In PowerShell, an array of hashtables allows you to store multiple key-value pairs within an array, enabling efficient data management and manipulation.
$people = @(
@{ Name = 'Alice'; Age = 30 },
@{ Name = 'Bob'; Age = 25 },
@{ Name = 'Charlie'; Age = 35 }
)
Understanding Hashtables
What is a Hashtable?
A hashtable in PowerShell is a data structure that stores data in key-value pairs. Each value is associated with a unique key, allowing for rapid data access. This structure is particularly useful when you need to store related pieces of information together.
Example: You can create a simple hashtable to store a person's details:
$hashtable = @{ "Name" = "John"; "Age" = 30 }
In this example, `Name` and `Age` are the keys, and `John` and `30` are their corresponding values.
Advantages of Using Hashtables
Using hashtables offers several advantages:
- Fast Data Retrieval: Keys allow for quick access to their corresponding values without needing to traverse the entire collection.
- Flexibility in Data Types: Hashtables can contain mixed data types as keys and values, making them versatile for various applications.
- Dynamic Resizing: Unlike arrays, hashtables can dynamically change size based on how many key-value pairs you store.
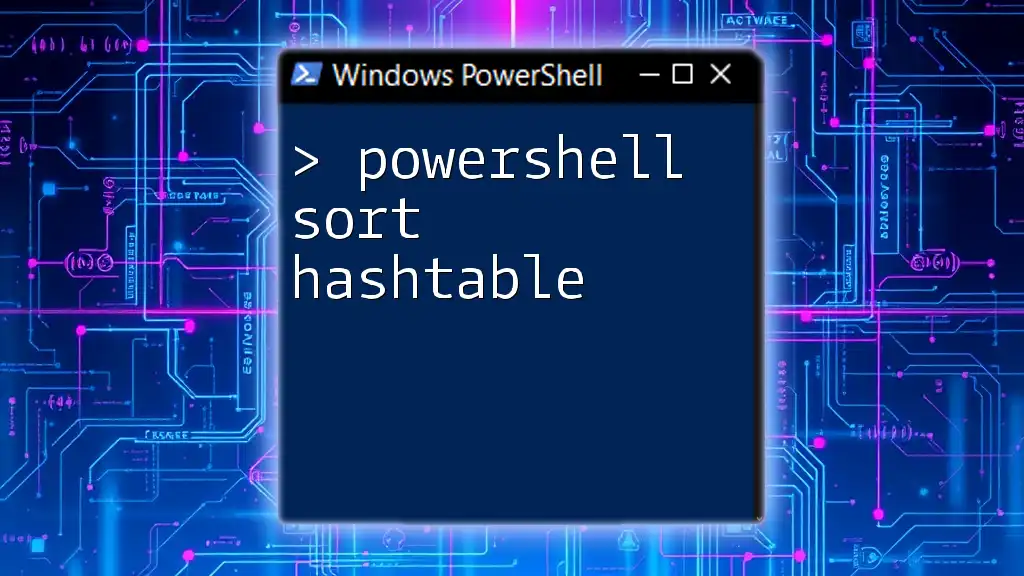
What is an Array?
Defining an Array
In PowerShell, an array is a collection that can store multiple values in a single variable. The values in an array can be of any data type and are stored in an ordered manner.
Example: You can create an array as follows:
$array = 1, 2, 3, 4, 5
This creates an array that contains five integers.
Benefits of Using Arrays
Arrays provide a number of advantages:
- Ordered Collection: The order of items is preserved, allowing you to access elements based on their position in the array.
- Accessing Elements by Index: Each element can be accessed using its index, which is efficient for processing collections of data.
- Batch Processing: Arrays are particularly well-suited for operations that involve multiple elements, such as loops and filters.
