To delete a folder in PowerShell only if it exists, you can use the following command:
if (Test-Path "C:\Path\To\Your\Folder") { Remove-Item "C:\Path\To\Your\Folder" -Recurse -Force }
Understanding PowerShell Command Basics
What is PowerShell?
PowerShell is a powerful task automation and configuration management framework developed by Microsoft. It consists of a command-line shell and an associated scripting language that allows users to execute commands, known as cmdlets, and automate various administrative tasks on Windows and other platforms.
PowerShell's versatility and capability to integrate with various services make it an essential tool for system administrators and developers alike. The framework enables users to perform system management tasks with ease, from file manipulation to advanced cloud management.
Why Use PowerShell for Folder Management?
Using PowerShell for folder management is advantageous due to its automation capabilities, allowing users to script and execute repetitive tasks quickly. For example, if you need to delete temporary files or clean up outdated folders regularly, a PowerShell script can save you time and effort while ensuring precision and consistency.
PowerShell also provides robust error handling, meaning that you can design scripts to manage potential issues effectively, ensuring minimal disruption to your workflow.
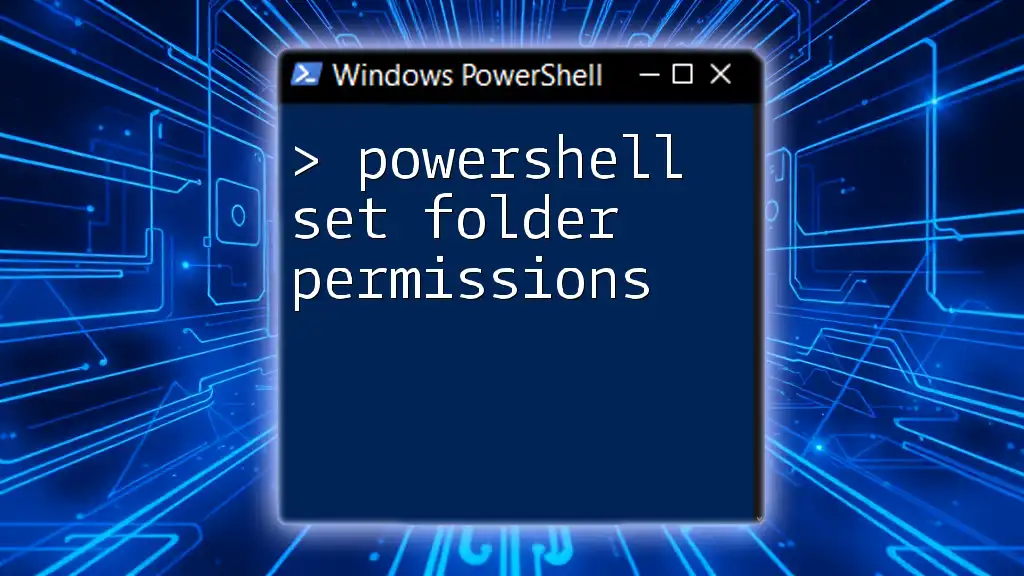
Checking if a Folder Exists
Using `Test-Path`
Before performing any action on a folder, it is crucial to check whether it exists. PowerShell’s `Test-Path` cmdlet is specifically designed for this purpose. It verifies the existence of files and folders, returning a Boolean value: `True` if the specified path exists and `False` if it does not.
Here’s a straightforward example of how to use `Test-Path`:
$folderPath = "C:\Path\To\Your\Folder"
if (Test-Path $folderPath) {
Write-Host "The folder exists."
} else {
Write-Host "The folder does not exist."
}
In this example, we define the variable `$folderPath` with the target folder's path. By utilizing `Test-Path`, we confirm whether the folder exists before executing any further actions. This step is vital as it prevents unnecessary errors during execution.
