To connect to a Microsoft SQL Server (MSSQL) database using PowerShell, you can utilize the `SqlClient` namespace from ADO.NET with the following code snippet:
$connectionString = "Server=your_server;Database=your_database;Integrated Security=True;"
$connection = New-Object System.Data.SqlClient.SqlConnection($connectionString)
$connection.Open()
Replace `your_server` and `your_database` with your actual server and database names.
Understanding MSSQL
What is Microsoft SQL Server?
Microsoft SQL Server is a relational database management system (RDBMS) developed by Microsoft. It is designed to store and retrieve data as requested by other software applications, which may run on the same computer or across a network. SQL Server is versatile, offering various editions ranging from free versions like SQL Server Express to enterprise-level solutions. Each edition is tailored to different users' needs, from individual developers to large enterprises.
Common Use Cases for MSSQL
MSSQL has a wide array of applications:
- Database management: Primarily used for storing and retrieving information for applications.
- Application development: Offers robust features that facilitate the development of custom applications.
- Data analysis: Allows organizations to analyze large datasets efficiently, providing insights that drive business decisions.
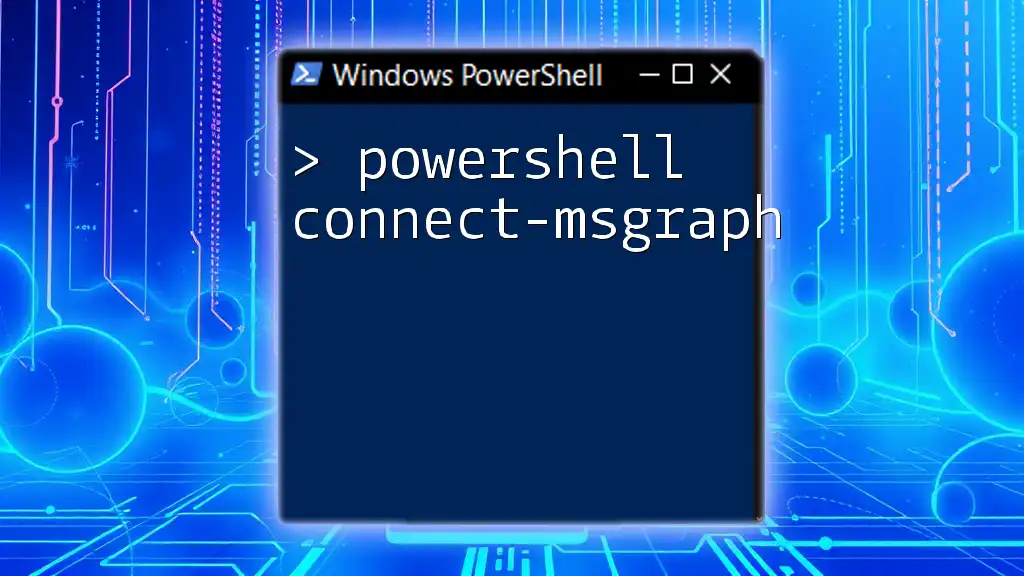
Setting Up Your Environment
Prerequisites for Connecting to MSSQL
Before diving into using PowerShell to connect to MSSQL, ensure that you meet the following prerequisites:
-
PowerShell Version: Make sure you have at least PowerShell 5.0 or higher. This ensures compatibility with various modules and command sets.
-
SQL Server Native Client: Check that the SQL Server Native Client is installed on your system. This component is essential for any kind of direct interaction with SQL Server.
-
Access Rights: You must possess appropriate permissions on the SQL Server you are attempting to connect to. Lack of proper permissions could lead to connection issues.
Installing SQL Server Management Studio (SSMS)
Installing SQL Server Management Studio (SSMS) is highly recommended for managing SQL Server connections effectively. Here’s a straightforward installation guide:
- Download: Go to the Microsoft website and download the appropriate version of SSMS for your system.
- Install: Follow the installation instructions to set it up on your machine.
- Launch: After installation, launch SSMS and ensure you can connect to your desired SQL Server instance.
