In PowerShell, you can delete files older than a specified number of days by using the `Get-ChildItem` and `Remove-Item` cmdlets together.
Here's a code snippet to achieve that:
$days = 30
$targetPath = "C:\Path\To\Directory"
Get-ChildItem -Path $targetPath -File | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-$days) } | Remove-Item
This command will remove files in the specified directory that haven't been modified in the last 30 days.
Understanding PowerShell's Cmdlets for File Management
What is a Cmdlet?
In PowerShell, a cmdlet is essentially a lightweight command designed to perform a single function. These cmdlets follow a verb-noun naming convention, making them intuitive to understand and use. For instance, `Get-ChildItem` retrieves items (files and folders) from a specified location, while `Remove-Item` deletes specified items. This structured approach to command execution empowers users to easily manipulate the file system.
Common Cmdlets for File Manipulation
Get-ChildItem is a foundational cmdlet used to list files and directories. You can think of it as a powerful file explorer within your command line. The Remove-Item cmdlet, as its name suggests, is the tool you'll use to delete files or folders from your system. Combine these cmdlets to manage your file system effectively.

Prerequisites for Deleting Files
Requirements Before Running the Commands
Before diving into file deletion, being aware of a few important prerequisites can save you from making critical errors. Administrative privileges are often necessary when deleting certain files, especially those located in system folders. If you lack the right permissions, you'll encounter errors when executing your commands.
Additionally, implementing backup strategies is crucial. Always secure essential data before running any deletion scripts. Data loss can be irreversible, and what may seem like a redundant file today could be crucial in the future.
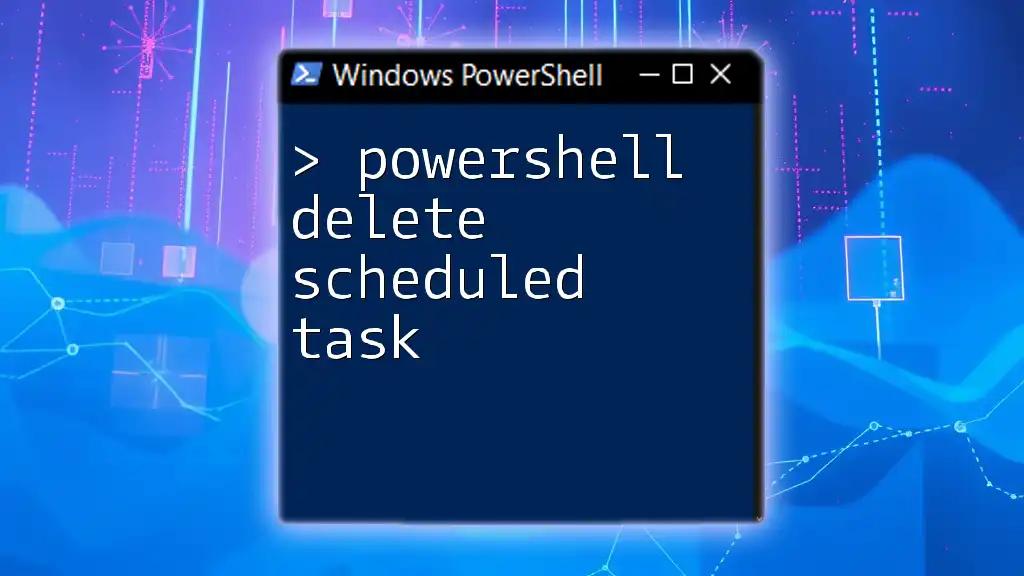
The Basics of Deleting Files Older Than a Specified Date
Using Get-ChildItem and Remove-Item Together
Deleting files older than a certain date is straightforward using PowerShell. You can leverage the combination of Get-ChildItem and Remove-Item cmdlets. Understanding how PowerShell assesses file age is vital. It uses properties like `LastWriteTime` to determine when a file was last modified.
Here's an essential command that will help you delete files older than 30 days in a specific directory:
Get-ChildItem "C:\Path\To\Directory" | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item
This command does the following:
- Get-ChildItem gathers all files in the specified directory.
- Where-Object filters the files based on their `LastWriteTime`. The condition checks if the last write date is older than 30 days.
- Remove-Item deletes the files that meet these criteria.
Breaking Down the Command
Get-ChildItem Explanation
The Get-ChildItem cmdlet can take several parameters that enhance its functionality. For example, you can use the `-Recurse` parameter to search within subdirectories and include all nested items. This is useful for operations across multiple directories.
Where-Object Explanation
The Where-Object cmdlet works by filtering the pipeline output based on conditions you define. In our example, we check the `LastWriteTime` property of each file. You can customize this property to evaluate other attributes, such as creation date or length.
