To connect to a remote computer and execute a command using PowerShell, you can use the `Invoke-Command` cmdlet with the `-ComputerName` parameter.
Here's a code snippet to demonstrate this:
Invoke-Command -ComputerName 'RemotePC' -ScriptBlock { Get-Process }
Understanding PowerShell Remoting
What is PowerShell Remoting?
PowerShell remoting is a feature that allows you to run commands on remote computers as if they were executed on your local system. It simplifies administrative tasks and enhances efficiency in managing multiple machines, making it an essential skill for IT professionals. Unlike traditional methods such as Remote Desktop Protocol (RDP), PowerShell remoting enables you to seamlessly and efficiently automate processes across your network.
Requirements for PowerShell Remoting
To effectively utilize PowerShell remoting, ensure that you have:
- PowerShell version compatibility: Make sure you are using a version that supports remoting (Windows PowerShell 2.0 and later).
- Network considerations: Verify that firewall settings permit communication between your local machine and the remote computer. By default, PowerShell remoting uses TCP ports 5985 for HTTP and 5986 for HTTPS.
- User permissions: You must have administrative rights on the remote machine, which means the user account needs appropriate permissions.
Key Components of PowerShell Remoting
PowerShell remoting relies on a couple of critical components:
- WinRM (Windows Remote Management): This is a service that enables the remote management of Windows machines. It’s crucial for establishing connections between your local and remote systems.
- PowerShell Session (PSSession): A PSSession is a persistent connection to a remote system, allowing multiple commands to be executed without re-establishing the connection.
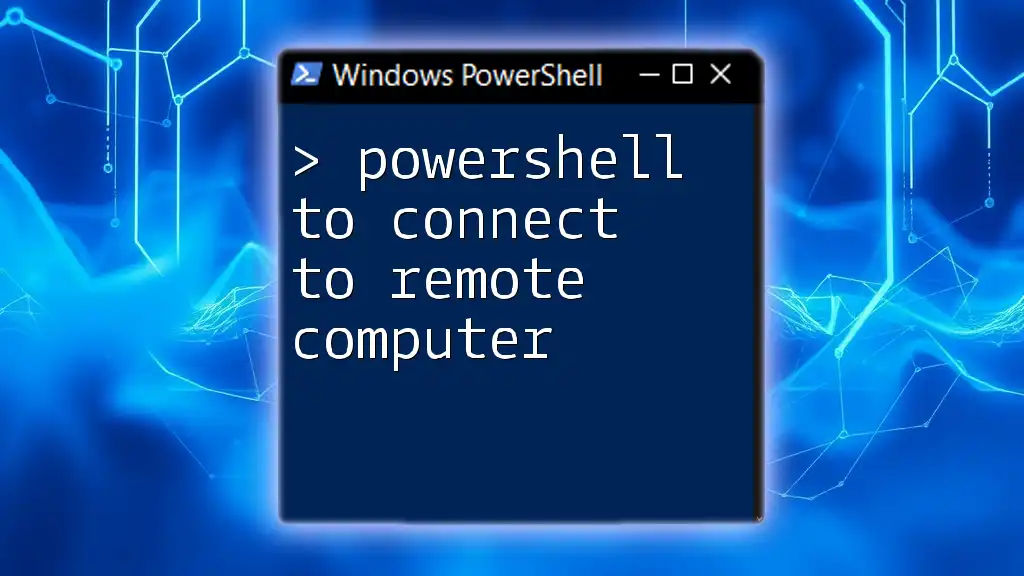
Setting Up PowerShell Remoting
Enabling PowerShell Remoting
Before you can connect to a remote computer and run commands, you need to enable remoting on your local machine. You can do this by opening PowerShell as an administrator and running the following command:
Enable-PSRemoting -Force
The `-Force` parameter ensures the command executes without prompting for confirmation, making the process smoother, especially for bulk operations.
Configuring the Remote Computer
For successful remote access, the remote computer must also be configured properly:
-
Firewall settings: Ensure that the appropriate ports are open. You can use this command to allow the necessary traffic for WinRM:
Set-NetFirewallRule -Name "WINRM-HTTP-In-TCP" -Enabled True
-
User configurations: Confirm that the user accounts have proper permissions to establish a remote session, which is essential for running commands remotely.
