To remove a computer from Active Directory using PowerShell, you can utilize the `Remove-ADComputer` cmdlet along with the computer's name.
Here’s a code snippet to achieve that:
Remove-ADComputer -Identity 'ComputerName' -Confirm:$false
Replace `'ComputerName'` with the actual name of the computer you wish to delete from Active Directory.
Understanding Active Directory
What is Active Directory?
Active Directory (AD) is a directory service developed by Microsoft for Windows domain networks. It serves as a centralized resource management system where administrators can store information about network resources — such as computers, users, groups, and more — and manage their access. AD is vital for security and efficiency in enterprise environments, allowing IT administrators to easily manage network permissions and policies.
Why Remove a Computer from Active Directory?
Several scenarios require the deletion of a computer from Active Directory. For instance, if a device has been decommissioned or if an employee leaves the organization, it's crucial to remove their associated computer account from AD. Failing to do so can lead to security risks, such as unauthorized access or resource misuse, and can clutter the directory with stale records.
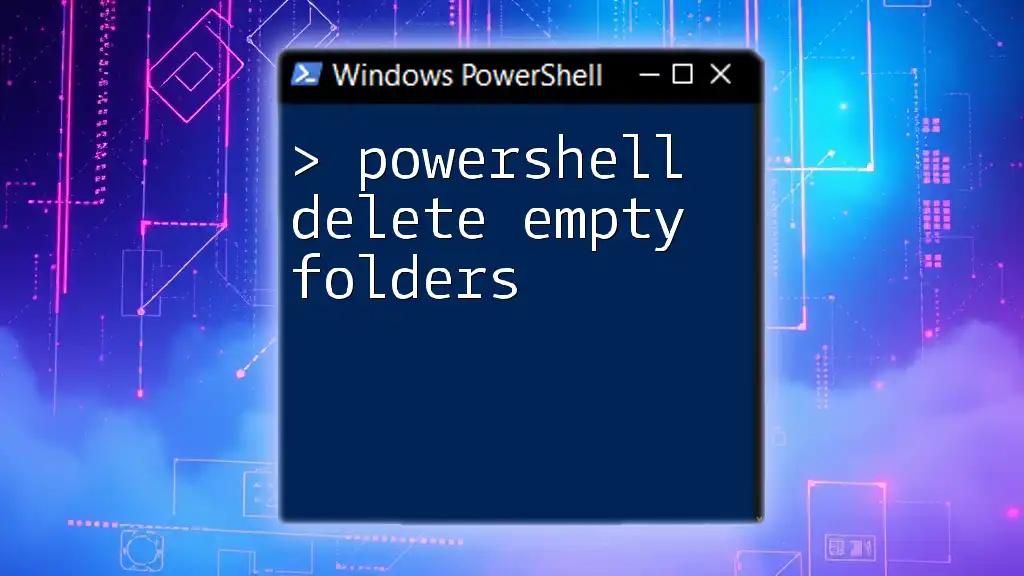
Getting Started with PowerShell
Prerequisites
Before you begin deleting a computer from Active Directory, ensure that you have:
- Basic knowledge of PowerShell: Familiarity with navigating PowerShell and executing commands.
- Proper permissions: Ensure that you have the required rights to delete objects from AD.
- Active Directory module installed: It is crucial for managing AD through PowerShell.
How to Open PowerShell
To launch PowerShell:
- Click on the Start Menu.
- Type PowerShell in the search bar.
- Right-click on Windows PowerShell and select Run as Administrator. Running with elevated privileges is necessary for executing administrative commands in Active Directory.