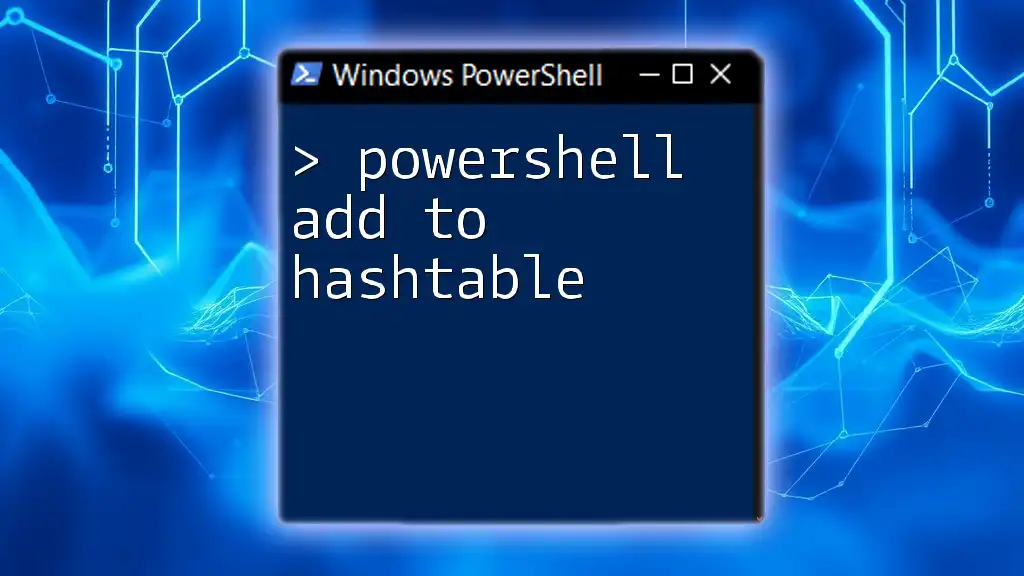
PowerShell Array of Hashtables
What is an Array of Hashtables?
An array of hashtables allows you to store multiple hashtables within a single array variable. This structure is particularly useful for managing collections of related objects where each object has various attributes.
Syntax and Structure
To create an array of hashtables, use the following syntax:
$arrayOfHashtables = @(
@{ "Name" = "John"; "Age" = 30 },
@{ "Name" = "Jane"; "Age" = 25 }
)
In this example, `$arrayOfHashtables` contains two hashtables, each representing a person with a `Name` and `Age`.
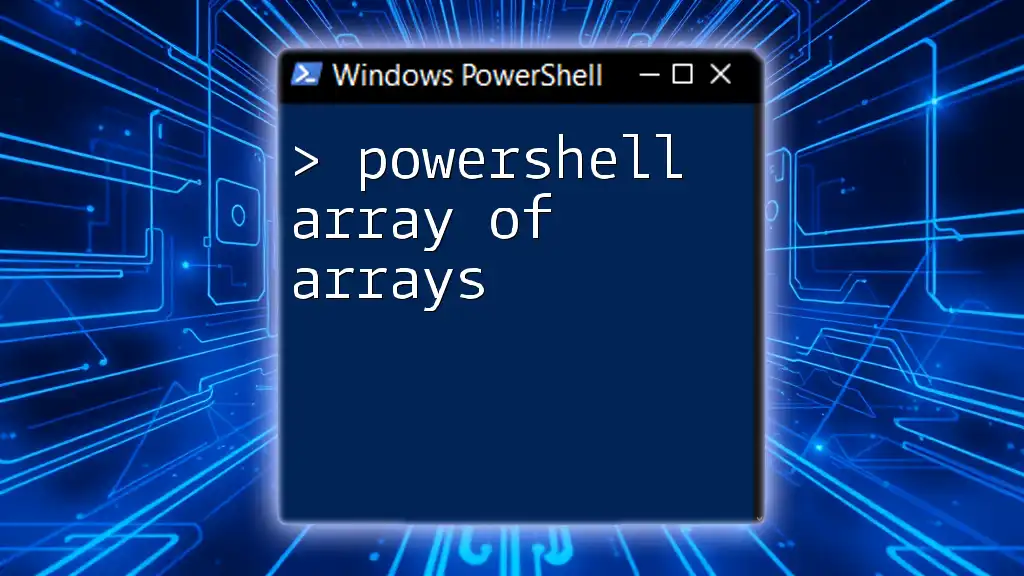
Accessing Data in an Array of Hashtables
Accessing Individual Hashtables
To access individual hashtables in the array, use their index. For example, to access the first hashtable:
$firstPerson = $arrayOfHashtables[0]
Retrieving Values from Hashtables
Once you've accessed an individual hashtable, you can retrieve values using their keys. For instance, to get the `Name` from the first hashtable:
$firstPersonName = $firstPerson["Name"]
This will assign the value `John` to the variable `$firstPersonName`.
