To retrieve the directory of a PowerShell script, you can use the built-in automatic variable `$PSScriptRoot`, which provides the full path of the folder containing the script.
# Display the directory of the script
$directory = $PSScriptRoot
Write-Host "The script is located in: $directory"
Understanding Script Context in PowerShell
What is a PowerShell Script?
PowerShell scripts are text files that contain a series of PowerShell commands, which can automate tasks and processes within Windows environments. These scripts are saved with the `.ps1` file extension and can be executed directly in a PowerShell console or scheduled to run at specific times.
The execution context of a PowerShell script refers to the environment in which it's running, including variables, functions, and the scope of execution. This context is essential for understanding how to access script-specific information, such as the script's directory.
Why Knowing Your Script Directory Matters
Grasping how to retrieve the script directory enhances reliability and flexibility in your automation tasks. For instance, scripts often rely on configuration files, logs, or other resources that may reside in the same directory as the script itself. By accessing the script's path, you can ensure that your script can dynamically locate these resources, which facilitates portability and scalability.
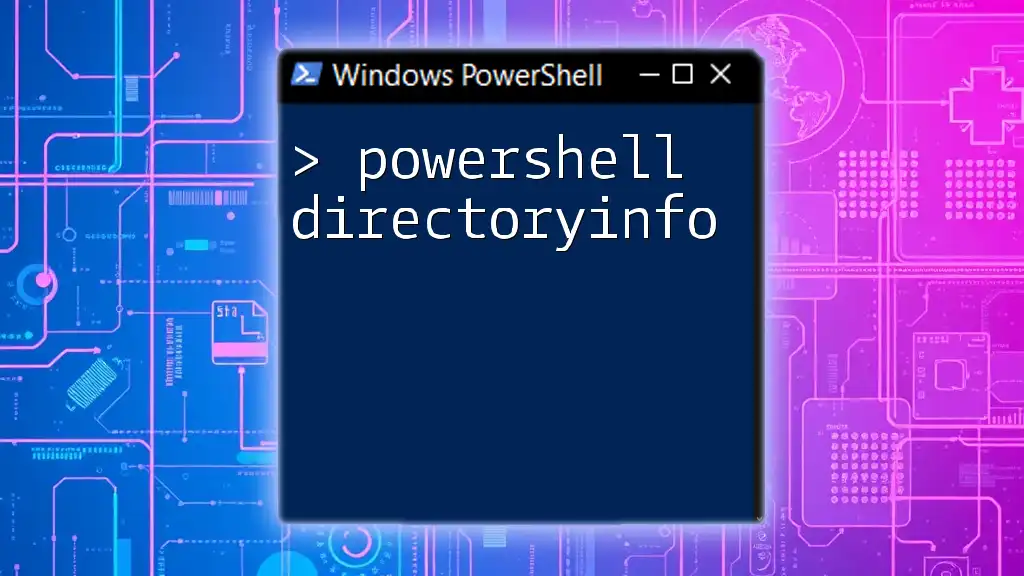
How to Get the Directory of the Current Script
Using the `$PSScriptRoot` Automatic Variable
The `$PSScriptRoot` variable is a built-in automatic variable in PowerShell that provides the directory path of the script being executed. This variable is available within script files but not in the interactive command line.
To use `$PSScriptRoot`, simply assign it to a variable:
$scriptDirectory = $PSScriptRoot
Write-Host "The directory of the script is: $scriptDirectory"
In the example above, `$scriptDirectory` will contain the path to the directory where the script resides. This method is particularly valuable for scripts that may be launched from different working directories, as it ensures the script can still locate its resources reliably.
Using the `Get-Location` Cmdlet
Another method to get the current script path involves using the `Get-Location` cmdlet in conjunction with `$MyInvocation`. The `$MyInvocation` variable reflects the details of the current command being executed, including the path of the script.
To retrieve the script path this way, you can use the following code:
$scriptPath = $MyInvocation.MyCommand.Path
$scriptDirectory = Split-Path $scriptPath
Write-Host "The directory of the current script is: $scriptDirectory"
With this approach, `$scriptPath` holds the full path of the script, and `Split-Path` extracts just the directory portion. This method works even if you need to retrieve the path from a command line script, giving it a slightly broader applicability than `$PSScriptRoot`.
