The `New-Object` cmdlet in PowerShell allows you to create an instance of a .NET Framework or COM object, enabling you to utilize its properties and methods in your scripts.
$newObject = New-Object -TypeName PSObject -Property @{Name='Example'; Value='Hello, World!'}
What is New Object in PowerShell?
PowerShell's `New-Object` command allows you to create instances of .NET objects and custom PowerShell objects. Understanding how to use `New-Object` effectively is essential for automating tasks within PowerShell, as it enables you to leverage the power of .NET libraries along with your custom configurations.

Understanding the Syntax of New-Object
Basic Syntax of New-Object
The basic syntax of the `New-Object` command consists of specifying the type of object you want to create alongside any relevant parameters:
New-Object -TypeName <TypeName> [-Property <hashtable>] [-ArgumentList <args>]
Here’s a simple example of creating a new PowerShell object:
$obj = New-Object -TypeName PSObject
This creates a new instance of the `PSObject` class.
Common Parameters of New-Object
-
-TypeName: This parameter specifies the type of object you want to create. It could be a PowerShell type, .NET Framework type, or a COM object.
-
-Property: This parameter allows you to initialize the properties of the object at the time of creation. For instance, creating a custom object with properties is straightforward:
$customObject = New-Object PSObject -Property @{
Name = "Alice"
Age = 25
}
- -ArgumentList: This parameter enables you to pass constructor arguments to the object. An example of its use is shown below:
$stringBuilder = New-Object -TypeName System.Text.StringBuilder -ArgumentList 100
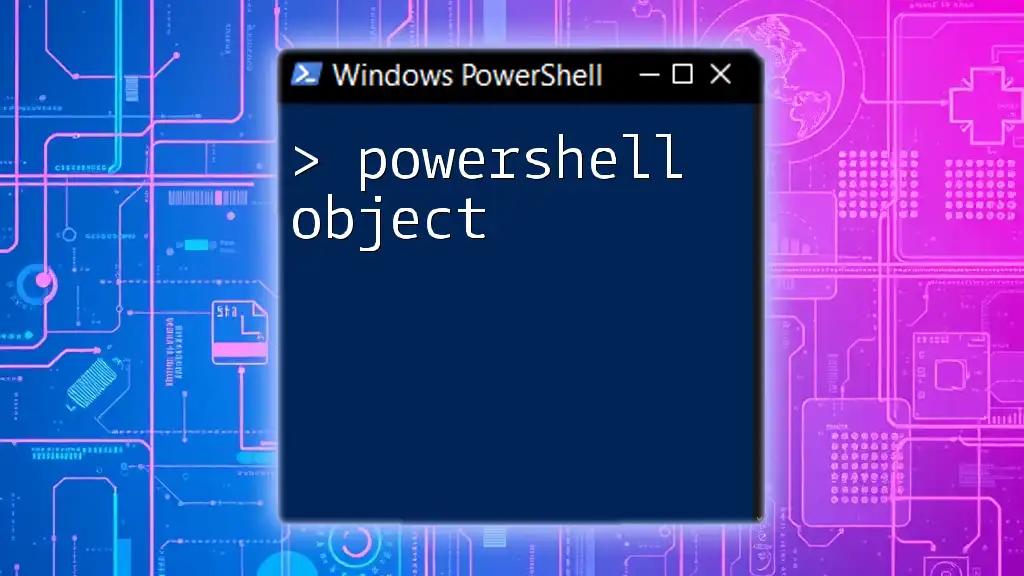
Types of Objects You Can Create
Creating Custom Objects
Custom objects are powerful for structuring complex data. You can define any set of properties to reflect your specific needs, which is extremely useful in scripts.
Example of a custom object:
$customObject = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
Occupation = "Developer"
}
Creating .NET Objects with New-Object
PowerShell provides seamless integration with .NET objects. You might want to use type libraries from .NET to manipulate text, numbers, dates, etc.
Example of creating a .NET object:
$dateTime = New-Object -TypeName DateTime
This allows the use of .NET methods on the `$dateTime` object, such as formatting and adding time.
Creating COM Objects
When you need to interact with applications that expose a COM interface (like Microsoft Excel), `New-Object` becomes essential.
For example, you can create a new Excel application instance:
$excel = New-Object -ComObject Excel.Application
This object can be manipulated directly via PowerShell to create worksheets, write data, and much more.

Using New-Object in Scripts
Integrating New-Object in Automation Scripts
Incorporating `New-Object` in your PowerShell scripts can lead to improved organization and functionality. You can create functions to automate repetitive tasks.
Here's an example function that creates a user object:
function CreateUser {
param($name, $email)
$user = New-Object PSObject -Property @{
UserName = $name
Email = $email
}
return $user
}
This function can return a user object with specified properties, proving beneficial in user management operations.
Best Practices for Creating Objects in PowerShell
-
Consistent Naming Conventions: Use clear naming conventions to make properties easily understandable.
