To set folder permissions in PowerShell, you can use the `Set-Acl` command along with a defined access control list (ACL) to control user permissions on a specified folder. Here's a code snippet:
$acl = Get-Acl "C:\Path\To\Your\Folder"
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("UserName","FullControl","Allow")
$acl.SetAccessRule($rule)
Set-Acl "C:\Path\To\Your\Folder" $acl
Understanding Folder Permissions in Windows
Folder permissions control who can access specific files and directories in a Windows environment. Understanding these permissions is crucial for effective system administration. Permissions are categorized primarily into Read, Write, and Modify.
- Read: Allows users to view the contents of a folder but not make changes.
- Write: Grants the ability to make changes within the folder, including adding and deleting files.
- Modify: A combination of Read and Write, enabling the modification of existing files.
Windows primarily uses two types of permissions: NTFS permissions and Share permissions. It's essential to distinguish between the two, as NTFS permissions apply to local user accounts and groups while Share permissions are relevant when folders are accessed over a network.
Additionally, understanding inheritance in folder permissions is vital. When permissions are set on a parent folder, those permissions can automatically apply to all subfolders and files within it, thus simplifying management.
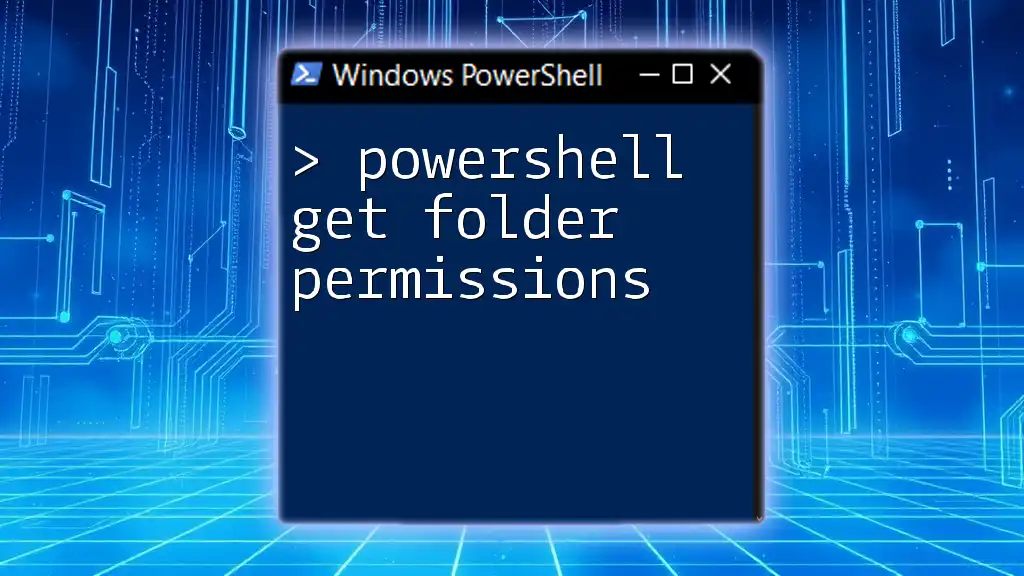
Using PowerShell to Set Folder Permissions
Overview of PowerShell Cmdlets for Managing Permissions
PowerShell is a powerful tool for managing folder permissions. Several key Cmdlets simplify this task:
- `Get-Acl`: Retrieves the access control list for a specified file or folder.
- `Set-Acl`: Applies a new access control list to a file or folder.
- `Add-AccessControlEntry`: Adds a new access rule to the current ACL.
- `Remove-AccessControlEntry`: Removes existing access rules.
These Cmdlets allow for comprehensive management of folder permissions, enabling users to set, modify, and review permissions efficiently.
