A PowerShell nested hash table is a data structure that allows you to store key-value pairs within other key-value pairs, enabling complex configurations and organized data storage.
$nestedHashTable = @{
'User1' = @{
'Name' = 'Alice'
'Age' = 30
}
'User2' = @{
'Name' = 'Bob'
'Age' = 25
}
}
What is a Nested Hash Table?
A nested hash table is essentially a hash table that contains one or more hash tables as its values. This powerful data structure allows you to create complex data representations, where each element can hold an additional layer of key-value pairs.
Using nested hash tables significantly enhances your ability to model real-world data structures and configurations, particularly when dealing with hierarchical data.
Use Cases for Nested Hash Tables
You might wonder, when should I consider using a nested hash table? Here are some compelling scenarios:
- Configuration Settings: Store application settings where options are categorized.
- Structured Data Representation: Model JSON-like data structures for APIs, making it easier to manipulate nested data.
- Organizational Hierarchies: Represent entities with sub-entities, such as departments and their respective roles.
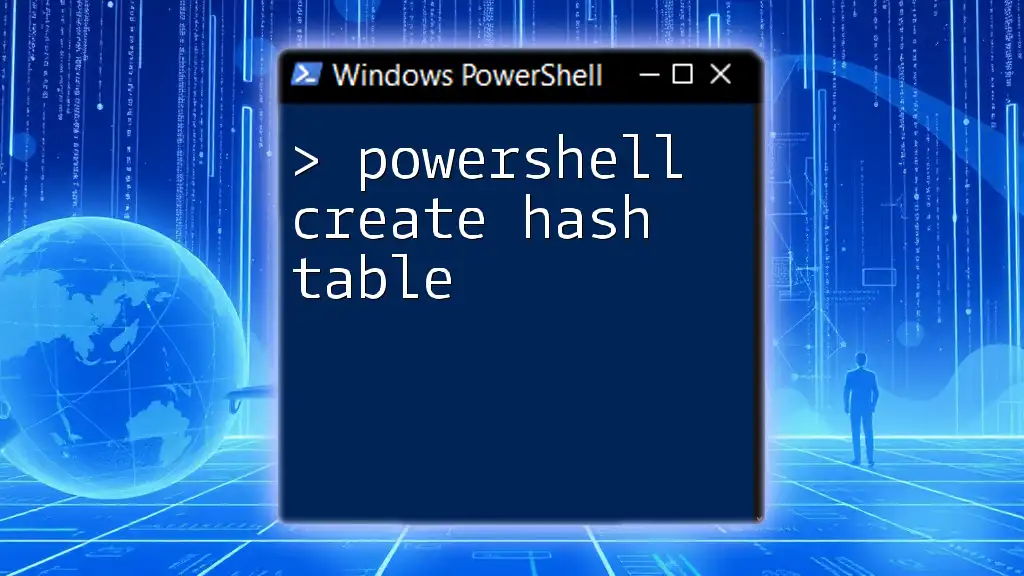
Creating a Nested Hash Table
Syntax and Structure
The syntax for creating a nested hash table in PowerShell is intuitive. You’ll use the `@{}` notation for defining your hash tables. As an example, consider the following code snippet:
$nestedHashTable = @{
Key1 = @{
SubKey1 = "Value1"
SubKey2 = "Value2"
}
Key2 = @{
SubKeyA = "ValueA"
SubKeyB = "ValueB"
}
}
This creates a nested hash table with two outer keys: `Key1` and `Key2`. Each of these keys contains its own hash table, showcasing how data can be structured hierarchically.
Adding Entries to a Nested Hash Table
Adding elements to a nested hash table is straightforward. You can introduce new sub-keys easily. Here's how you can add a new sub-key under `Key1`:
$nestedHashTable['Key1']['SubKey3'] = "Value3"
This code snippet adds `SubKey3` to `Key1` with the value `"Value3"`, demonstrating the flexibility of nested hash tables. You can keep expanding the structure as needed.
