase64 -inputString $fileContent
Example: Converting a File to Base64
Let’s assume you have a text file that contains the text "This is a test file." The steps above will take the content of this file, encode it as Base64, and store it in the `$base64File` variable.
Step-by-step Breakdown:
- Read the File: Get-Content retrieves the full content of the file specified by `$filePath`.
- Convert to Base64: By invoking the `Convert-ToBase64` function, you can easily encode the file into Base64 format.
Understanding the Output
The output will be a long string of Base64 encoded characters. This transformed data can be transmitted, stored, or embedded as needed while maintaining the original content integrity.

Decoding Base64 Back to Original
Creating a Function for Decoding
To decode Base64 strings back to their original form, you can make another function. Here’s how you can create a decoding function:
function Convert-FromBase64 {
param (
[string]$base64String
)
[System.Text.Encoding]::UTF8.GetString([Convert]::FromBase64String($base64String))
}
Example of Decoding Base64
To decode a previously encoded string, you can use the following:
$decodedString = Convert-FromBase64 -base64String $base64String
Write-Output $decodedString
Understanding the Output
The output of this command will be the original string that you encoded earlier, allowing you to verify that the conversion process works in both directions.
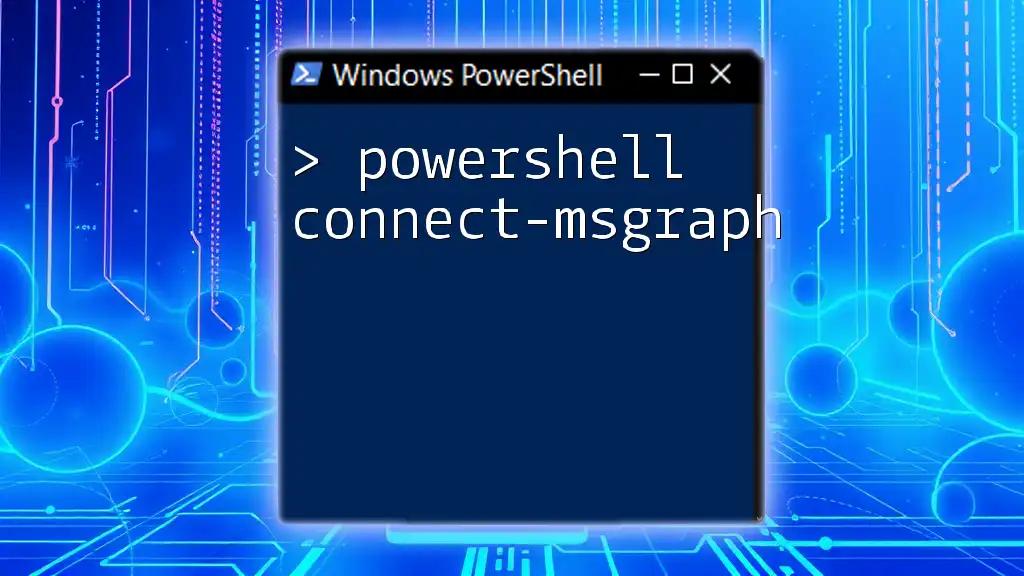
Common Errors and Troubleshooting
When working with Base64 encoding and decoding, users might encounter some common issues. Here are a few potential pitfalls:
- Invalid Base64 Strings: Make sure the string you are trying to decode is correctly encoded. Base64 strings must be a multiple of 4 characters, and they can only contain certain characters.
- Character Encoding Issues: Ensure that you are using the correct character encoding (UTF-8 is typically the standard).
- File Path Errors: Check for valid paths when reading file contents. Incorrect paths will lead to errors that can interrupt the conversion process.
If any issues arise, double-check your code syntax and input values.
