To quickly retrieve the permissions of a folder and its subfolders using PowerShell, you can use the `Get-Acl` cmdlet combined with a loop through all items in the directory.
Here's a concise code snippet to accomplish this:
Get-ChildItem 'C:\Your\Folder\Path' -Recurse | Get-Acl | Select-Object Path, Access
This command will display the path and access permissions for all files and folders within the specified directory.
Understanding NTFS Permissions
What are NTFS Permissions?
NTFS (New Technology File System) permissions determine what users and groups can do with files and folders on a Windows operating system. These permissions can vary from granting basic read access to allowing full control over files. Understanding these permissions is crucial for data security and management.
Among the various types of permissions, you'll frequently encounter:
- Read: Allows viewing of files and folders.
- Write: Allows adding or changing files.
- Modify: Allows modifying files/folders and deleting them.
- Full Control: Grants all permissions, including changing permissions for others.
Importance of Managing Permissions
Properly managing folder and subfolder permissions is critical in any environment where data sensitivity is a concern. Mismanaged permissions can lead to unauthorized access, data leaks, and compliance violations—issues that can damage an organization's reputation and bottom line.
Common scenarios where checking permissions is vital include:
- Preparing for an audit.
- Troubleshooting access issues for users.
- Enforcing stricter data access policies.

Preparing Your PowerShell Environment
Running PowerShell as Administrator
To effectively manage folder permissions using PowerShell, administrative privileges are often required. To launch PowerShell with these privileges:
- Press `Windows + X` and select Windows PowerShell (Admin) or Windows Terminal (Admin).
- Confirm any User Account Control prompts that appear.
Ensuring Required Modules are Loaded
Before diving into commands, ensure you have the necessary modules. You can check for installed modules by running:
Get-Module
While the `Get-Acl` cmdlet, which retrieves permissions, is built into PowerShell, having the latest version of PowerShell can improve performance and compatibility.
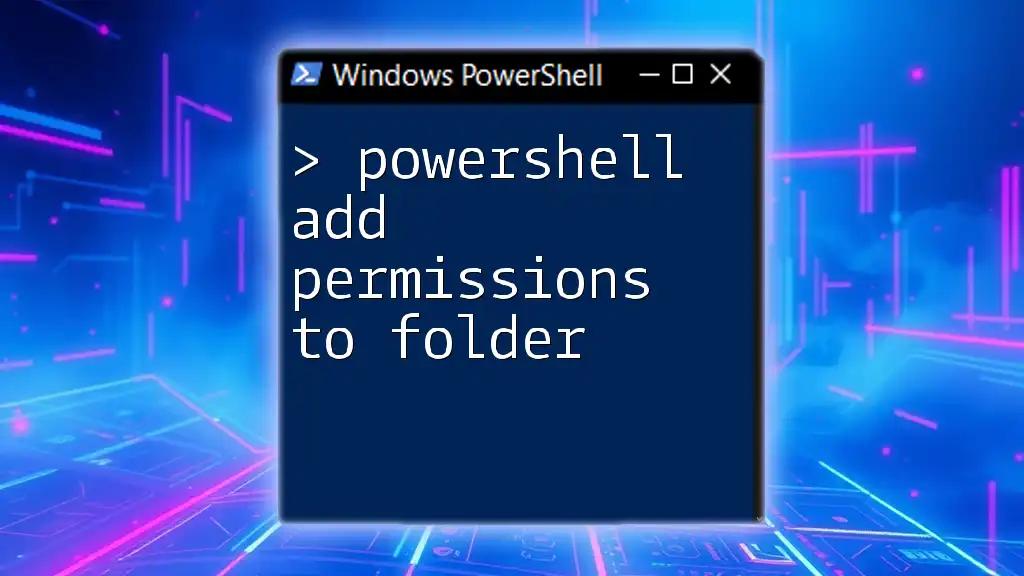
Using PowerShell to Retrieve Folder Permissions
Basic Command Structure
To retrieve permissions for a specific folder, you can utilize the `Get-Acl` cmdlet. The basic syntax is:
Get-Acl -Path "C:\Path\To\Your\Folder"
Example: Getting Permissions for a Single Folder
To illustrate, let’s retrieve the permissions for a folder named SampleFolder using the following code snippet:
$folderPath = "C:\SampleFolder"
$acl = Get-Acl -Path $folderPath
$acl | Format-List
This command will output the Access Control List (ACL) details for the specified folder. The output typically contains essential information such as the users/groups and their respective permissions. Observing these details allows for quick assessments of who can access or modify files within the folder.
