The `Copy-Item` command in PowerShell not only allows you to copy files and directories, but it can also create a new folder when the destination path does not exist.
Here's a code snippet to demonstrate how to use `Copy-Item` to create a folder while copying a file:
Copy-Item -Path "C:\source\example.txt" -Destination "C:\destination\newfolder\example.txt" -Force
In this example, if the `newfolder` does not exist in the destination path, PowerShell will create it automatically.
Understanding Copy-Item
What is Copy-Item?
The `Copy-Item` cmdlet is a critical command in PowerShell that allows users to copy files and directories from one location to another. It serves multiple purposes, such as backing up data, organizing files, or moving data between environments. By mastering `Copy-Item`, you can automate various file management tasks that would otherwise require manual intervention.
Syntax of Copy-Item
The general syntax for using `Copy-Item` is as follows:
Copy-Item -Path <String> -Destination <String> [-Recurse]
- `-Path`: Specifies the path to the item you want to copy. This can include files, directories, and wildcards.
- `-Destination`: Indicates where you want the item to be copied. This can be a folder or a new file name.
- `-Recurse`: A switch that allows copying of items recursively, meaning it includes all child items in the specified path.
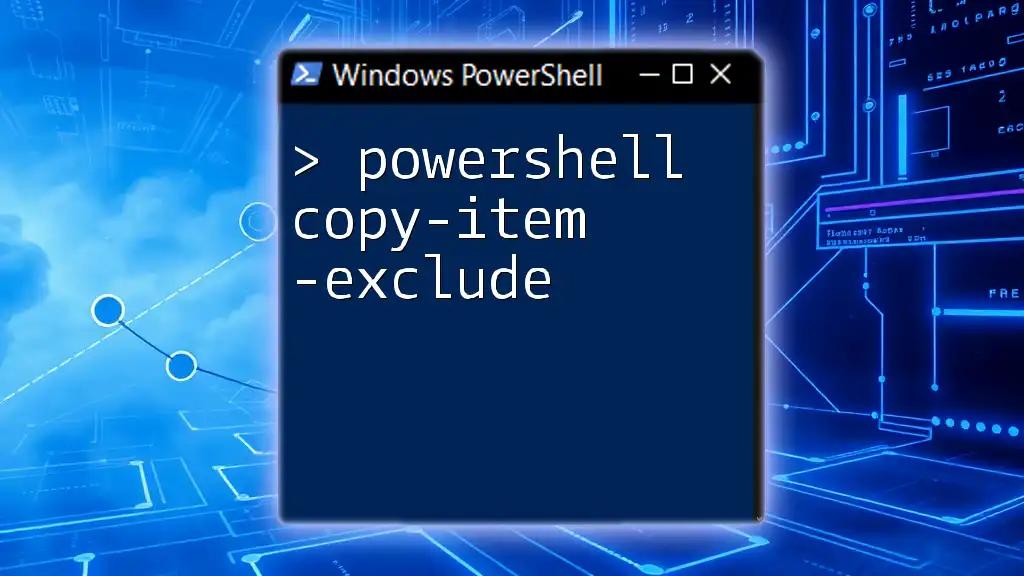
Creating Folders with Copy-Item
How to Create a Folder Using Copy-Item
You can leverage `Copy-Item` not just for copying files, but also for creating new folders by copying items into a non-existent destination. When attempting to copy items to a new folder, PowerShell will automatically create that folder if it doesn't already exist.
For instance, let's consider a scenario where you want to copy an entire folder of documents into a newly created backup folder:
Example Code Snippet
$sourcePath = "C:\SourceFolder"
$destinationPath = "C:\DestinationFolder\NewBackup"
Copy-Item -Path $sourcePath -Destination $destinationPath -Recurse
In this example:
- SourcePath: Specifies the folder containing the documents you want to back up.
- DestinationPath: Points to the new folder where the backup will be created.
- The `-Recurse` parameter ensures that all files and subdirectories from the source path are also copied.
Handling Non-Existent Destination
What Happens if the Destination Doesn't Exist?
If you attempt to use `Copy-Item` with a destination that does not exist, PowerShell will create that destination folder for you—provided it's a folder path. If the parent folder in the path does not exist, however, you will encounter an error.
To address this, you can use the `-Force` parameter, which compels PowerShell to create the folder structure, even if it encounters existing files with the same name.
