In PowerShell, you can add a property to an object using the `Add-Member` cmdlet to extend the object's functionality dynamically.
Here's a code snippet demonstrating how to do this:
$myObject = New-Object PSObject -Property @{Name='John'; Age=30}
$myObject | Add-Member -MemberType NoteProperty -Name 'Location' -Value 'New York'
$myObject
Understanding PowerShell Objects
What Are PowerShell Objects?
PowerShell objects are the foundational elements of any script or command. They encapsulate data and provide a way to work with it programmatically. An object typically consists of properties (which store data) and methods (which are actions you can perform on the object). Common types of objects in PowerShell include file system items, services, and even the output of cmdlets like `Get-Process` or `Get-Service`.
Why Use Custom Properties?
Adding custom properties to objects enhances usability and adaptability. Customization allows you to mold the default objects to better align with your specific goals or requirements. Moreover, using custom properties expands the functionality of an object, enabling you to streamline your data handling processes. Additionally, you can improve organizational structure within your scripts, leading to easier maintenance and clearer logic.
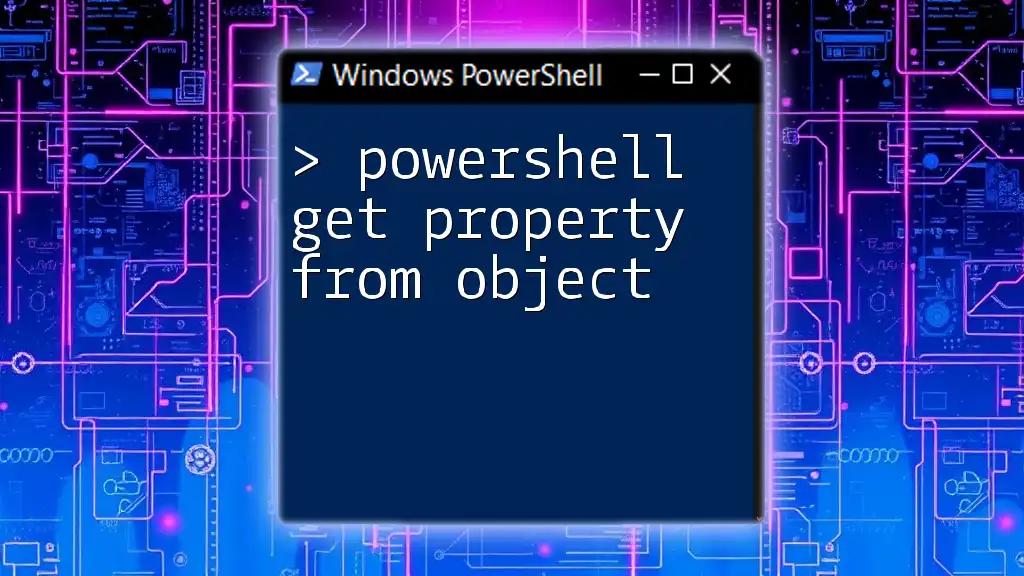
The `Add-Member` Cmdlet
Introduction to Add-Member
The `Add-Member` cmdlet is a crucial tool in PowerShell for augmenting objects with additional properties and methods. It provides a straightforward method to customize existing objects or create entirely new ones with tailor-made attributes, making it indispensable for anyone wishing to manipulate data efficiently.
Syntax of Add-Member
The basic syntax for `Add-Member` is as follows:
Add-Member -InputObject <PSObject> -MemberType <MemberType> -Name <String> -Value <Object>
This command structures the addition of a property or method by specifying the target object, the member type, the name of the new property or method, and its value.
Member Types
Understanding the various `MemberType` values is essential:
- Property: This represents a new property similar to existing ones.
- Method: It enables you to define a functional action associated with the object.
- NoteProperty: A simpler approach for adding properties that don’t involve complex data types.
