The `New-Item` cmdlet in PowerShell is used to create a new item, such as a file, folder, or registry key, at a specified location.
Here's a code snippet to create a new text file using `New-Item`:
New-Item -Path "C:\path\to\your\file.txt" -ItemType "File"
Understanding New-Item
What is New-Item?
The `New-Item` cmdlet in PowerShell is an essential tool for creating new items, be they files, folders, or other objects, within your file system or other data store. It streamlines the process of file and folder management, making it a critical function for system administrators, developers, and anyone looking to automate tasks within the Windows operating system. By mastering `New-Item`, you unlock the potential to manage resources effectively, ultimately enhancing productivity and efficiency in your workflows.
Syntax of New-Item
Understanding the syntax of `New-Item` is fundamental to using it effectively. The cmdlet can be expressed as follows:
New-Item [-Path] <String> [-Name <String>] [-ItemType <String>] [-Value <String>] [-Force] [-PassThru] [-Credential <PSCredential>] [<CommonParameters>]
In this syntax:
- -Path specifies the location where the new item will be created.
- -Name is the name of the item, which is necessary for file creation when specifying the item type.
- -ItemType defines what type of item is being created (e.g., File, Directory).
- -Value allows you to set content for a file during its creation.
- -Force allows operation without prompt in case of existing items or permissions.
- -PassThru lets you retrieve the created object for further manipulation.
- -Credential permits you to provide credentials for executing commands in a restricted context.
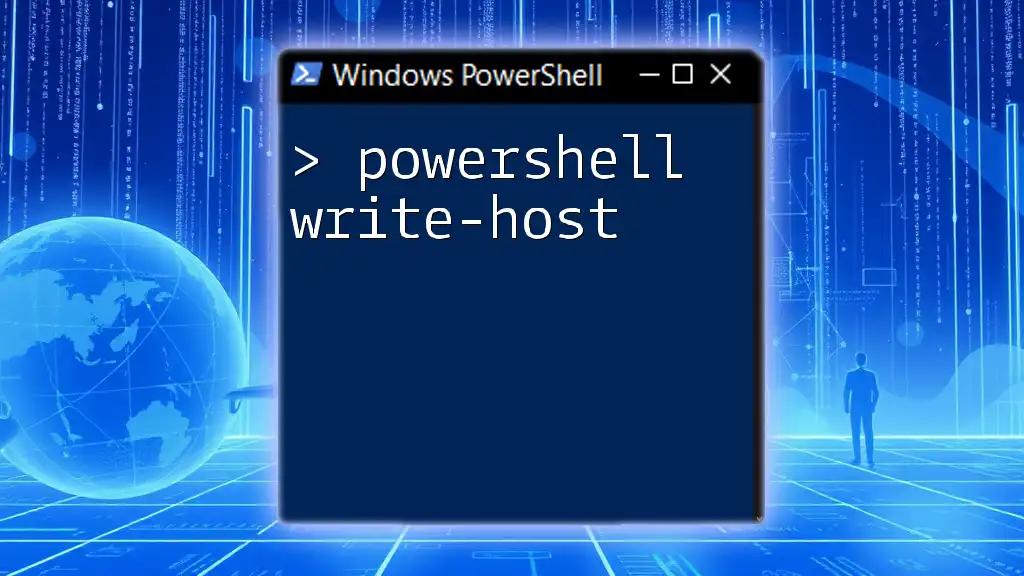
Parameters Explained
Path
The `-Path` parameter is crucial, as it determines where in your filesystem (or other data store) your new item will be created. You can specify a full path or a relative path based on the current location in PowerShell. For example, to create an item directly in the root of the C: drive, you would specify:
New-Item -Path "C:\" -ItemType "Directory" -Name "NewFolder"
Name
The `-Name` parameter is used to give a name to the new item. While the name is not strictly necessary when the item type is a directory and you are specifying a path, it is essential when creating a file. The following example illustrates creating a folder with a specified name:
New-Item -Path "C:\MyDocuments" -Name "ProjectFiles" -ItemType "Directory"
ItemType
The `-ItemType` parameter specifies what kind of item you are creating. The most commonly used types are:
- File: Creates a new file.