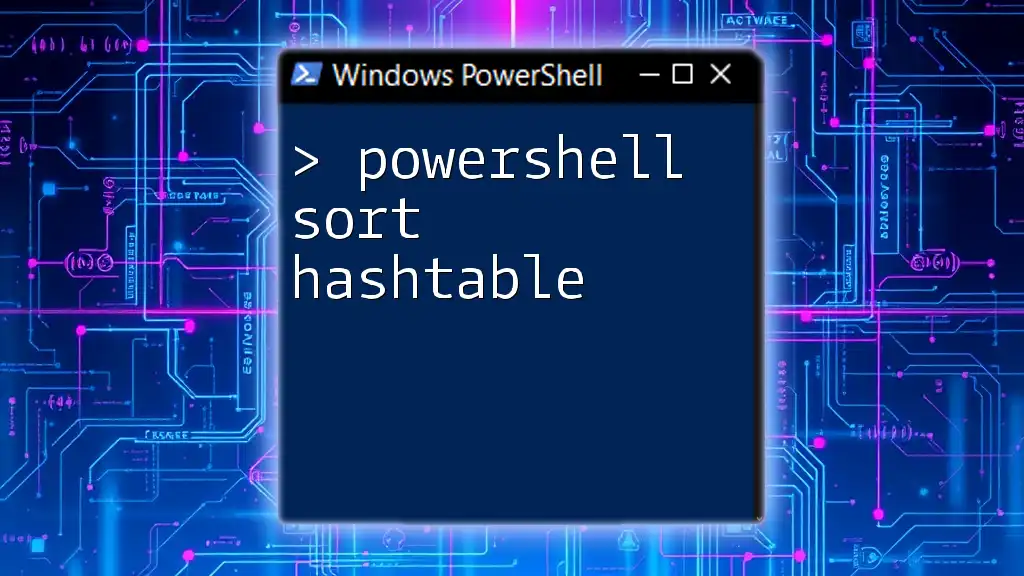
Accessing Data in a Nested Hash Table
Retrieving Values
To retrieve values from a nested hash table, you simply reference the keys in sequence. For instance, if you want to access `SubKey1` under `Key1`, you can do this:
$value = $nestedHashTable['Key1']['SubKey1']
This will assign `"Value1"` to the variable `$value`. The intuitive indexing makes retrieval seamless and efficient.
Iterating Through Entries
When you need to inspect or utilize all the entries, iteration comes into play. You can loop through the outer keys and then through the nested keys as shown in this example:
foreach ($outerKey in $nestedHashTable.Keys) {
Write-Host "Outer Key: $outerKey"
foreach ($innerKey in $nestedHashTable[$outerKey].Keys) {
$value = $nestedHashTable[$outerKey][$innerKey]
Write-Host " Inner Key: $innerKey, Value: $value"
}
}
This code will print both the outer and inner keys along with their respective values, providing a clear view of your nested data structure.
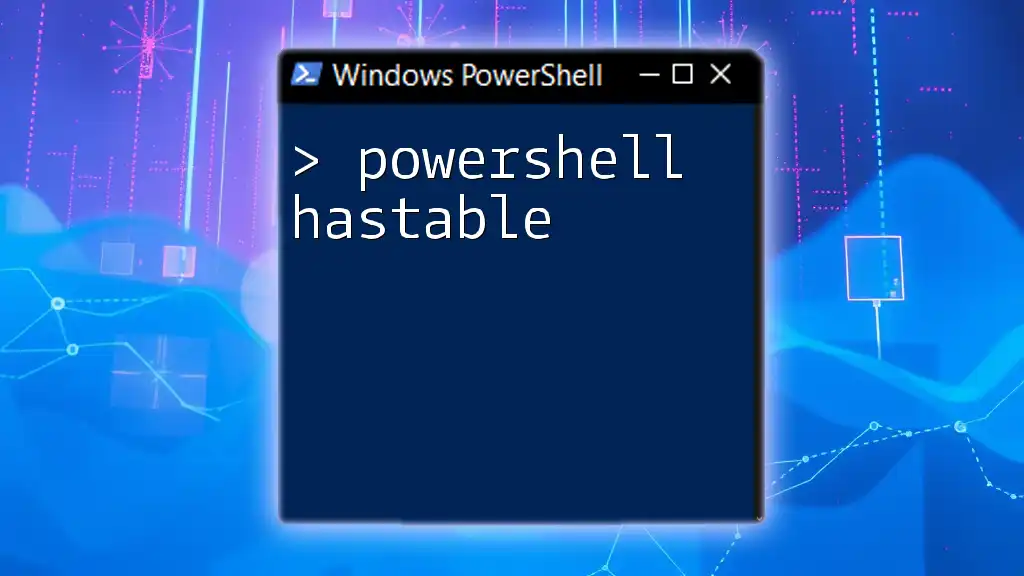
Modifying and Removing Entries
Modifying Existing Values
Updating values in a nested hash table is as simple as accessing the current value and assigning a new one. For instance, to change `SubKey1` under `Key1`:
$nestedHashTable['Key1']['SubKey1'] = "UpdatedValue"
After executing this, whenever you access `SubKey1`, you will get `"UpdatedValue"`.