Setting Permissions on a Folder with PowerShell
Using `Set-Acl` Cmdlet
To set permissions on a particular folder, the `Set-Acl` Cmdlet is essential. The syntax is straightforward, as shown in this example:
$acl = Get-Acl "C:\YourFolderName"
$permission = "DOMAIN\UserOrGroupAccessRights"
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule($permission, "FullControl", "Allow")
$acl.SetAccessRule($accessRule)
Set-Acl "C:\YourFolderName" $acl
In this code snippet:
- `Get-Acl` fetches the current ACL for "C:\YourFolderName".
- A new access rule is created allowing full control for a specified user or group.
- Finally, `Set-Acl` applies the modified ACL back to the folder.
Be cautious of the permission level you assign. Assigning overly permissive access can lead to security vulnerabilities.
Setting Permissions on Folder, Subfolders, and Files
Using Recursive ACLs
Setting permissions on just a folder often isn't enough; you'll typically want to apply settings across all subfolders and files. This can be achieved by using recursive ACLs.
Here’s how you can set recursive permissions:
$path = "C:\YourFolder"
$acl = Get-Acl $path
$permission = "DOMAIN\UserOrGroupAccessRights"
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule($permission, "Modify", "Allow")
$acl.SetAccessRule($accessRule)
Get-ChildItem $path -Recurse | ForEach-Object { Set-Acl $_.FullName $acl }
In this example:
- The path for which permissions will be set is defined.
- The current ACL for that path is retrieved.
- A new access rule is established, and the rule is then applied recursively across all contained items.
This approach is highly effective for applying consistent access levels across large directory trees.
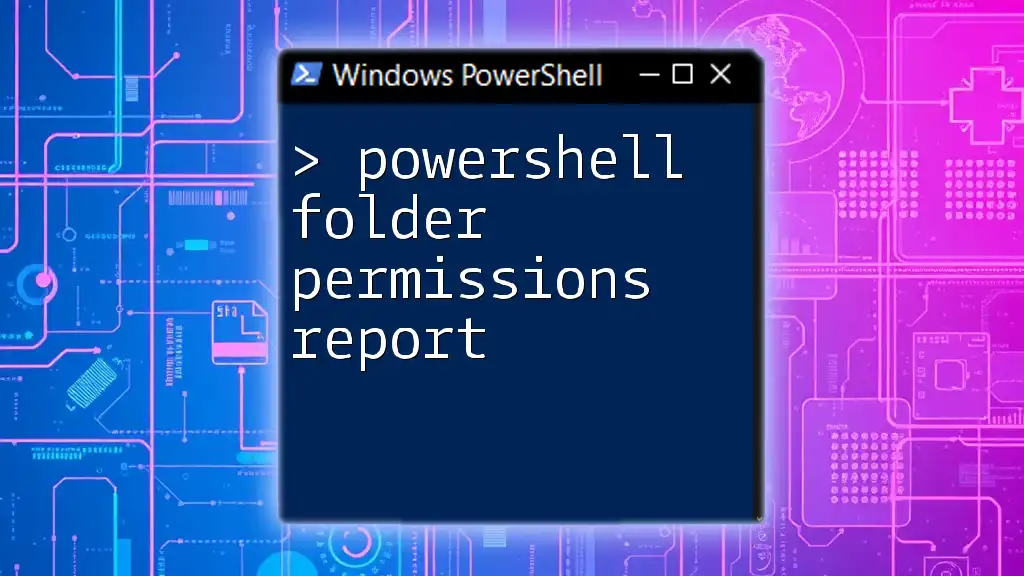
PowerShell Commands to Modify Existing Folder Permissions
Viewing Current Permissions
Before making changes, it’s crucial to understand what permissions are currently set. You can easily view current permissions with the `Get-Acl` command:
Get-Acl "C:\YourFolderName" | Format-List
This command will produce a detailed output of the existing permissions for the specified folder, allowing you to assess whether modifications are necessary.