-
Consider Object Lifecycles: Be mindful of scoping, particularly when passing objects between functions.
-
Initialization: Initialize properties upfront to avoid null reference errors during runtime.
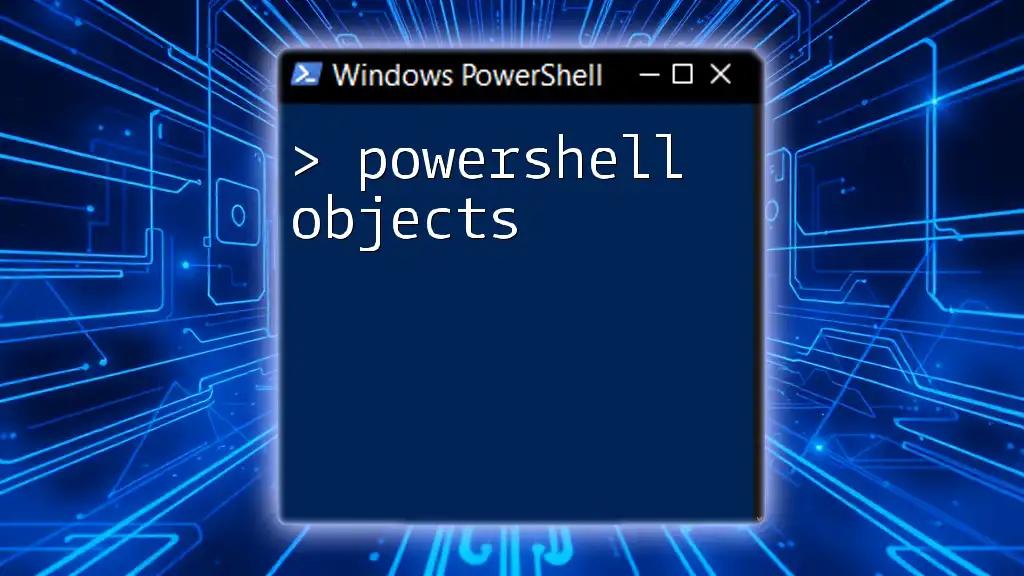
Alternatives to New-Object
Using the [PSCustomObject] Type
While `New-Object` is powerful, using `[PSCustomObject]` for creating custom objects is often more efficient and syntactically cleaner.
Example of a PSCustomObject:
$psCustomObject = [PSCustomObject]@{
Name = "Jane Doe"
Age = 28
}
This approach provides better performance and is easier for PowerShell to optimize.
Creating Objects Without New-Object
You can create simple objects without using `New-Object`. For example:
$user = [PSCustomObject]@{
Username = "SampleUser"
Email = "user@example.com"
}
This method is often more readable and concise compared to using `New-Object`.

Troubleshooting Common Errors with New-Object
Common Error Messages
When using `New-Object`, you may encounter some common issues, such as:
- Cannot find type: Occurs when the specified type name is incorrect.
- Invalid parameter: Happens when an unsupported parameter is passed to the command.
Debugging Techniques
To debug issues, you can use `Write-Host` or `Write-Output` to log variable states and detect where the failure occurs. Enabling execution policies and running scripts in the PowerShell ISE can also provide helpful insights.
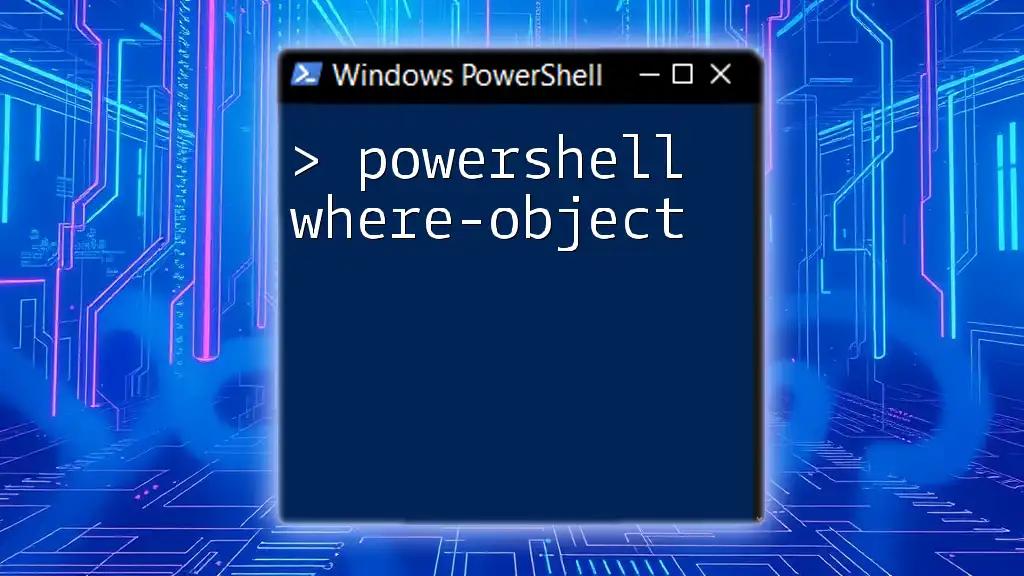
Conclusion
Mastering the `New-Object` command in PowerShell is crucial for creating and managing objects within your scripts. Through the use of `New-Object` and its alternatives, you can efficiently structure your automation tasks, leading to cleaner and more effective PowerShell scripts. As you practice creating various objects, you'll find that your ability to manipulate data and automate processes will enhance significantly.