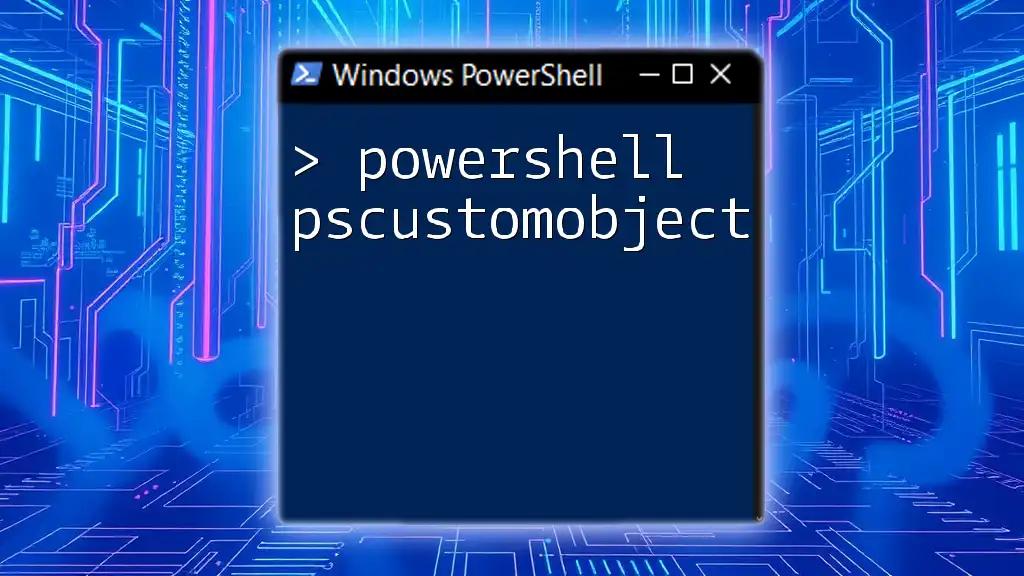
Adding Properties: Step-by-Step Guide
Creating a Base Object
Before we can add properties, we need an object to work on. For example, we can create a simple `person` object:
$person = New-Object PSObject -Property @{
FirstName = "John"
LastName = "Doe"
}
This command constructs an object with two properties: `FirstName` and `LastName`.
Adding a Simple Property
Next, you might want to enrich this object with additional data. Suppose we want to add the person's age. Here’s how to do it:
$person | Add-Member -MemberType NoteProperty -Name Age -Value 30
In this example, the `Add-Member` cmdlet adds a new property called `Age` set to 30. You can easily inspect the object afterward, and the age will appear alongside the name properties, showcasing how PowerShell allows you to dynamically enhance objects.
Adding Multiple Properties at Once
To add several properties seamlessly, you can use the pipeline with `Add-Member` like this:
$person | Add-Member -MemberType NoteProperty -Name City -Value "New York" -PassThru |
Add-Member -MemberType NoteProperty -Name JobTitle -Value "Developer"
In this code, we create two additional properties, `City` and `JobTitle`, respectively. The `-PassThru` parameter is essential here as it allows the modified object to be passed along the pipeline, making it incredibly efficient and convenient to observe the cumulative changes.
