The `Move-Item` command in PowerShell allows you to move files or directories and can overwrite an existing item at the destination if specified by the `-Force` parameter.
Here’s a code snippet to demonstrate this:
Move-Item -Path 'C:\Source\file.txt' -Destination 'C:\Destination\file.txt' -Force
What is Move-Item?
PowerShell's `Move-Item` cmdlet serves the vital purpose of moving files and folders from one location to another within the file system. Its versatility makes it particularly useful in various scenarios, such as organizing files, renaming them, or even transporting them across different drives. Understanding how to use this cmdlet effectively can enhance your productivity and streamline your file management processes.
Unlike other cmdlets such as `Copy-Item`, which duplicates files, `Move-Item` completely transfers the original item, thereby reducing redundancy and helping manage disk space efficiently. Common use cases include moving configuration files to a new directory, archiving old documents, or relocating application data.
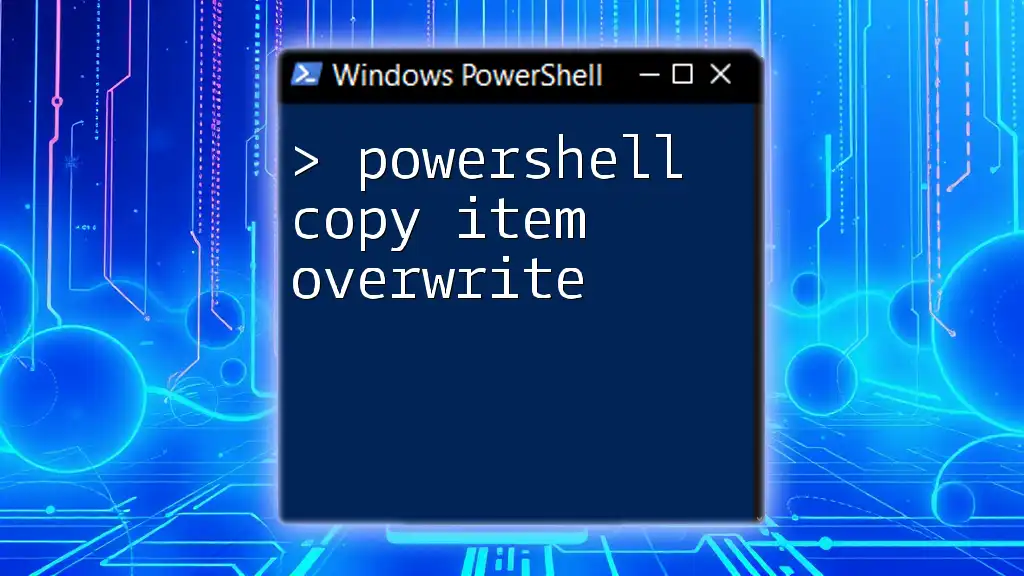
Understanding Overwriting Behavior
In PowerShell, overwriting files is a critical behavior, especially when dealing with similar names at the destination. By default, if a file with the same name exists in the target directory, `Move-Item` will not overwrite it without specific parameters being set. Instead, it throws an error, informing you that the destination file already exists.
Here’s an example of the default behavior of `Move-Item`:
Move-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt"
Trying to execute the above command will generate this error: `Cannot overwrite the item ... as it already exists.` Before using `Move-Item`, it's crucial to comprehend these nuances to avoid potential data loss and ensure that your file management operations go smoothly.
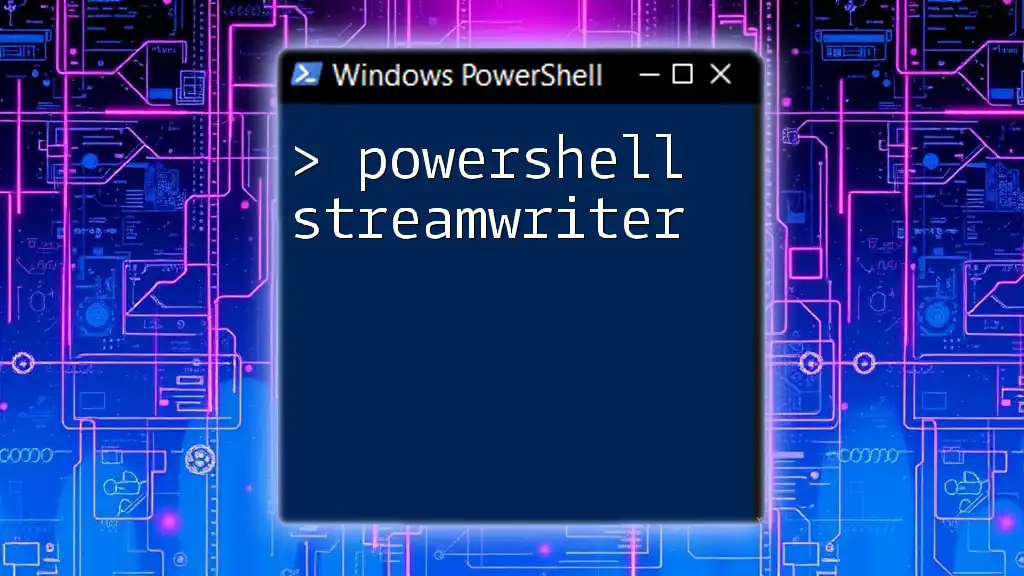
How to Use Move-Item with Overwrite
Using the -Force Parameter
To allow overwriting of existing files, you will need to use the `-Force` parameter with `Move-Item`. This parameter tells PowerShell to overwrite the target file if it exists, thus facilitating straightforward file management without interruptions.
