In PowerShell, you can add a key-value pair to a hashtable using the syntax `$hashtable['key'] = 'value';` where `$hashtable` is your hashtable variable.
Here’s a code snippet demonstrating this:
$hashtable = @{}
$hashtable['Name'] = 'John Doe'
$hashtable['Age'] = 30
Understanding Hashtables in PowerShell
What is a Hashtable?
A hashtable is a collection of key-value pairs, similar to a dictionary. In PowerShell, a hashtable allows you to store data in a way that provides quick access to values via unique keys. This data structure is ideal when you need to store related information and retrieve it efficiently based on keys.
Key Features of Hashtables
Hashtables boast several distinctive features:
- Key-Value Pairs: Each entry consists of a unique key and an associated value.
- Dynamic Size: As the data grows, hashtables automatically adjust their size to accommodate changes.
- Fast Lookups: Hashtables provide quick access to their values because they use a hash function.
When to Use Hashtables
Using hashtables makes sense in various scenarios, such as when you need to manage configuration settings, store user information, or maintain pairs of data that will be accessed frequently. For example, an application might need to keep track of user roles and associated permissions where each user role is linked to a set of permissions.
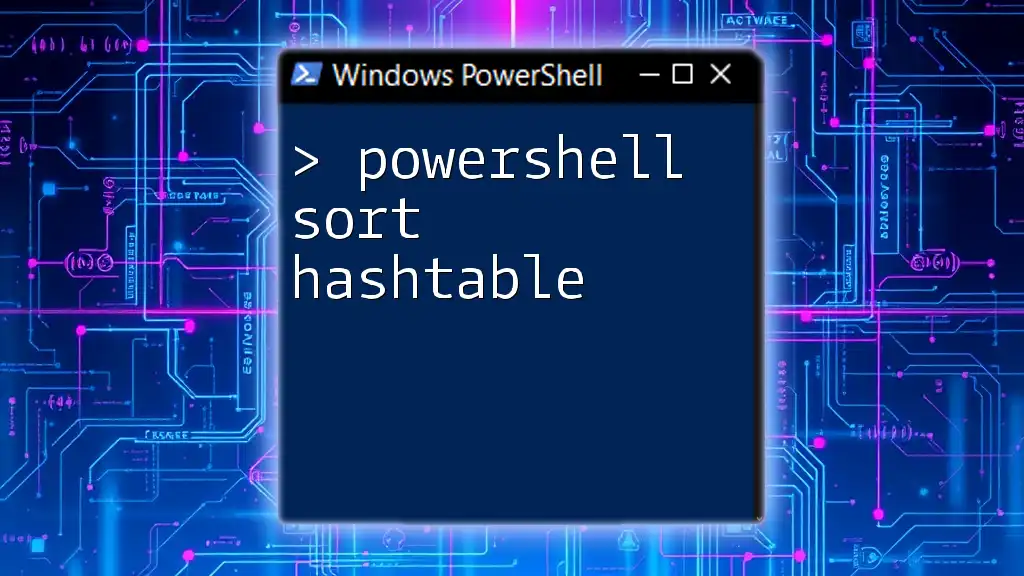
How to Create a Hashtable in PowerShell
Syntax for Creating a Hashtable
Creating a hashtable in PowerShell is straightforward. You begin with the `@{}` syntax.
Code Snippet
$myHashtable = @{}
Initializing a Hashtable with Predefined Values
You can initialize a hashtable with predefined key-value pairs. This enables you to set up your data structure with relevant data right from the start.
Code Snippet
$person = @{
Name = "John Doe"
Age = 30
Occupation = "Engineer"
}
In this snippet, the hashtable `$person` holds information about an individual, demonstrating how easy it is to store related data compactly.
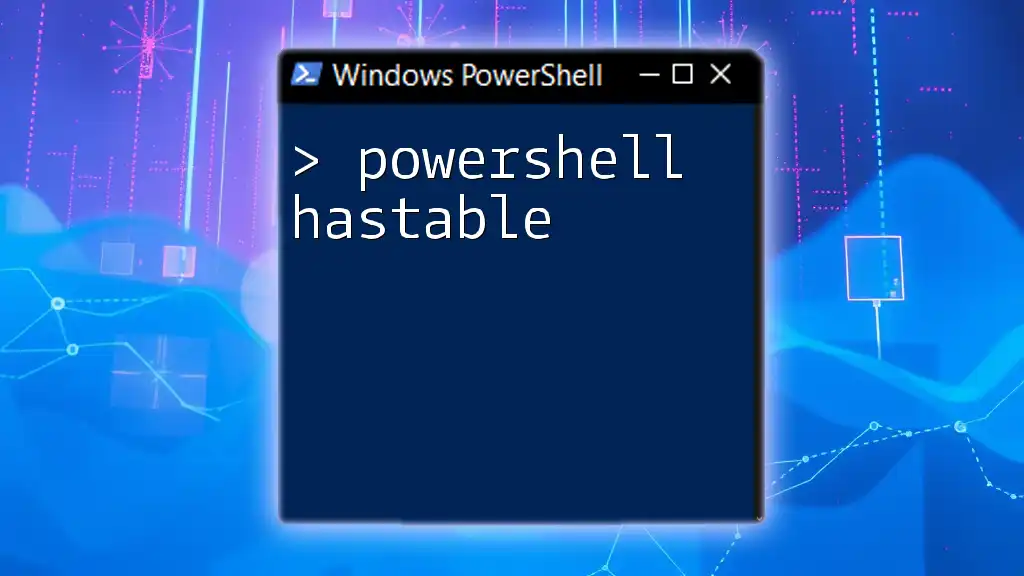
Adding Items to a Hashtable
PowerShell Hashtable Add Method
PowerShell provides a built-in `Add` method to insert new items into a hashtable. The syntax for this method requires two parameters: the key and the value.
