Certainly! A nested `if` statement in PowerShell allows you to check multiple conditions sequentially, where the second `if` statement is executed only if the first `if` condition is true. Here's a simple example:
$number = 10
if ($number -gt 5) {
Write-Host 'Number is greater than 5'
if ($number -lt 15) {
Write-Host 'Number is also less than 15'
}
}
Understanding Conditional Logic in PowerShell
What Are If Statements?
In PowerShell, if statements are fundamental elements used for decision-making in scripts. They allow your code to execute specific blocks based on whether a given condition evaluates to true or false. The basic syntax is:
if (condition) {
# Code to execute if condition is true
}
For example, a simple if statement can be as follows:
$number = 5
if ($number -gt 3) {
Write-Host "The number is greater than 3."
}
In this example, the message will be displayed because the condition (`$number -gt 3`) evaluates to true.
Why Use Nested If Statements?
Nested if statements allow you to create more complex decision structures by embedding one or more if statements inside another. This is particularly useful for handling multiple layers of conditions, where the outcome depends on the result of the first condition.
Use Cases for Nested Conditions
- User Input Validation: Validating user inputs uses nested if statements to check a series of conditions.
- Role-Based Access Control: Determining user permissions based on multiple criteria.
Advantages of Using Nested If Statements
- They help manage complex logic effectively.
- Improve code organization by compartmentalizing checks.

Structure of Nested If Statements
Syntax of Nested If Statements
The general structure of a nested if statement involves layering conditions within another if statement. The syntax can be illustrated as follows:
if (condition1) {
if (condition2) {
# Code for when both conditions are true
} else {
# Code for when condition1 is true but condition2 is false
}
} else {
# Code for when condition1 is false
}
This setup allows for intricate decision-making processes by evaluating conditions in a hierarchical manner.
Key Components of Nested If Statements
Conditions
In nested if statements, conditions are essential for controlling flow. The following are commonly used comparison operators in PowerShell:
- `==` (equal)
- `!=` (not equal)
- `-lt` (less than)
- `-gt` (greater than)
- `-le` (less than or equal to)
- `-ge` (greater than or equal to)
These operators can be combined within your nested logic to achieve precise checks.
Blocks of Code
It is crucial to note the importance of using curly braces `{}` to define the blocks of code for each condition. These blocks contain the code that will be executed when their respective conditions evaluate to true.
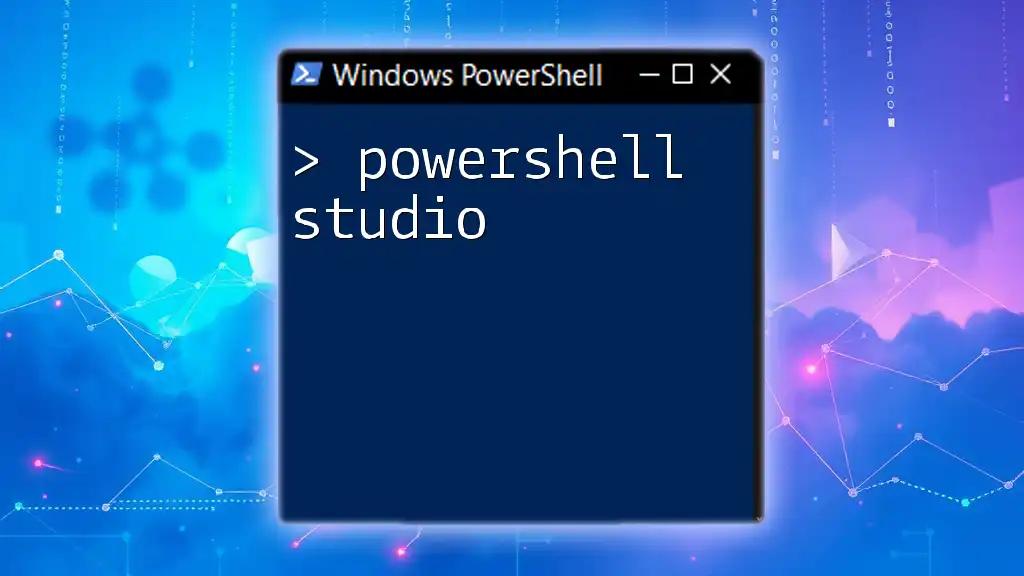
Working with Nested If Statements
Practical Example 1: User Input Validation
Consider a scenario where you want to validate a user input. Nested if statements can make the validation process clear and effective. For example, you might want to check if a number entered by the user falls within a specified range:
$userInput = Read-Host "Enter a number between 1 and 10"
if ($userInput -ge 1) {
if ($userInput -le 10) {
Write-Host "Your input is valid!"
} else {
Write-Host "Input must be 10 or less."
}
} else {
Write-Host "Input must be 1 or greater."
}
In this example:
- The first if checks if the input is greater than or equal to 1.
- The nested if checks if the input is less than or equal to 10.
- Feedback is provided based on the user's input, ensuring clarity in validation.
Practical Example 2: Role-Based Access Control
Another practical use of nested if statements is in role-based access control, where user roles dictate what resources they can access. For instance:
$userRole = Read-Host "Enter user role (Admin/User)"
if ($userRole -eq "Admin") {
Write-Host "You have full access."
} elseif ($userRole -eq "User") {
$userDepartment = Read-Host "Enter department (Sales/Other)"
if ($userDepartment -eq "Sales") {
Write-Host "You can access Sales tools."
} else {
Write-Host "Access limited to general tools."
}
} else {
Write-Host "Invalid role entered."
}
Here:
- The first if checks if the user is an Admin.
- If they are a User, a nested check verifies their department, allowing for differentiated access.

Best Practices for Nested If Statements
Keep It Simple
While nested if statements can be powerful, it's important to keep your code readable and maintainable. Over-complicating your logic with too many nested conditions can lead to confusion. Aim for simplicity; if your logic becomes too deep, consider refactoring.
Documentation
Comment your code diligently, especially when you're using nested if statements. Clear explanations help others (and your future self) understand the logic behind your conditions. For example:
# Check user role and department for access rights
if ($userRole -eq "Admin") {
# Admin has full access
} elseif ($userRole -eq "User") {
# Users need department validation
}
Use Alternatives Where Appropriate
Whenever possible, employ alternatives such as `switch` statements or ternary operations for simpler conditions. These methods can often simplify your code logic and improve clarity without sacrificing functionality.

Conclusion
In summary, PowerShell nested if statements are a powerful tool for developing complex logic in your scripts. They allow for intricate decision-making by letting code evaluate multiple conditions. Mastering nested if statements will significantly enhance your scripting skills and lead to cleaner, more effective code. As you become more proficient, you'll find that these structures become second nature, empowering you to create impactful PowerShell scripts.