Changing Existing Permissions
Removing Permissions
Sometimes you may need to remove specific permissions instead of adding them. You can do this using the `Remove-AccessControlEntry` Cmdlet. Here’s an example snippet:
$acl = Get-Acl "C:\YourFolderName"
$permission = "DOMAIN\UserOrGroupAccessRights"
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule($permission, "FullControl", "Deny")
$acl.RemoveAccessRule($accessRule)
Set-Acl "C:\YourFolderName" $acl
In this case:
- Get-Acl retrieves the existing ACL for the folder.
- You create a rule to deny the specified access.
- Set-Acl is then used to apply the updated ACL.
Be cautious with removal actions; ensuring that you do not inadvertently restrict necessary access to essential files is crucial.
Practical Examples
Consider a development folder shared among team members. You might want to ensure that developers can modify files but not delete them. Here’s how you can apply such permissions succinctly using PowerShell:
$path = "C:\DevFolder"
$acl = Get-Acl $path
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule("DevelopersGroup", "Modify", "Allow")
$acl.SetAccessRule($accessRule)
Set-Acl $path $acl
For collaboration, you might want to grant Read & Execute permissions to a team, whereas critical admin functions should maintain Full Control for specific users.
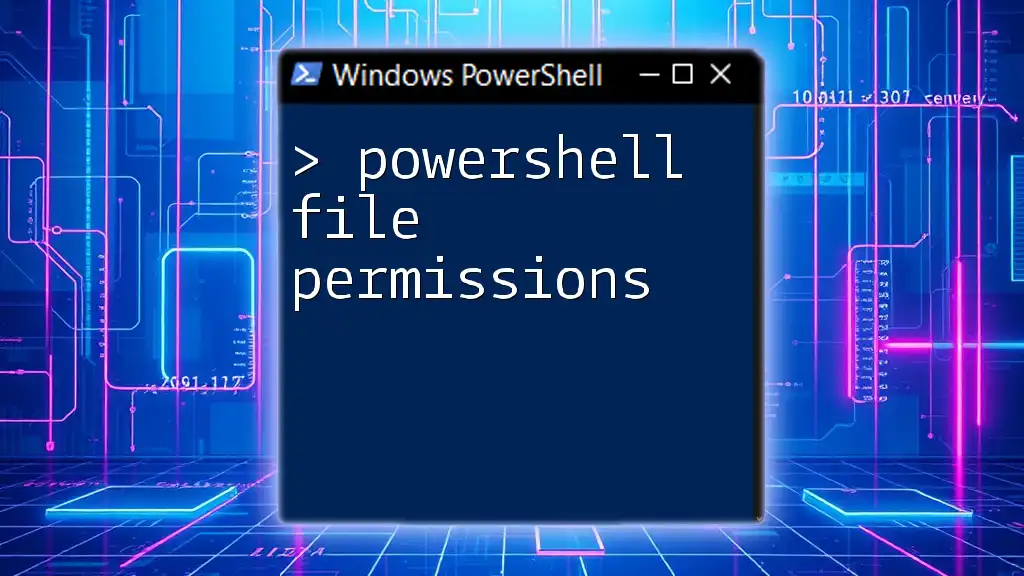
Best Practices for Managing Folder Permissions with PowerShell
- Conduct regular audits of folder permissions to ensure compliance with security policies.
- Use descriptive comments in your scripts to provide clarity for future administrators reviewing your work.
- Document all changes to permissions in a change log, holding individuals accountable and maintaining transparency within your team.