Removing Keys and Their Values
You can also remove keys and their associated values from a nested hash table. For example, to remove `SubKey2` under `Key1`, use the `Remove` method:
$nestedHashTable['Key1'].Remove('SubKey2')
This will eliminate `SubKey2` from `Key1`, ensuring that your data structure remains clean and relevant.
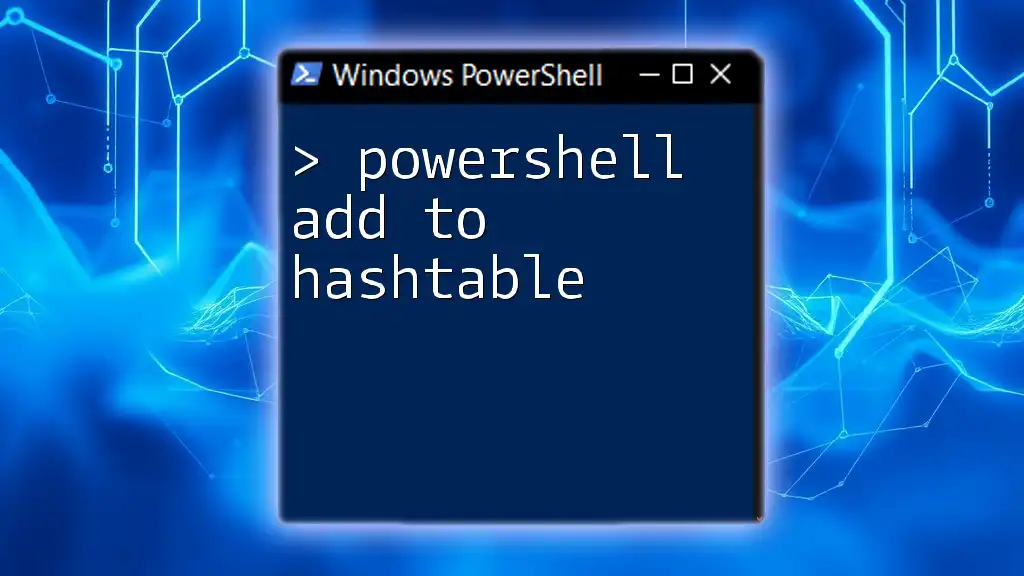
Best Practices for Using Nested Hash Tables
Maintainability
Maintainability is crucial when working with nested hash tables. Ensure that your keys are meaningful, and consider using comments to clarify complex structures. Proper formatting and consistent naming conventions will enhance the readability of your code.
Performance Considerations
Nested hash tables can become complex, potentially affecting performance when manipulated excessively. To optimize:
- Avoid deeply nested structures unless necessary.
- Profile scripts to identify bottlenecks if large datasets are involved.