Remove-Item Explanation
When you reach the Remove-Item cmdlet, you are ready to delete. However, be cautious; deletion is often irreversible. It's essential to ensure that you're operating on the right files, as misclassification can lead to data loss.
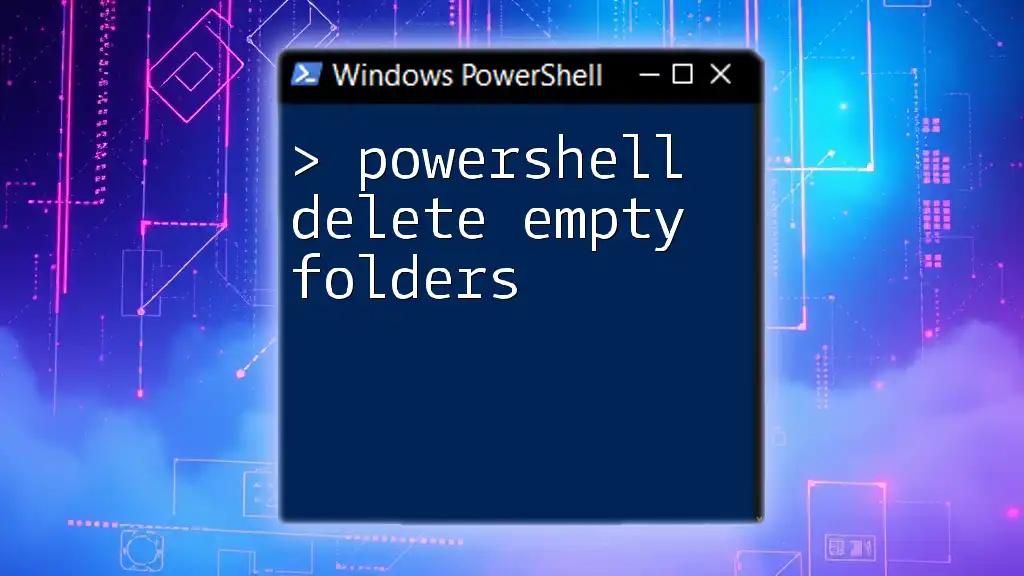
Advanced Techniques for Deleting Files
Using Filters with Get-ChildItem
Filtering by File Types
You can refine your deletions by specifying file types. Suppose you only want to delete log files older than 30 days. The command would look like this:
Get-ChildItem "C:\Path\To\Directory\*.log" | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item
By using the `*.log` filter, you're narrowing down your command to operate solely on log files, which can help avoid unintended deletions of other file types.
Using -Filter Parameter
In PowerShell, the `-Filter` parameter can significantly improve performance when searching for files. This parameter lets you specify a wildcard filter at the file system level rather than within PowerShell, which can be much faster. Here’s how you might use it:
Get-ChildItem -Path "C:\Path\To\Directory" -Filter *.log | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item
This command is optimized to find log files immediately rather than pulling all items and filtering them afterward.
Scheduling Automatic Deletion with Task Scheduler
If you want to make file deletions a routine task, consider using Task Scheduler in Windows. This allows scripts to run automatically at specified intervals.
Here's how to create a scheduled task for a deletion script:
# Schedule a script to run daily at 3 AM
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "C:\Path\To\YourScript.ps1"
$trigger = New-ScheduledTaskTrigger -Daily -At 3am
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "DeleteOldFiles" -Description "Deletes files older than 30 days."
In this example:
- New-ScheduledTaskAction specifies the action (running your PowerShell script).
- New-ScheduledTaskTrigger defines when the task should run.
- Register-ScheduledTask finalizes the creation of this automated task.

Best Practices for File Deletion
Testing Before Execution
Before executing potentially destructive commands, it’s wise to test them. Leverage the -WhatIf parameter to simulate command execution without making any changes:
Get-ChildItem "C:\Path\To\Directory" | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item -WhatIf
This command will show you what files would be deleted without actually performing the deletion, offering a chance to double-check your criteria.
Logging and Auditing Actions
To maintain accountability and traceability, implement logging in your scripts. Logging will help keep track of what files were deleted and when:
$logFile = "C:\Path\To\DeleteLog.txt"
Get-ChildItem "C:\Path\To\Directory" | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | ForEach-Object {
Remove-Item $_.FullName
Add-Content -Path $logFile -Value "$($_.FullName) deleted on $(Get-Date)"
}
In this script:
- We're logging the full path of each file deleted alongside the timestamp, allowing for comprehensive record-keeping.