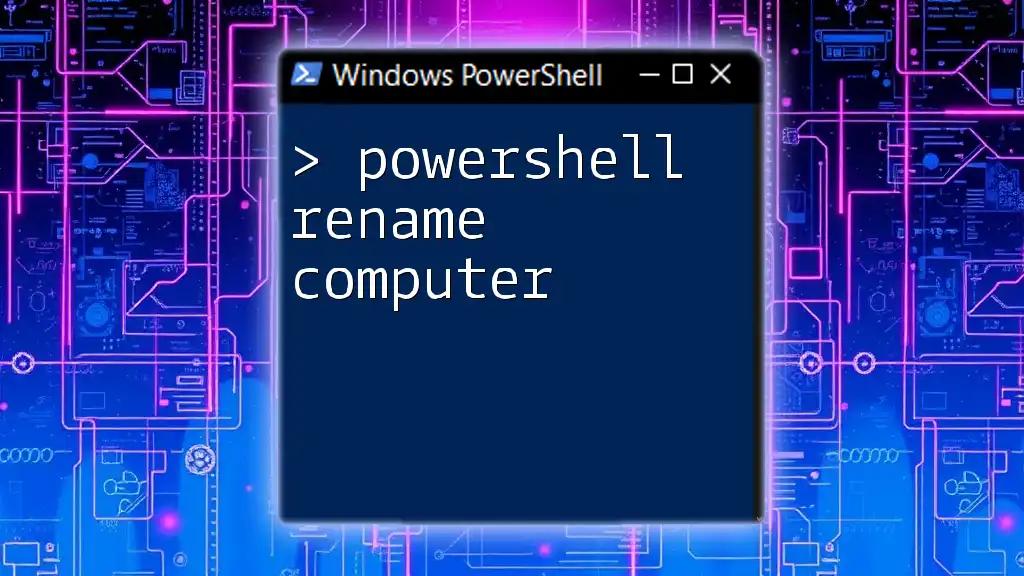
Deleting a Computer from Active Directory
Identifying the Computer to Delete
Before you delete a computer, it's essential to identify the correct account. Use PowerShell to list all computers in Active Directory:
Example Command:
Get-ADComputer -Filter * | Select-Object Name
This command retrieves all computer objects from AD and displays only their names. Ensure you note the exact name of the computer account you intend to delete.
Using the Remove-ADComputer Cmdlet
Explain the Cmdlet
The `Remove-ADComputer` cmdlet is specifically designed to delete computer objects from Active Directory. This command is straightforward but must be executed carefully to avoid accidental deletions.
Syntax of Remove-ADComputer
The basic syntax for the `Remove-ADComputer` command is:
Remove-ADComputer -Identity "<ComputerName>" [-Credential <PSCredential>] [-Confirm] [-PassThru]
In this syntax:
- -Identity specifies the computer account you wish to delete.
- Optional parameters like -Credential, -Confirm, and -PassThru can be included based on your specific requirements.
Example Command
To delete a computer account named "ComputerName", you would execute:
Remove-ADComputer -Identity "ComputerName" -Confirm:$false
In this command:
- `-Identity "ComputerName"` specifies the exact name of the computer account.
- `-Confirm:$false` bypasses the confirmation prompt, allowing for quicker execution. Use this with caution, as it will delete the object without asking for further confirmation.
Confirmation of Deletion
Once the command is executed, it's prudent to confirm that the computer has been successfully deleted. You can do this by running:
Get-ADComputer -Identity "ComputerName"
If the computer account has been deleted, PowerShell will return an error stating that the specified object cannot be found.
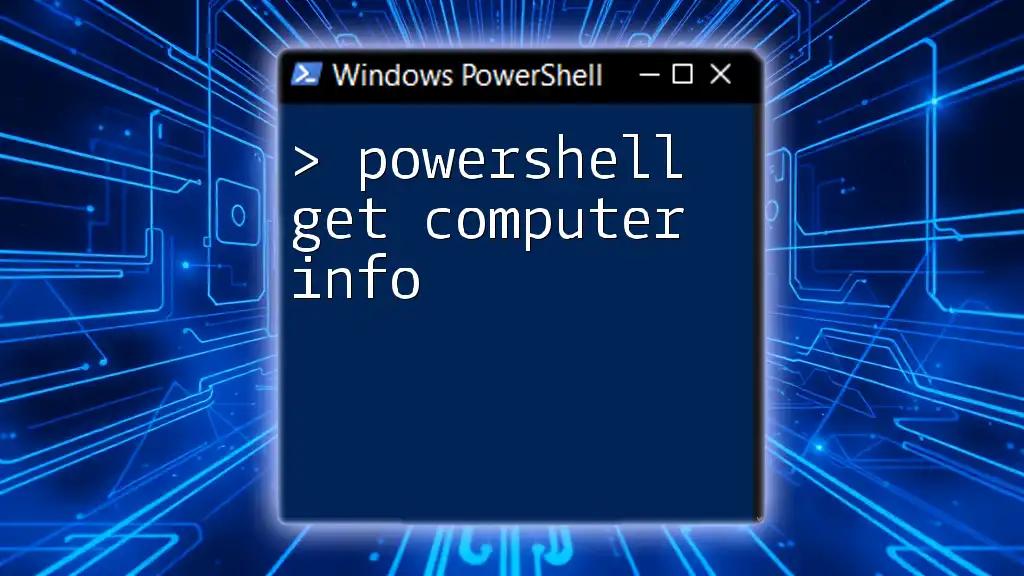
Common Errors and Troubleshooting
Potential Error Messages
When attempting to delete a computer from Active Directory, you may encounter several common errors:
- Cannot find an object with identity: This usually indicates either a typo in the computer name or that the computer already exists.