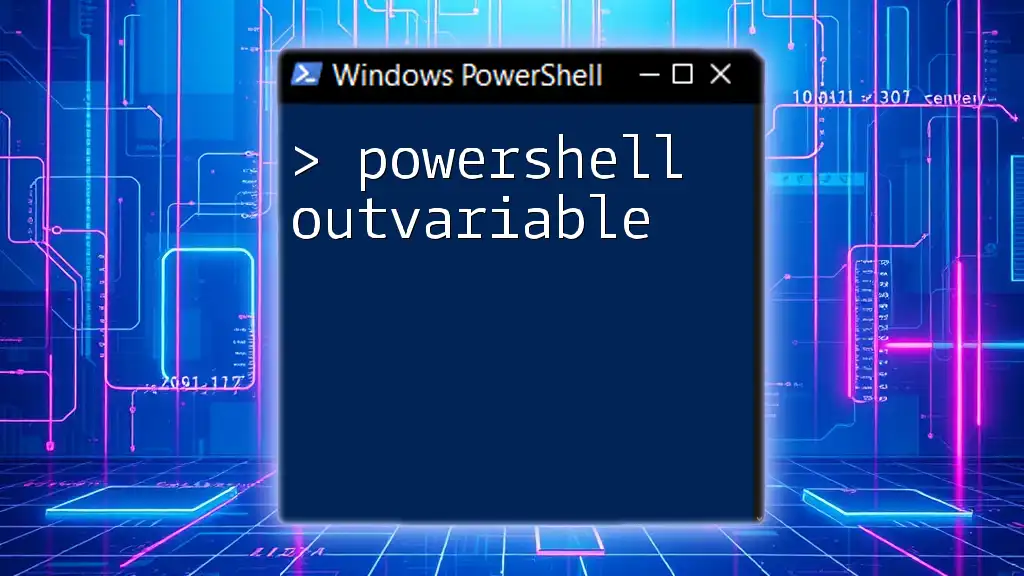
Real-World Scenarios and Examples
Configuring Settings with Nested Hash Tables
Consider a sample configuration where an application may have various environments like development and production. Here’s a conceptual structure:
$config = @{
Development = @{
Database = "DevDB"
Timeout = 30
}
Production = @{
Database = "ProdDB"
Timeout = 120
}
}
This approach provides an efficient way to manage environment-specific settings using nested hash tables.
Data Representation for APIs
When consuming web APIs that return nested JSON responses, nested hash tables can easily represent such data. For example:
$response = @{
User = @{
Name = "Jane Doe"
Details = @{
Age = 30
Address = "123 Main St"
}
}
}
This structure mirrors the usual JSON response, allowing easy data manipulation after fetching it from an API.
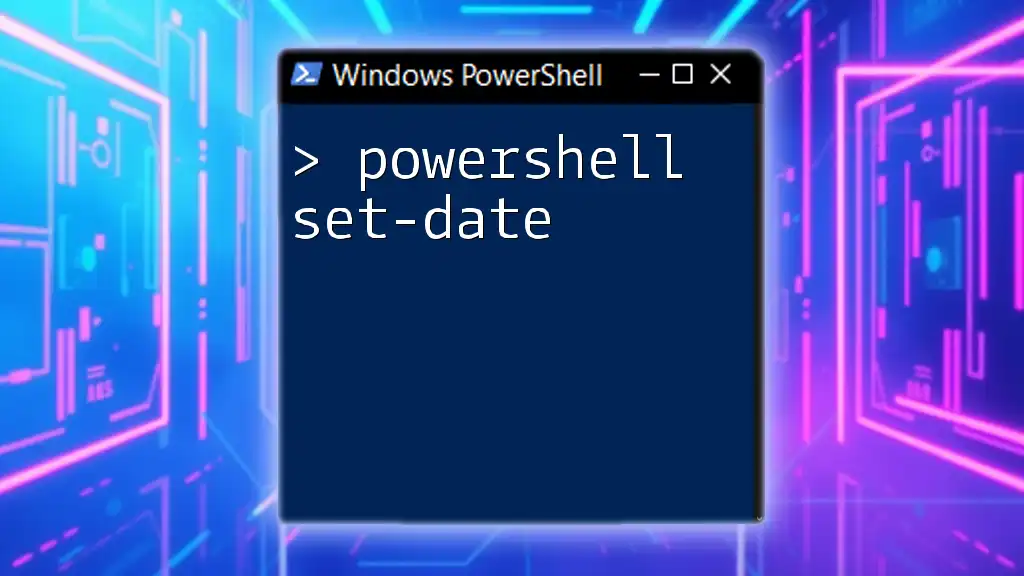
Troubleshooting Common Issues
Understanding Errors
While working with nested hash tables, you may encounter errors such as key not found. This usually occurs when trying to access a key that doesn't exist. Ensure all keys are correctly defined before attempting access.
Debugging Tips
Effective debugging involves printing the keys and values in your hash tables at various points in your script. Utilize the `Get-Member` cmdlet and other debugging tools, such as breakpoints in your IDE, to investigate issues rapidly.