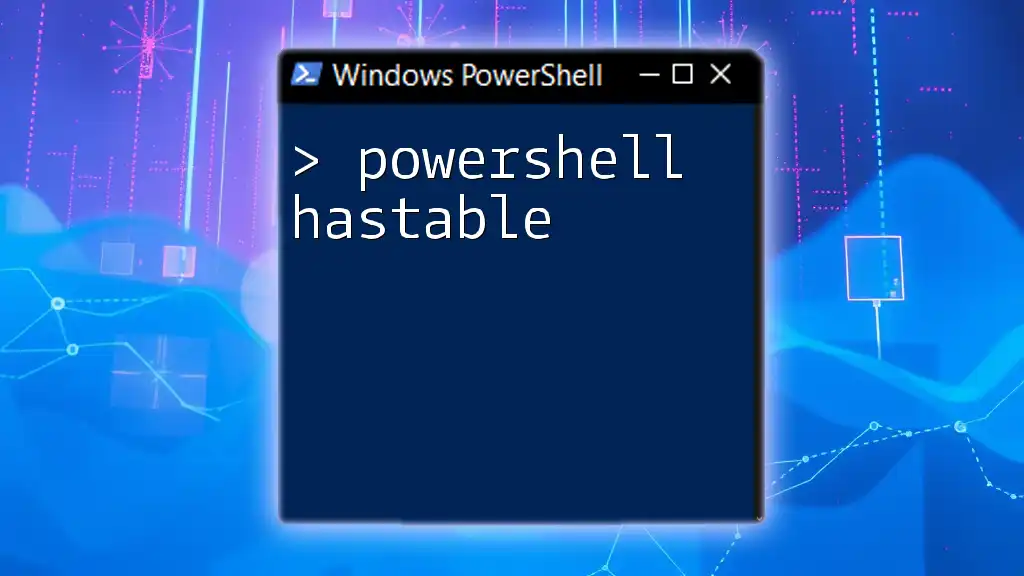
Looping Through an Array of Hashtables
Using ForEach Loop
A ForEach loop allows you to iterate through each hashtable in the array efficiently. Here’s how you can print out the names and ages of each person:
foreach ($person in $arrayOfHashtables) {
Write-Output "Name: $($person['Name']), Age: $($person['Age'])"
}
Using Other Loop Types
Besides the ForEach loop, you can use other loops, like the For loop, to iterate through the array. Here’s an example:
for ($i = 0; $i -lt $arrayOfHashtables.Count; $i++) {
Write-Output $arrayOfHashtables[$i]
}
This loop will output each hashtable in the array.
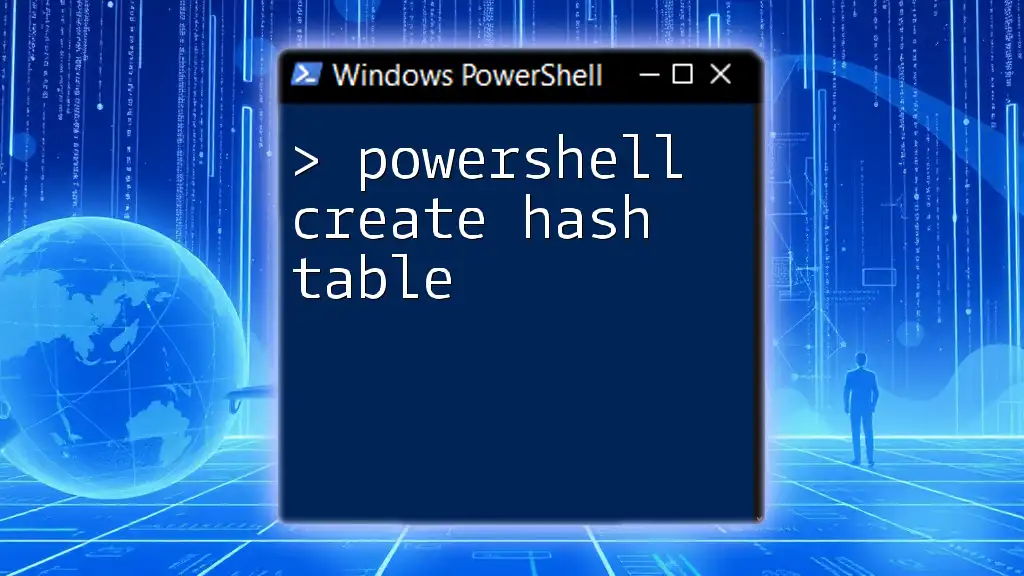
Modifying Hashtables in an Array
Adding New Hashtables
You can append a new hashtable to an existing array like so:
$arrayOfHashtables += @{ "Name" = "Doe"; "Age" = 40 }
This action dynamically expands the array, allowing you to add more data as needed.
Updating Values in a Hashtable
To update existing values in a hashtable, simply access the specific hashtable by its index and then specify the key to change. For example, to update the age of the first person:
$arrayOfHashtables[0]["Age"] = 31
This modifies the existing hashtable to reflect the new age.