Recursively Getting Permissions for Subfolders
Using a Loop to Retrieve Permissions
In many cases, you may need to check permissions for multiple subfolders. Here, using a loop can significantly streamline the process. Here's how you can leverage a loop:
$folderPath = "C:\SampleFolder"
Get-ChildItem -Path $folderPath -Recurse | ForEach-Object {
$subFolderAcl = Get-Acl -Path $_.FullName
Write-Output "$($_.FullName): $($subFolderAcl.Access)"
}
This code snippet will iterate through all items in SampleFolder, including subfolders, and output their permissions. Understanding how each line of code works is helpful: `Get-ChildItem` retrieves the items, and `Get-Acl` retrieves the permissions for each item.
Using `Get-ChildItem` with `Select-Object`
Alternatively, you can extract permissions using `Select-Object` for a more concise output:
Get-ChildItem -Path "C:\SampleFolder" -Recurse | Select-Object FullName, @{Name='Permissions';Expression={(Get-Acl $_.FullName).Access}}
This approach not only lists the full path of each folder but also summarizes the permissions in a clean format, making it easier to review at a glance.
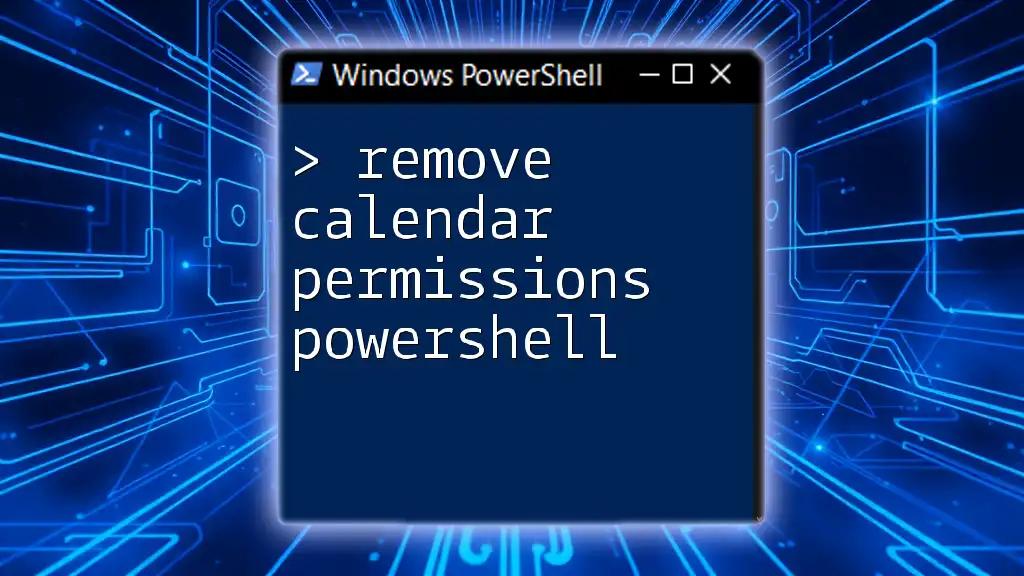
Understanding and Modifying Permissions
Interpreting the ACL Output
When you retrieve the ACL information, it’s crucial to comprehend the output. The primary property fields include:
- IdentityReference: Indicates the user or group that has permission.
