To remove a specific character from a string in PowerShell, you can use the `-replace` operator, as demonstrated in the following code snippet:
$string = "Hello, World!"; $modifiedString = $string -replace ",", ""; Write-Host $modifiedString
This example removes the comma from the string "Hello, World!" resulting in "Hello World!".
Understanding Strings in PowerShell
What is a String?
In PowerShell, a string is a sequence of characters enclosed in quotes. Strings can contain letters, numbers, and symbols. They play a crucial role in scripting, as they're often used for data input and output.
For example, you can create a simple string like this:
$greeting = "Hello, PowerShell!"
Common Use Cases for String Manipulation
String manipulation is essential in various scenarios, including:
- Data Cleaning: Preparing strings by removing unwanted characters or formatting inconsistencies.
- Formatting Outputs: Ensuring that strings appear correctly in logs or user interfaces.
- Comparing Values: Preparing strings for comparisons to improve logic accuracy in scripts.
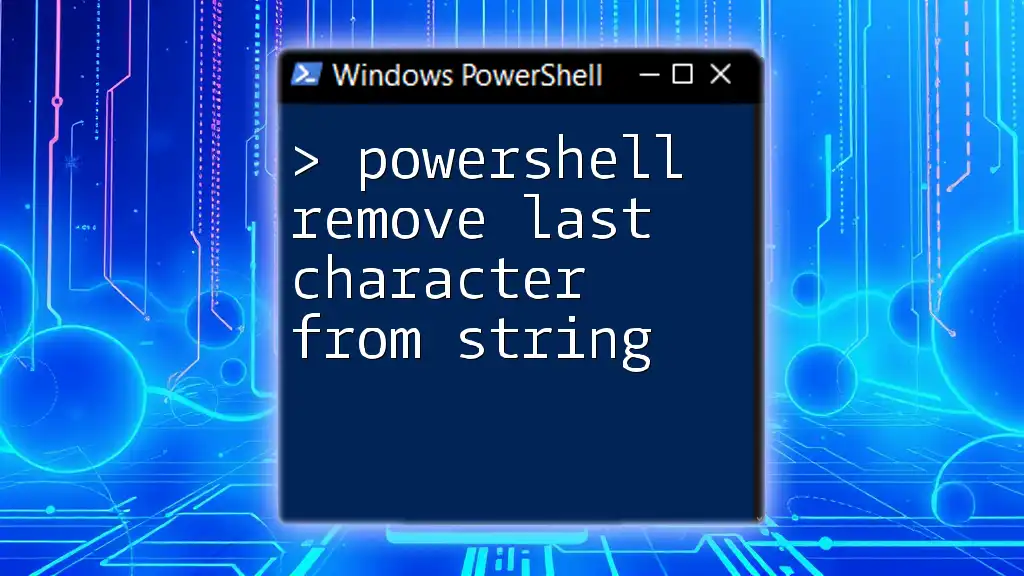
Removing Characters from Strings
Why Remove Characters?
Removing characters from strings can help to clean up data inputs, eliminate noise in information, or create formatted outputs. Understanding how to effectively manipulate strings enhances your overall scripting efficiency.
Basic Methods for Character Removal
Using the `Replace` Method
The `Replace` method is a straightforward way to achieve character removal. It replaces occurrences of a specified character with another character (or removes it entirely by replacing it with an empty string).
Syntax:
string.Replace(oldValue, newValue)
Example:
$originalString = "Hello World!"
$modifiedString = $originalString.Replace("o", "")
Write-Output $modifiedString # Output: Hell Wrld!
In this example, the character "o" is removed, showcasing how simple the `Replace` method is to implement.
Using String Indexing and Substring
Another method involves using string indexing and the `Substring` method. This approach is helpful when you know the exact positions of the characters you want to keep.
Example:
$originalString = "PowerShell"
$newString = $originalString.Substring(0, 5) + $originalString.Substring(6)
Write-Output $newString # Output: PowerShe
In this case, the character at index 5 (the letter "S") is removed by concatenating two substrings.
Regular Expressions for Complex Character Removal
Introduction to Regular Expressions in PowerShell
Regular expressions (regex) are patterns used to match character combinations in strings. They offer great flexibility for complex string manipulations and can be more efficient than basic methods when dealing with various character sets.
Removing Specific Characters with Regex
In PowerShell, regular expressions can be utilized with the `-replace` operator for effective character removal.
Example:
$text = "P@ssw0rd!"
$cleanText = $text -replace "[^a-zA-Z0-9]", ""
Write-Output $cleanText # Output: Pssw0rd
In this snippet, the regex pattern `[^a-zA-Z0-9]` matches any character that is not an uppercase letter, a lowercase letter, or a digit, effectively cleaning the string of unwanted symbols.
Removing Multiple Different Characters
You can also adapt regex to remove multiple specific characters in one go.