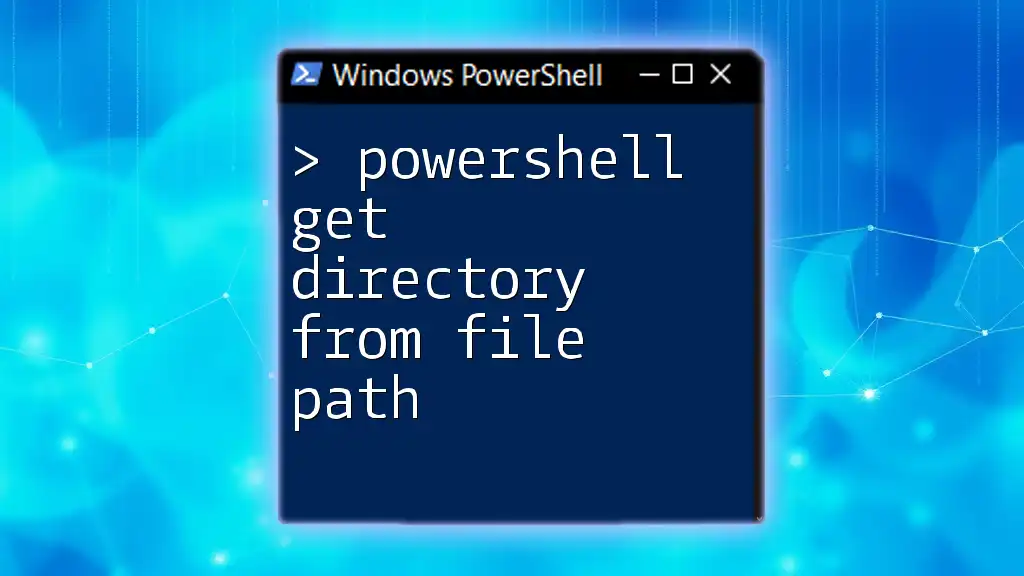
Advanced Techniques for Getting the Script Directory
Handling Script Arguments
When scripts are executed with arguments, you might want to combine the script directory with the arguments passed. This allows you to generate file paths based on user input or script parameters. Here’s a simple example that demonstrates how to join the script directory with an additional path:
param (
[string]$additionalPath
)
$fullPath = Join-Path -Path $PSScriptRoot -ChildPath $additionalPath
Write-Host "The full path including additional path is: $fullPath"
In this snippet, `Join-Path` combines the script directory with whatever additional path is provided by the user, creating a complete file path.
Combining with Other Cmdlets for Enhanced Functionality
You can also utilize the `Get-ChildItem` cmdlet to retrieve a list of files or directories within the current script directory. This is particularly useful when you need to manage or manipulate files that are located alongside your script.
For example:
Get-ChildItem -Path $PSScriptRoot
This command will list all items in the script's folder, making it easier to work with local files.
Creating Reusable Functions
Creating a reusable function to obtain the script directory can save time and keep your code DRY (Don't Repeat Yourself). Here’s an example of how to define such a function:
function Get-ScriptDirectory {
return $PSScriptRoot
}
By calling `Get-ScriptDirectory`, you can consistently retrieve the script path without repeating code across multiple scripts. This approach encourages modularity and maintainability.
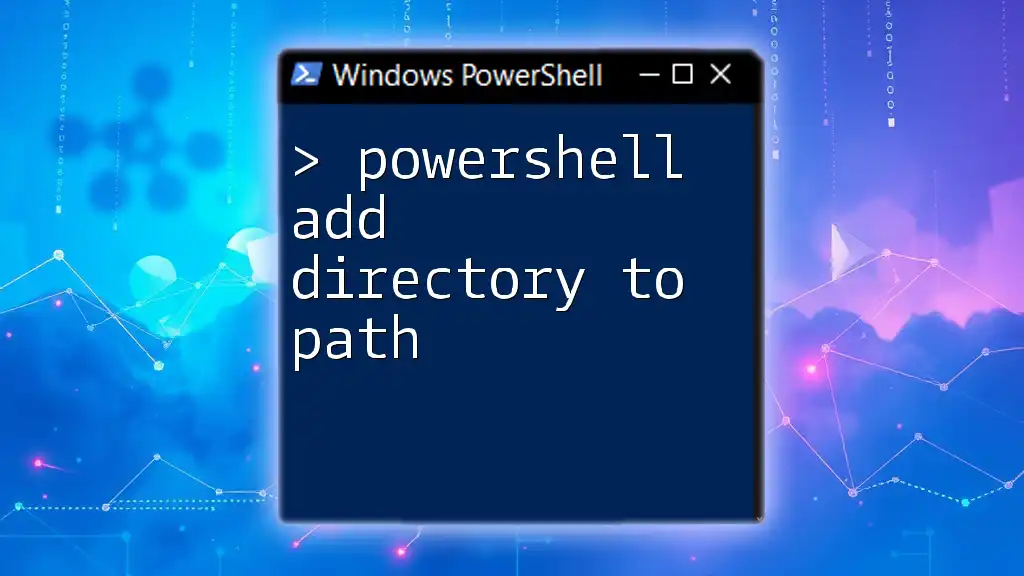
Common Pitfalls to Avoid
Incorrect Context Usage
One of the most common mistakes is attempting to use `$PSScriptRoot` in an interactive prompt or in a script context where it’s not applicable. This variable will return an empty value if the script is executed in a context where it’s not defined. Understanding the execution context is fundamental to avoid errors.