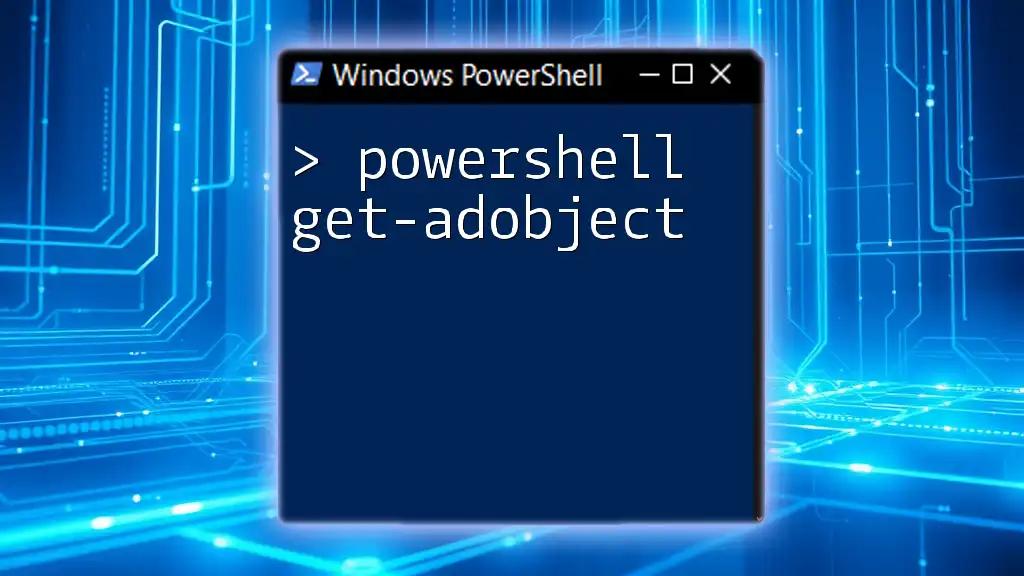
Additional Resources
For further reading on PowerShell objects, consider consulting the official Microsoft documentation. Engaging with communities and forums can also provide valuable insights and support as you continue your PowerShell journey.
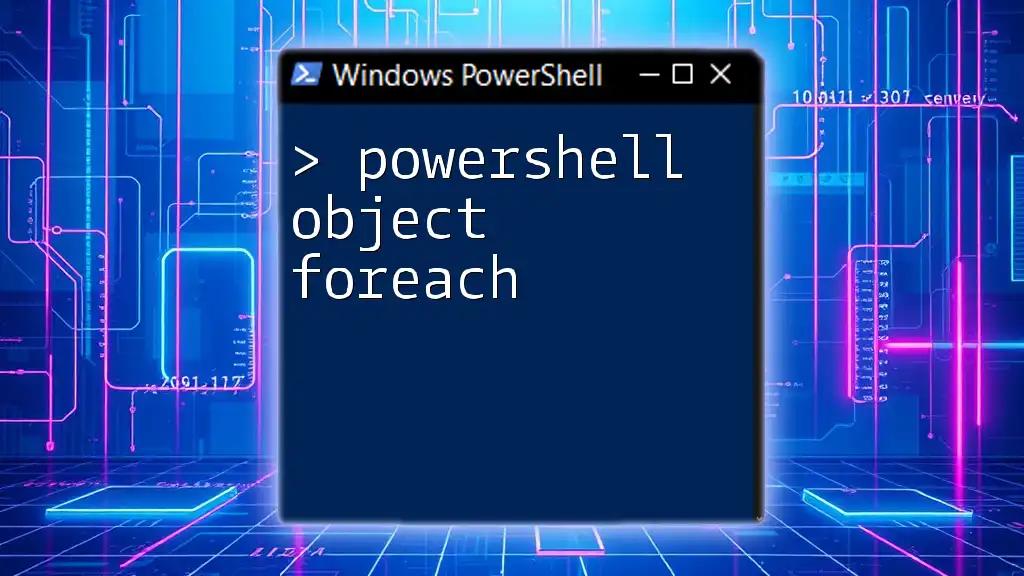
Call to Action
Join our courses to further enhance your PowerShell skills. Share your experiences with object creation in PowerShell and let us know how it has improved your workflow!