To move a partition to the end of the disk using PowerShell, you can use the `Move-Partition` cmdlet from the Storage module, as illustrated in the code snippet below:
Move-Partition -Partition $partition -Disk $disk -Offset $newOffset
Make sure to replace `$partition`, `$disk`, and `$newOffset` with your specific values for partition, disk, and the desired offset.
Understanding Disk Partitions
What is a Disk Partition?
A disk partition is a logical division of a hard disk drive, allowing multiple file systems to coexist on a single physical disk. Each partition can be formatted with its own file system and operated independently, making it an integral component in system organization and management.
Types of Disk Partitions
Primary Partitions are the main divisions on a disk and can hold an OS. Extended Partitions serve as a container for Logical Drives, while Logical Partitions are subdivisions of extended partitions, allowing for more flexible disk management. Understanding these types is crucial as you manipulate partitions, especially when using commands to move them.

Prerequisites to Move a Partition Using PowerShell
Required Permissions
To successfully move a partition, you must have Administrator Privileges. This is because modifying disk partitions can significantly affect system behavior. Always ensure that you run PowerShell as an Administrator to avoid access issues when executing commands.
PowerShell Version
It’s important to use a version of PowerShell that supports disk management cmdlets. Make sure you are using at least PowerShell 5.0 or later. You can easily check your PowerShell version with the command:
$PSVersionTable.PSVersion
Backup Important Data
Before diving into moving partitions, backing up important data is essential. Disk operations come with the risk of data loss, and a backup ensures that you do not lose critical information in case of errors.
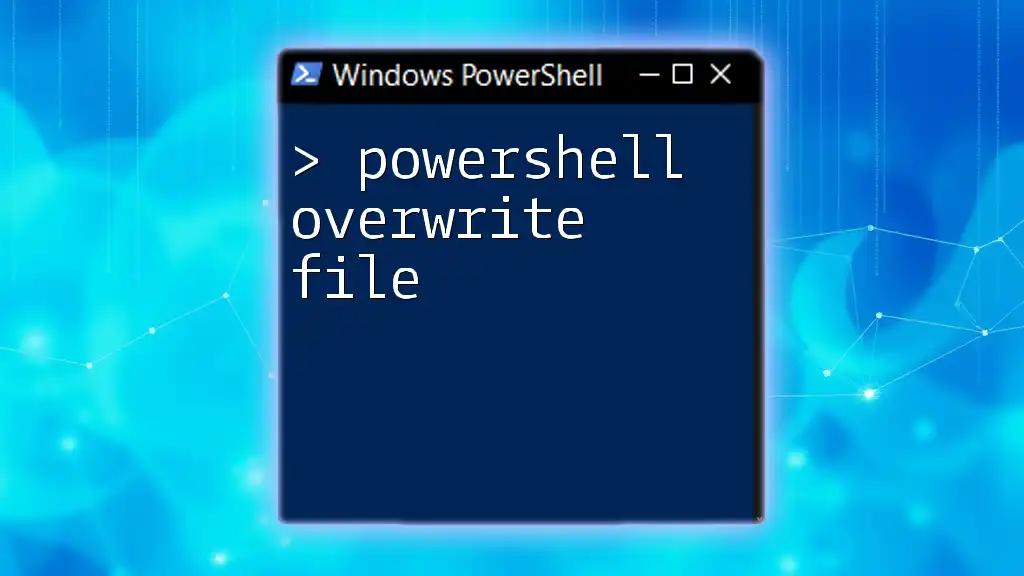
Tools and Cmdlets for Managing Disk Partitions
Using Disk Management Cmdlets
PowerShell provides several cmdlets specifically for managing disks and partitions. These cmdlets grant you the power to retrieve disk information and perform actions effectively.
Get-Disk
This cmdlet allows you to view a list of available disks on your system. It serves as the starting point for any disk management task.
Get-Disk
Get-Partition
Once you know the disk you want to manage, you can retrieve detailed information about the partitions on that disk.
Get-Partition -DiskNumber 1
This command lists all partitions on the specified disk. You can identify the partition you wish to move using this information.
Resize-Partition
Before moving a partition, it may be necessary to resize it to free up space. Use the `Resize-Partition` cmdlet for this purpose.
Resize-Partition -DiskNumber 1 -PartitionNumber 1 -Size 100GB
This command resizes the specified partition to 100GB. Always ensure you have enough unallocated space before proceeding to move a partition.
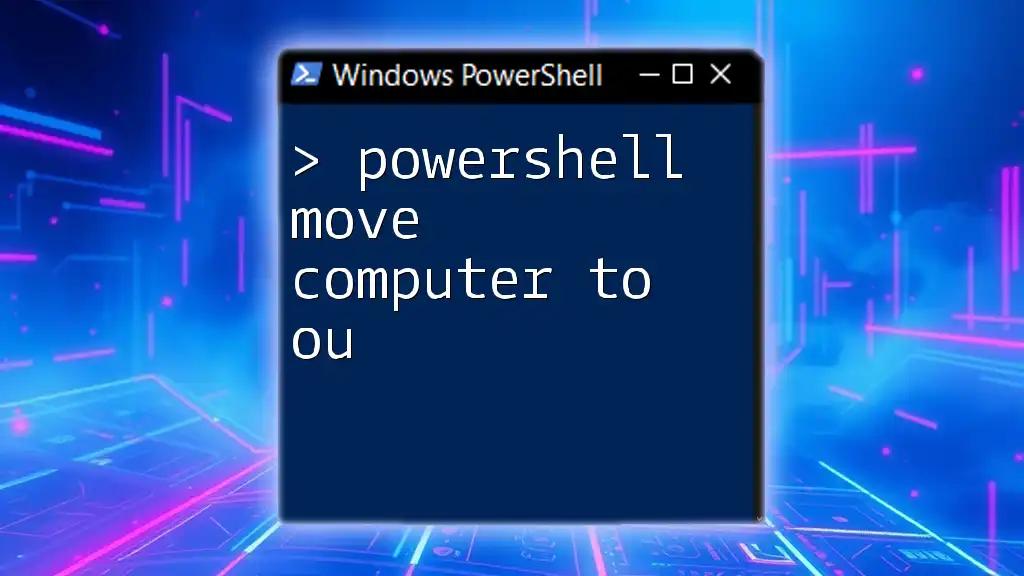
Steps to Move a Partition to the End of a Disk
Analyze Current Disk Layout