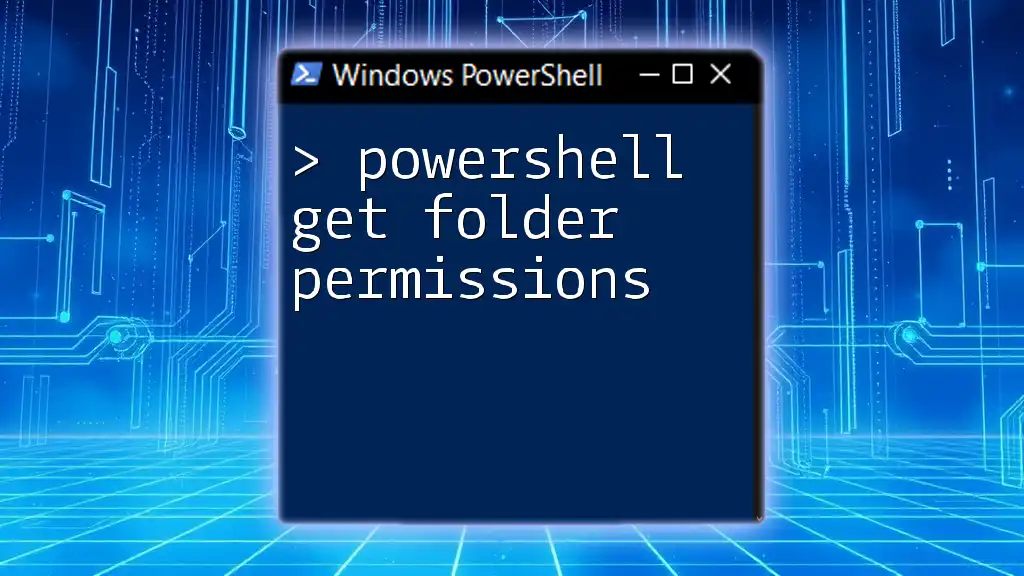
Deleting a Folder
Using `Remove-Item`
Once we establish that a folder exists, we can proceed to delete it using the `Remove-Item` cmdlet. This command allows you to delete files, folders, and other items from a specified path.
Here’s the basic syntax for deleting a folder:
Remove-Item -Path "C:\Path\To\Your\Folder" -Recurse -Force
In this snippet, the `-Recurse` parameter instructs PowerShell to delete all contents within the folder, while the `-Force` parameter bypasses any prompts that may normally appear, allowing for a smoother, uninterrupted operation.
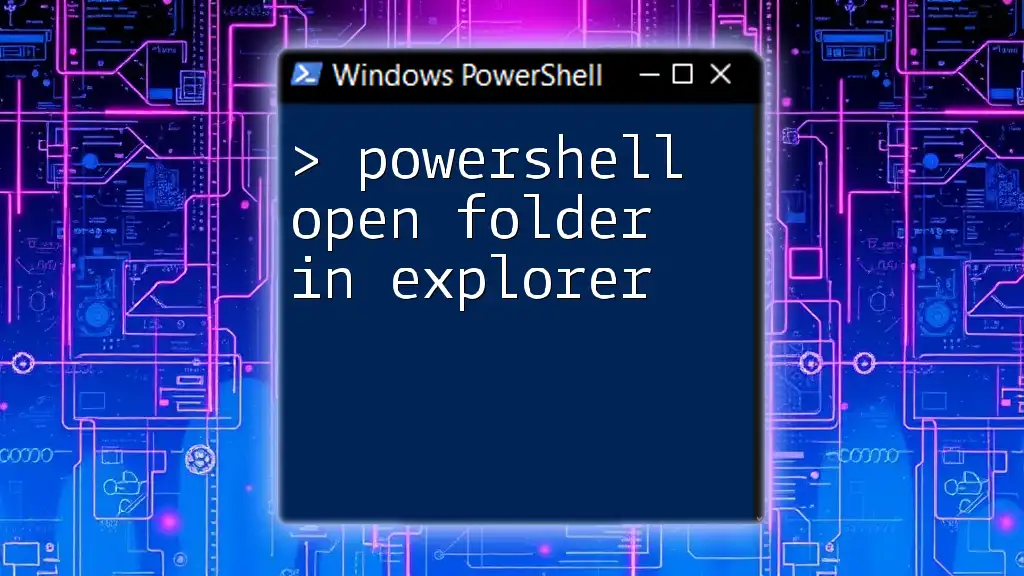
Combining Checks and Deletion
Deleting a Folder Only if It Exists
To efficiently utilize PowerShell for folder deletion, combining existence checks with the deletion command in a single script allows for a seamless process. This approach ensures that no actions are taken on non-existent folders, thereby avoiding potential errors.
Here’s how to implement this:
$folderPath = "C:\Path\To\Your\Folder"
if (Test-Path $folderPath) {
Remove-Item -Path $folderPath -Recurse -Force
Write-Host "Folder deleted."
} else {
Write-Host "Folder does not exist, no action taken."
}
This script first checks if the folder exists. If it does, it proceeds with deletion and notifies the user. If not, it informs the user that no action will be taken. This flow control in PowerShell not only improves efficiency but also enhances user experience by providing feedback.
