To remove spaces from a string in PowerShell, you can use the `-replace` operator with a regular expression to match and replace all space characters with an empty string. Here’s a code snippet to demonstrate this:
$string = "Hello, World!"
$cleanedString = $string -replace '\s+', ''
Write-Host $cleanedString
This will output: `Hello,World!`
Understanding Whitespace in PowerShell
What is Whitespace?
Whitespace refers to any character that is invisible in text formatting, primarily including spaces, tabs, and newlines. In programming and data handling, understanding whitespace is crucial, as its presence can lead to unexpected behaviors or errors. For instance, an extra space in a string can cause comparisons to fail or lead to improperly formatted output.
Common Scenarios for Removing Spaces
Removing spaces from strings is a common practice in various scenarios, such as:
- Data cleaning: When processing data from CSV files, ensuring there are no extraneous spaces can simplify analysis.
- Formatting user input: Input from forms or user-generated content often contains leading or trailing spaces, which should be trimmed for uniformity.
- Preparing strings for database storage: Ensuring that data is free from unnecessary whitespace can prevent issues in search functionality and storage optimization.
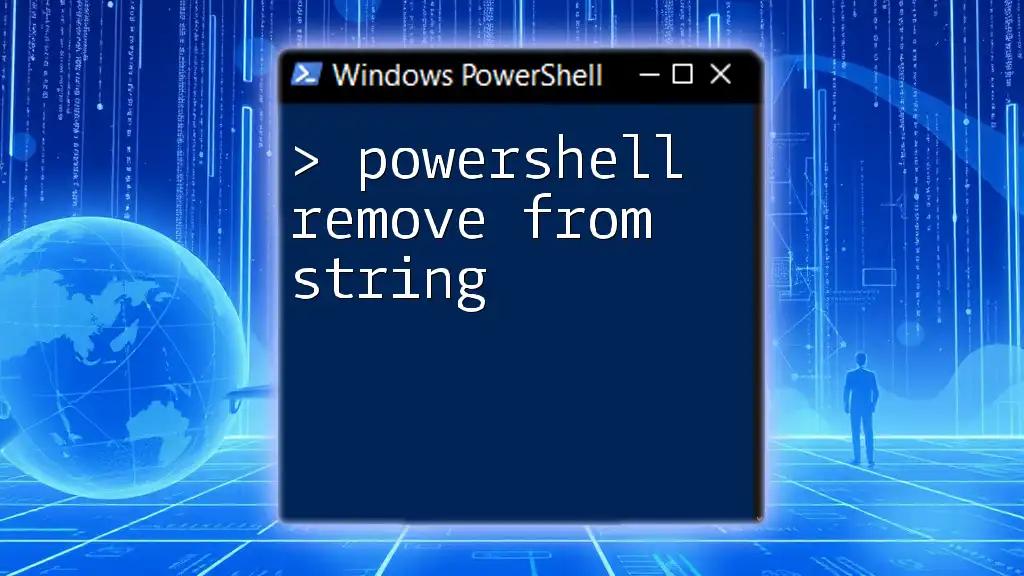
Methods to Remove Spaces in PowerShell
Using the `Trim()` Method
The `Trim()` method in PowerShell is designed to remove leading and trailing spaces from a string. This is particularly useful when you want to clean up user input or data that may have inconsistent spacing.
Code Example:
$string = " Hello World! "
$trimmedString = $string.Trim()
Write-Output $trimmedString
Output: `"Hello World!"`
In this example, notice how both leading and trailing spaces are effectively removed, leaving you with just the content of the string.
Using the `Replace()` Method
The `Replace()` method allows you to target specific substrings within a string, making it a powerful tool for removing spaces. You can use it to replace all instances of a single space (`" "`) with an empty string (`""`).
Code Example:
$string = "Hello World!"
$noSpaceString = $string.Replace(" ", "")
Write-Output $noSpaceString
Output: `"HelloWorld!"`
This approach eliminates all spaces within the string but might not be suitable if you wish to retain single spaces between words. Therefore, use this method carefully based on your requirements.
Utilizing Regular Expressions with `-replace`
PowerShell supports regular expressions, allowing for more sophisticated string manipulation. The `-replace` operator can be particularly useful for removing all types of whitespace, not just spaces.
Code Example:
$string = "Hello World!"
$cleanedString = $string -replace '\s+', ' '
Write-Output $cleanedString
Output: `"Hello World!"`
In this case, `\s+` is a regular expression that matches one or more whitespace characters, effectively converting multiple spaces into a single space. This provides cleaner output while respecting separation between words.
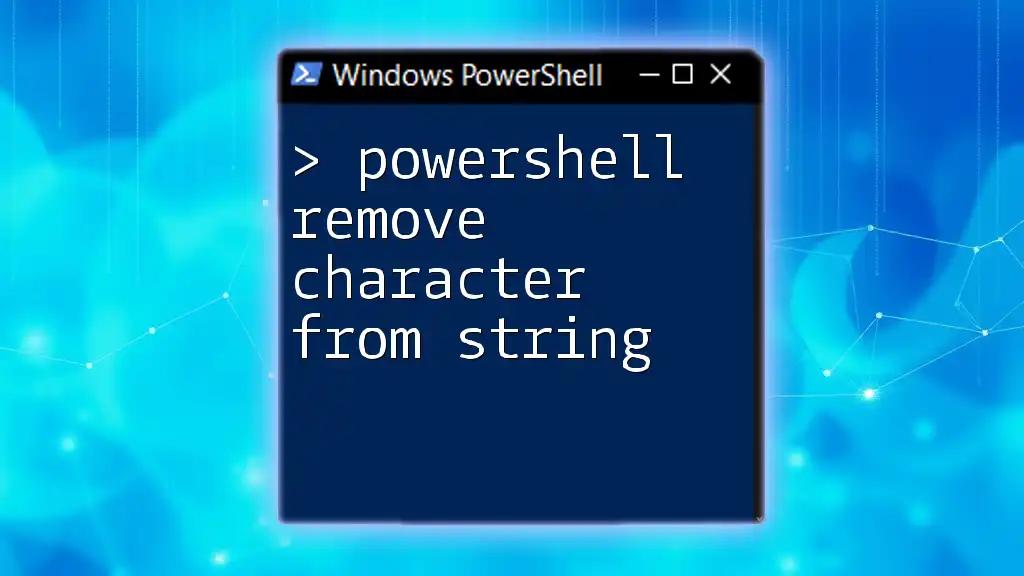
Advanced Techniques for Trimming Spaces
Combining `Trim()`, `Replace()`, and `-replace`
In some cases, you may need to use a combination of methods to ensure your string is formatted just right. For example, if your string has leading/trailing spaces and multiple intervening spaces, combining the methods can be valuable.