Example:
$input = "2023-10-03"
$result = $input -replace "-", ""
Write-Output $result # Output: 20231003
This approach is particularly useful for dealing with formatted data such as dates, where you may need to eliminate separators.
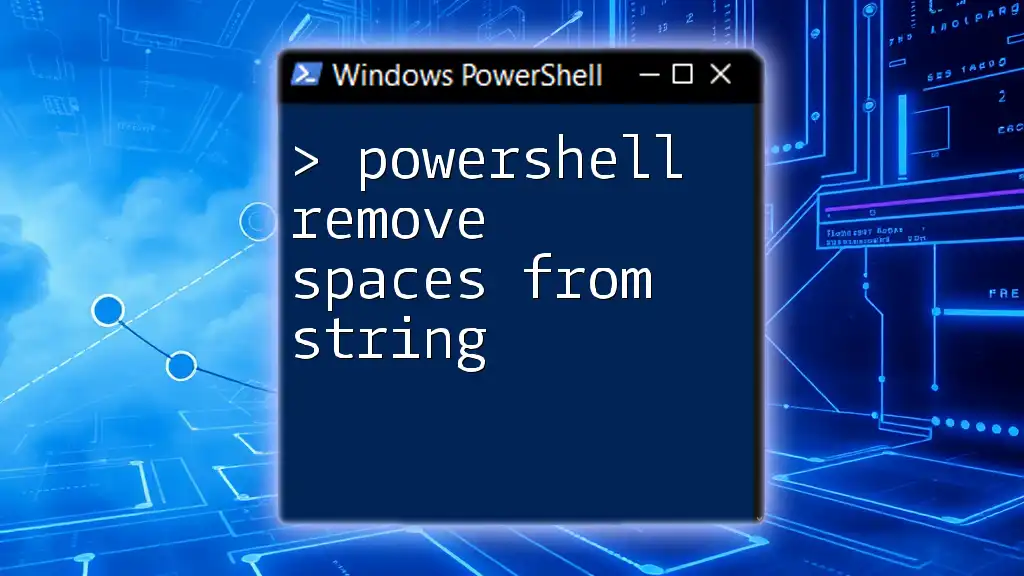
Practical Examples
Use Case: Cleaning User Input
A common task in scripting is to clean user input to prevent errors.
Example:
$username = "User#123*"
$cleanUsername = $username -replace "[^\w]", ""
Write-Output $cleanUsername # Output: User123
In this case, the regex strip unwanted characters, allowing for a valid username that only contains word characters.
Use Case: Formatting Dates
In contexts where dates are dealt with, removing characters can help streamline processes.
Example:
$dateInput = "01-02-2022"
$formattedDate = $dateInput -replace "-", ""
Write-Output $formattedDate # Output: 01022022
Here, hyphens are removed from the date, which can be useful for data entry or formatting tasks.
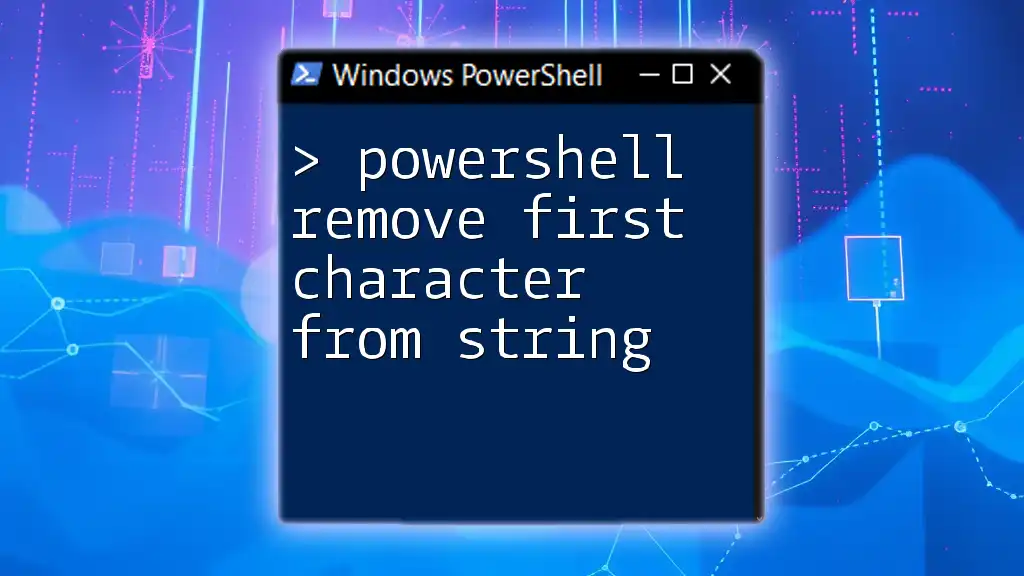
Performance Considerations
Efficiency of Different Methods
While all the methods for removing characters have their own merits, it's crucial to consider performance. In larger scripts with significant string manipulation, regex might introduce some overhead compared to simple string methods. It's wise to evaluate the context of script execution and choose methods accordingly.