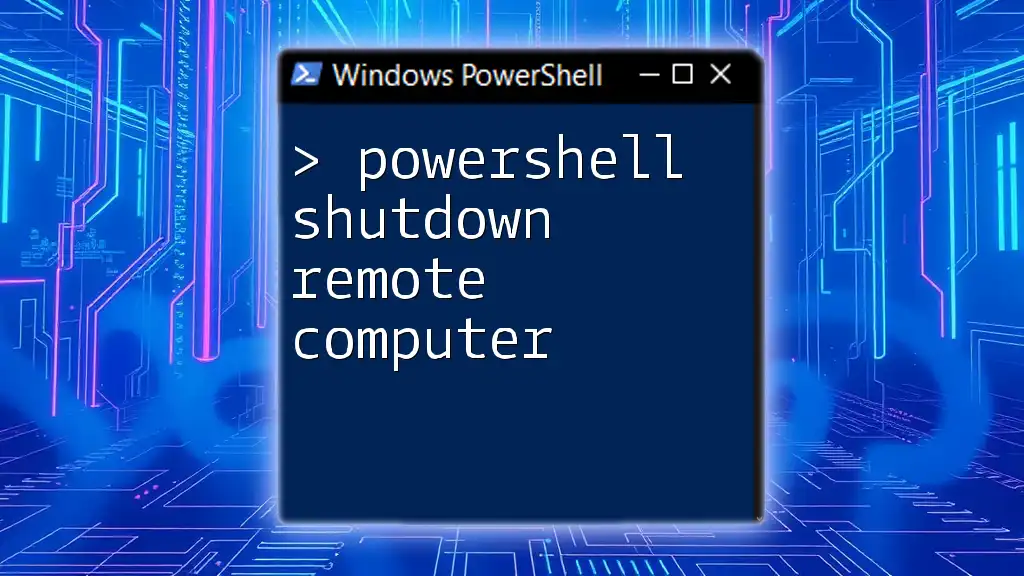
Connecting to a Remote Computer
Establishing a Remote Session
You can establish a remote session using the `Enter-PSSession` command, which creates an interactive session with a single remote computer. Here’s an example:
Enter-PSSession -ComputerName RemotePC -Credential (Get-Credential)
In this command, `RemotePC` is the name of the remote computer, while `(Get-Credential)` prompts you to enter the username and password for authentication. The credentials must belong to a user who has administrative rights on the remote machine.
Using `Invoke-Command` for Multiple Commands
For executing commands across multiple remote computers or running script blocks, `Invoke-Command` is your best option. It allows you to run a command without entering a full interactive session. Here’s how you can execute a command to retrieve processes from a remote machine:
Invoke-Command -ComputerName RemotePC -ScriptBlock { Get-Process }
In this example, `-ScriptBlock` allows you to specify a block of PowerShell commands to execute on the remote system.
Example: Running a Script on a Remote Computer
If you have a script that you want to execute on a remote machine, you can use the `-FilePath` parameter with `Invoke-Command`. Here’s a step-by-step example:
Invoke-Command -ComputerName RemotePC -FilePath "C:\Path\To\YourScript.ps1"
This command will run the specified PowerShell script located on your local machine on the remote computer.
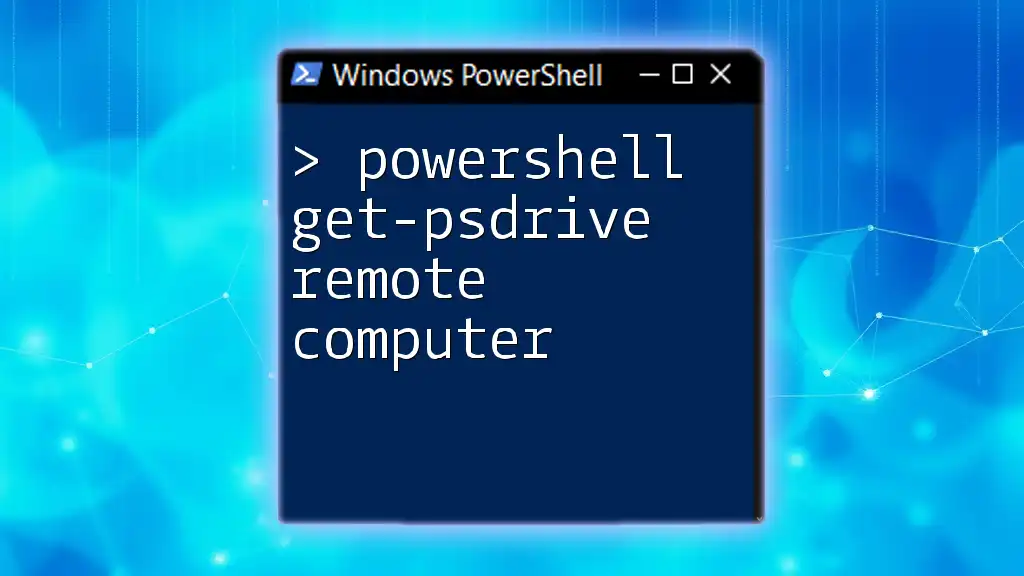
Managing Remote Sessions
Viewing Active Sessions
Once you have established connections, it often becomes necessary to manage them effectively. To view all active remote sessions, you can use:
Get-PSSession
This command will list all established sessions, allowing you to monitor their statuses.