Code Snippet
$myHashtable.Add("Key1", "Value1")
$myHashtable.Add("Key2", "Value2")
In this example, two entries are added to the hashtable `$myHashtable`. The first entry associates "Key1" with "Value1", while the second does the same for "Key2".
Using the Indexer to Add Items
In addition to the `Add` method, you can also utilize the indexer, which allows you to add items more succinctly. This method is often preferred for its clarity and efficiency.
Code Snippet
$myHashtable["Key3"] = "Value3"
The above code snippet demonstrates how to add "Key3" with the value "Value3" using the indexer. This method can be particularly useful when you need to quickly add or update values.
Overwriting Existing Keys
A critical feature of hashtables is the ability to overwrite existing keys effortlessly. If you attempt to add a key that already exists using the `Add` method, PowerShell will throw an error. However, you can update a value using the indexer.
Code Snippet
$myHashtable["Key1"] = "NewValue1" # Overwriting the value of Key1
This code snippet replaces the existing value of "Key1" with "NewValue1". It’s essential to remember this behavior when designing your hashtable logic.
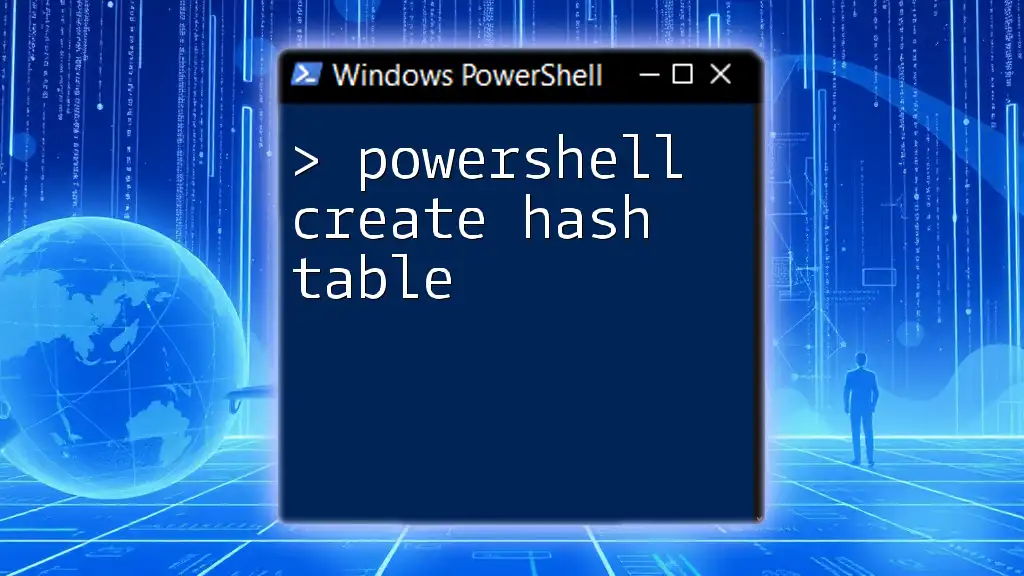
Common Use Cases for Adding to a Hashtable
Building Configuration Settings
Hashtables can efficiently manage configuration settings in your PowerShell scripts. By organizing these settings into key-value pairs, you can easily modify and retrieve configurations as needed.
Storing User Information
Consider a situation where you need to manage user data for an application. Hashtables are well-suited for this, as they can store various attributes for each user in a structured format.
Code Snippet
$userData = @{}
$userData["user1"] = @{
fullname = "Alice Smith"
email = "alice@example.com"
}
$userData["user2"] = @{
fullname = "Bob Johnson"
email = "bob@example.com"
}
In this example, the `$userData` hashtable holds multiple user records, each containing a nested hashtable of related data. This nested structure is beneficial when you need to maintain organized information.