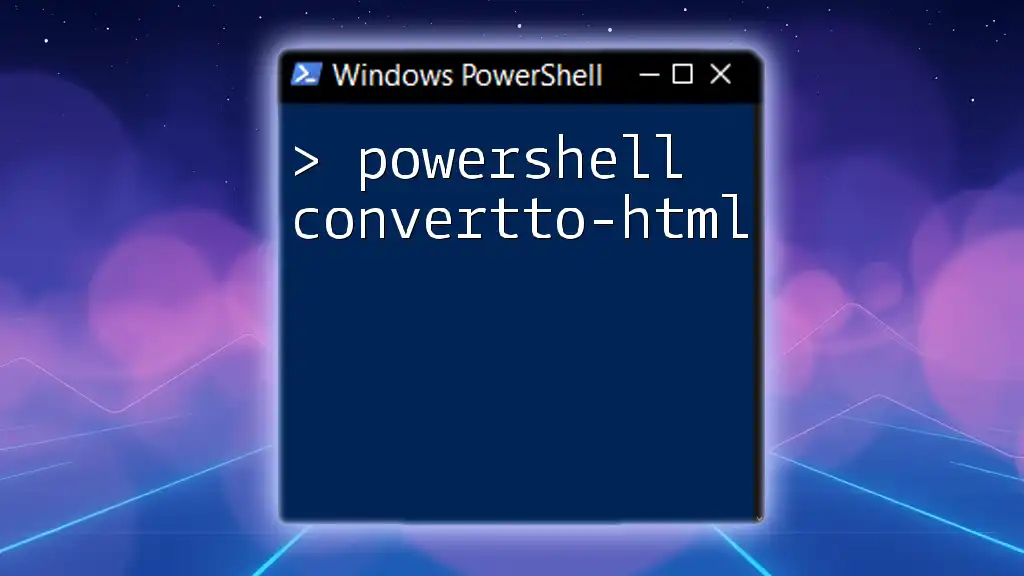
Connecting to MSSQL Using PowerShell
Using SQL Server Management Objects (SMO)
Introduction to SMO
SQL Server Management Objects (SMO) are a collection of objects designed for programming all aspects of managing Microsoft SQL Server. SMO simplifies the process of managing SQL Server, as it allows users to use a rich object-oriented programming model.
How to Install
To use SMO in PowerShell, you need to install the SQLServer module. Here’s how you can do it:
Install-Module -Name SqlServer -AllowClobber -Force
This command installs the SQLServer module, which contains SMO and other helpful cmdlets for interacting with SQL Server.
Basic Connection Syntax
Connecting to an MSSQL database involves forming a connection string that specifies details about the server:
$sqlConnection = New-Object System.Data.SqlClient.SqlConnection
$sqlConnection.ConnectionString = "Server=SERVER_NAME;Database=DATABASE_NAME;Integrated Security=True;"
Replace `SERVER_NAME` and `DATABASE_NAME` with the actual server and database names.
Establishing the Connection
Using Windows Authentication
Windows Authentication is a secure way to connect to SQL Server if your system is part of a domain:
$sqlConnection.Open()
Grouping the above code into a logical flow ensures you're successfully opening the connection.
Using SQL Server Authentication
If you prefer SQL Server authentication, adjust the connection string:
$sqlConnection.ConnectionString = "Server=SERVER_NAME;Database=DATABASE_NAME;User Id=USER_NAME;Password=PASSWORD;"
$sqlConnection.Open()
Make sure to replace `USER_NAME` and `PASSWORD` with your actual SQL credentials.
Error Handling in Connections
Even experienced users face errors. Implement error handling to manage connection issues effectively:
try {
$sqlConnection.Open()
# Your code goes here
}
catch {
Write-Host "Error: $_"
}
Using a `try-catch` block allows you to capture any exceptions and act accordingly, thereby improving your script's reliability.