Working with UNC Paths
If your script is being run from a network path (UNC path), be cautious as it can lead to unexpected behavior. Always verify the paths collected using `$PSScriptRoot` or `$MyInvocation` when your scripts are executed in various environments, whether they are local or on a network share.
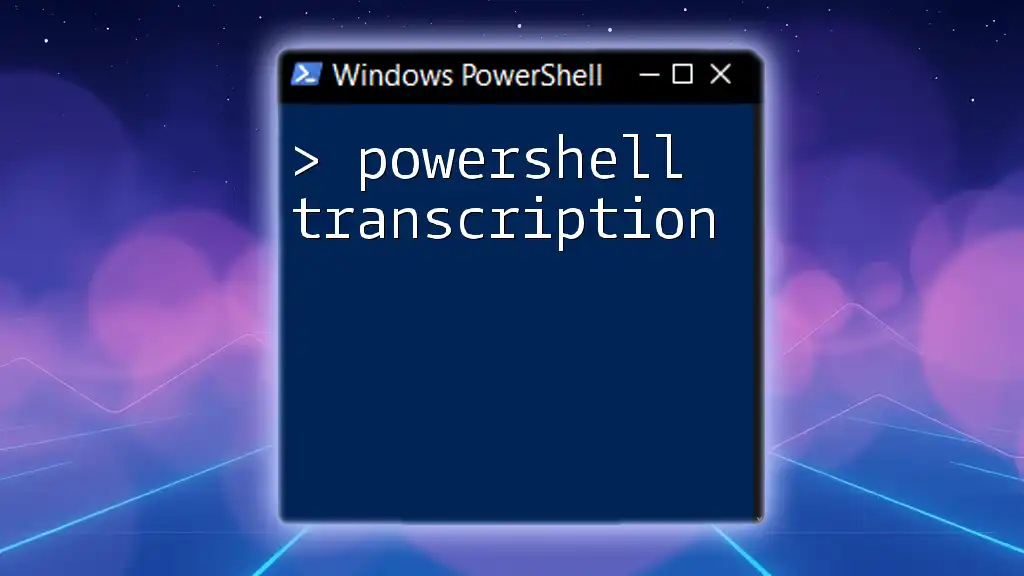
Conclusion
Mastering how to get the directory of the script in PowerShell is essential for writing robust and portable scripts. Utilizing automatic variables and cmdlets effectively allows you to navigate script directories seamlessly, ensuring your scripts can reliably access necessary resources.
By exploring the various methods discussed—such as `$PSScriptRoot`, `$MyInvocation`, and creating reusable functions—you can enhance your scripting capabilities and efficiency. Don't hesitate to try these techniques in your PowerShell projects!
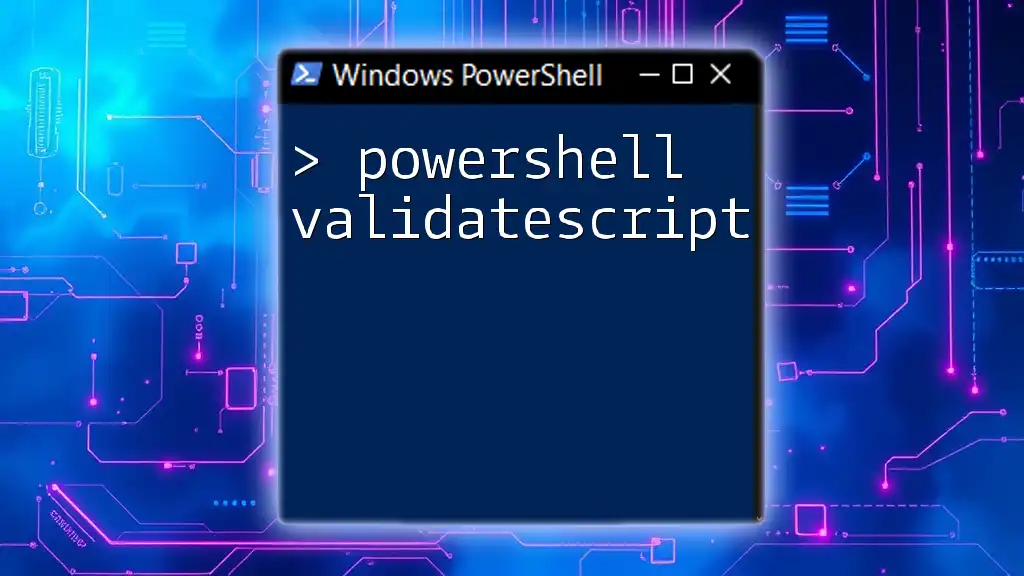
Additional Resources
For further reading, consider exploring the Microsoft documentation for PowerShell. Understanding the underlying principles and best practices will bolster your scripting skills and improve your overall proficiency in PowerShell.