To delete empty folders in PowerShell, you can use the `Remove-Item` cmdlet in combination with `Get-ChildItem` to find and remove all empty directories within a specified path.
Here's a code snippet for that:
Get-ChildItem -Path "C:\Your\Directory\Path" -Directory | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item
Understanding Empty Folders
What Defines an Empty Folder?
An empty folder is a directory that does not contain any files or subdirectories. This absence includes not just visible files but also hidden or system files. Recognizing truly empty folders is crucial, as deleting folders that contain indispensable files can lead to data loss.
Why Delete Empty Folders?
Managing empty folders can significantly enhance your file system's organization. Regularly deleting these folders helps to:
- Unclutter your file structure: An overabundance of empty folders can make navigation cumbersome.
- Improve system performance: Although the performance impact might be minor, a cleaner structure can facilitate quicker access to needed directories.
- Enhance organization: By removing unnecessary clutter, you can improve the overall usability of your file system.

Getting Started with PowerShell
Opening PowerShell
To begin your journey into using PowerShell, you first need to open it. Here’s how:
- Press `Windows + X` to open the Power User menu.
- Click on Windows PowerShell (or Windows PowerShell (Admin) if you need administrative privileges).
Basic PowerShell Command Syntax
Understanding the syntax of PowerShell commands (or cmdlets) will aid in executing operations smoothly. A cmdlet typically follows this structure:
Verb-Noun -Parameter Value
For example, in `Remove-Item -Path C:\Example`, `Remove-Item` is the cmdlet, and `-Path` is the parameter that specifies the item to be removed.
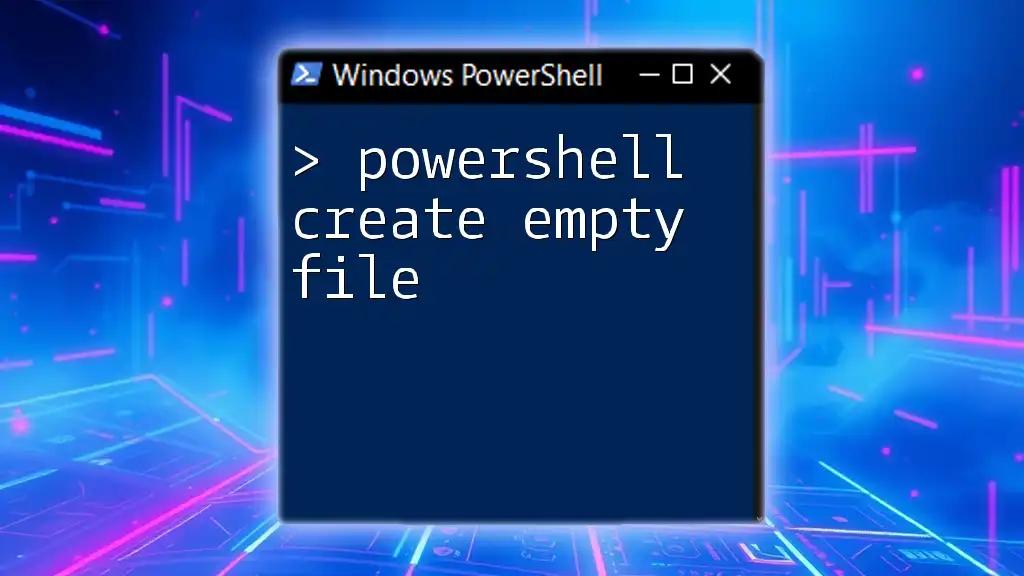
Deleting Empty Folders in PowerShell
Using the Remove-Item Cmdlet
The Remove-Item cmdlet is essential for deleting items in PowerShell, including folders. Here’s how to delete a specific empty folder:
Remove-Item -Path "C:\Example\EmptyFolder" -Force
The `-Force` parameter ensures that PowerShell does not prompt for confirmation before deleting the folder.
Searching for Empty Folders
Using Get-ChildItem
Before deleting, you might want to identify which folders are empty. The Get-ChildItem cmdlet is invaluable for listing folders. You can find empty folders as follows:
Get-ChildItem -Path "C:\Example" -Directory | Where-Object { $_.GetFileSystemInfos().Count -eq 0 }
In this command, `-Directory` ensures only directories are listed, and the `Where-Object` filter checks if the folder contains files. If the count is zero, the folder is deemed empty.
Combining Commands: Finding and Deleting
To streamline the process, you can combine finding and deleting empty folders into a single command:
Get-ChildItem -Path "C:\Example" -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item -Force
This command will recursively search for empty folders starting from the specified path and delete them immediately.
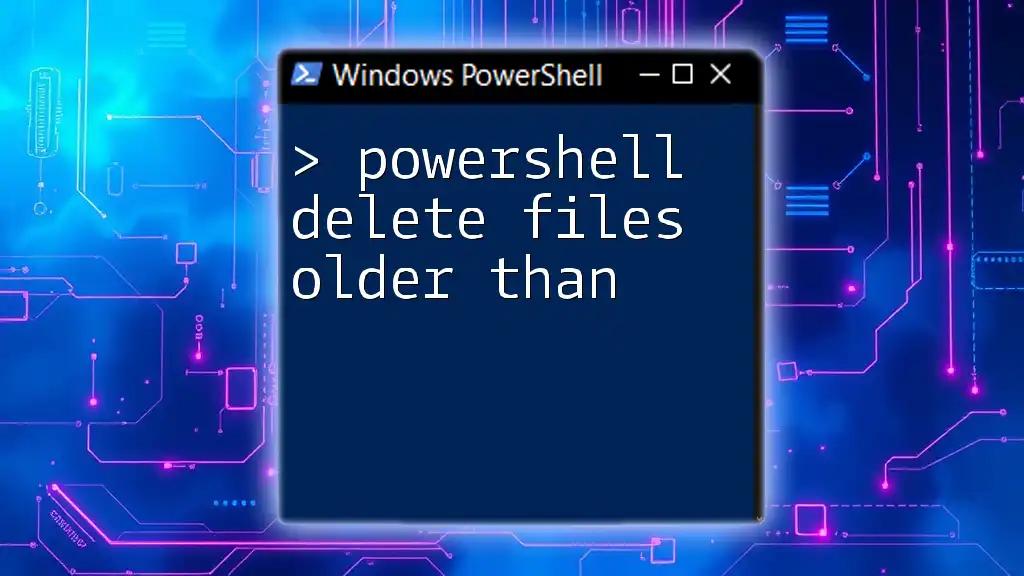
Using Advanced Techniques
Recursive Deletion of Empty Folders
Including the `-Recurse` parameter means PowerShell will search through all nested directories. While this adds convenience, it’s essential to use it judiciously. You may unintentionally delete empty folders deep within your directory structure.
Handling Errors
Try-Catch Blocks
To ensure your scripts run smoothly and errors don’t halt your progress, you can use Try-Catch blocks. This error handling approach makes your commands robust. Here’s an example:
try {
Get-ChildItem -Path "C:\Example" -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item -Force
} catch {
Write-Host "An error occurred: $_"
}
This setup allows you to catch and display errors without crashing your process.