- Directory: Creates a new folder (directory).
Understanding the item types allows you to use `New-Item` correctly. For example, to create a new text file, you could use:
New-Item -Path "C:\MyFiles" -Name "Notes.txt" -ItemType "File"
Value
The `-Value` parameter is particularly useful when creating files, as it defines the content of the file upon creation. If you're creating a file and want to set its initial content, this is the parameter to use. Here’s an example:
New-Item -Path "C:\MyFiles" -Name "Greeting.txt" -ItemType "File" -Value "Hello, PowerShell!"
Additional Parameters
Force
The `-Force` parameter is a powerful feature that allows the cmdlet to bypass restrictions. For instance, if you attempt to create a file in a location where a file with the same name exists, using `-Force` will overwrite it without prompting:
New-Item -Path "C:\MyFiles\Notes.txt" -ItemType "File" -Value "This will overwrite the previous content." -Force
PassThru
By using the `-PassThru` parameter, you can direct PowerShell to return the newly created item as an output. This can then be used for additional processing:
$myFile = New-Item -Path "C:\MyFiles" -Name "Output.txt" -ItemType "File" -Value "This is the output." -PassThru
Credential
For cases where you need to create items in protected paths or remote locations, the `-Credential` parameter allows you to specify credentials. This is particularly useful when working in environments with strict permission settings.
$cred = Get-Credential
New-Item -Path "\\RemoteServer\SharedFolder" -Name "DataFile.txt" -ItemType "File" -Value "Hello from PowerShell!" -Credential $cred
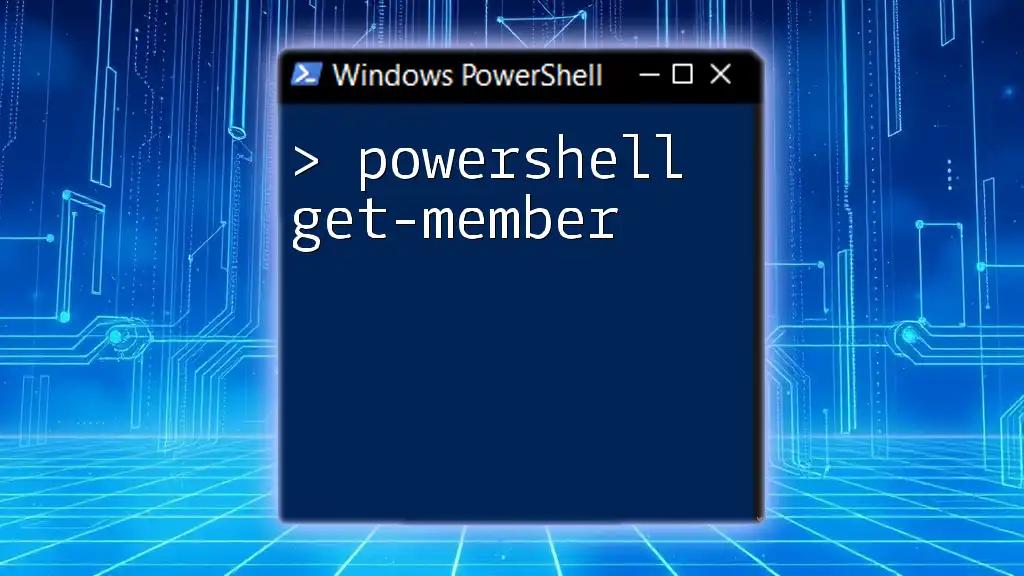
Creating Files and Directories
Creating a Directory
Creating a directory using `New-Item` can be accomplished with a straightforward command. Below is an example that outlines the steps:
New-Item -Path "C:\ExampleDirectory" -ItemType "Directory"
This command will create a new directory called "ExampleDirectory" in the C: drive. A best practice is to verify that your path is correct before executing the command to avoid unintentional errors.
Creating a File
To create a file using PowerShell with `New-Item`, you need to specify the path and item type. Additionally, you can create a file with initial content using the `-Value` parameter, as shown below:
New-Item -Path "C:\ExampleDirectory\example.txt" -ItemType "File" -Value "Hello, World!"
