In PowerShell, you can remove an item from an array by using the `Where-Object` cmdlet to filter out the unwanted element.
Here's a code snippet demonstrating how to remove the number 3 from an array:
$array = 1, 2, 3, 4, 5
$array = $array | Where-Object { $_ -ne 3 }
Understanding the Need to Remove Items from Arrays
Removing items from arrays in PowerShell is often necessary for various reasons, such as data cleaning, real-time updates, or managing user preferences. By effectively removing unwanted items, developers can maintain a clean dataset, which can lead to more efficient script performance. For instance, you may have a list of elements, and as your project evolves, some elements may no longer be applicable. In such scenarios, understanding how to remove them can enhance data integrity and usability.
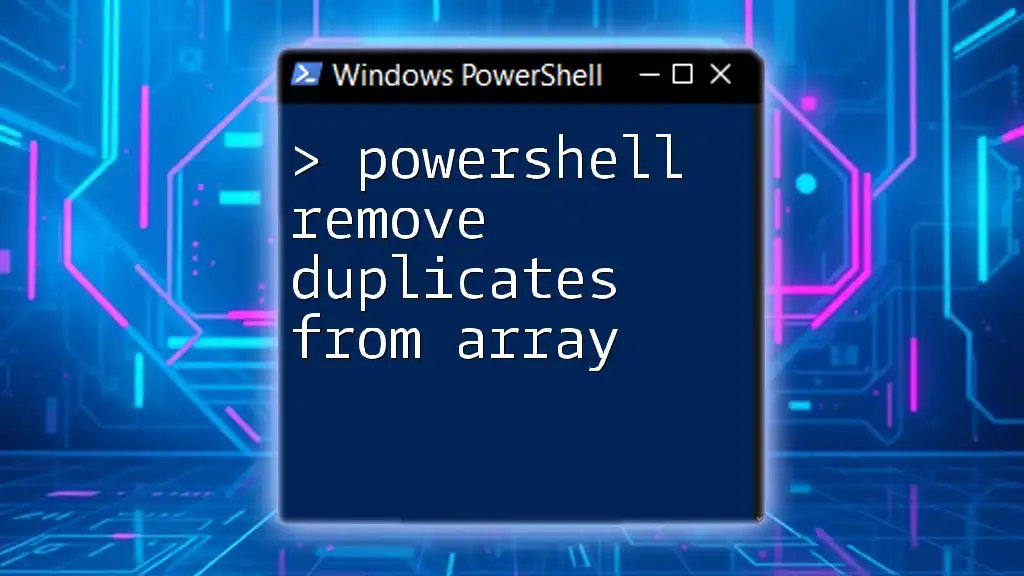
How to Remove an Item from an Array in PowerShell
Using the `Where-Object` Cmdlet
One of the most common methods for removing an item from an array in PowerShell is by utilizing the `Where-Object` cmdlet. The general syntax allows you to filter an array based on specified criteria.
Here’s a basic example:
$fruits = @('apple', 'banana', 'cherry', 'date')
$fruits = $fruits | Where-Object {$_ -ne 'banana'}
In this example, the array `$fruits` originally contains four items: 'apple', 'banana', 'cherry', and 'date'. The `Where-Object` cmdlet filters out 'banana', leaving the updated array as `('apple', 'cherry', 'date')`.
This method is straightforward and highly effective when you need to remove specific items based on conditions.
Using the `ArrayList` Class
PowerShell also allows you to use an `ArrayList`, which is a more dynamic array structure that provides methods for item management, including removing items. This method can often be more efficient when dealing with larger datasets.
To utilize an `ArrayList`, you can do the following:
$arrayList = New-Object System.Collections.ArrayList
$arrayList.AddRange(@('apple', 'banana', 'cherry', 'date'))
$arrayList.Remove('banana')
In this case, we create an `ArrayList` and populate it with fruit names. The `Remove` method efficiently removes 'banana', updating the list to `('apple', 'cherry', 'date')`. This approach is advantageous when you frequently need to add or remove items dynamically.
Using Index and Slicing
You can also remove items from an array using indexing and slicing. This method involves creating a new array that excludes the specified item by its index.
Here’s how it works:
$fruits = @('apple', 'banana', 'cherry', 'date')
$fruits = $fruits[0..1] + $fruits[3..3]
In this example, we specify the indices of the items we want to keep. The result will still be `('apple', 'cherry', 'date')`, effectively removing 'banana'. This method offers clarity and is useful when removing items at known positions, but it’s less flexible than using the `Where-Object`.
