To convert an array to a string in PowerShell, you can use the `-join` operator, which concatenates the elements of the array into a single string.
$array = @('Hello', 'World', 'from', 'PowerShell')
$string = $array -join ' '
Write-Host $string
Understanding PowerShell Arrays
What is an Array in PowerShell?
In PowerShell, an array is a collection of items, which can be of any type (strings, numbers, objects, etc.). Arrays are very useful in scripting as they allow you to group related data together and manipulate them as a single entity. Some key characteristics include:
- Zero-based indexing: The first element in an array is accessed with index 0.
- Dynamic sizing: You can add or remove elements from an array without defining its size in advance.
Common Use Cases for Arrays
Arrays are invaluable in a variety of situations, such as:
- Data collection: They can hold user names, file paths, or any list of items you may need to process.
- Storing command results: Arrays can efficiently store outputs from executed commands for further manipulation.
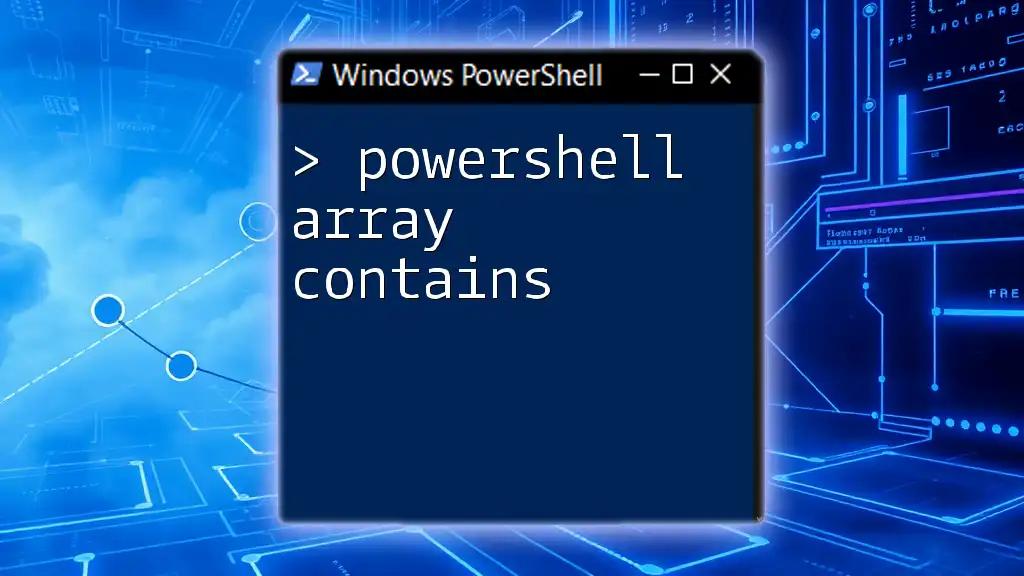
PowerShell Convert Array to String
Why Convert an Array to a String?
Converting an array to a string is often necessary in scenarios like:
- Logging and Displaying Data: Cleanly formatting output for logs or user interface displays.
- Concatenation: Joining array elements into a single string for easier data handling or output formatting.
- Writing to Files: Saving string representations of data for reporting or exporting.
Basic Methods to Convert Array to String
Using `-join` Operator
One of the simplest and most efficient ways to convert an array to a string in PowerShell is to use the `-join` operator. This operator allows you to specify a separator between each element:
$array = @("Apple", "Banana", "Cherry")
$string = $array -join ", "
Write-Host $string # Output: Apple, Banana, Cherry
In this example, each element is joined by a comma and a space, turning the array into a single, readable string. This method is particularly fast and straightforward.
Using `ForEach-Object` Cmdlet
Another method of converting an array into a string is by using the `ForEach-Object` cmdlet. Although slightly more verbose, it can provide more flexibility in certain situations:
$array = @("One", "Two", "Three")
$string = $array | ForEach-Object { $_ } -join " "
Write-Host $string # Output: One Two Three
In this code snippet, the array is piped into `ForEach-Object`, which processes each item. After processing, we use the `-join` operator to create a single string.
Advanced Methods for Conversion
String Interpolation
PowerShell allows you to embed variables directly within strings, known as string interpolation. This method can be quite handy:
$array = @("red", "green", "blue")
$string = "$($array -join ', ') are colors."
Write-Host $string # Output: red, green, blue are colors.
This technique not only converts the array but also allows you to style the output with additional text, making it a powerful tool in your scripting arsenal.
Using `Out-String` Cmdlet
The `Out-String` cmdlet converts a collection of objects into strings. Although not primarily designed for array-to-string conversions, it can work well in specific situations, especially if the objects have a specific string representation:
$array = @("Line1", "Line2", "Line3")
$string = $array | Out-String
Write-Host $string
This method is useful for maintaining formatting when dealing with complex object types.