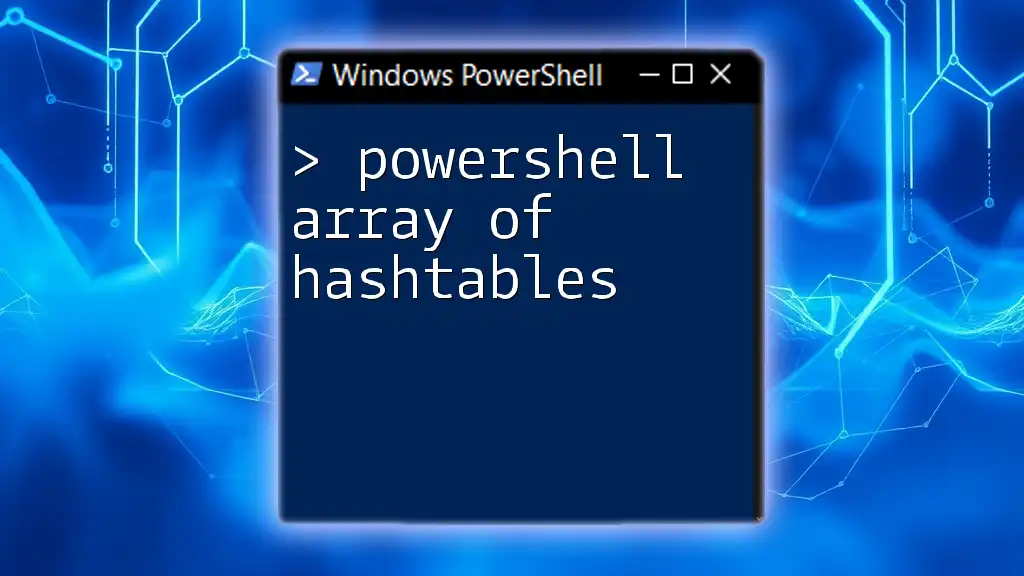
Best Practices for Working with Hashtables
Valid Key and Value Types
When working with hashtables, keep in mind that the keys must be unique and can be of various data types, including strings, numbers, or even other objects. Values can also be any data type, providing flexibility in how you store your information.
Avoiding Common Pitfalls
One common mistake is attempting to add a key that already exists in the hashtable. This will result in an error. Always check if the key exists before trying to add it or use the indexer method to update values instead.
Performance Considerations
Hashtables provide fast lookups, making them an excellent choice for managing large datasets. However, if you have performance-critical applications with huge data traffic, be mindful of the growth characteristics of hashtables to optimize performance.
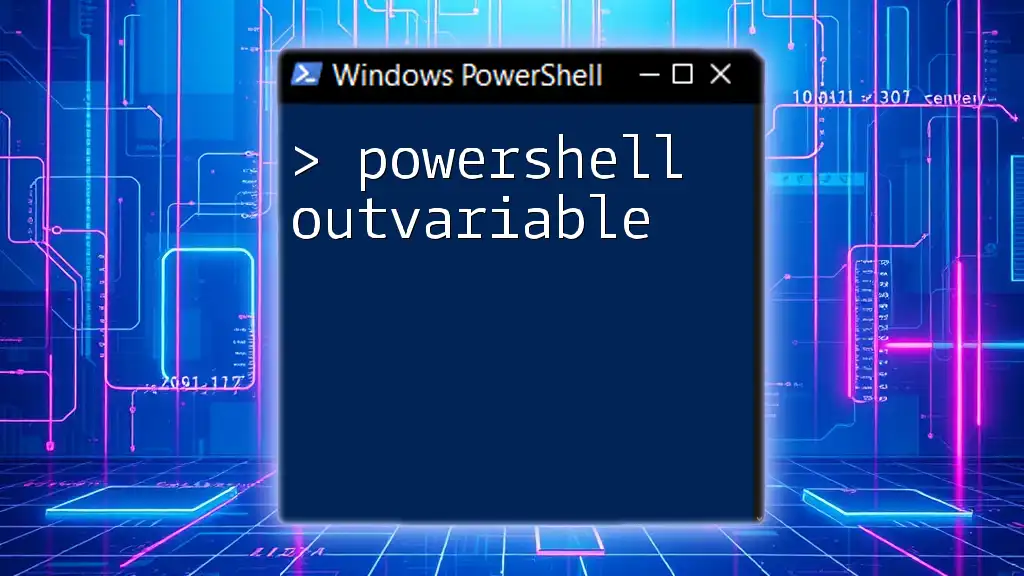
Conclusion
In this guide, we explored PowerShell's functionality for adding to hashtables. With the power they provide in organizing and managing data, mastering hashtables is a valuable skill for any PowerShell user. By applying the techniques discussed, from initialization to dynamic updates and best practices, you can enhance your scripting capabilities greatly.
With additional resources available for further learning, you are now equipped to dive deeper into the world of PowerShell and leverage hashtables effectively in your projects.