The `Where-Object` cmdlet in PowerShell is used to filter objects from a collection based on specified conditions.
Get-Service | Where-Object { $_.Status -eq 'Running' }
Understanding `Where-Object`
What is Where-Object in PowerShell?
`Where-Object` is a powerful cmdlet in PowerShell that allows you to filter objects based on specified criteria. Every time you work with PowerShell commands, you're often dealing with collections of objects—files, processes, services, and more. The `Where-Object` cmdlet helps you to sift through these collections to find exactly what you need by applying complex filtering conditions.
Syntax of Where-Object
The basic syntax of `Where-Object` is straightforward but is key to its functionality:
Where-Object { <condition> }
In this syntax, `<condition>` represents the criteria that each object will be evaluated against.
For example, to filter out processes with a CPU usage greater than 50:
Get-Process | Where-Object { $_.CPU -gt 50 }
The `$_` variable represents the current object in the pipeline.

Utilizing `Where-Object`
How to Use Where-Object
Using `Where-Object` effectively involves piping output from other cmdlets into it. You can apply a single condition or multiple conditions within the script block. The filtered results can then be used for reporting, analysis, or further processing.
Example of Basic Usage
To find all services that are currently running:
Get-Service | Where-Object { $_.Status -eq 'Running' }
This command extracts the services, and the `Where-Object` filters it down to only those that are 'Running'.
PowerShell Where Object Filtering
Filtering can be as simple or as complex as you need. You can access properties of the object to filter them based on their values.
Filtering with Multiple Properties
You can employ logical operators such as `-and`, `-or`, and even negation `-not` to create complex conditions. For instance, to get all running services that also have a name starting with 'M':
Get-Service | Where-Object { $_.Status -eq 'Running' -and $_.Name -like 'M*' }

Advanced Usage of Where-Object
PowerShell `Where-Object` Like Operator
The `-like` operator is used for pattern matching in string properties, making it extremely useful for filtering based on partial matches. When combined with wildcards (`*`), you can simplify searches.
Example: Filtering with the Like Operator
To filter files to obtain only `.txt` files from a directory, you could use:
Get-ChildItem | Where-Object { $_.Name -like '*.txt' }
This command showcases how `Where-Object` can dynamically filter content based on file extensions.
Using `Where-Object` with Select-Object
Often, once you’ve filtered the necessary objects, you will also want to select specific properties. This is where combining `Where-Object` with `Select-Object` becomes beneficial.
Example
A typical operation could involve processes consuming a lot of CPU:
Get-Process | Where-Object { $_.CPU -gt 10 } | Select-Object Name, CPU
This filters processes with high CPU usage and selects only their names and CPU usage for display.

Tips and Best Practices
Using Where-Object Effectively
Efficient use of `Where-Object` can improve both the readability and the performance of your scripts. Always ensure that your conditions are as simple as necessary. Complex conditions can be broken down into simpler logical components to make the code easier to understand.
Performance Considerations
While `Where-Object` is handy, relying heavily on it may slow down scripts, especially when filtering large datasets. In cases where performance is crucial, consider alternative methods such as using `Where-Object` at the end of a pipeline or filtering within the commands themselves when possible.

