In PowerShell, you can extract the directory from a file path using the `Split-Path` cmdlet. Here's a code snippet to demonstrate this:
$directory = Split-Path "C:\Example\MyFile.txt"
Write-Host $directory
Understanding File Paths
What is a File Path?
A file path is a string that specifies the location of a file or directory in a file system. Understanding file paths is fundamental to manipulating files in any programming or scripting language, including PowerShell.
File paths can be categorized into two types:
- Absolute Paths: These paths specify the complete directory hierarchy from the root to the file. For example, `C:\Users\Username\Documents\File.txt`.
- Relative Paths: Unlike absolute paths, relative paths are based on the current directory. For example, if your current directory is `C:\Users\Username`, the relative path `Documents\File.txt` refers to the same file as the absolute path.
Each part of a file path has specific components:
- Drive Letter: The storage device (e.g., C:).
- Directories: The folders leading to the file.
- File Name and Extension: The name of the file and its type (e.g., File.txt).
Why You Need to Extract Directories
Extracting directories from file paths is crucial in various scenarios within scripting and automation. For instance, it allows you to:
- Organize files by moving them into designated folders.
- Create backup scripts that require specific destination directories.
- Modify file names while keeping track of their locations.
PowerShell excels in these tasks, making the process efficient and straightforward.
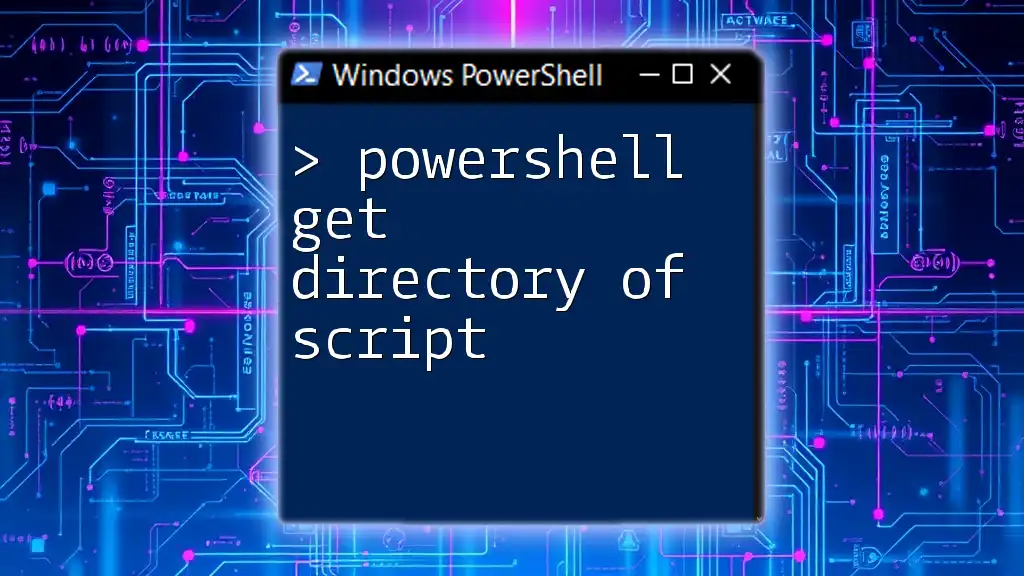
Getting Started with PowerShell
Setting Up PowerShell
Whether you are using Windows PowerShell or PowerShell Core (cross-platform), launching PowerShell is simple. You can open it by searching for "PowerShell" in your applications or command line. Familiarize yourself with PowerShell versions and their features; using the latest version is preferable for accessing new cmdlets and functionalities.
When starting out, the Integrated Scripting Environment (ISE) can be beneficial. It provides a user-friendly interface where you can write, test, and debug your scripts in an interactive environment.
Basic Syntax and Commands
In PowerShell, commands are referred to as cmdlets. Each cmdlet follows a specific syntax format, typically structured as `Verb-Noun`. For example, `Get-Process` retrieves a list of running processes.