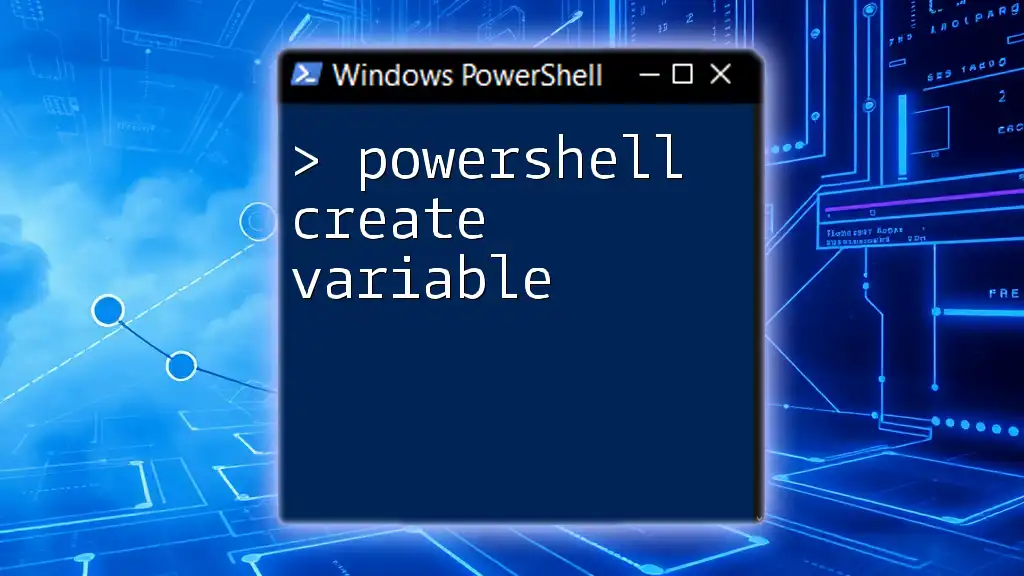
Conclusion
In summary, understanding PowerShell nested hash tables opens the door to powerful data structures that can greatly enhance your scripting capabilities. By utilizing these nested constructs, you can create clean, organized, and efficient code that is both easy to read and maintain.
As you experiment with nested hash tables, you'll find their versatility in problem-solving. Dive in, practice these examples, and enjoy building more sophisticated PowerShell scripts!
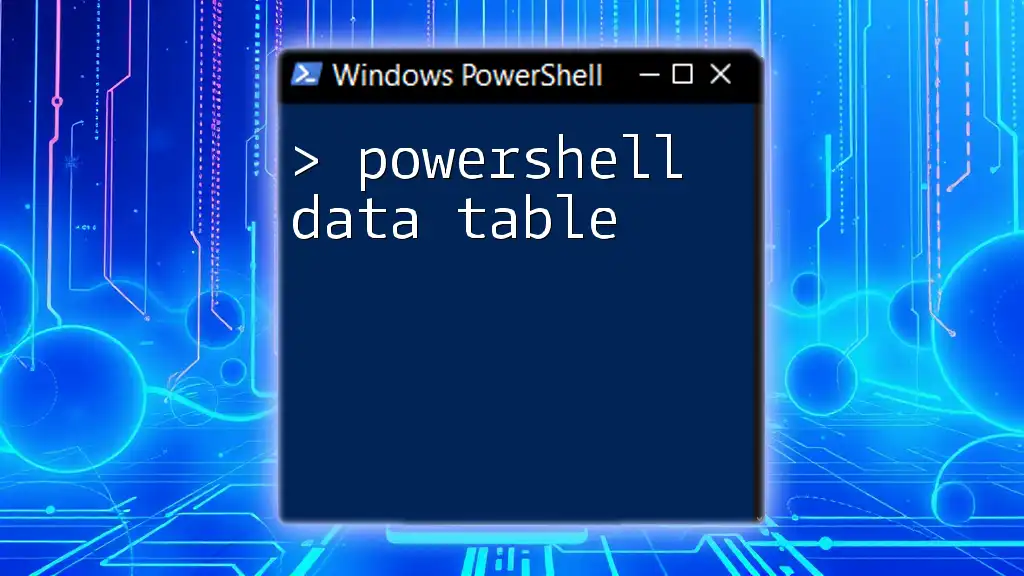
Additional Resources
For those eager to deepen their understanding, consider exploring the official PowerShell documentation and other relevant articles on advanced data structures. Engaging with the community through forums and discussions can also provide invaluable insights and support.