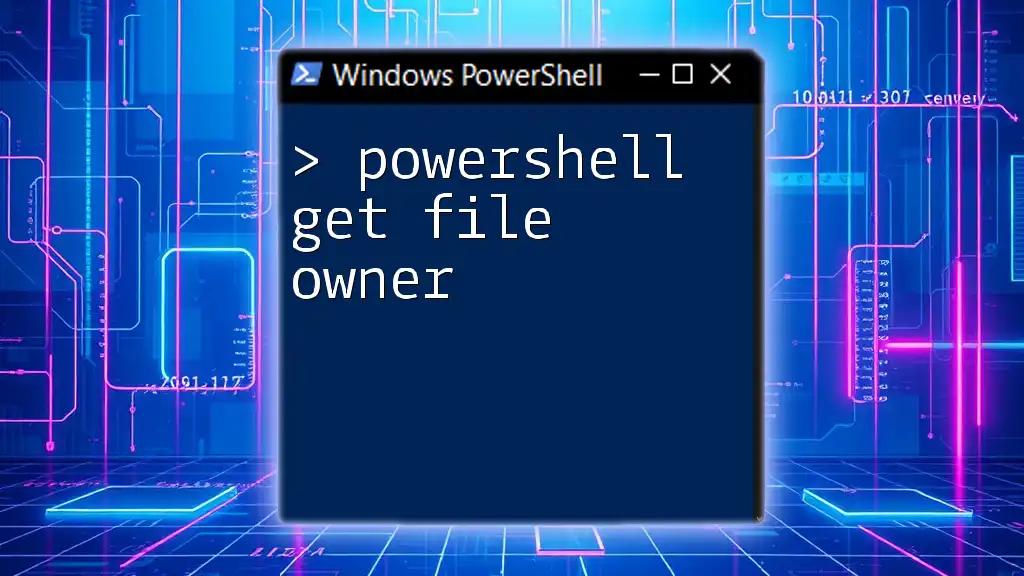
Troubleshooting Common Issues
Common Errors During Deletion
As with any command, errors may arise:
Access Denied Errors: Often occur when attempting to delete files in protected directories. If you encounter this, ensure your PowerShell session is running with the necessary administrative privileges.
File in Use Errors: Sometimes, files in use by applications cannot be deleted. Identifying the application and closing it may resolve this issue.
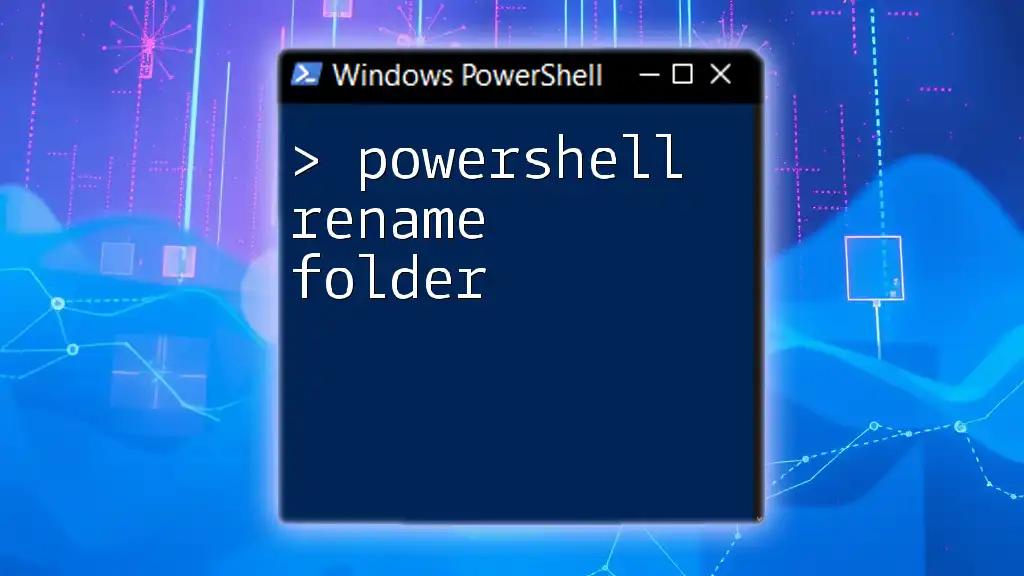
Conclusion
In summary, understanding how to PowerShell delete files older than a specified date can streamline your file management processes and improve system performance. The combination of cmdlets like Get-ChildItem and Remove-Item gives you control and flexibility. By implementing best practices such as testing deletions and logging actions, you can ensure that your file management is not only efficient but also safe.
As you become more familiar with these commands, you can further customize scripts to suit your specific needs. Embrace the power of PowerShell and start optimizing your file management today!

Additional Resources
For further reading on PowerShell cmdlets and file management practices, consider exploring the official Microsoft Documentation. Additionally, joining forums or communities focused on PowerShell can provide ongoing support and share advanced techniques for optimizing your scripting skills.