Solutions to Common Errors
- Verify the computer name: Check for spelling errors or ensure the computer is indeed listed in AD by using the `Get-ADComputer` cmdlet.
- Check permissions: Ensure your user account has the necessary rights to delete objects from AD.
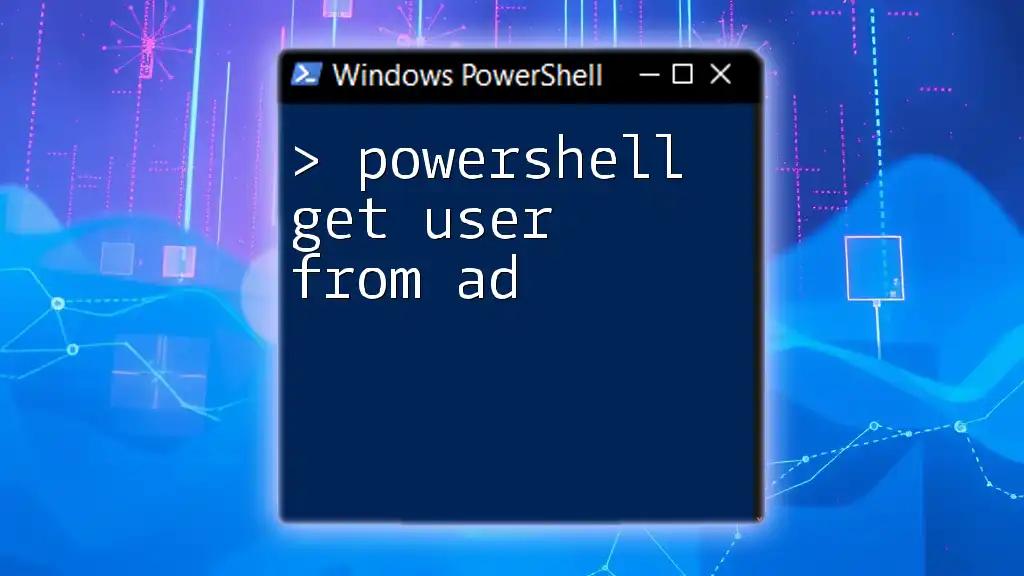
Best Practices for Managing Computer Accounts in Active Directory
Regular Audits
Regular audits of your Active Directory setup, especially the computer accounts, can prevent issues from arising. Consider scheduling routine checks every few months to identify computers that are no longer in use. You can use the following PowerShell script to list inactive computers:
Get-ADComputer -Filter 'LastLogonDate -lt (Get-Date).AddMonths(-6)' | Select-Object Name, LastLogonDate
This command retrieves computers that haven't logged on in the last six months, making it easier to spot potential candidates for removal.
Maintaining a Clean Directory
Keeping Active Directory organized is crucial for efficiency and security. Develop a policy for regularly updating and deleting stale accounts. Consider creating a PowerShell script that automatically flags or deletes computers based on their last logon date. This can enhance your IT environment by ensuring only relevant and active accounts are maintained.
