PowerShell automation and scripting for cybersecurity empowers professionals to streamline security tasks, enhance incident response, and automate routine processes to protect systems effectively.
Here’s a simple code snippet that demonstrates how to scan for open ports on a target machine:
$target = "192.168.1.1"
$ports = 1..1024
foreach ($port in $ports) {
$connection = Test-NetConnection -ComputerName $target -Port $port
if ($connection.TcpTestSucceeded) {
Write-Host "Port $port is open."
}
}
Understanding PowerShell in Cybersecurity
What is PowerShell?
PowerShell is a powerful scripting language and shell designed primarily for system administration and automation. It allows users to execute commands, manipulate objects, and manage configurations in a variety of software environments. Key features include:
- Cmdlets: Specialized .NET classes built within PowerShell to perform specific tasks. They have a consistent naming convention, making them easier to learn and use.
- Scripts: Collections of cmdlets and functions that can be executed in batch to automate workflows.
- Modules: Packaged scripts and cmdlets that extend PowerShell’s functionality, allowing seamless integration with other tools.
Why Use PowerShell for Cybersecurity?
PowerShell has become an essential tool in the cybersecurity domain due to its versatility and effectiveness. Some notable advantages include:
- Efficiency and Speed: Many routine cybersecurity tasks can be completed much quicker using automated scripts rather than through manual intervention. This time-saving aspect is critical when responding to security threats.
- Integration Capabilities: PowerShell can work alongside a variety of security products and tools, making it a natural fit for integrating and managing security environments.
- Customization: Users can create tailored scripts to address specific security challenges, providing greater control over security processes.
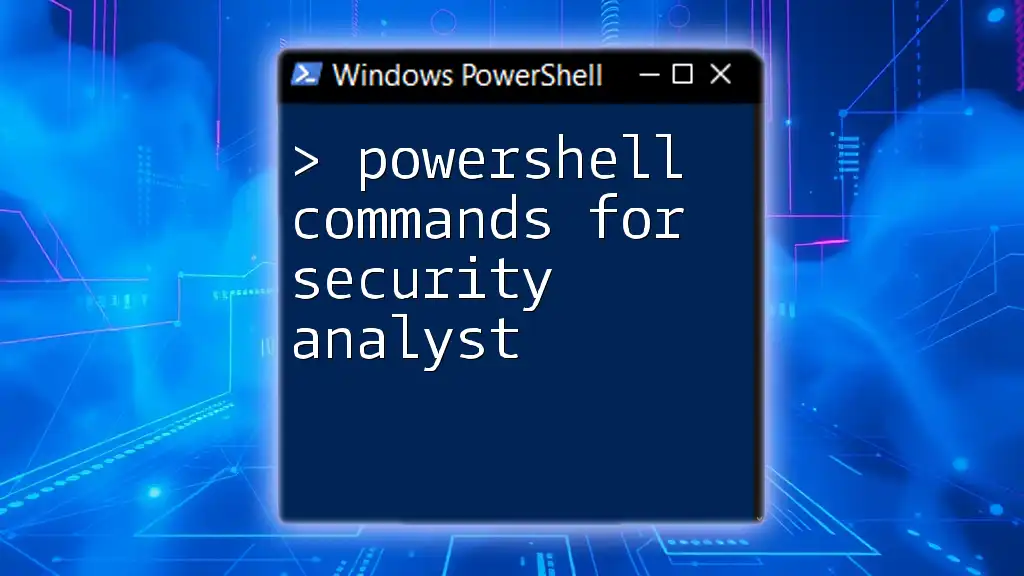
Getting Started with PowerShell
Setting Up the PowerShell Environment
To start utilizing PowerShell for cybersecurity tasks, you need to ensure that PowerShell is properly installed and configured on your machine. Installation varies by platform:
- Windows: PowerShell typically comes pre-installed. Update to the latest version of PowerShell Core for cross-platform accessibility.
- Linux and macOS: You can download and install PowerShell Core from the official GitHub repository.
Familiarizing yourself with the PowerShell Integrated Scripting Environment (ISE) or using Visual Studio Code can improve your scripting experience. Both environments offer syntax highlighting and debugging support.
