In PowerShell, you can retrieve a specific property from an object using the dot notation to access the property directly.
# Example: Retrieve the 'Name' property from an object
$object = New-Object PSObject -Property @{ Name = 'John Doe'; Age = 30 }
$object.Name
Understanding Objects in PowerShell
What is an Object?
In programming, an object is a collection of properties and methods that define its characteristics and behaviors. In PowerShell, everything is treated as an object. When you run a command, the output is often an object that contains data you can manipulate.
The key components of an object in PowerShell are its properties and methods. Properties are attributes of the object, such as its size or status, while methods are actions that the object can perform, like starting or stopping a process.
Common Object Types in PowerShell
PowerShell can handle various types of objects, including those representing files, processes, services, and even user accounts. For example, when you get information about running processes, PowerShell returns process objects. You can create a test object for demonstration:
$person = New-Object PSObject -Property @{ Name = "John Doe"; Age = 30; Occupation = "Developer" }
In this example, `$person` is an object with properties such as `Name`, `Age`, and `Occupation`.

Getting Started with `Get-Member`
Introduction to Get-Member
To work effectively with objects, it's crucial to understand their structure. The `Get-Member` cmdlet allows you to inspect the properties and methods of an object, providing insights into what you can do with it.
Using Get-Member to Explore Properties
You can use `Get-Member` to list the properties of an object. Here’s a code snippet for retrieving properties of a running PowerShell process:
$process = Get-Process -Name "powershell"
$process | Get-Member -MemberType Property
This command outputs a list of properties associated with the PowerShell process, helping you understand what data you can access.
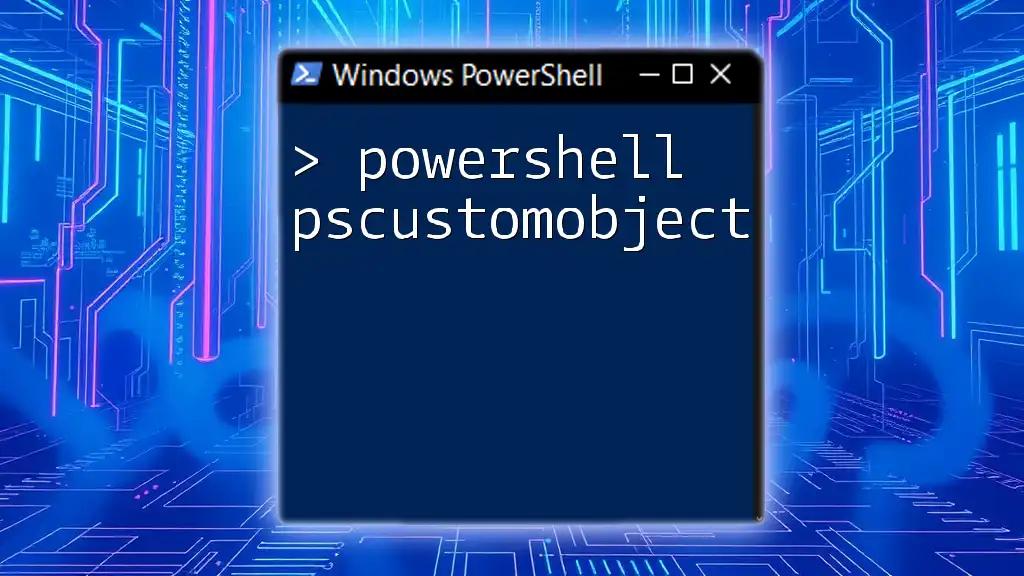
PowerShell Get Object Property
Using Dot Notation
One of the easiest ways to access a property of an object is by using dot notation. This means directly referencing the property after the object variable. For example, if you want to get the ID of the PowerShell process:
$process = Get-Process -Name "powershell"
$process.Id
Here, `$process.Id` fetches the ID of the specified process, providing a quick way to access that specific piece of data.
Using Select-Object
Introduction to Select-Object
The `Select-Object` cmdlet is essential for selecting properties from objects, allowing you to tailor the output to specific needs.
Selecting Specific Properties
If you're only interested in certain properties, you can use `Select-Object`. For example, if you want to see the `Id` and `Name` of the PowerShell process, you can do:
$process | Select-Object Id, Name
This command provides a focused view of only the details you're interested in, which is extremely useful when dealing with large datasets.
Filtering Values with Where-Object
You can also filter objects based on property values using `Where-Object`. This is useful when you want to hone in on specific results. For example, to find processes consuming more than 100 seconds of CPU time:
Get-Process | Where-Object { $_.CPU -gt 100 } | Select-Object Id, Name, CPU
This command filters the running processes to return only those with a CPU time greater than 100, displaying their `Id`, `Name`, and `CPU`.