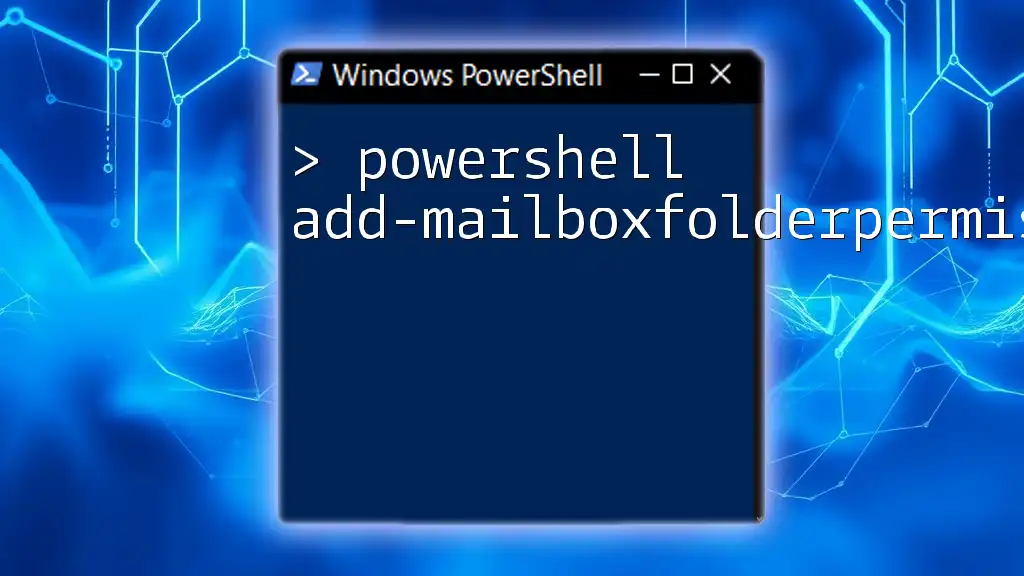
Troubleshooting Common Issues
When managing folder permissions, you may encounter some typical issues, such as access denied errors. It’s critical to check:
- If the user running the script has sufficient privileges.
- Whether there are conflicting rules impacting the effective access rights.
- Confirm if inheritance settings are applied correctly.
Testing permission changes in a non-production environment is advisable before deploying changes widely to prevent unexpected disruptions.
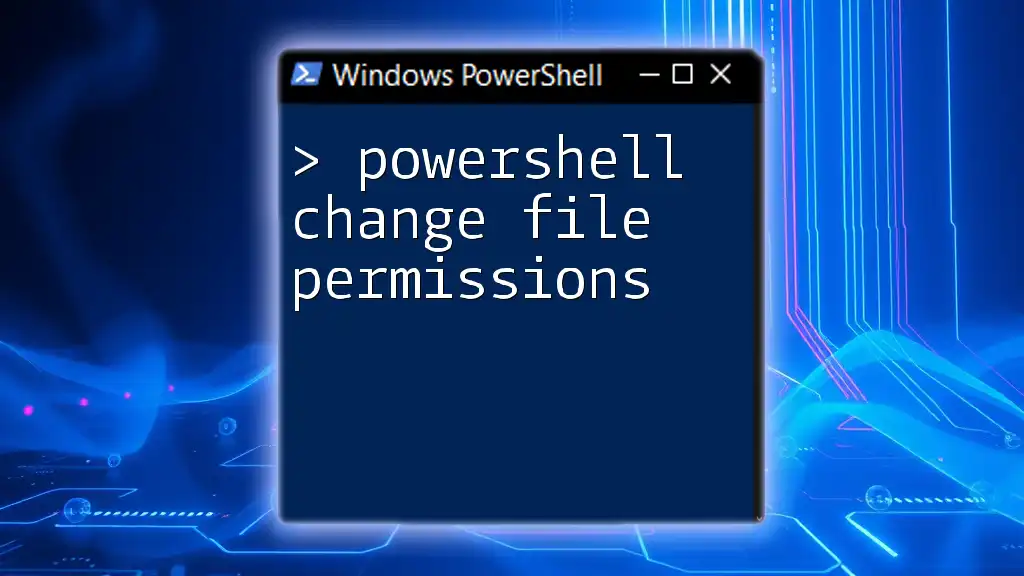
Conclusion
Managing folder permissions using PowerShell is not just a necessary skill but an essential one for effective system administration. With the examples and techniques outlined, you now have the tools to set, modify, and review folder permissions confidently.
Experimenting with these commands will deepen your understanding and enable you to effectively safeguard your systems against unauthorized access. Remember, as with any administrative task, to proceed with caution and be diligent in your implementation practices.