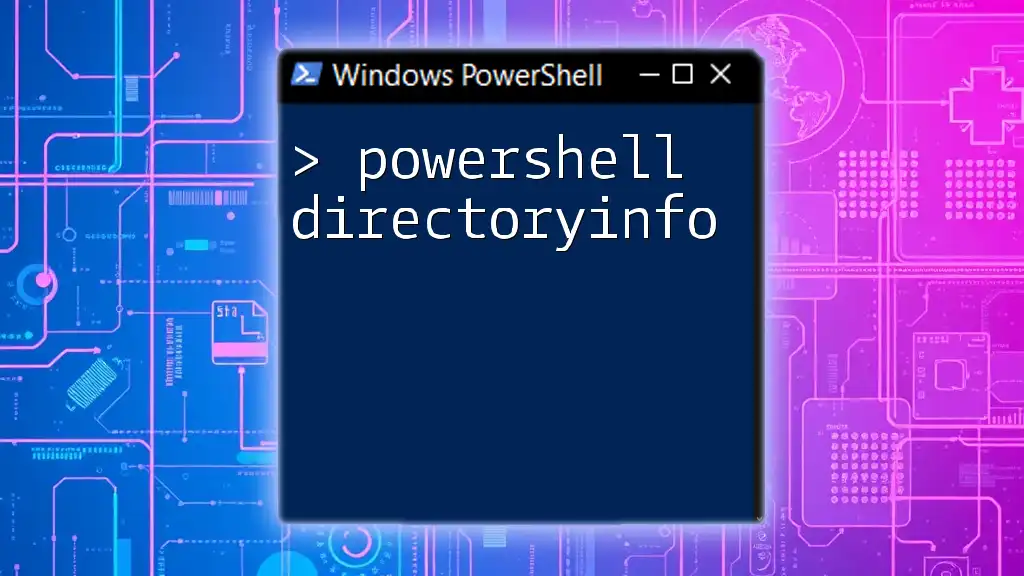
Extracting Directory from File Path
Using the Split-Path Cmdlet
Overview of Split-Path
The Split-Path cmdlet is a powerful tool for parsing file paths. It helps in extracting components of a path without needing complex string manipulations.
Basic Usage of Split-Path
Using `Split-Path` is straightforward. You can retrieve the directory from a full file path easily.
Example:
$path = 'C:\Folder\File.txt'
$directory = Split-Path -Path $path
Write-Output $directory # Output: C:\Folder
In this example, `Split-Path` processes the `$path` variable and returns the directory, which is `C:\Folder`.
Advanced Usage of Split-Path
Extracting Other Elements
The `Split-Path` cmdlet isn't limited to just directories; it can also retrieve other elements of the file path. For instance, you can get the file name or the parent directory.
To get the filename:
$fileName = Split-Path -Path $path -Leaf
Write-Output $fileName # Output: File.txt
To get the parent directory:
$parentDirectory = Split-Path -Path $path -Parent
Write-Output $parentDirectory # Output: C:\Folder
Working with Relative Paths
When working with relative paths, `Split-Path` is equally effective. You can combine relative paths with the current location to derive the absolute path.
Example:
$relativePath = '.\Folder\File.txt'
$fullPath = Split-Path -Path (Get-Location) -ChildPath $relativePath
Write-Output $fullPath
This snippet retrieves the absolute path of the file by combining the current directory with the relative path.
Combining Split-Path with Other Cmdlets
To further enhance its usefulness, `Split-Path` can be combined with other cmdlets, such as `Get-ChildItem`, which retrieves a list of files and directories.
Example:
$files = Get-ChildItem -Path 'C:\Folder'
foreach ($file in $files) {
$directory = Split-Path -Path $file.FullName
Write-Output $directory
}
In this example, the script iterates through all files in `C:\Folder` and outputs their corresponding directories, demonstrating practical utilization.
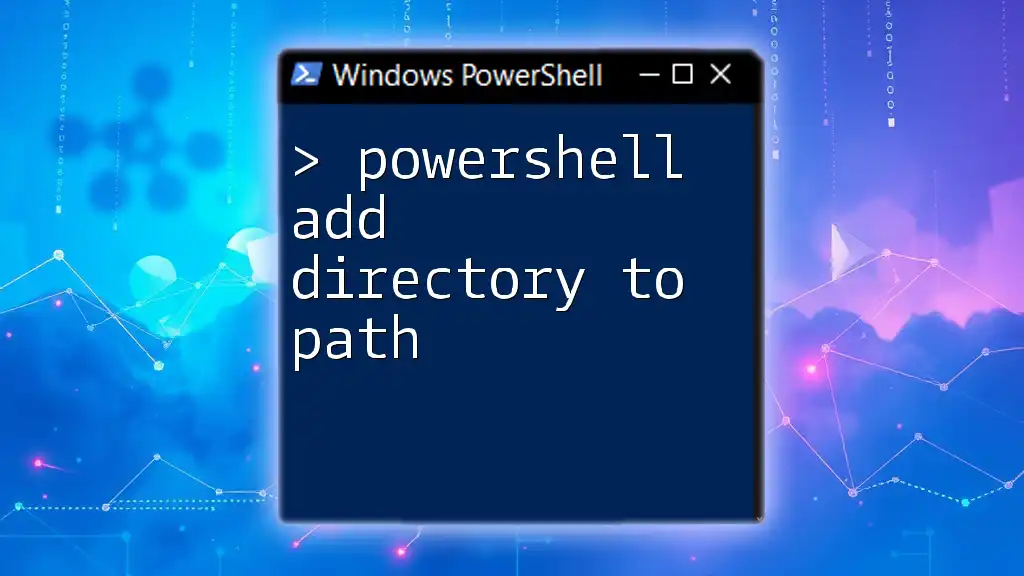
Practical Applications
Automating Directory Management
Understanding how to extract directories can significantly automate tasks like file organization, cleanup, and reporting. For instance, you could write scripts that check for specific file types in a directory and move or delete them based on your set criteria.