PowerShell Get Object Properties
Retrieving All Properties
If you're unsure which properties an object has, you can retrieve all of them at once. By using `Get-Member`, you can list every property the object contains:
$process | Get-Member -MemberType Property | Select-Object Name
This approach is beneficial when you’re exploring unknown objects and want to understand their structure comprehensively.
Using `Format-Table` for Display
To enhance readability, you might want to format the output using `Format-Table`. For instance, you can combine it with `Select-Object` to create a structured display:
Get-Process | Select-Object Id, Name | Format-Table
This makes the output visually appealing and easier to read, especially useful for reports or logs.
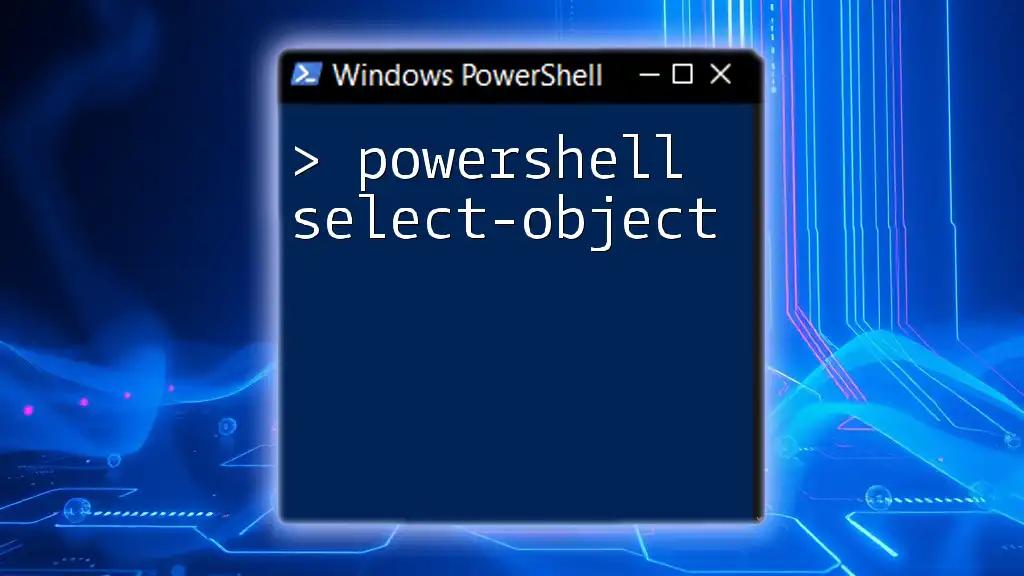
PowerShell Select-Object Value Only
Limiting Output to Value Only
When you need only the values from an object's property, PowerShell allows you to extract just the essential data. For instance, using the `-ExpandProperty` parameter with `Select-Object` can simplify your results:
$names = Get-Process | Select-Object -ExpandProperty Name
This command retrieves a list of process names without additional property information, creating a cleaner output that is easier to work with.
Use Cases for Value-Only Selections
Extracting values only is particularly helpful in scripting and automation tasks. If you feed a list of names into a notification system or a reporting function, having a clean array of values simplifies the process and reduces complexity.
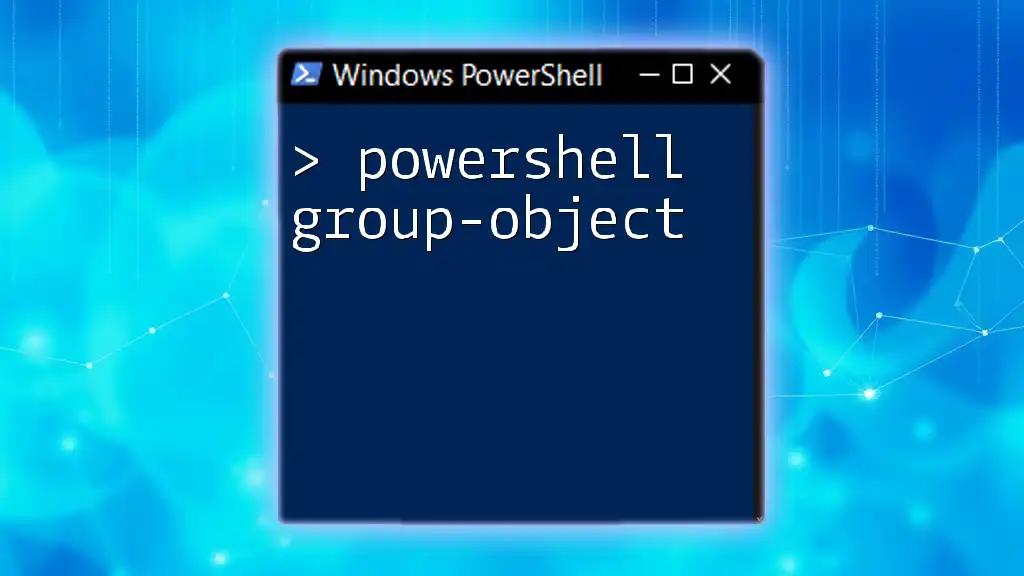
Conclusion
Understanding how to use PowerShell get property from object effectively allows you to harness the true power of PowerShell. By mastering commands like `Get-Member`, `Select-Object`, and `Where-Object`, you gain the ability to manage, filter, and utilize data from objects in a streamlined manner.