The basic syntax looks like this:
Move-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt" -Force
Breakdown of the syntax:
- `-Path`: Specifies the source file to be moved.
- `-Destination`: Indicates the destination location.
- `-Force`: Allows the command to overwrite the file at the destination.
It's important to ensure that when using the `-Force` parameter, you are aware that any existing file in the destination will be permanently replaced.
Handling Different File Types
When using `Move-Item` with the `-Force` parameter, the operation behaves similarly whether you're moving files or entire folders. Here’s how to handle both cases:
Moving Files:
Move-Item -Path "C:\Source\TextFile.txt" -Destination "C:\Destination\TextFile.txt" -Force
In this command, if `TextFile.txt` already exists in the `C:\Destination\` directory, it will be overwritten.
Moving Folders:
Move-Item -Path "C:\Source\Folder" -Destination "C:\Destination\Folder" -Force
In this situation, if `Folder` already exists in the destination, the entire folder and its contents will be replaced, so caution is advised.
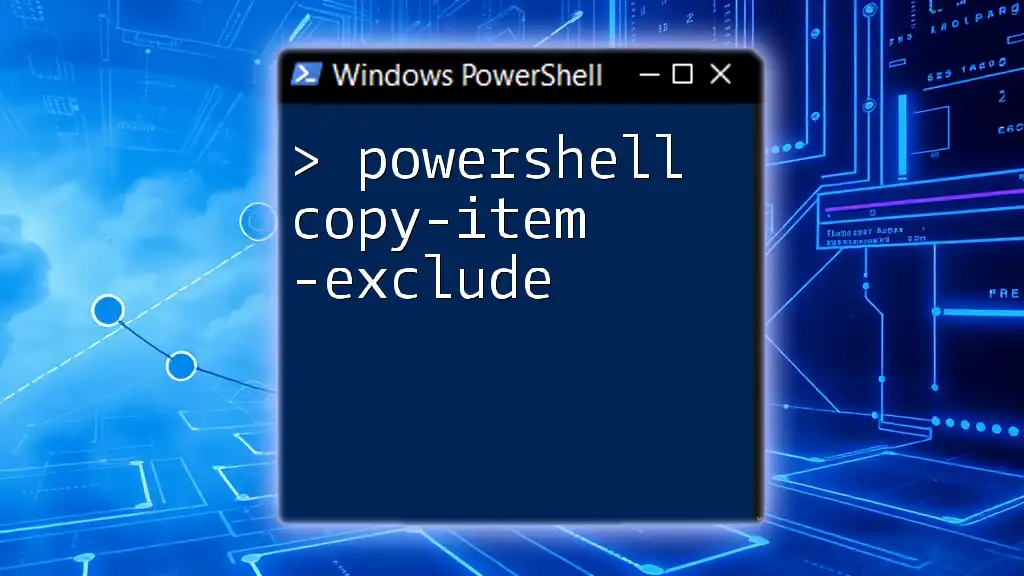
Safety Precautions and Best Practices
Verifying Existing Files Before Moving
Before executing a moving operation, it's wise to check whether the target file or folder already exists. Employing the `Test-Path` cmdlet allows you to verify this.
Example:
if (Test-Path "C:\Destination\File.txt") {
Write-Host "File exists, proceeding with overwrite."
} else {
Write-Host "File does not exist, moving file."
}
This snippet ensures that you're making an informed decision before overwriting important files.
Creating Backups Before Moving
Another essential practice is to create backups before performing any move operations, especially if you may need to restore the files later.
For instance, you can create a backup like so:
Copy-Item -Path "C:\Destination\File.txt" -Destination "C:\Backup\File_Backup.txt"
In this example, the original file is backed up, ensuring that even if something goes wrong in the moving process, you can recover your data easily.