Examples of Where-Object in Real-World Use Cases
Aggregate Data with Where-Object
Common scenarios include using `Where-Object` for inspections and reports. For instance, if you want to analyze which services are stopped in your system:
Get-Service | Where-Object { $_.Status -eq 'Stopped' }
This simple command provides insight into your system's services and helps in managing startup configurations.
Practical Scenarios
-
Finding Services: To find services that are not running:
Get-Service | Where-Object { $_.Status -eq 'Stopped' }
-
File Management: To list files larger than 10MB in a directory:
Get-ChildItem | Where-Object { $_.Length -gt 10MB }
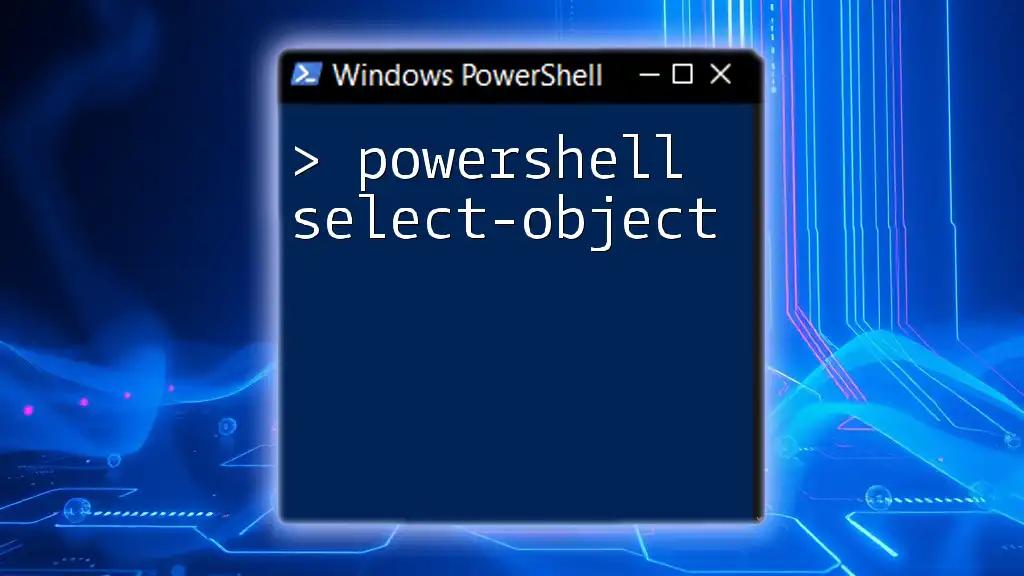
Conclusion
The `PowerShell Where-Object` cmdlet serves as an essential tool for any PowerShell scripter, allowing for dynamic filtering of data. Mastering this cmdlet can significantly enhance your ability to manage and manipulate data in PowerShell effectively.
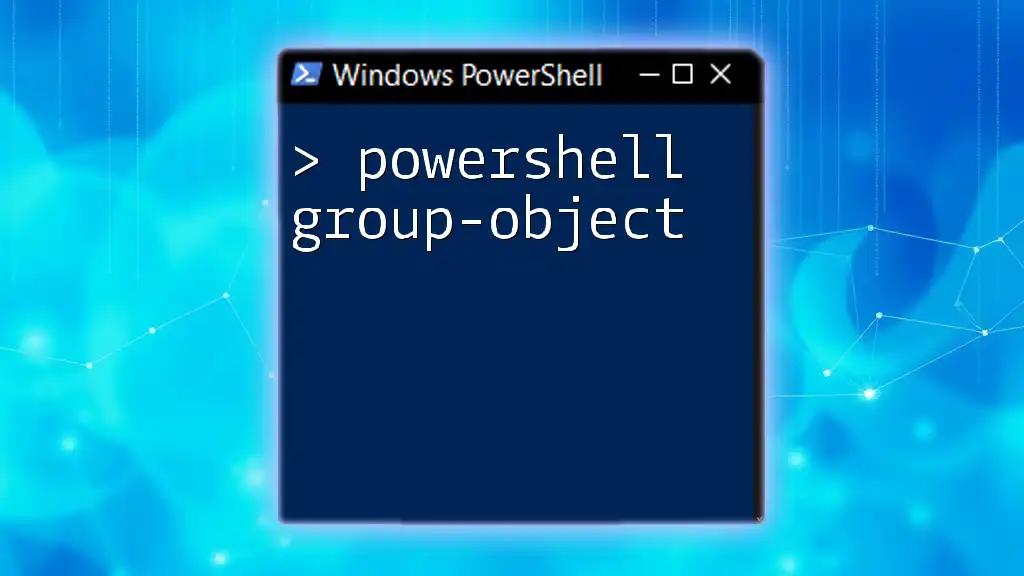
Additional Resources
For further learning, refer to the official PowerShell documentation which provides extensive details and examples. Exploring related cmdlets like `Select-Object` will also deepen your understanding of PowerShell.
Call to Action
Share your experiences with `Where-Object` or any unique filtering techniques you employ in PowerShell scripts, and stay tuned for more insights and practical tips from our continued learning resources!