Before making any changes, it’s essential to analyze the current disk layout. The Windows Disk Management tool can be useful for visualizing partitions, helping you better understand how partitions are organized on your disk.
Move the Partition
Step-by-Step Process
-
Identify the Target Partition: Use the `Get-Partition` cmdlet to specify the partition you want to move. For example, if you want to move the partition with drive letter "D", you can assign it to a variable for easier reference.
$partition = Get-Partition -DiskNumber 1 | Where-Object { $_.DriveLetter -eq 'D' }
-
Check for Unallocated Space: Before moving the partition, ensure that sufficient unallocated space exists on the disk. You can check for unallocated space using:
Get-Partition -DiskNumber 1 | Where-Object { $_.IsUnallocated -eq $true }
If you don’t see any unallocated space, it may be necessary to resize or delete another partition.
-
Resize the Partition if Necessary: If needed, resize the partition to create unallocated space using the `Resize-Partition` cmdlet mentioned earlier.
-
Moving the Partition Using DiskPart: Since traditional PowerShell cmdlets do not directly support moving partitions, you can transition to `DiskPart`. DiskPart is a powerful command-line tool for disk partition management.
Open DiskPart in PowerShell:
diskpart
Then, follow these commands:
select disk 1 select partition 1 move partition 1 to end
These commands select the disk and partition you plan to move and execute the move operation. In this example, "1" represents the disk and partition numbers and may need to be modified depending on your specific setup.
Confirming the Move
After you have moved the partition, it is crucial to confirm the operation’s success. Use the following command to check the current partitions on your disk.
Get-Partition -DiskNumber 1
This command will display the updated partition layout, allowing you to verify that the partition has been successfully moved to the end of the disk.
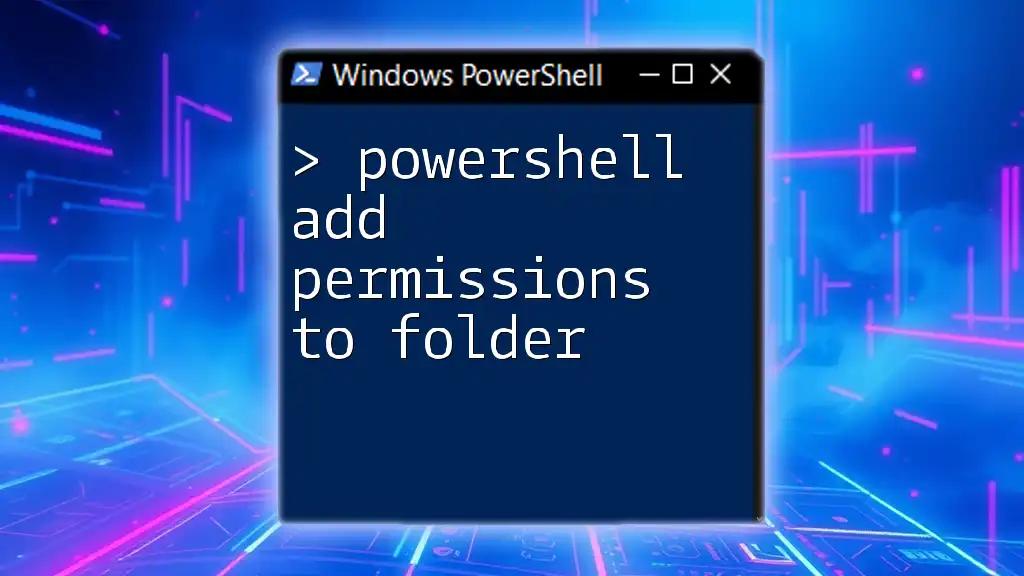
Troubleshooting Common Issues
Permissions Issues
If you encounter access denied errors, ensure that you are running PowerShell with administrative privileges. You cannot perform disk operations without adequate permissions.
Disk in Use
If you find the partition is locked or in use, make sure to safely unmount or dismount the volume before proceeding. If necessary, you can use the `Dismount-Partition` cmdlet or manage the partition via Disk Management to free it up for the move.

Conclusion
Moving partitions using PowerShell is a powerful technique that can optimize your disk layout for better performance and organizational needs. By understanding the commands and steps involved, you can efficiently manage and modify your disk partitions.
Call to Action
Explore further resources and practice your skills with PowerShell commands. Familiarize yourself with the syntax and the tools available to you as you delve deeper into disk management and automation.

Additional Resources
For further information, refer to the official Microsoft documentation on PowerShell disk management. You can also explore additional guides and tutorials to refine your disk management techniques.