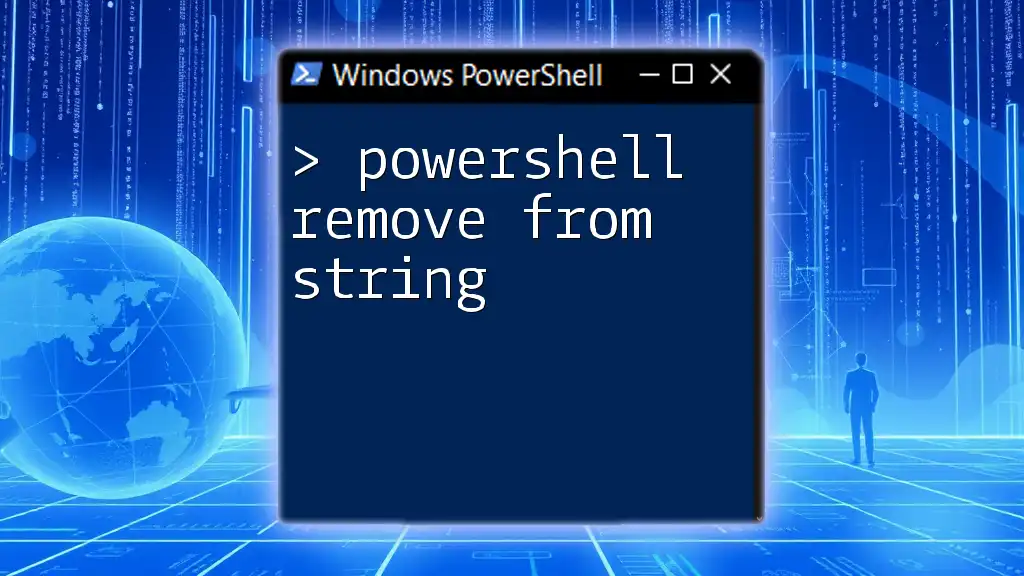
Best Practices for String Manipulation in PowerShell
Consistency in String Handling
For robust scripting, maintaining consistency in how strings are formatted and manipulated is essential. Standardizing formats and practices minimizes errors and improves maintainability.
Code Readability and Maintenance
Effective commenting and organization of your string manipulation script make it easier for others (and yourself) to understand the logic behind actions. Always ensure your methods are clear, and help structures support readability.
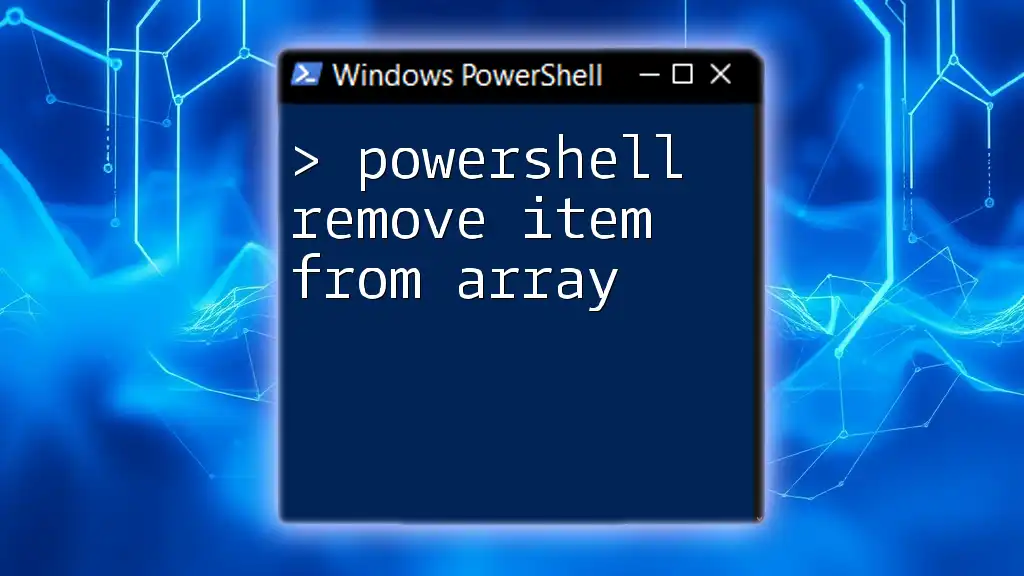
Conclusion
Mastering how to effectively remove characters from strings in PowerShell can significantly enhance your scripting capabilities and data management skills. By familiarizing yourself with methods such as `Replace`, `Substring`, and regular expressions, you'll be equipped to handle a variety of scenarios with ease.