Code Example:
$string = " Hello World! "
$cleanedString = $string.Trim().Replace(" ", "-")
Write-Output $cleanedString
Output: `"-Hello---World!-"`
Here, leading and trailing spaces are removed first, and then all spaces within the string are replaced with hyphens. Customizing such outputs can be very useful in certain applications.
Custom Function to Clean Strings
Creating a reusable function for cleaning spaces can save time and enhance code clarity across your scripts. This encapsulates the logic, making it easy to maintain and reuse.
Code Example:
function Remove-Spaces {
param([string]$inputString)
return $inputString.Trim() -replace '\s+', ' '
}
$result = Remove-Spaces " Hello World! "
Write-Output $result
Output: `"Hello World!"`
This function takes an input string, applies both trimming and whitespace reduction, and returns the cleaned string. Utilizing functions is a best practice that promotes code reuse and organization.

Additional Considerations when Removing Whitespace
Performance Considerations
When dealing with large datasets, the performance of your string manipulation methods can become significant. While methods like `Trim()` and `Replace()` are generally efficient, in high-volume scenarios, be mindful of using `-replace` with complex patterns, as it could introduce overhead. Always measure and optimize for performance based on your specific use case.
Handling Different Types of Whitespace
It's important to recognize that whitespace isn't limited to spaces. Newlines and tabs may also appear in strings. To handle these correctly, consider using the same regular expression techniques.
Code Example:
$stringWithTabs = "Hello`tWorld"
$cleanedString = $stringWithTabs -replace '\s+', ' '
Write-Output $cleanedString
Output: `"Hello World"`
This example highlights how effective regular expressions can be in cleaning up various types of whitespace, creating a more robust string-handling strategy.

Real-World Applications of Removing Spaces
Data Preparation for Analysis
In data analytics, ensuring that your strings are clean and consistent plays a pivotal role in achieving accurate results. For example, if you are analyzing customer feedback and some strings have extra spaces, it could lead to miscounts or misalignment in your analysis.
User Input Validation
By effectively removing unnecessary spaces from user inputs, you enhance both data integrity and the user experience. A well-formatted string not only looks good but is more functional and easier to work with, feeding into successful application logic and database entries.
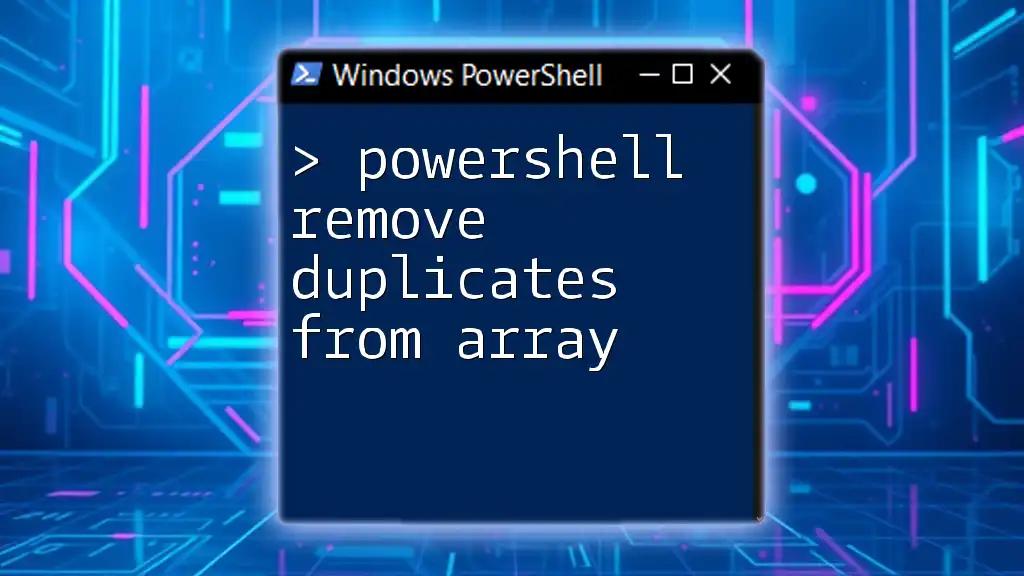
Conclusion
In this article, we have explored various techniques to remove spaces from strings in PowerShell, identifying methods such as `Trim()`, `Replace()`, and leveraging regular expressions for more advanced scenarios. Understanding these tools empowers you to handle strings more efficiently, ensuring your scripts are not only functional but also reliable.
Harness these techniques in your own PowerShell practices to improve your coding efficiency and data integrity. Keep experimenting and refining your approach as you become more familiar with the capabilities of PowerShell!