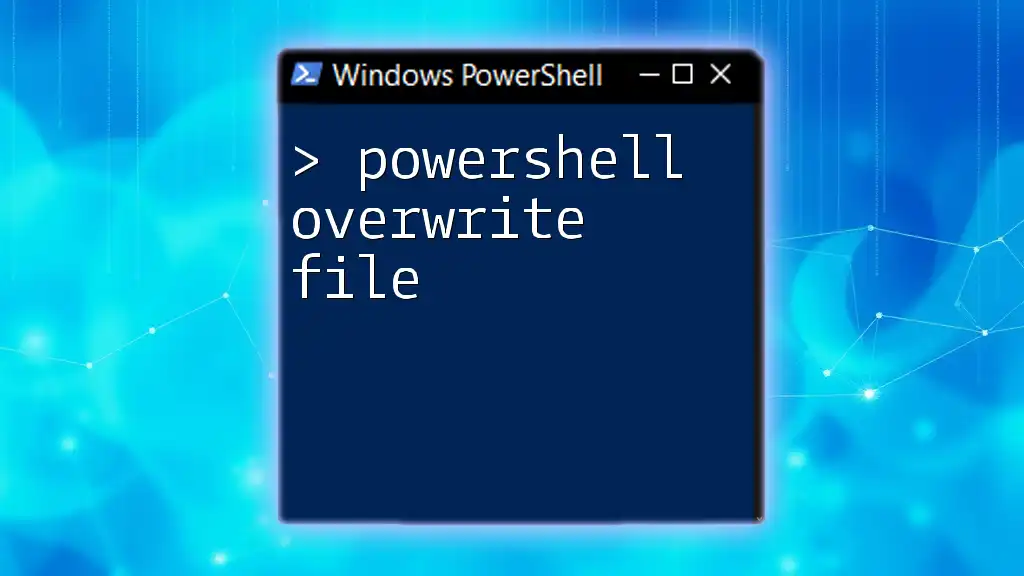
Additional Considerations
Using Wildcards with Move-Item
The flexibility of `Move-Item` extends to using wildcards, which is particularly useful when you wish to move multiple files that share specific characteristics.
For example, to move all `.txt` files from one directory to another, use:
Move-Item -Path "C:\Source\*.txt" -Destination "C:\Destination\" -Force
This command will overwrite any existing `.txt` files in the destination without raising errors.
Error Handling in PowerShell
While using `Move-Item`, errors may arise, especially when conflicts occur. Implementing error handling using `Try-Catch` blocks is a solid practice to catch and respond to potential issues.
Example:
try {
Move-Item -Path "C:\Source\File.txt" -Destination "C:\Destination\File.txt" -Force
} catch {
Write-Host "An error occurred: $_"
}
This ensures that if an error occurs during the move, you will receive a descriptive message instead of encountering unhandled exceptions.
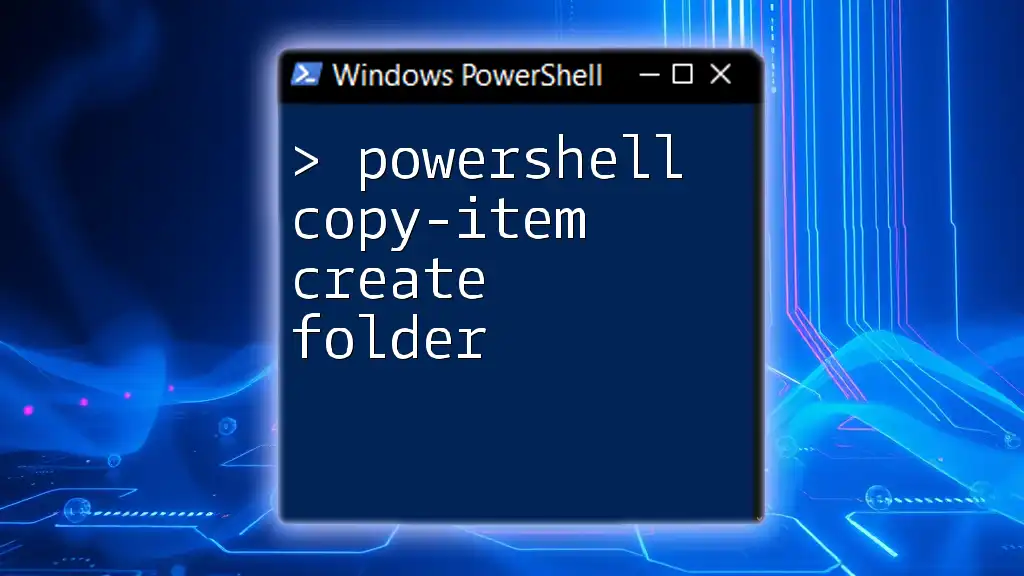
Conclusion
In closing, mastering the `Move-Item` cmdlet with the overwrite functionality is indispensable for effective file management in PowerShell. By leveraging the `-Force` parameter and adhering to safety precautions such as verifying file existence and creating backups, you can perform complex file operations seamlessly and with confidence.
As you venture further into the world of PowerShell, practicing these concepts will greatly enhance your file management skills. Explore its capabilities and integrate them into your workflow for more efficient operations.
Call to Action
Have you used the `Move-Item` cmdlet in your PowerShell adventures? Share your experiences and any tips you might have in the comments. For more PowerShell tips and tutorials, consider signing up for our newsletters and stay informed on the latest guides!
References
For additional reading and resources on `Move-Item` and other PowerShell commands, please refer to the official PowerShell documentation and community tutorials.