Fundamental PowerShell Commands for Cybersecurity
To effectively conduct cybersecurity operations, you should be comfortable with several key PowerShell commands. Here are a few essential cmdlets:
-
`Get-Process`: Use this command to monitor running processes on a system. Example usage:
Get-Process
-
`Get-Service`: This command checks the operational status of services on your machine. Example:
Get-Service
-
`Get-EventLog`: Vital for analyzing security logs, allowing you to review events, warnings, and errors. Example:
Get-EventLog -LogName Security
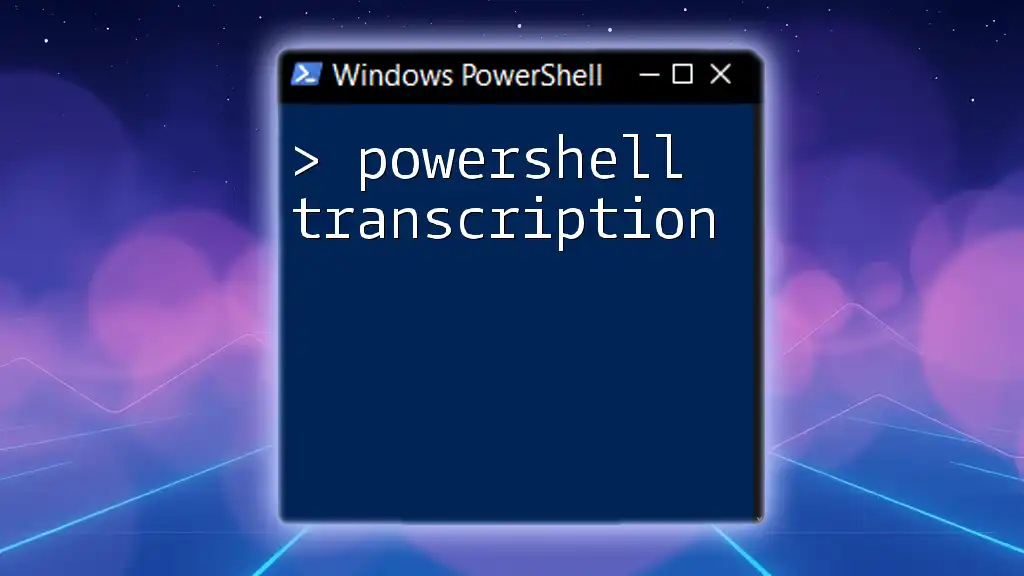
PowerShell Scripting Basics
Writing Your First Script
Creating scripts in PowerShell can streamline cybersecurity tasks. Here is a simple script to check system uptime:
$uptime = (Get-Date) - (Get-CimInstance Win32_OperatingSystem).LastBootUpTime
Write-Output "System Uptime: $uptime"
This script retrieves the current date and subtracts the last boot-up time of the operating system, giving you the total system uptime.
Error Handling in PowerShell Scripts
When creating scripts, error handling is crucial for stability and troubleshooting. Effective scripts anticipate possible errors and handle them gracefully. Using the Try-Catch block is a standard approach:
Try {
Get-Process -Name "NotExist"
} Catch {
Write-Output "Error: $_"
}
This script attempts to retrieve a process by a non-existing name. If it fails, it will capture the error and display a user-friendly message.
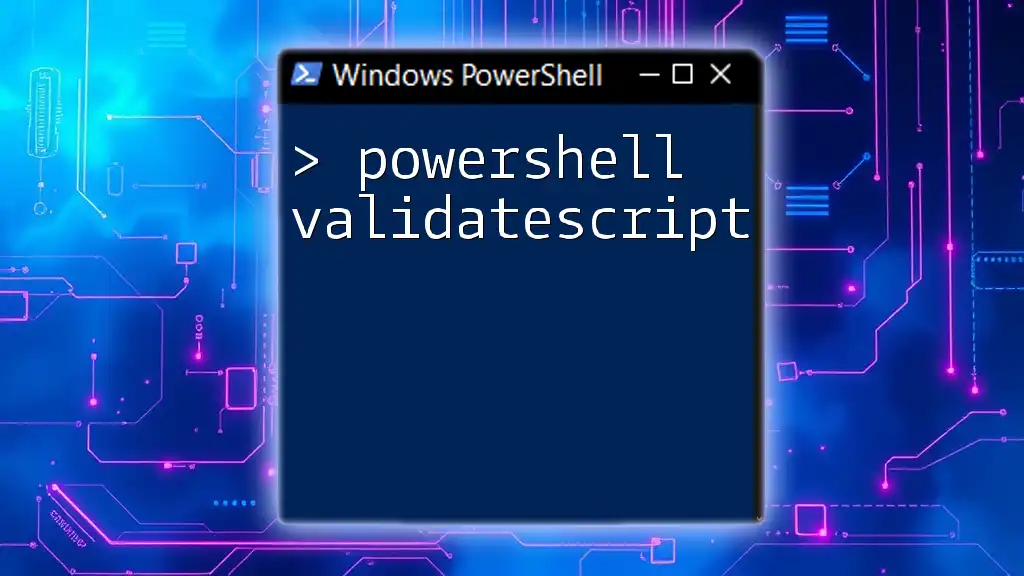
Automating Cybersecurity Tasks with PowerShell
Automating User Account Management
PowerShell allows you to automate the management of user accounts efficiently, streamlining operations such as user creation, modification, or removal. Here’s how to create a new user account:
New-LocalUser -Name "NewUser" -Password (ConvertTo-SecureString "Password123" -AsPlainText -Force) -FullName "New User" -Description "A new user account"
This command creates a new local user with a specified password, full name, and description.