Creating Multiple Items
With PowerShell's flexibility, you can even create multiple items in a loop. Below is a sample code snippet that demonstrates how to create several files in a directory:
foreach ($i in 1..5) {
New-Item -Path "C:\ExampleDirectory\File$i.txt" -ItemType "File"
}
This command creates five new files named File1.txt through File5.txt within the specified directory.
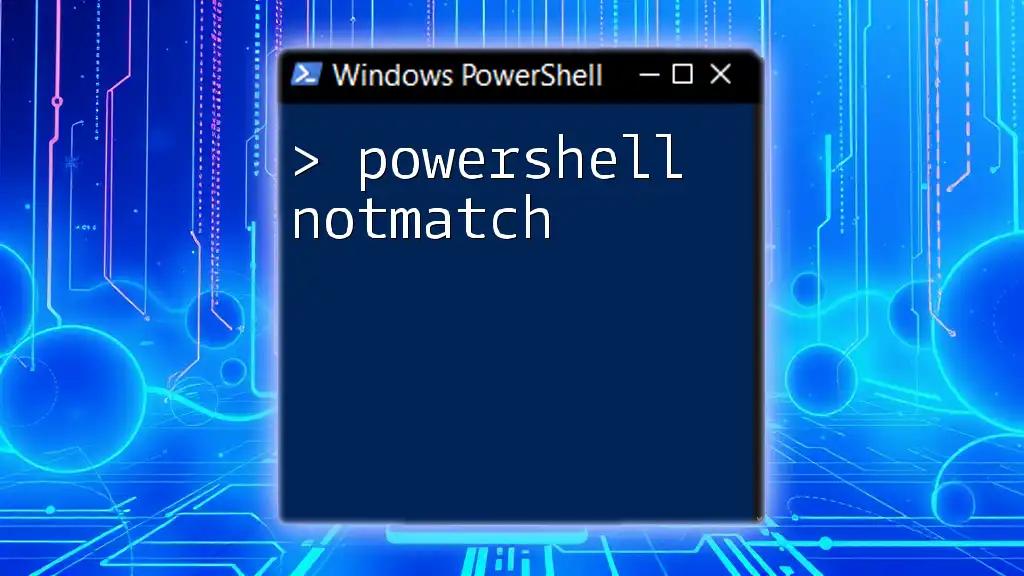
Advanced Usage of New-Item
Using New-Item with other Cmdlets
Combining `New-Item` with other cmdlets can lead to efficient workflows. For example, after creating a script file, you might want to populate it using `Set-Content`:
New-Item -Path "C:\Scripts" -Name "MyScript.ps1" -ItemType "File" -Value "# PowerShell Script"
Set-Content -Path "C:\Scripts\MyScript.ps1" -Value "Write-Host 'My PowerShell script is running!'"
Error Handling
When using `New-Item`, various errors may arise, such as permission issues or incorrect paths. Best practices in error handling include utilizing `Try-Catch` blocks to manage exceptions gracefully and provide meaningful feedback:
try {
New-Item -Path "C:\ProtectedFolder" -Name "NewItem.txt" -ItemType "File"
} catch {
Write-Host "Error encountered: $_"
}
Troubleshooting New-Item
If you encounter issues using `New-Item`, consider checking the following:
- Permissions: Ensure you have the necessary permissions to the specified path.
- Path Validity: Confirm the path exists or is correctly formatted.
- ItemType Availability: Verify that you are using supported item types for the new item.
