In PowerShell, you can remove duplicates from an array by using the `Select-Object` cmdlet with the `-Unique` parameter.
$array = @(1, 2, 2, 3, 4, 4, 5)
$uniqueArray = $array | Select-Object -Unique
Write-Host $uniqueArray
Understanding Arrays in PowerShell
In PowerShell, an array is a collection of items stored in a single variable. Arrays can hold various data types, including strings, integers, or even complex objects. PowerShell makes it easy to work with arrays, enabling users to manipulate them efficiently in scripts and commands. Common applications for arrays include storing user data, organizing output from commands, and facilitating batch operations on multiple items.

Importance of Removing Duplicates from Arrays
Duplicates in an array can lead to several issues. Data integrity is compromised when multiple identical values exist where unique values are expected. This can cause erroneous calculations or misleading outputs, especially during data processing and analysis.
Performance can also suffer; processing large arrays with duplicates may incur unnecessary overhead. By removing duplicates, not only do we clean up our data, but we also enhance script efficiency, reducing runtime and memory consumption.
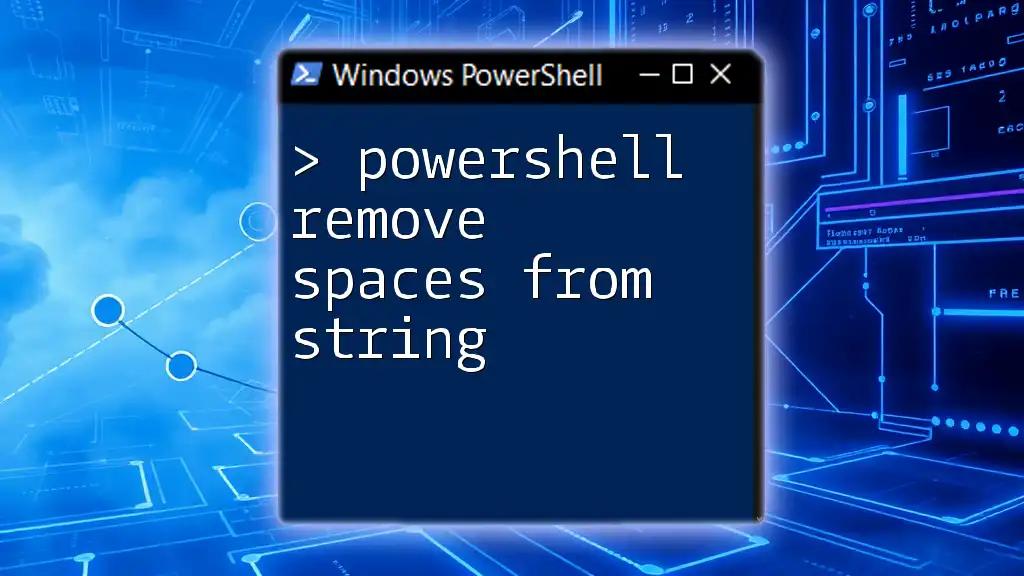
Methods to Remove Duplicates from PowerShell Arrays
Using the `Sort-Object` Cmdlet
`Sort-Object` is a versatile cmdlet in PowerShell that can both sort and filter arrays. One of its powerful features is the `-Unique` parameter, which automatically eliminates duplicate entries.
Code Example: Remove Duplicates with Sorting
$array = 1, 2, 2, 3, 4, 4, 5
$uniqueArray = $array | Sort-Object -Unique
In this example, the original array contains the numbers 1, 2, 2, 3, 4, 4, and 5. When we pipe it to `Sort-Object` with the `-Unique` option, the result stored in `$uniqueArray` will be 1, 2, 3, 4, and 5. This method is simple and efficient, making it an excellent choice for many situations.
Utilizing the `Get-Unique` Cmdlet
Though less commonly used than `Sort-Object`, `Get-Unique` provides another method for deduplication. It's worth noting that `Get-Unique` requires the array to be sorted beforehand to function correctly.
Code Example: Utilizing `Get-Unique`
$array = 1, 2, 3, 3, 3, 4, 5
$uniqueArray = $array | Sort-Object | Get-Unique
In this scenario, `Sort-Object` ensures the array is organized before `Get-Unique` processes it to remove duplicates. While this method is straightforward, itβs essential to remember that using `Get-Unique` requires prior sorting, which may not always be optimal in larger operation contexts.