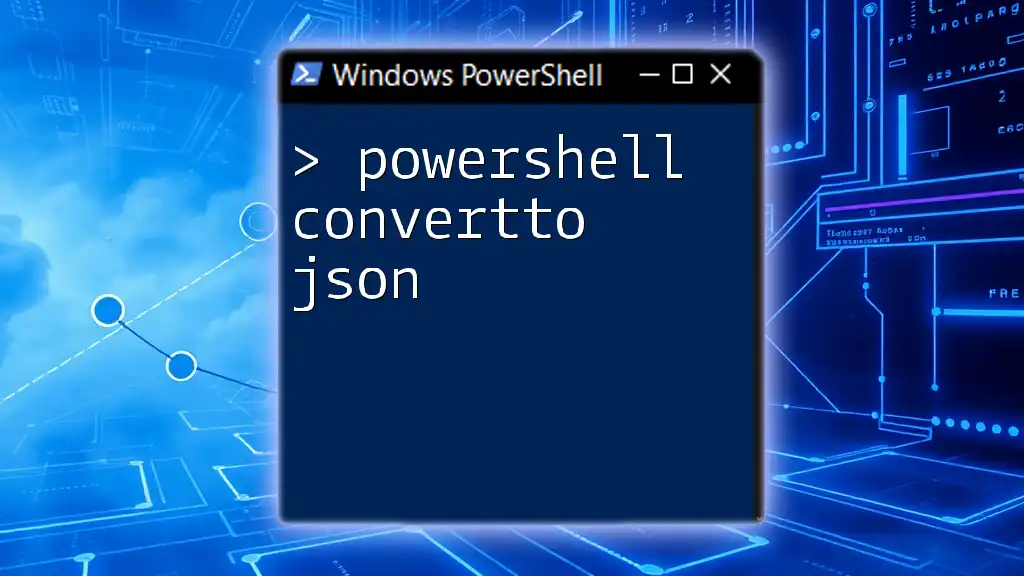
Executing SQL Queries
Basic Query Execution
With the connection established, you can start executing SQL queries. The `SqlCommand` class is essential for sending SQL commands to the database:
Using `SqlCommand`
You can create your command and execute it as shown below:
$command = $sqlConnection.CreateCommand()
$command.CommandText = "SELECT * FROM YourTable"
$reader = $command.ExecuteReader()
Fetching and Displaying Data
To read the results from your SQL command, loop through the returned data:
while ($reader.Read()) {
Write-Host $reader["ColumnName"]
}
$reader.Close()
In this scenario, replace `ColumnName` with the actual name of the column you wish to display. Closing the reader after use is crucial for resource management.
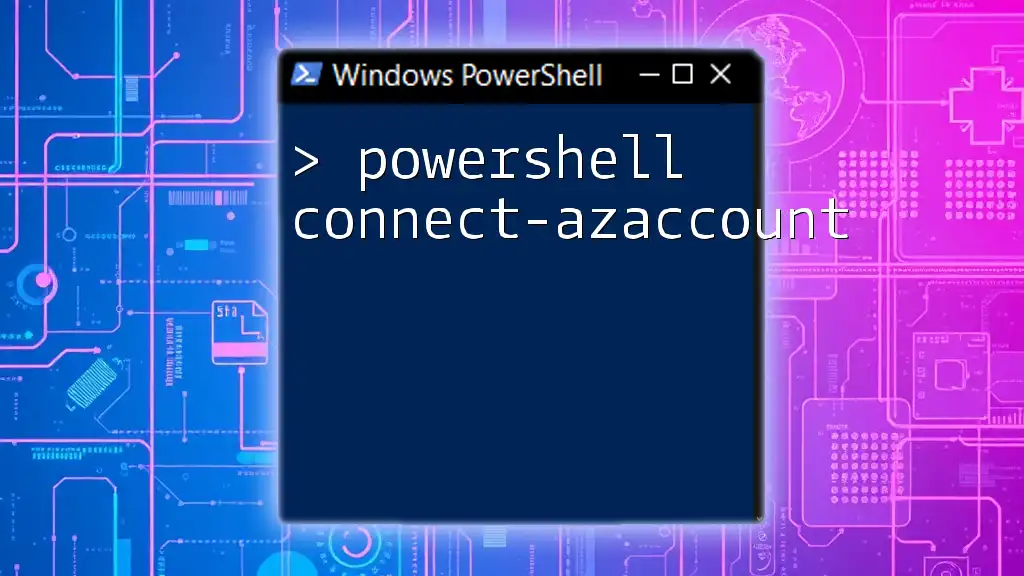
Closing the Connection
Always remember to close your SQL connection once you're done, as this frees up resources:
$sqlConnection.Close()
Best practice recommends wrapping opening and closing connections in a `try-catch-finally` block to ensure the connection is closed even if an error occurs.