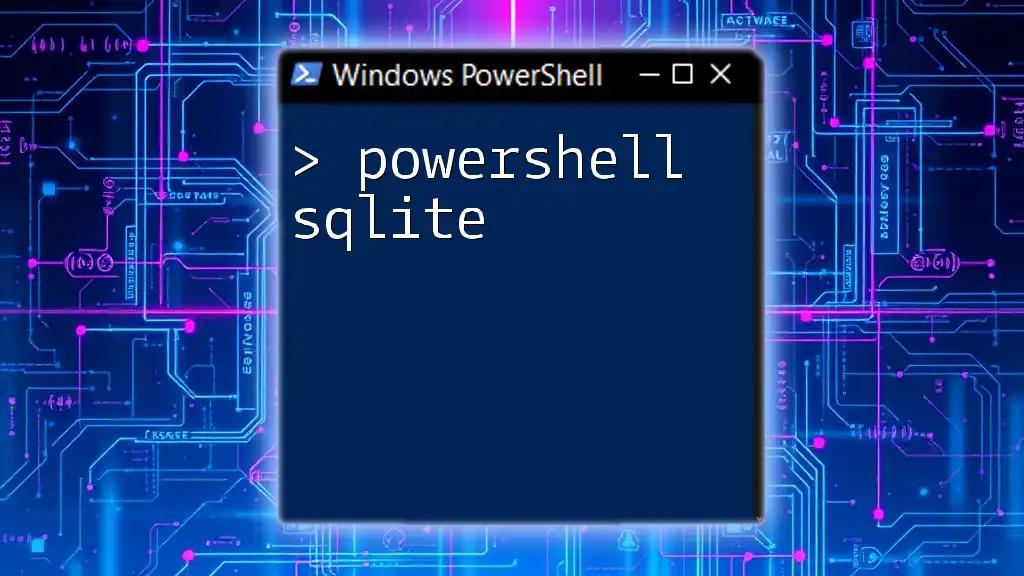
Conclusion
The `New-Item` cmdlet is an essential part of PowerShell for anyone looking to improve their file and folder management skills. Not only does it allow for the quick creation of items, but it also integrates smoothly with other cmdlets for more complex automation tasks. By engaging with the command and experimenting with its different parameters and use cases, users can seamlessly enhance their productivity. Mastering `New-Item` is an invitation to delve deeper into the broader world of PowerShell, ultimately transforming your approach to system administration and scripting.
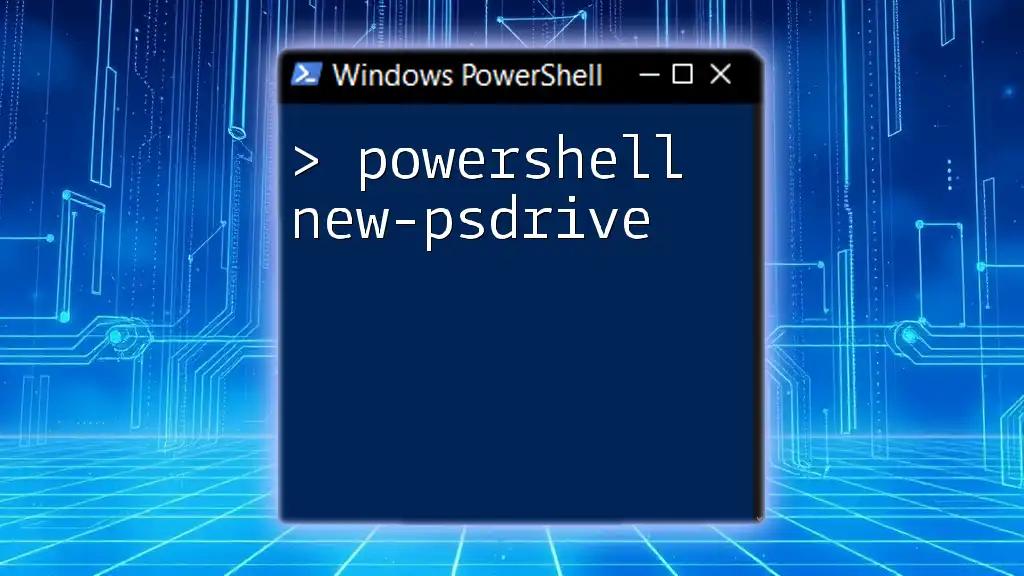
Call to Action
If this guide has empowered you to explore the power of `New-Item`, we invite you to subscribe for more informative tips on PowerShell and automation. Share your experiences or questions in the comments below, and look out for our upcoming courses and resources that will enrich your PowerShell skillset!