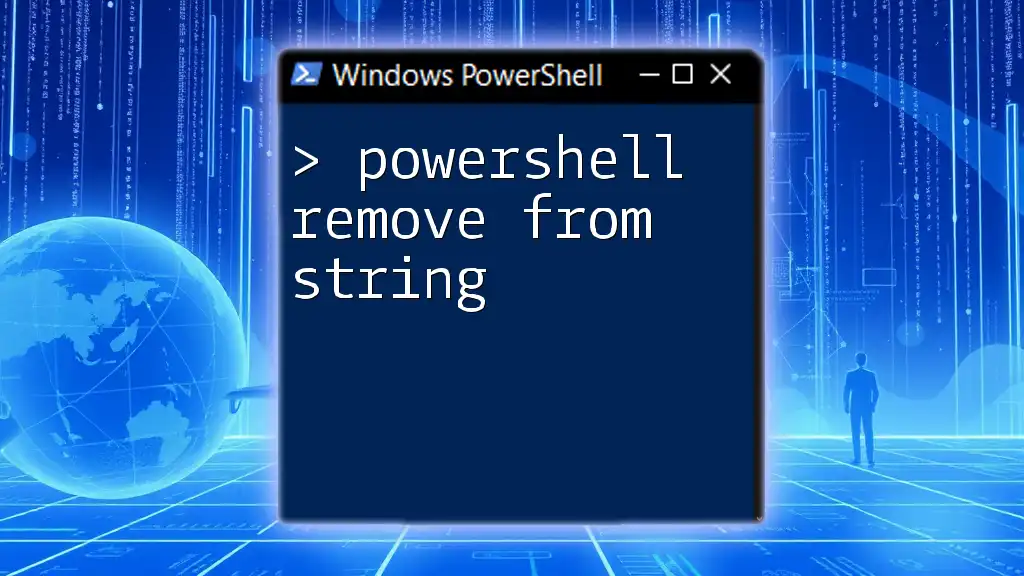
Removing Multiple Items from an Array
Using `Where-Object` with Multiple Conditions
When you need to remove multiple items from an array, the `Where-Object` cmdlet can handle this elegantly. You can filter out items using the `-notin` operator which allows you to specify more than one item.
Look at the following example:
$fruits = @('apple', 'banana', 'cherry', 'date', 'fig')
$fruits = $fruits | Where-Object {$_ -notin @('banana', 'fig')}
By running this code, both 'banana' and 'fig' are removed from the original array, resulting in `('apple', 'cherry', 'date')`. This method is both intuitive and powerful when managing larger or more complex datasets.
Using a Loop for Conditional Removal
For scenarios where conditions may vary or require additional logic, using a loop to evaluate items is a viable option. This method allows for removing items based on custom criteria as displayed below:
$fruits = @('apple', 'banana', 'cherry', 'date')
foreach ($fruit in $fruits) {
if ($fruit -eq 'banana' -or $fruit -eq 'cherry') {
$fruits = $fruits -ne $fruit
}
}
Here, the loop checks each fruit and removes 'banana' and 'cherry' from the `$fruits` array. However, note that this isn't the most efficient way for larger arrays. While it illustrates the flexibility of PowerShell, using filter methods like `Where-Object` can enhance performance.
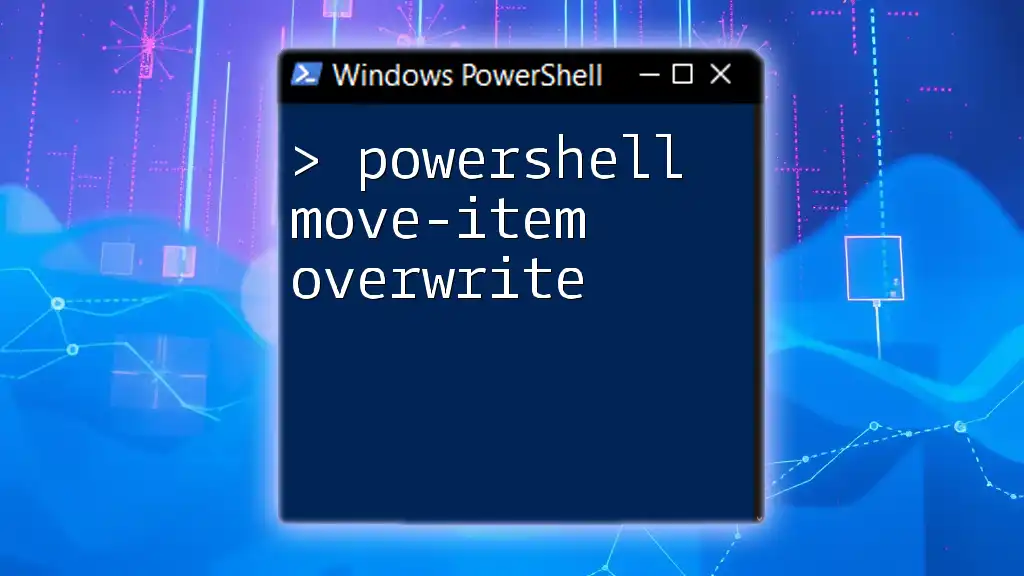
Best Practices for Removing Items from PowerShell Arrays
Maintaining Code Readability
As you manipulate arrays with the `powershell remove item from array` command, keeping the code clear and straightforward is critical. By consistently utilizing meaningful variable names and incorporating comments, you enhance readability. Well-structured code aids in debugging and makes it easier for others to understand your logic.