Manual Method Using a Loop
There are times when more control over the deduplication process is required. In such cases, using a loop to manually check for duplicates can be beneficial.
Code Example: Removing Duplicates with a Loop
$array = 1, 2, 2, 3, 3, 4, 5
$uniqueArray = @()
foreach ($item in $array) {
if (-not $uniqueArray.Contains($item)) {
$uniqueArray += $item
}
}
Here, we initialize an empty array, `$uniqueArray`, and iterate through the original array. For each item, we check whether it already exists in the `$uniqueArray`. If not, we add it. This method grants flexibility, allowing for additional conditions or modifications as required. However, it may be less efficient with larger datasets due to its O(n^2) complexity.
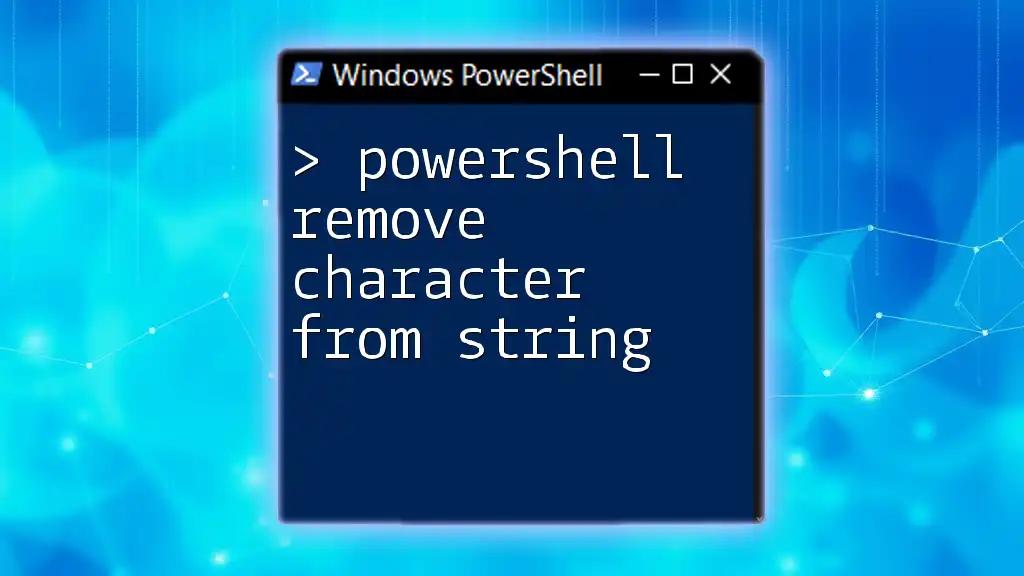
Performance Comparison of Methods
When removing duplicates from PowerShell arrays, the choice of method impacts performance. The `Sort-Object` cmdlet, while efficient for moderate-sized arrays, might not be the best choice for very large datasets due to its sorting step.
In contrast, the manual loop provides maximum flexibility β ideal for complex cases β but is typically slower. Understanding the performance implications helps select the right approach for specific scenarios and ensures scripts run efficiently.
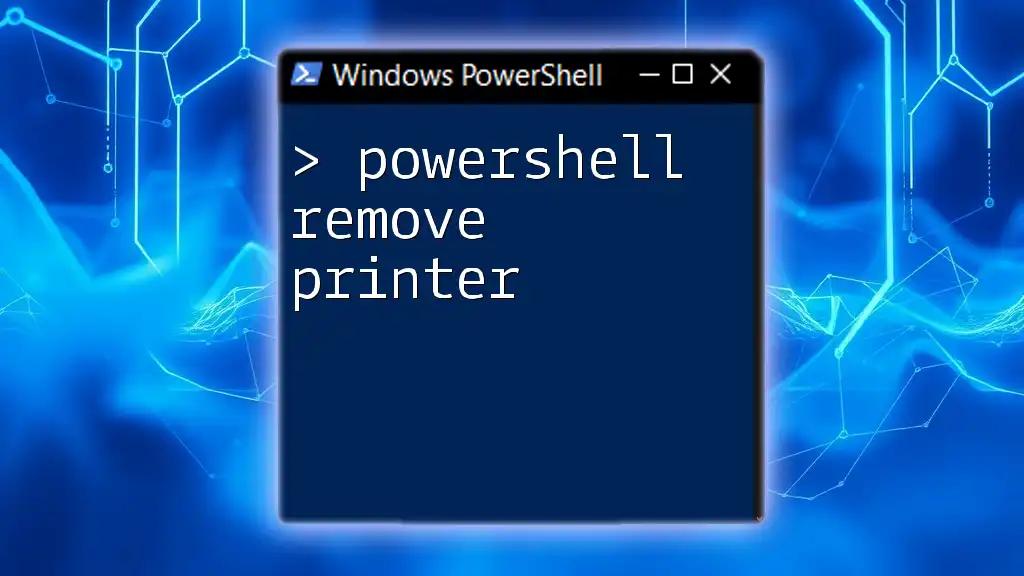
Handling Complex Data Types
When dealing with arrays of objects, removing duplicates can be more intricate. For instance, you might want to eliminate duplicates based on a specific property of the objects.