Automating Log Analysis
Analyzing security logs is crucial for identifying potential threats. PowerShell can help retrieve and filter logs effectively. Here is a script example to find failed login attempts:
Get-EventLog -LogName Security | Where-Object { $_.EventID -eq 4625 }
This command searches through the Security event log for events with an Event ID of 4625, which indicates a failed login attempt.
Threat Detection Automation
PowerShell scripts can also be utilized for real-time threat detection. Consider the following example, which scans running processes for known malware:
$badProcesses = @("malware1", "malware2")
Get-Process | Where-Object { $badProcesses -contains $_.Name }
This script checks all running processes against a predefined list of known malicious processes and returns any matches.
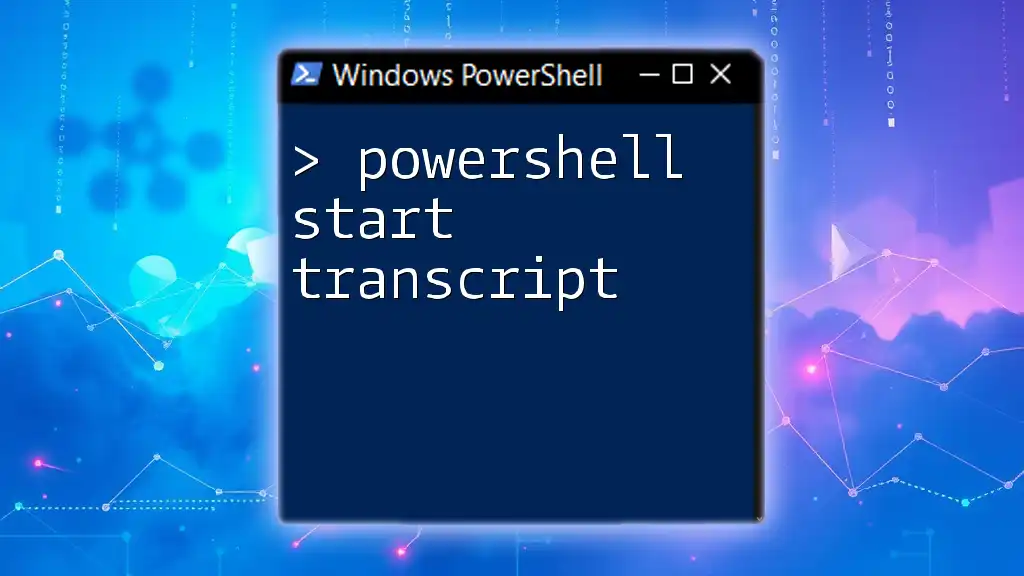
Advanced PowerShell Techniques for Cybersecurity
Integrating PowerShell with Other Security Tools
PowerShell shines when integrated with other cybersecurity tools. Utilizing PowerShell Remoting enables you to manage multiple machines from a single command line, making it easier to enforce security policies network-wide.
Additionally, PowerShell can interface with Security Information and Event Management (SIEM) tools like Splunk or AlienVault, enabling comprehensive log collection and analysis.
Creating Custom PowerShell Modules
For more complex automation tasks, consider creating custom PowerShell modules. This allows you to group related functions and scripts, providing a modular approach to cybersecurity scripting. Developing a custom module can increase code reusability and ease of maintenance.