Best Practices for Using PowerShell
Testing Commands Before Deletion
To prevent accidental deletion of crucial folders, always test your commands first. Utilize the `-WhatIf` parameter to simulate the action without making any changes:
Get-ChildItem -Path "C:\Example" -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item -Force -WhatIf
This command will show you what would happen without actually deleting any folders.
Regular Maintenance
Incorporating regular checks for empty folders into your routine can keep your file system tidy. Utilizing Task Scheduler to automate these checks can ensure consistent maintenance.
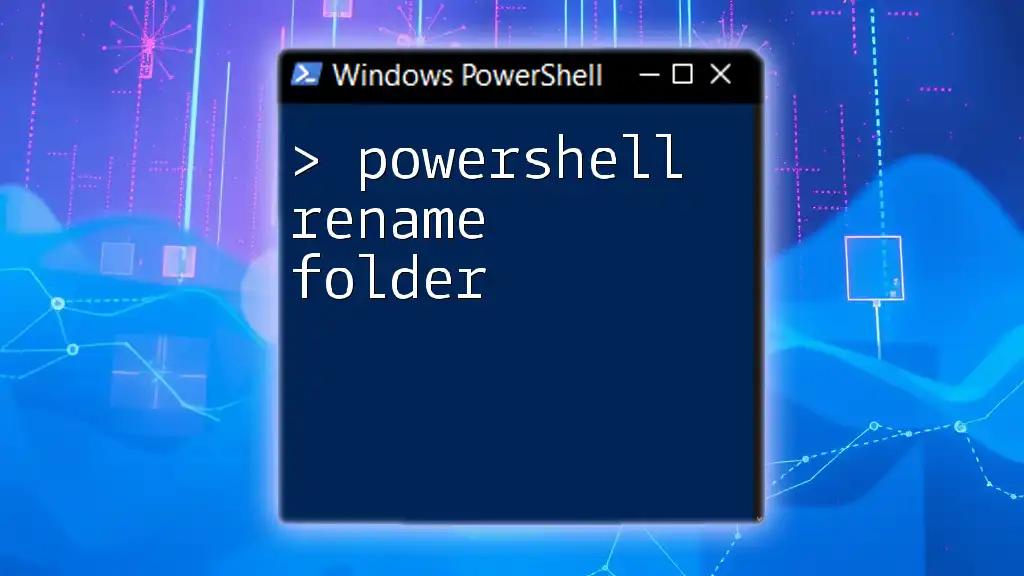
Common Use Cases
Understanding when to delete empty folders can guide effective file management. Some scenarios include:
- Post-software installation: Many applications create installation folders that become empty after the process.
- After moving files: Moving or organizing files can result in leftover empty directories that should be cleaned up.

Conclusion
In summary, developing a habit of managing empty folders with PowerShell can enhance both the organization and performance of your file system. Regular practice of these commands will not only improve your skills but also keep your environment efficient. Embracing these strategies discussed in this guide will pave the way for a well-maintained file structure.

Further Reading and Resources
Explore the official Microsoft PowerShell documentation for in-depth knowledge and advanced commands. Consider investing in books and courses dedicated to PowerShell for a more structured learning experience.