- FileSystemRights: Displays the type of access granted.
- AccessControlType: Specifies whether the permission is allowed or denied.
For example, an entry like `DOMAIN\User Allow Read` indicates that the specified user can read the contents of the folder.
Modifying Permissions Using PowerShell
In addition to retrieving permissions, you might need to make changes. For instance, to add a permission such as granting read access, you would use the `Set-Acl` cmdlet. Here’s how to do that:
$acl = Get-Acl -Path "C:\SampleFolder"
$permission = "DOMAIN\User","Read","Allow"
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission
$acl.AddAccessRule($accessRule)
Set-Acl -Path "C:\SampleFolder" -AclObject $acl
This snippet first retrieves the current ACL for the folder, defines a new access rule, and finally applies the change. Always remember to validate changes by re-running a permission check after modification.

Best Practices for Managing Permissions
Regular Audits
Regularly auditing folder permissions is crucial for ensuring security. Performing periodic checks helps in identifying any unauthorized changes and ensuring compliance with data access policies. PowerShell scripts can make this auditing process efficient and automated.
Documenting Changes
When changing permissions, keeping detailed documentation of each change is vital. This practice not only helps in tracking who has access to sensitive data but also assists in resolving disputes over permissions. Consider creating a simple log that includes the date, user, changed permissions, and a brief reason for the change.
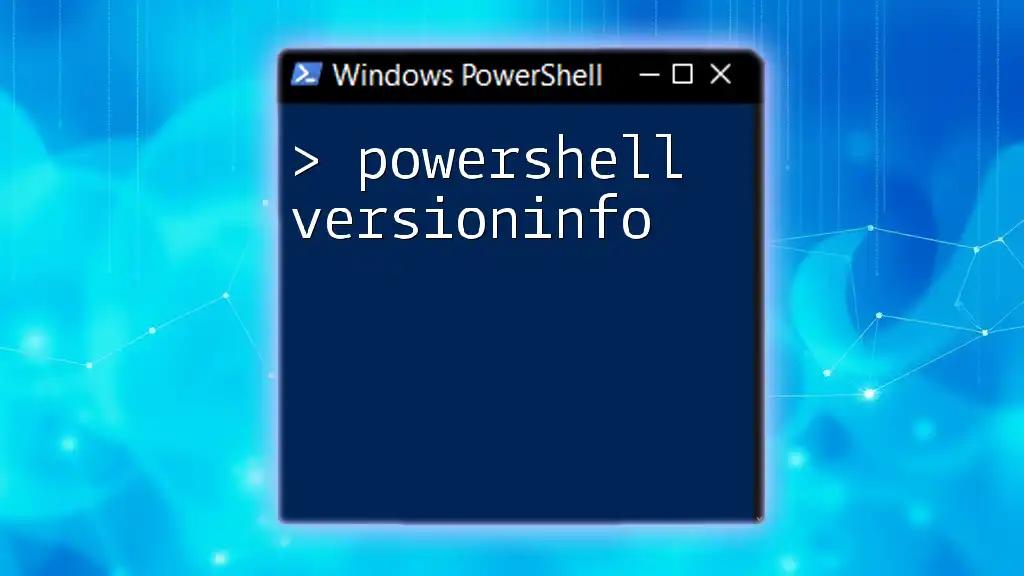
Conclusion
In summary, mastering the PowerShell commands for managing folder and subfolder permissions is an invaluable skill for any system administrator or IT professional. By understanding NTFS permissions, utilizing simple commands like `Get-Acl`, and modifying permissions securely, you can manage your file system's security effectively. Regular audits and documentation further enhance your security posture.
For those eager to dive deeper, continuous practice with PowerShell commands will lead to greater efficiency and proficiency in managing Windows environments. Be sure to explore additional resources and community forums to enhance your learning journey.