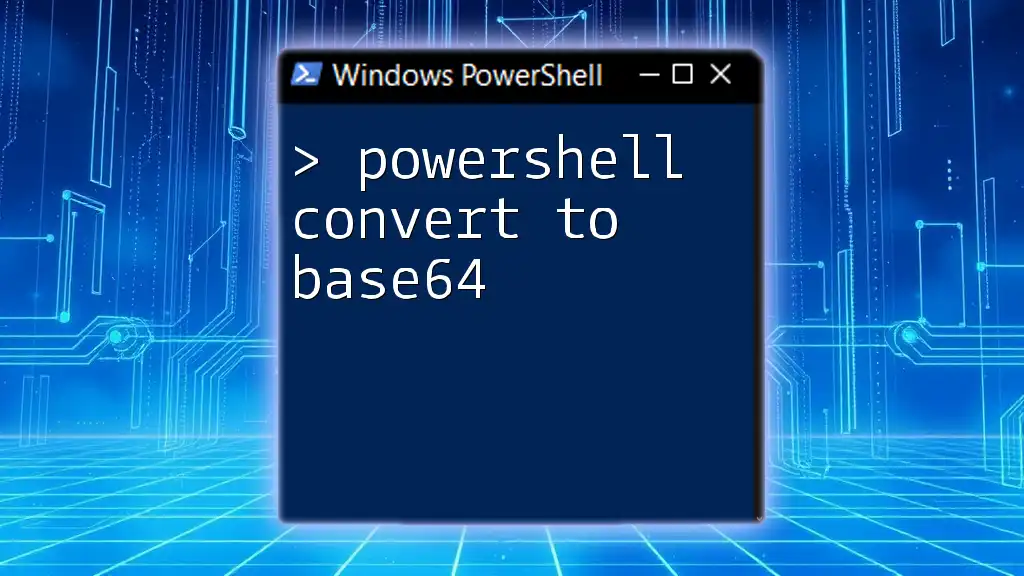
Performance Tips
Best Practices for Connection Management
To optimize performance when working with MSSQL connections:
-
Connection pooling: Utilize connection pooling, which reduces overhead and optimizes reuse of active connections.
-
Using `using` statements: Employ `using` statements to automatically dispose of the connection and any related resources.
Optimizing SQL Queries
Writing efficient SQL queries is essential. Consider using indexes, proper JOINs, and filtering techniques to minimize processing time. Familiarize yourself with query execution plans to identify bottlenecks in your SQL commands.
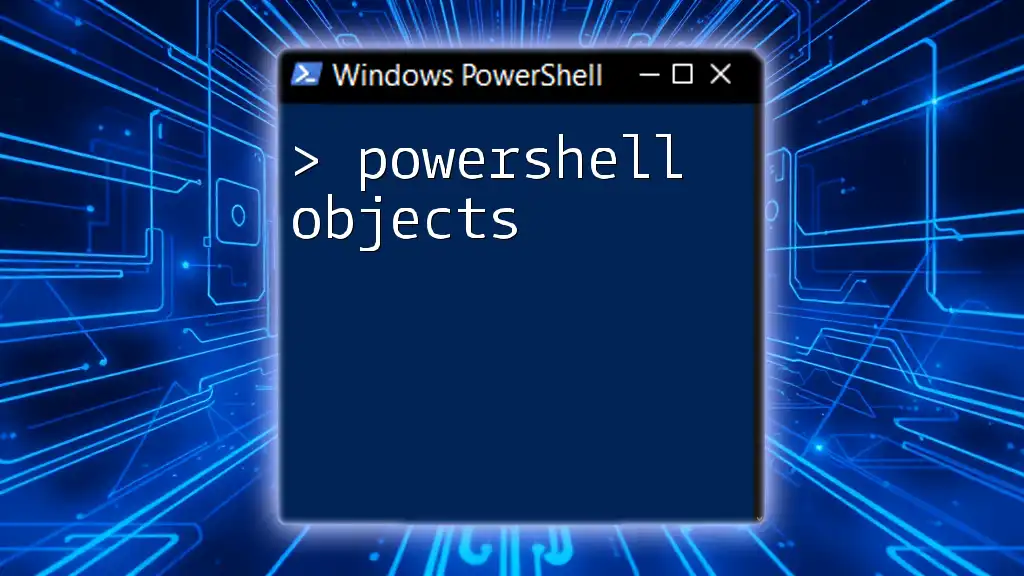
Troubleshooting Common Issues
Connection Errors
If you encounter connection errors, verify the following:
- Ensure that the SQL Server service is running.
- Check the firewall settings to allow connections on the SQL Server port (default is 1433).
- Confirm that the instance name is correct when connecting to a SQL Server instance.
SQL Command Errors
SQL command errors may arise due to syntax issues or database structure changes. Always review the command text you are executing to ensure it is valid.