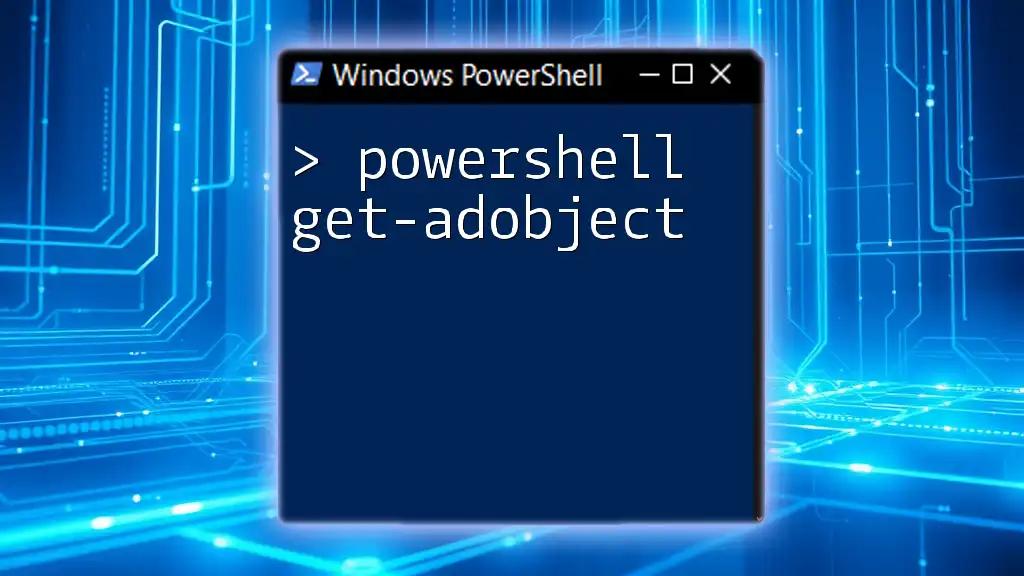
Call to Action
Enhance your skills with PowerShell by practicing with the examples provided, and stay tuned for more tips and tricks to elevate your PowerShell expertise. Join our community to discuss further and share your experiences with PowerShell commands!
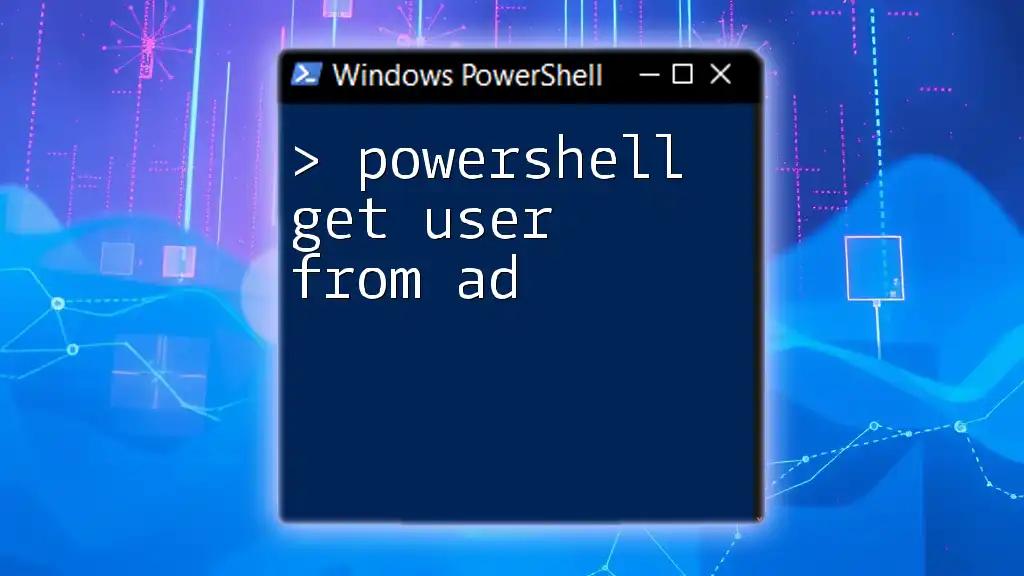
Resources
For further reading, please refer to the official PowerShell documentation for comprehensive guides and deeper insights into object management. Recommended resources include online courses and books that cover PowerShell scripting and automation in detail.