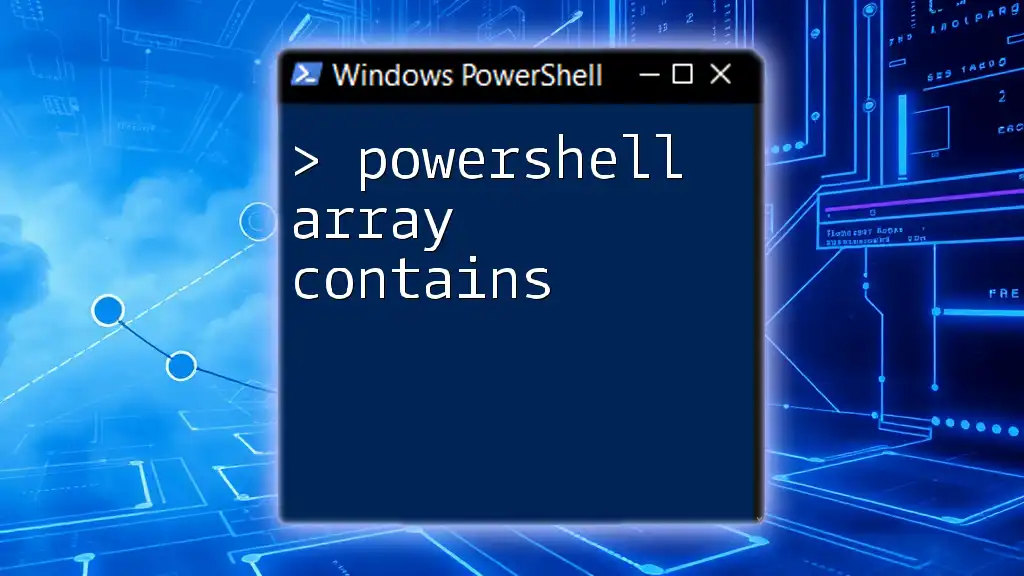
Common Use Cases
Example: Storing Configuration Data
An array of hashtables is an excellent way to store configuration data for applications. Each hashtable can represent settings for a different application.
Example: Defining configuration data can look like this:
$configurations = @(
@{ "AppName" = "App1"; "Version" = "1.0" },
@{ "AppName" = "App2"; "Version" = "1.1" }
)
Example: Collecting User Information
If you want to collect dynamic user data, an array of hashtables can be useful.
Example: Here’s how to prompt users for their information and store it:
$users = @()
for ($i = 0; $i -lt 3; $i++) {
$name = Read-Host "Enter Name"
$age = Read-Host "Enter Age"
$users += @{ "Name" = $name; "Age" = $age }
}
In this scenario, the script will collect the name and age from three users and store each as a hashtable in the `$users` array.
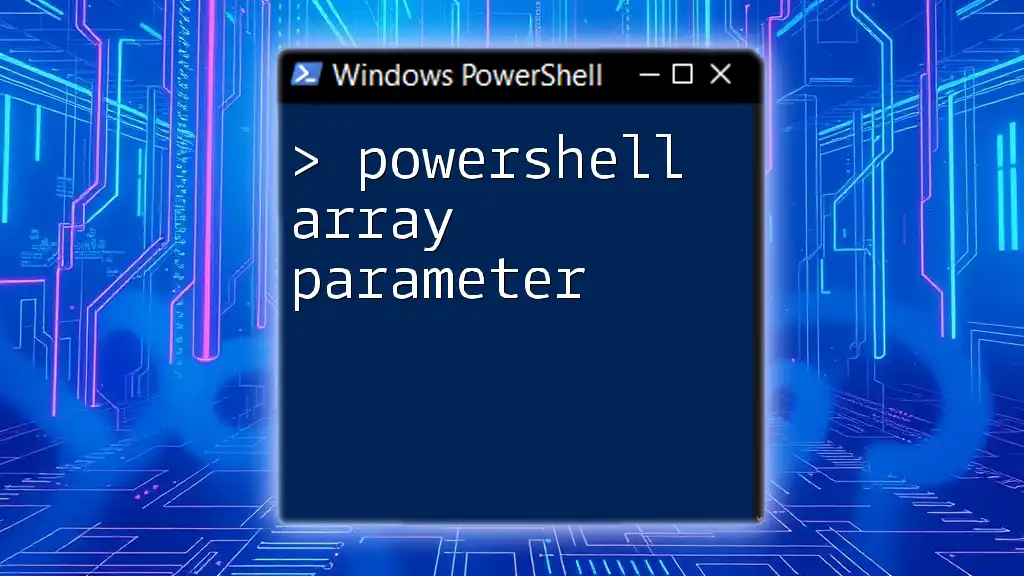
Conclusion
The concept of a PowerShell array of hashtables is a powerful technique that facilitates the organization and management of complex data structures. By mastering this technique to create, access, and manipulate arrays of hashtables, you can enhance your PowerShell scripting capabilities, better organize your data, and ultimately streamline your workflows. Don't hesitate to experiment with the examples provided, as practical implementation is key to mastering PowerShell!