Code Example: Using a Property to Remove Duplicates
$objectsArray = @(
[PSCustomObject]@{ Id = 1; Name = "Alice" },
[PSCustomObject]@{ Id = 1; Name = "Alice" },
[PSCustomObject]@{ Id = 2; Name = "Bob" }
)
$uniqueObjects = $objectsArray | Select-Object -Unique Id
In this example, we create an array of custom objects representing users. By piping the array to `Select-Object` with the `-Unique` flag, we can extract unique entries based on the `Id` property. This approach is invaluable when filtering data such as user records, where each entry must be distinct.

Practical Examples and Use Cases
Removing duplicates is not merely a theoretical exercise; it has real-world applications. Data cleaning in system administration scripts is often critical, particularly when aggregating logs or user input. For instance, when compiling reports from multiple data sources, eliminating duplicate entries ensures the accuracy of the analysis.
Consider a PowerShell script that gathers user activities; you wouldn't want repeated entries skewing your metrics. The foundational knowledge of how to remove these duplicates thus becomes essential for delivering clear and accurate information.

Conclusion
In conclusion, the ability to remove duplicates from arrays in PowerShell is a fundamental skill that enhances data integrity and performance. Through methods like `Sort-Object`, `Get-Unique`, and manual loops, you can choose the best approach that suits your specific needs. Practice with these techniques will empower you to handle arrays effectively and efficiently, ensuring your scripts yield the best results possible.
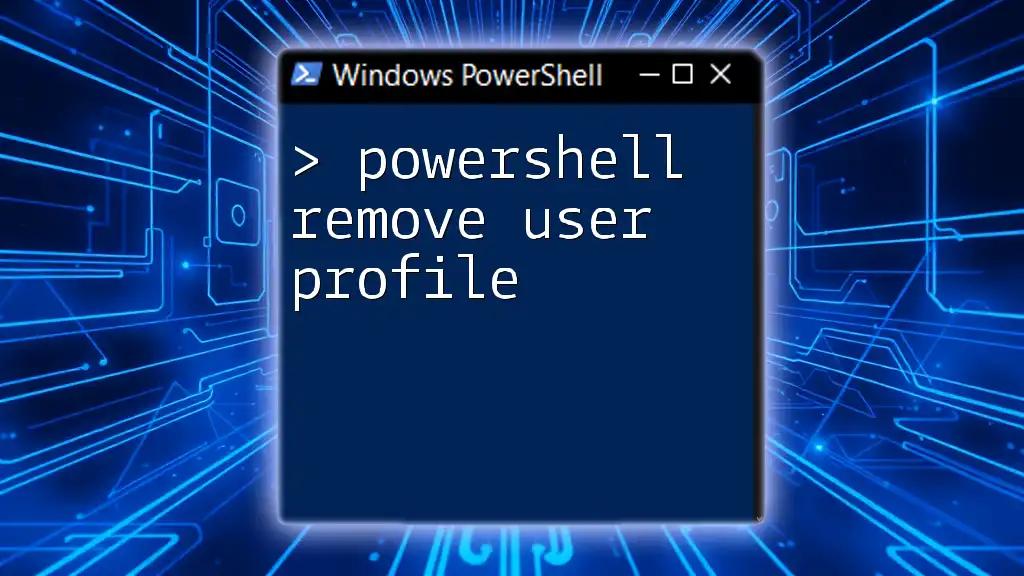
Additional Resources
To further your PowerShell knowledge, consider exploring the official Microsoft documentation and engaging with community forums. These resources are invaluable for discovering new tips, tricks, and best practices as you master PowerShell scripting.