Creating Nested Folders
Creating nested folders can also be achieved using `Copy-Item`. This can simplify your file management by allowing you to copy entire directory structures in a single command.
Example Code Snippet
$nestedDestinationPath = "C:\ParentFolder\ChildFolder\NewSubFolder"
Copy-Item -Path $sourcePath -Destination $nestedDestinationPath -Recurse
In this code:
- You can see how the path contains multiple levels.
- By using `-Recurse`, you ensure that all contents from `$sourcePath` are copied into the newly created subdirectory structure.
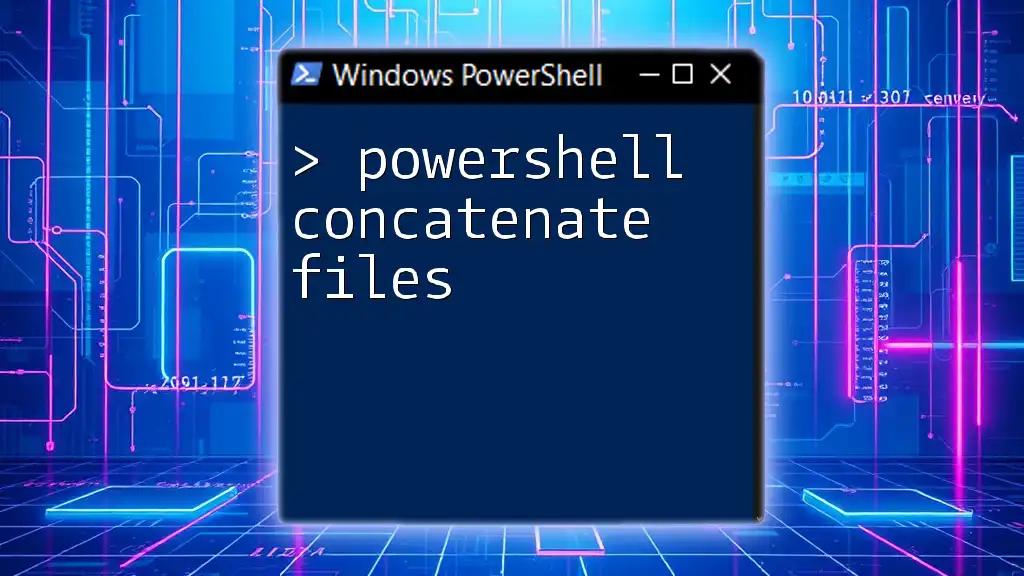
Advanced Techniques
Using Copy-Item with Wildcards
Wildcards can significantly streamline your operations. For example, if you want to copy all text files from a source folder to a destination folder, you can use the `*` wildcard.
Example Code Snippet
Copy-Item -Path "C:\SourceFolder\*.txt" -Destination "C:\DestinationFolder\TextFiles"
This command tells PowerShell to copy only the `.txt` files, allowing for more precise operations without copying unnecessary file types.
Combining Copy-Item with Other Cmdlets
You can also combine `Copy-Item` with other cmdlets like `New-Item` to create folders explicitly-ensuring they exist before performing copy operations.
Example Code Snippet
New-Item -Path "C:\NewFolder" -ItemType Directory
Copy-Item -Path "C:\SourceFolder\*" -Destination "C:\NewFolder"
Here, `New-Item` creates the "C:\NewFolder" directory first before moving all files from `C:\SourceFolder` into it. This combination not only ensures that your code runs without errors but also enhances clarity and reliability.