Disconnecting and Closing a Session
Once you’re done executing commands, it’s a good practice to disconnect and close your sessions. You can disconnect a session using:
Disconnect-PSSession -Session $session
Properly managing your sessions is crucial to conserve resources and maintain system performance.
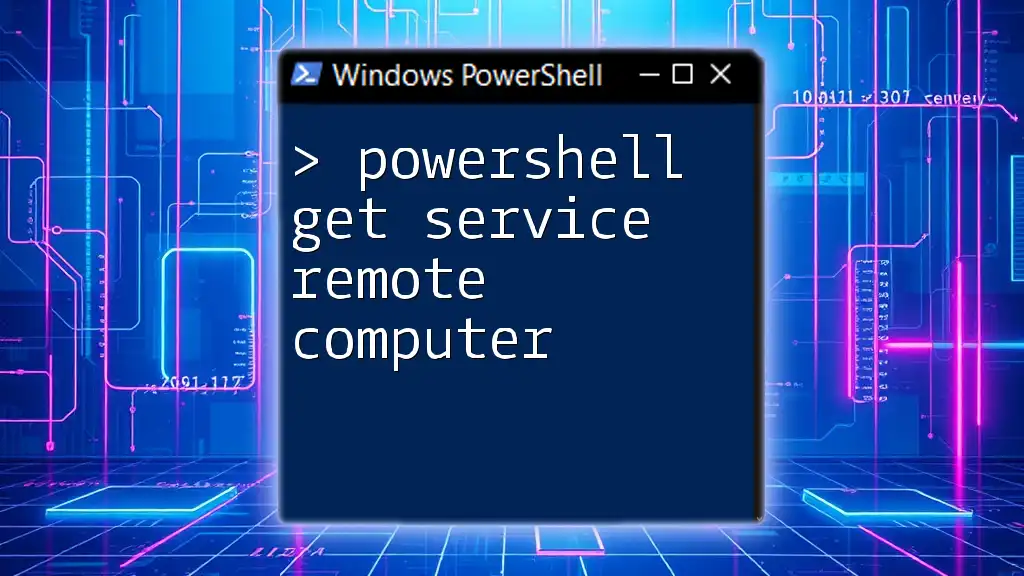
Troubleshooting Remote Connections
Common Issues and Solutions
As with any system, you may encounter issues when attempting to run commands on a remote computer. Here are a few common problems:
- Permission denied error: This usually indicates that the user account you used does not have administrative access on the remote machine.
- Network issues: If you can’t reach the remote computer, check your network connection and ensure proper configuration.
- Misconfigured WinRM: Verify that the WinRM service is running and configured correctly on both local and remote machines.
Testing Connectivity
If you encounter connectivity issues, using the `Test-WSMan` command can help. This command checks whether the WinRM service is running and accessible on the remote machine:
Test-WSMan -ComputerName RemotePC
Analyzing the output will provide clues to any underlying issues and how you might resolve them.