Writing Your Own Functions
Creating custom functions in PowerShell allows you to encapsulate logic and reuse it throughout your scripts. Here is a simple function that takes a file path as input and returns the directory:
function Get-DirectoryFromPath {
param (
[string]$FilePath
)
return Split-Path -Path $FilePath
}
This function can be invoked whenever you need to extract the directory from a file path, enhancing your scripting efficiency.
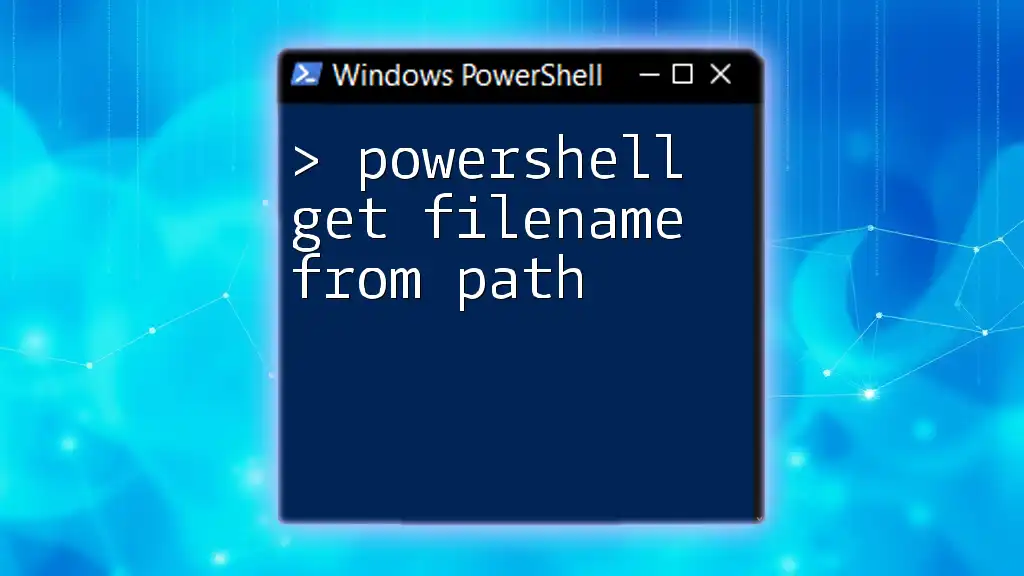
Conclusion
In summary, mastering how to use PowerShell to get the directory from a file path using the `Split-Path` cmdlet is both straightforward and powerful. From managing files to automating tasks, this knowledge serves as a foundation for more advanced PowerShell techniques.
As you delve deeper into PowerShell, you'll find that this skill can significantly enhance your productivity, whether in system administration or personal projects.
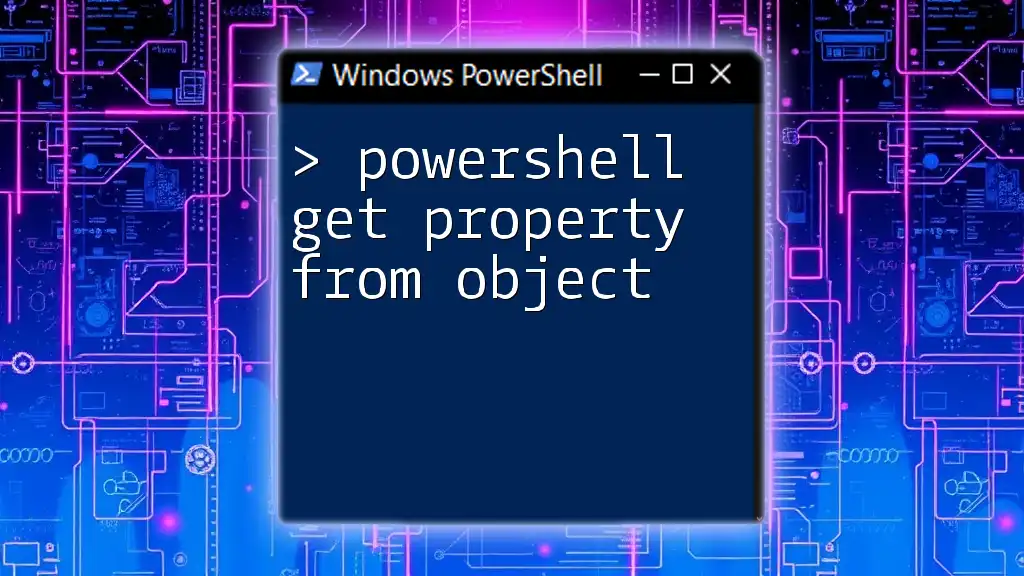
Additional Resources
Further Reading
To enhance your learning, consider reading the official PowerShell documentation and delving into community blogs that focus on advanced scripting techniques.
Tools for PowerShell Users
Explore tools and extensions like Visual Studio Code with PowerShell support or dedicated PowerShell learning platforms that provide lessons and practical exercises to foster skill development.