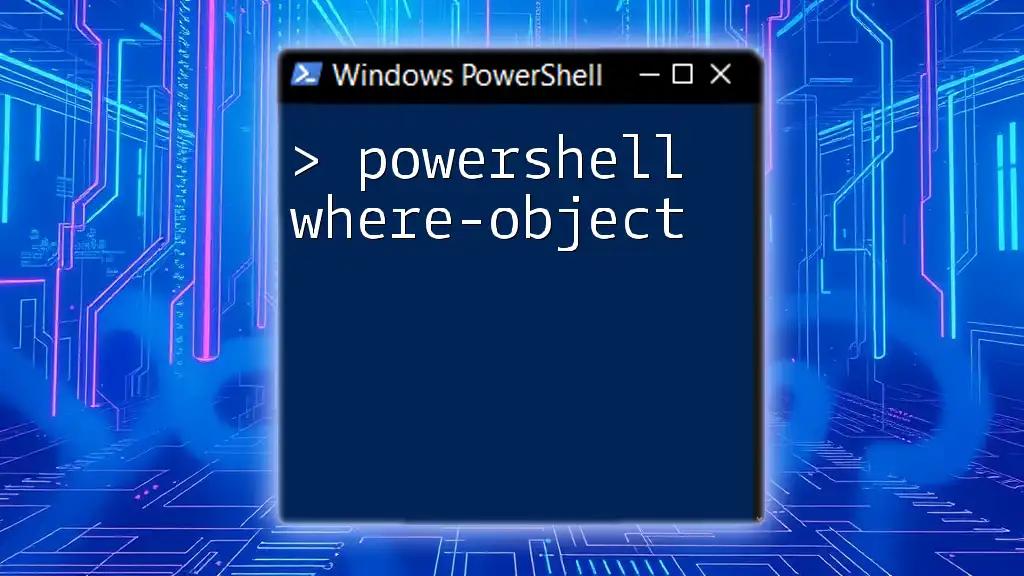
Practical Examples of Adding Properties
Use Case 1: Customizing User Profiles
Consider the scenario of creating a user profile object with additional details. Here’s how you can accomplish that:
$userProfile = New-Object PSObject -Property @{
Name = "Alice"
Role = "Admin"
}
$userProfile | Add-Member -MemberType NoteProperty -Name PhoneNumber -Value "555-1234"
In this case, you create a user profile and then add a new `PhoneNumber` property. Such customization ensures that every object can convey all relevant information for your applications.
Use Case 2: Managing Server Configurations
Another practical example could relate to managing server configurations. Consider this:
$serverConfig = New-Object PSObject -Property @{
ServerName = "Server01"
Status = "Active"
}
$serverConfig | Add-Member -MemberType NoteProperty -Name IPAddress -Value "192.168.1.1"
Here, we constructed a basic server configuration object and added an IP address as a new property. This structure allows for organized and efficient management of server data, which can be crucial for network administrators.
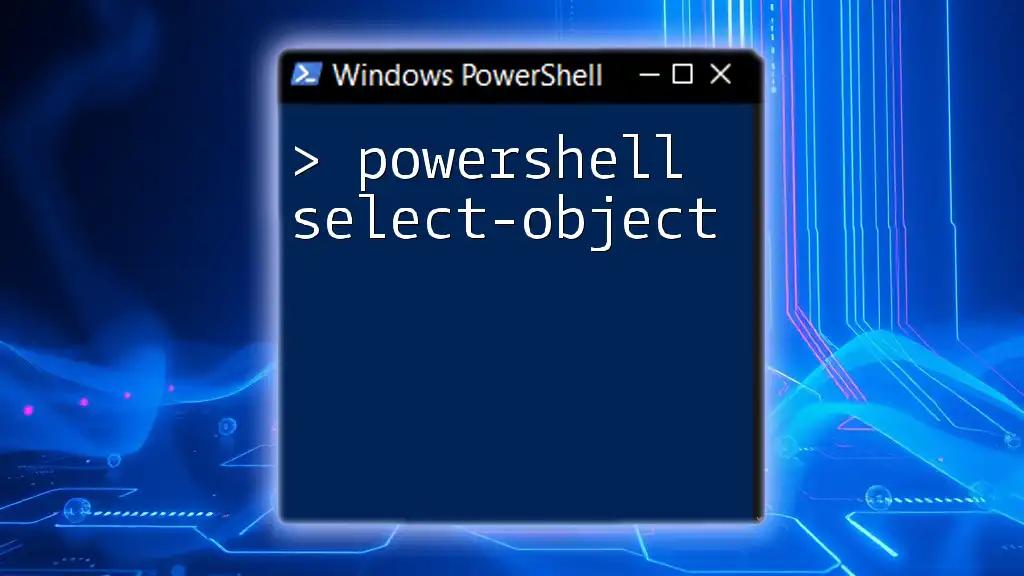
Viewing and Modifying Properties
Inspecting Object Properties
To view the properties of any object, you can use the `Get-Member` cmdlet, allowing you to see the newly added properties in context:
$person | Get-Member
This command produces a list of all properties and methods associated with the object, providing clarity on its structure.