Performance Considerations
In terms of performance, using the `Where-Object` cmdlet or `ArrayList` class is generally faster for larger datasets when you frequently remove items. Understanding these nuances will help you choose the most efficient method for your specific use-case scenarios.
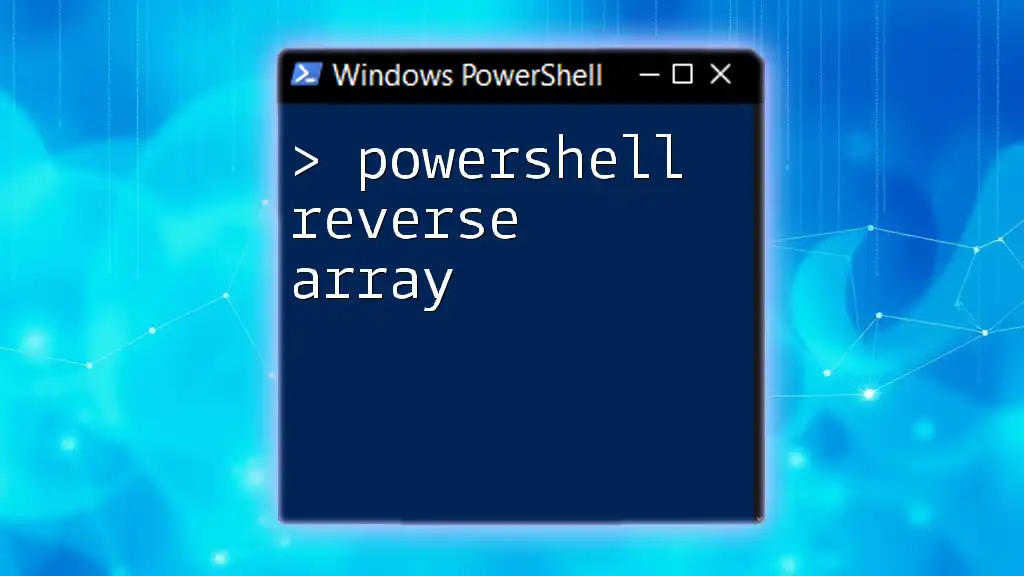
Troubleshooting Common Issues
Users often encounter common errors while trying to remove items from arrays, such as:
- Attempting to remove an item that does not exist, which can lead to confusion.
- Variable scope issues when working with loops.
To debug effectively, break down your array manipulations into smaller steps. Check the contents of your arrays after each operation to ensure that your logic aligns with your expected outcomes.
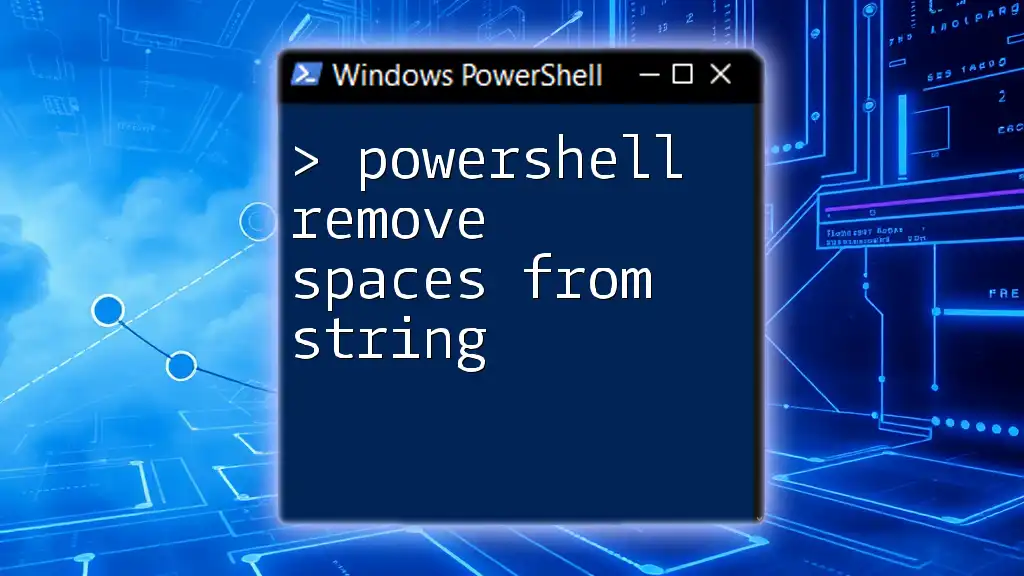
Conclusion
Knowing how to efficiently remove items from an array is an essential PowerShell skill that enhances your ability to manage collections of data effectively. Whether using `Where-Object`, the `ArrayList` class, or straightforward indexing and slicing, each method serves unique scenarios. By mastering these techniques, you will greatly improve your scripting efficiency and code quality.