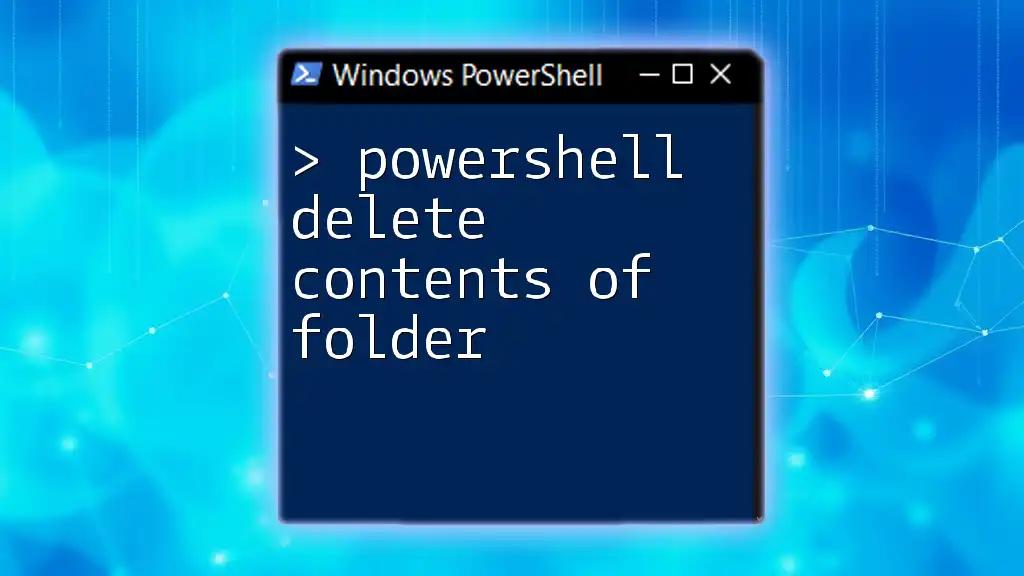
Conclusion
Knowing how to use PowerShell to delete computer accounts from Active Directory is an essential skill for IT professionals. It not only ensures that your AD remains clean but also mitigates risks associated with stale accounts. Practice the commands provided in this guide, and familiarize yourself with managing AD through PowerShell.
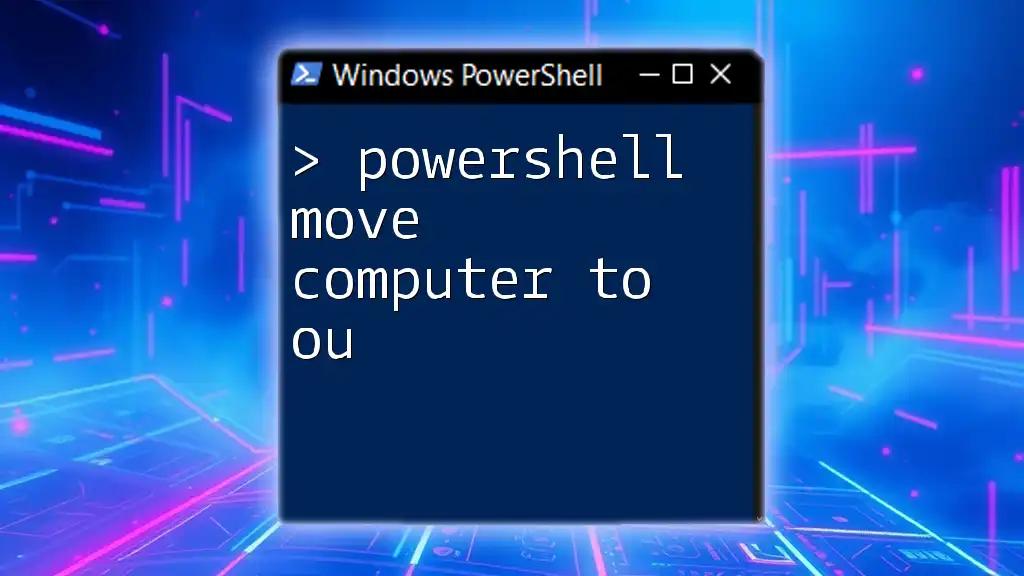
Additional Resources
For further learning, check out the official [Microsoft PowerShell Documentation](https://docs.microsoft.com/en-us/powershell/) and explore community resources where you can ask questions and share insights on managing Active Directory effectively.