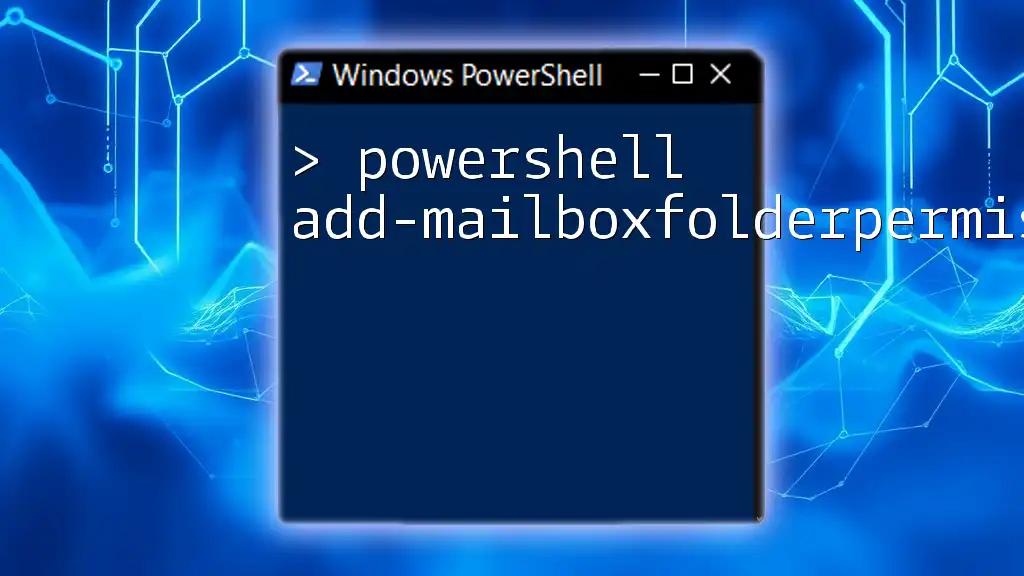
Troubleshooting Common Issues
Common Errors and How to Solve Them
Two common issues you might face when using `Copy-Item` include:
- Permissions-related errors: Ensure that you have the necessary permissions to access both the source and destination paths.
- Destination already exists: If the destination folder exists and you try to overwrite it without `-Force`, PowerShell will throw an error.
Best Practices for Using Copy-Item
- Verify Paths: Always double-check your source and destination paths to avoid accidental file loss.
- Use the `-WhatIf` Parameter: This parameter is incredibly useful as it simulates the action without making any changes.
Example Code Snippet
Copy-Item -Path $sourcePath -Destination $destinationPath -WhatIf
This way, you can see what would happen if the command were executed, helping you avoid costly mistakes.
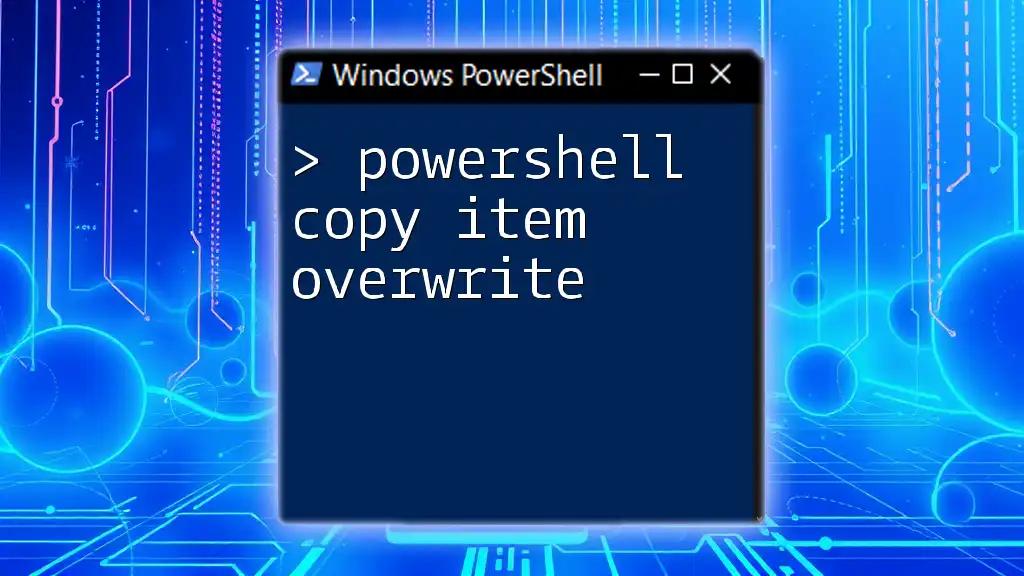
Conclusion
In this comprehensive guide, we have explored the powerful capabilities of the `Copy-Item` cmdlet in PowerShell, particularly its ability to create folders through effective file management. By understanding its syntax, leveraging advanced techniques, and adhering to best practices, you’ll be well-equipped to automate folder creation and file copying tasks efficiently.
PowerShell is a versatile tool, and by practicing these commands, you can enhance your automation skills significantly. Take the time to explore further, and remember to share your experiences or questions in the comments section!