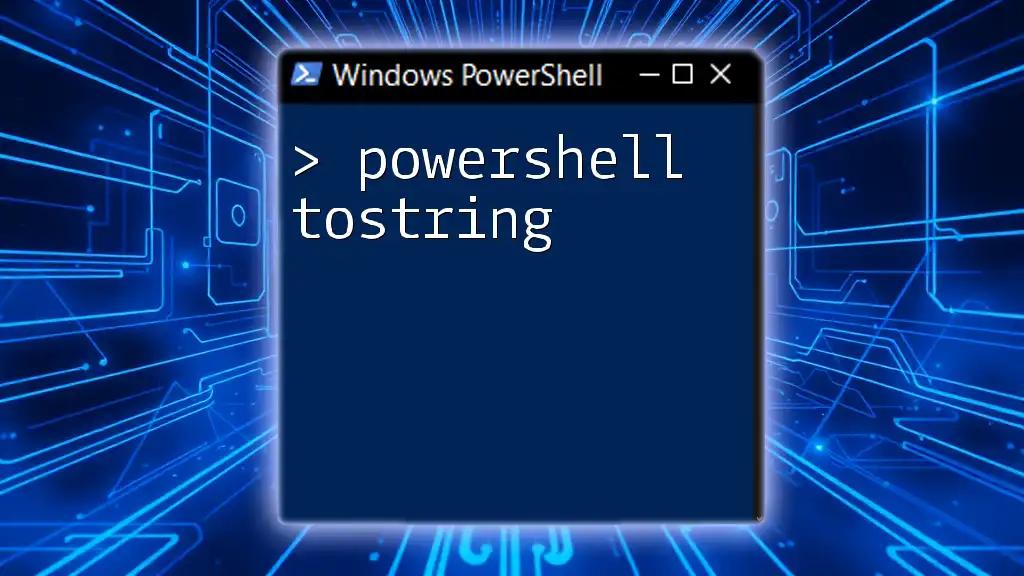
Handling Delimiters in String Conversion
Custom Delimiters
When converting arrays to strings, the choice of delimiter can significantly impact the readability of the result. You can easily define custom delimiters as seen below:
$array = @("Item1", "Item2", "Item3")
$string = $array -join " | "
Write-Host $string # Output: Item1 | Item2 | Item3
In this example, we used a pipe (`|`) as the delimiter, which may improve clarity in certain contexts, such as logs or reports.
Concatenating with Additional Text
You can enhance the string representation by adding text before and after the converted array. Here’s how you can do that:
$array = @("First", "Second", "Third")
$string = "Items: " + ($array -join ", ")
Write-Host $string # Output: Items: First, Second, Third
This method is practical for situations where you need to label data outputs clearly.

Converting Multidimensional Arrays to Strings
Explanation of Multidimensional Arrays
Multidimensional arrays in PowerShell allow you to represent tabular data structures, making them suitable for scenarios involving grids or matrices.
Strategies for Conversion
To convert a multidimensional array to a string effectively, you can flatten it using a combination of looping structures:
$multiArray = @(@("Row1Col1", "Row1Col2"), @("Row2Col1", "Row2Col2"))
$string = ($multiArray | ForEach-Object { $_ -join ", " }) -join " | "
Write-Host $string # Output: Row1Col1, Row1Col2 | Row2Col1, Row2Col2
In this case, each sub-array is joined into a string, and then those strings are joined together with a different separator, resulting in a well-formatted string representation of the multidimensional array.
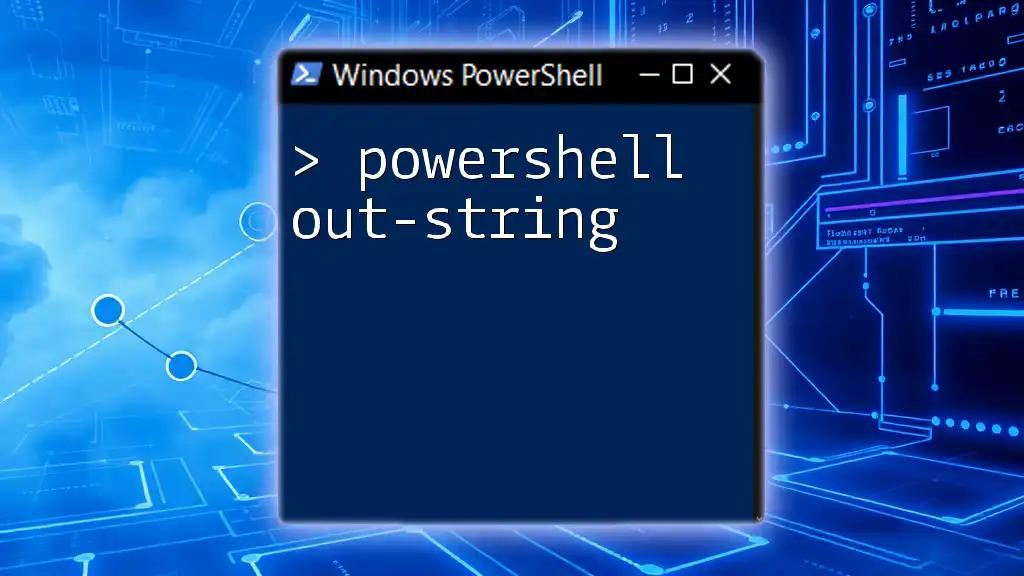
Error Handling During Conversion
Common Conversion Errors
When working with arrays, you may encounter issues such as null values or empty arrays. These common pitfalls can generate errors when you're attempting to join or manipulate them.
Using Try-Catch for Robust Scripts
It's always wise to incorporate error handling in your scripts. Utilizing `try-catch` blocks ensures that your script handles unexpected situations gracefully:
try {
$array = @() # Empty array
$string = $array -join ", "
} catch {
Write-Host "An error occurred: $_"
}
With this error handling, if the array is empty, the script provides a clear error message instead of crashing or producing unexpected results.

Conclusion
Converting a PowerShell array to a string is a fundamental skill that enhances your scripting efficiency and output clarity. From the basic `-join` operator to advanced strategies like string interpolation and custom delimiters, a variety of methods exist to tailor the conversion to your specific needs. Don't hesitate to experiment with different techniques and utilize error handling to create resilient scripts. With practice, you'll find the best approach for any scenario!