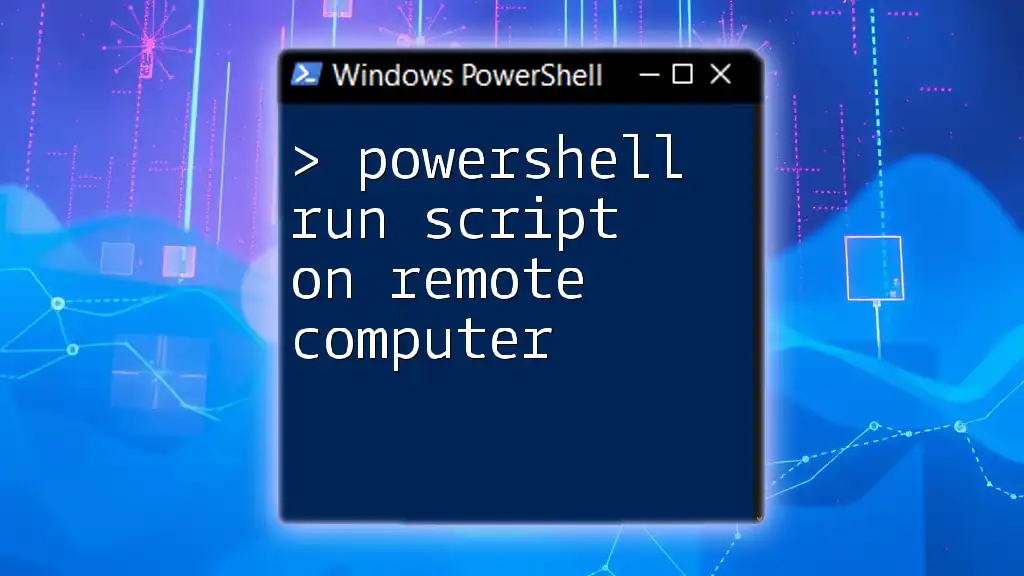
Best Practices for PowerShell Remoting
Security Considerations
When dealing with remote connections, security should always be a top priority. Here are some best practices:
- Use secure connections: Whenever possible, opt for HTTPS instead of HTTP to encrypt your communication.
- Credential management: Avoid hardcoding credentials in scripts; utilize credentials stored securely or prompting for input.
- Implement Just Enough Administration (JEA): This allows you to delegate specific permissions to users without granting full administrative rights.
Efficient Command Execution
For commands that take a long time to process, consider using background jobs. This allows you to continue working in the PowerShell session while the command executes in the background. You can start a job like this:
Start-Job -ScriptBlock { Get-Process }
Leveraging jobs not only enhances productivity but also allows you to manage multiple tasks simultaneously.
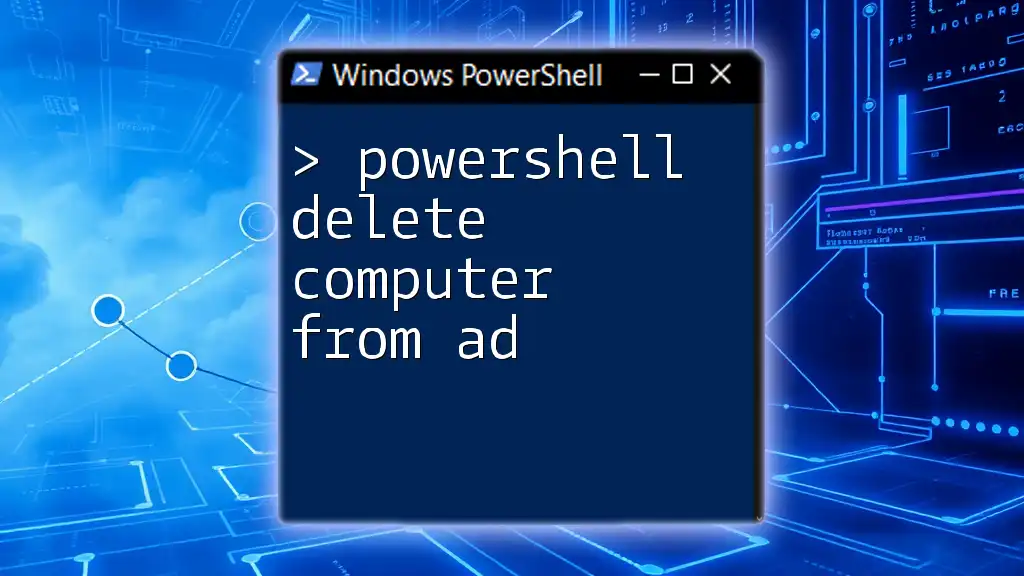
Conclusion
In this guide, you’ve learned how to connect to a remote computer and run commands using PowerShell remoting. From establishing sessions to troubleshooting common issues, these skills are invaluable in a modern IT environment. As you practice and refine your abilities, you’ll discover that PowerShell remoting can drastically improve your workflow and efficiency. To deepen your expertise, consider exploring additional resources and communities dedicated to PowerShell.