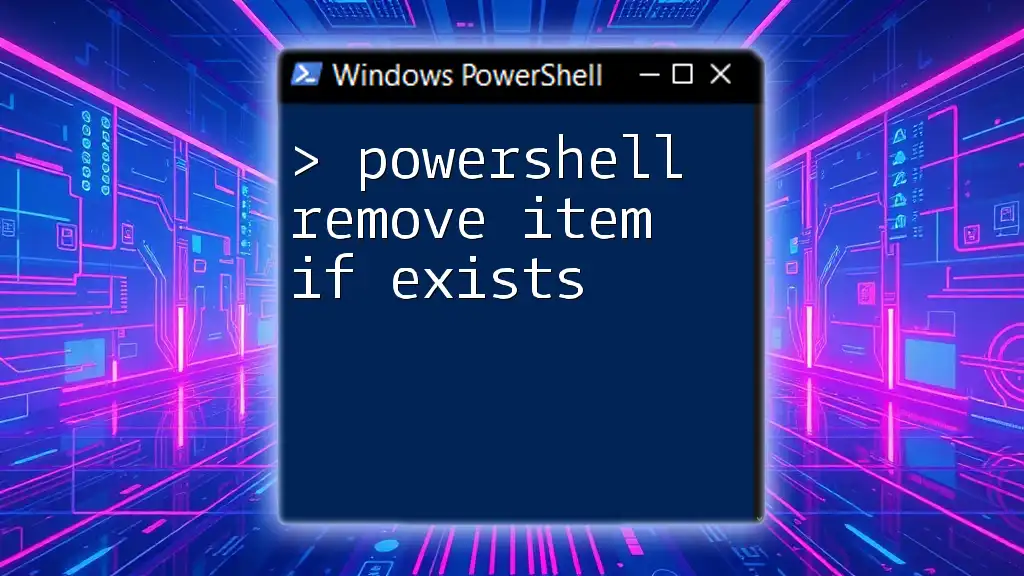
Common Pitfalls and Best Practices
Caution with `-Recurse`
While the `-Recurse` parameter is a powerful feature, it must be used with caution. Its function may lead to unintended data loss, especially if the specified path includes important files or folders that are not intended for deletion. As a best practice, always double-check the folder path and contents before executing commands that modify or delete data.
Logging Actions
To maintain clarity and accountability within your scripts, consider implementing logging for any deletion actions. Logging enables you to track what actions were taken during script execution, making it easier to audit and review activity.
Here’s a simple way to log deletions:
$logFile = "C:\Path\To\Your\log.txt"
if (Test-Path $folderPath) {
Remove-Item -Path $folderPath -Recurse -Force
Add-Content -Path $logFile -Value "$(Get-Date): Deleted folder $folderPath"
}
Incorporating logging like this not only serves as a record for future reference but also helps in troubleshooting in case something goes wrong.
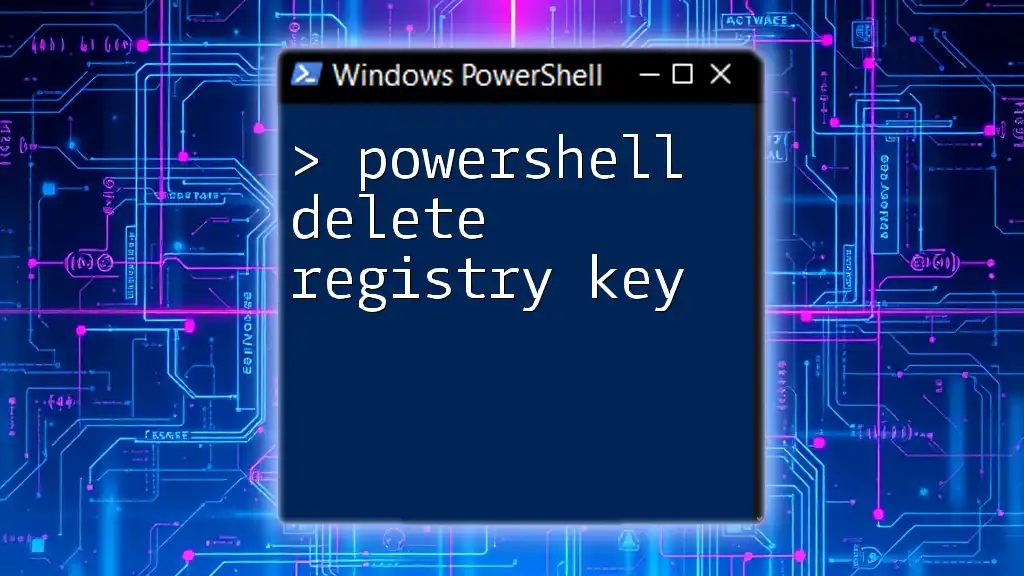
Conclusion
In summary, mastering how to use PowerShell to delete a folder if it exists is a fundamental skill that enhances your ability to manage system resources efficiently. By leveraging commands such as `Test-Path` and `Remove-Item`, you can create scripts that handle folder management seamlessly.