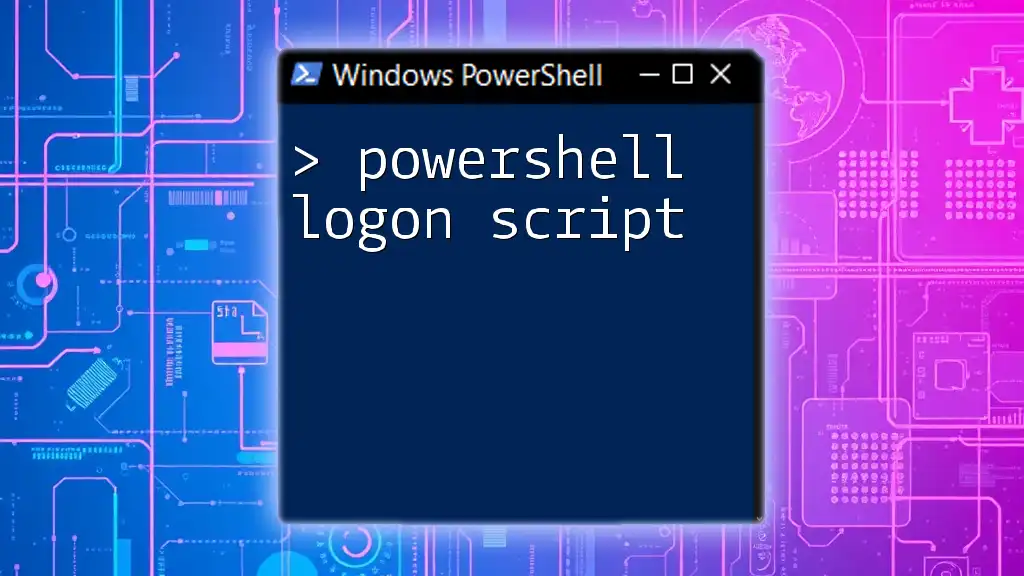
Best Practices for PowerShell in Cybersecurity
Security Considerations
While PowerShell is a powerful tool, it can also be a target for attackers if not secured properly. Always run scripts with least-privilege rights to minimize potential damage in case of a compromised script. Additionally, validate and sanitize user inputs within your scripts to prevent injection attacks.
Documentation and Maintenance
Documentation is a key aspect of any scripting effort. Create comprehensive documentation for your scripts detailing their purpose, input/output expectations, and any dependencies. Routine maintenance of your scripts—ensuring they align with the latest security practices and technologies—is equally essential.
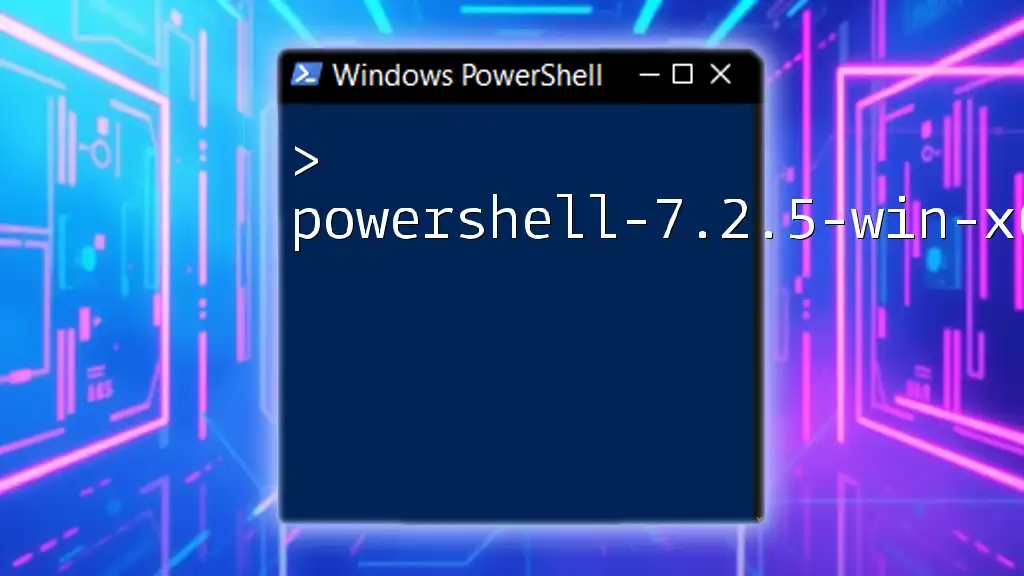
Conclusion
PowerShell automation and scripting for cybersecurity can significantly enhance your ability to manage security tasks efficiently and effectively. Embracing these techniques allows for quicker responses to threats and a more robust security posture. The more you practice and experiment with PowerShell, the better equipped you will be in navigating the ever-evolving landscape of cybersecurity threats. To expand your knowledge further, consider exploring recommended online courses, forums, and blogs dedicated to PowerShell and cybersecurity.