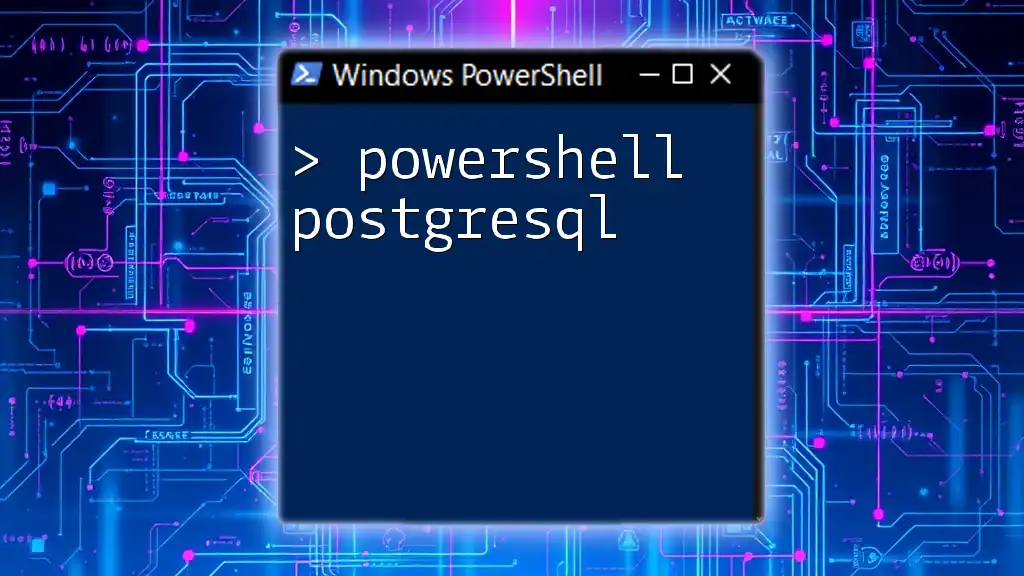
Conclusion
Mastering how to PowerShell connect to MSSQL can significantly enhance your database management tasks. By following the guidance provided in this article, you can efficiently establish connections, execute queries, handle errors, and close connections properly. Practicing these techniques will not only improve your PowerShell skills but also optimize your workflow when managing SQL Server databases.
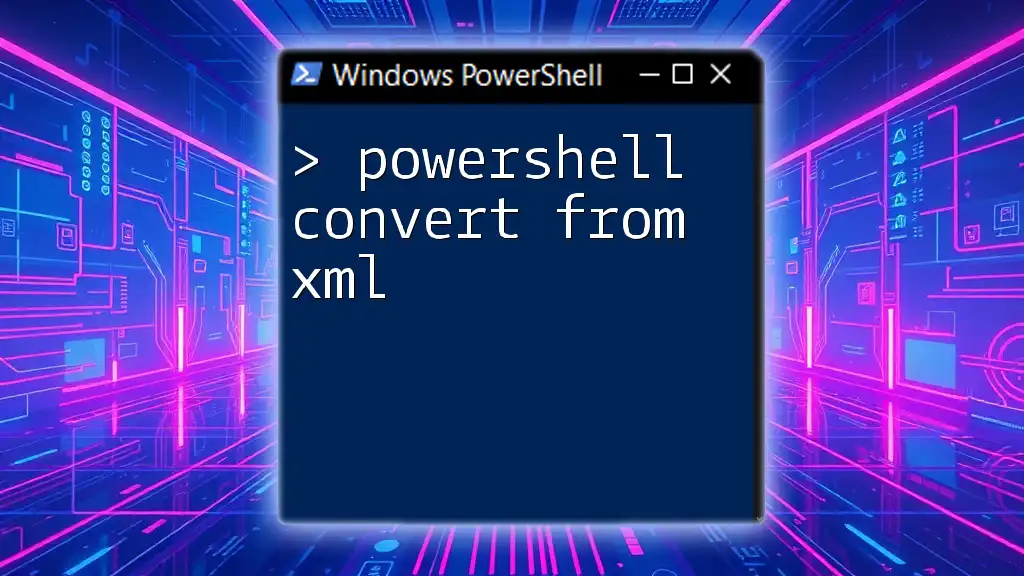
Additional Resources
To deepen your understanding and expertise in PowerShell and MSSQL, consider exploring documentation, community forums, and online courses tailored to both beginner and advanced users. Books and comprehensive tutorials are also available to provide detailed insights and hands-on learning experiences.