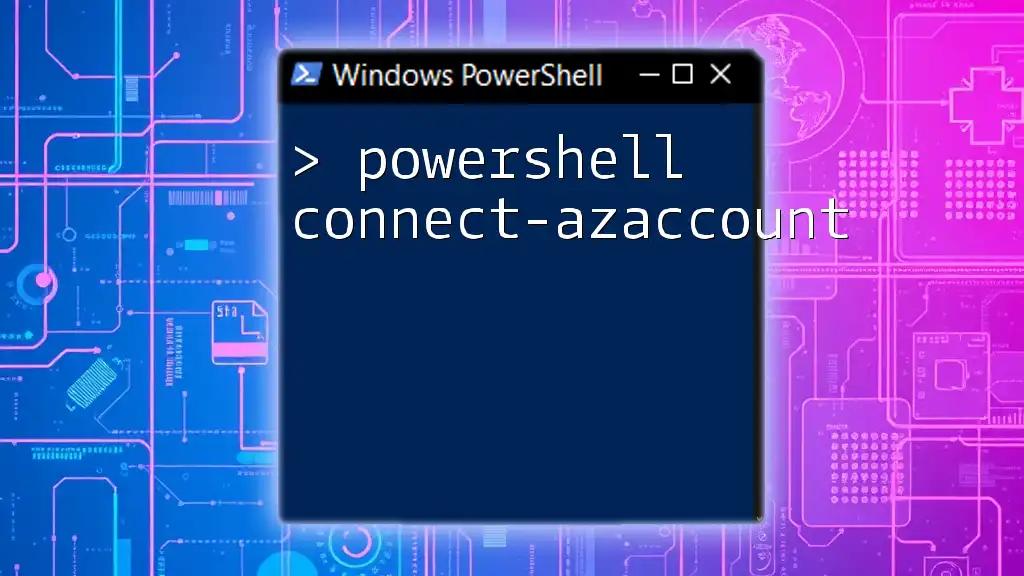
Conclusion
In this guide, we explored how to convert to Base64 using PowerShell effectively. From creating functions to convert strings and files, to decoding Base64 back into its original format, PowerShell makes these operations simple and efficient. Understanding these principles can significantly streamline your workflows, particularly in data handling and transmission.
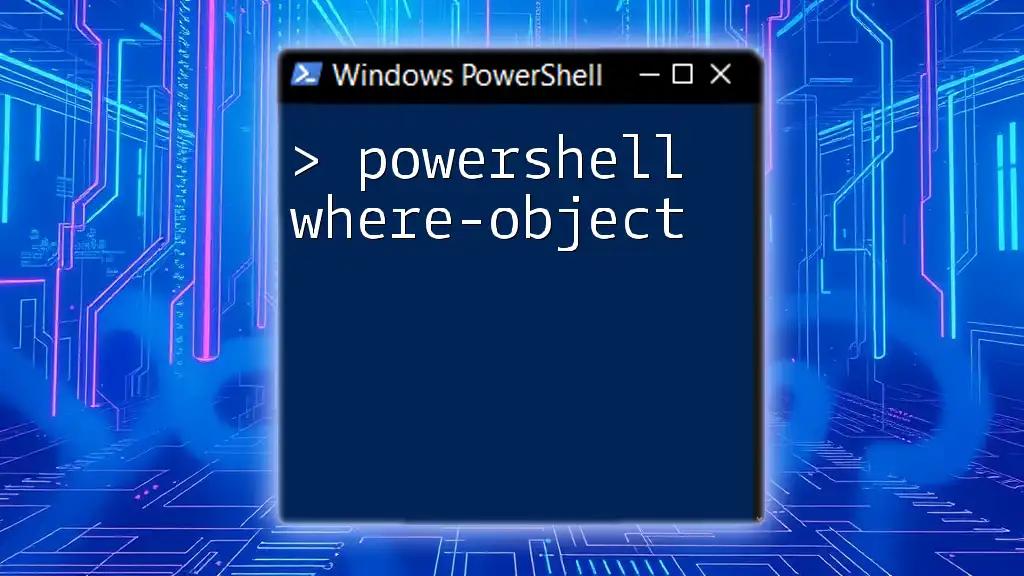
Additional Resources
To further expand your knowledge, consider exploring more about PowerShell functions and Base64 encoding through official documentation and online tutorials that can provide deeper insights into these topics.