Modifying Existing Properties
You can also modify existing properties conveniently using dot notation. For instance, if you want to update `Age`, you can run:
$person.Age = 31 # Modifying the Age property
This approach makes it easy to directly access and modify properties without needing additional cmdlets.
Removing Properties
If you find yourself in need of removing a property, `Remove-Member` comes into play:
$person | Remove-Member -Name Age
This command effectively removes the `Age` property from the object, demonstrating PowerShell’s flexibility in data management.
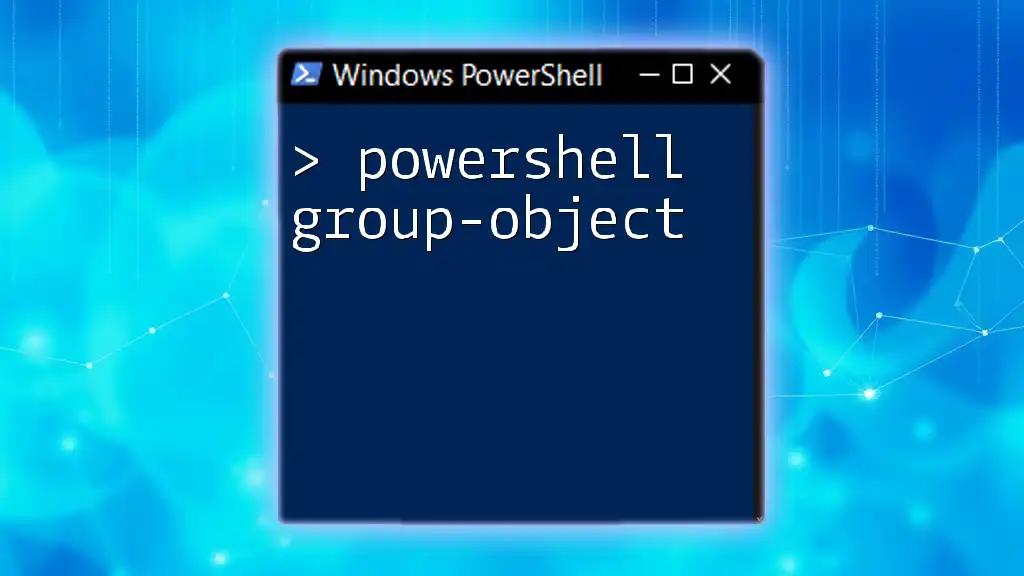
Best Practices for Adding Properties
Naming Conventions
Establishing clear and consistent naming conventions for your properties is vital. Meaningful names enhance readability and maintainability, making it easier for others (or you later on) to navigate your scripts.
Performance Considerations
While it is easy to add properties, be mindful of performance implications. Avoid adding excessive properties without necessity, as they can strain memory and processing power, especially in large scripts or applications.
Documentation and Maintenance
Always document any added properties for reference in the future. This practice ensures that you or your team can understand the data structure and any modifications made over time.