Remember to always follow best practices when deleting folders, including verifying paths and implementing logging for any significant operations. By doing so, you can ensure that your scripts run smoothly and that data integrity is maintained.
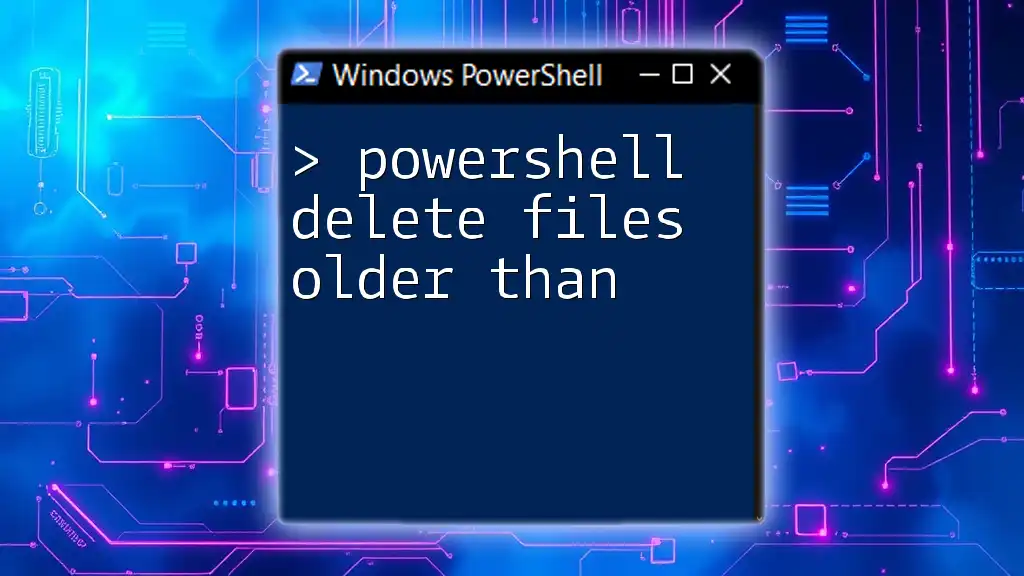
Additional Resources
Recommended PowerShell Documentation
For further reading, check out the official [Microsoft PowerShell documentation](https://docs.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.2) to deepen your understanding and explore advanced concepts.
Related Topics in PowerShell
Consider diving into other PowerShell commands related to file management, such as `Get-ChildItem`, `Copy-Item`, and `Move-Item`, to expand your automation toolkit.
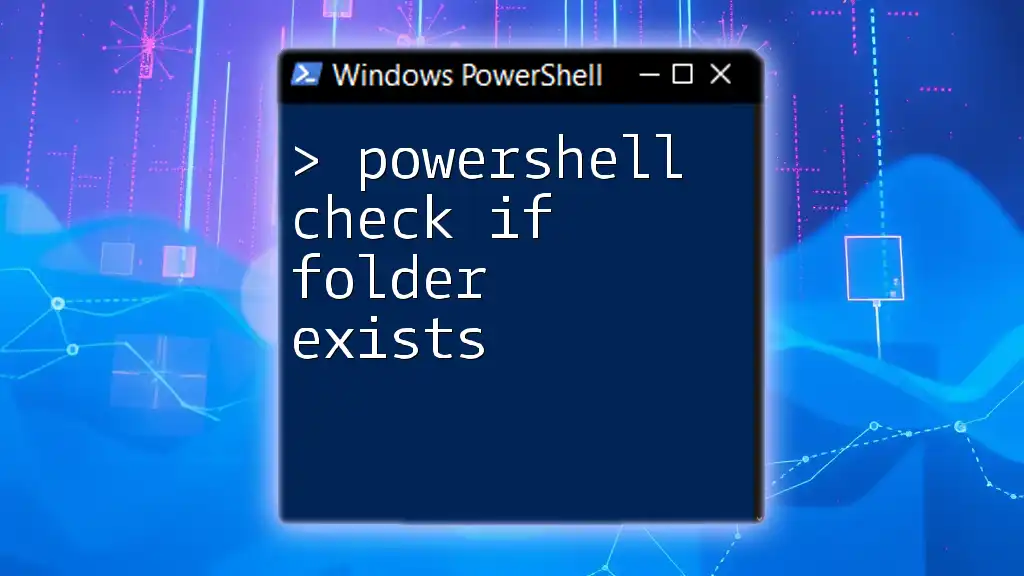
Call to Action
To elevate your PowerShell skills further, consider subscribing to our blog for more insights and tips, or sign up for our dedicated PowerShell training sessions. As a special incentive, you can receive a free guide on PowerShell basics as you start your journey in mastering this invaluable tool.