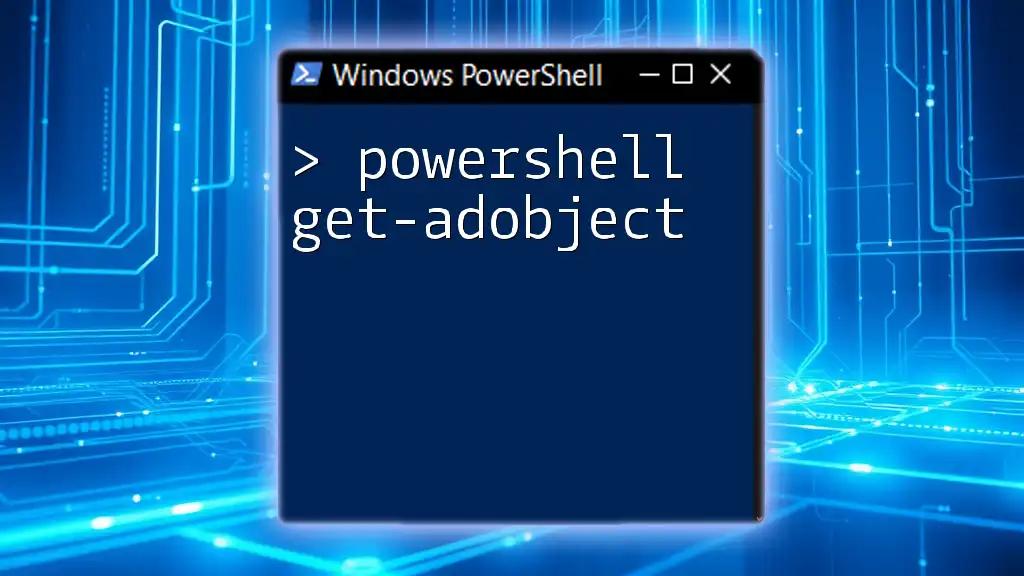
Conclusion
Understanding how to add properties to objects in PowerShell is invaluable for anyone looking to customize their scripting experience. The `Add-Member` cmdlet provides an effective way to tailor objects to your needs, enhancing both functionality and structure. As you experiment with the provided code snippets, feel free to delve deeper and broaden your PowerShell knowledge.
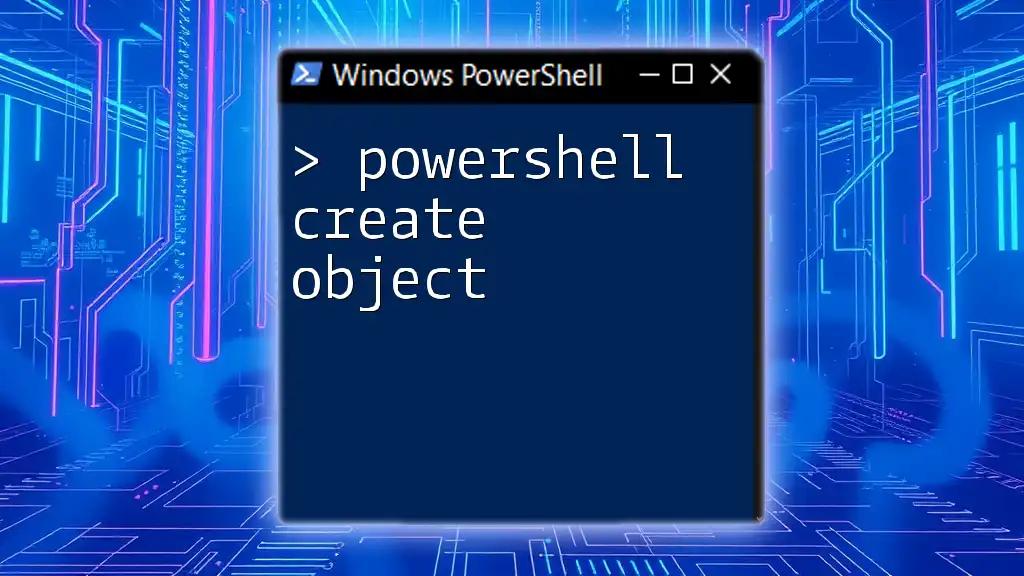
Call to Action
We encourage you to share your experiences or questions concerning adding properties to PowerShell objects. Your insights enrich our community, and by following our blog, you can stay updated on more tips and guides that deepen your PowerShell expertise.

Additional Resources
To further bolster your learning, explore the official PowerShell documentation and consider enrolling in recommended books or online courses tailored to developing your PowerShell skills. These resources can offer structure and depth to your ongoing education in scripting.