In PowerShell, you can check if an array contains a specific value using the `-contains` operator, as shown in the following code snippet:
$array = 1, 2, 3, 4, 5
if ($array -contains 3) { Write-Host 'Array contains the value 3' }
Understanding Arrays in PowerShell
What is an Array?
In PowerShell, an array is a collection of items that can be of any data type. It allows you to store multiple values in a single variable. Arrays are incredibly useful for organizing and managing data efficiently. For example, you can create simple arrays to hold lists of names, numbers, or other types of objects.
Here is a basic example of defining an array in PowerShell:
$fruits = @('Apple', 'Banana', 'Cherry')
This array, `$fruits`, contains three strings: Apple, Banana, and Cherry.
Array Syntax
Accessing elements in an array is straightforward. You can reference an array by its index, which starts at 0. For instance, to obtain the first fruit in the array, you can use:
$firstFruit = $fruits[0]
This means `$firstFruit` will hold the value Apple.
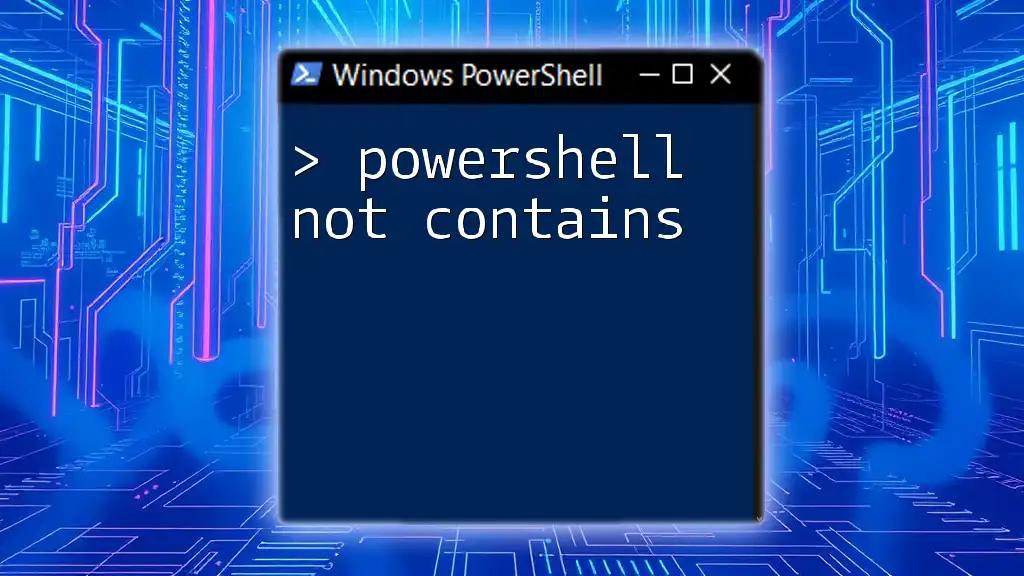
PowerShell Check if String is in Array
Using `-contains` Operator
When you need to check if a specific string exists in an array, the `-contains` operator offers a simple solution. It returns a Boolean value: `True` if the element is found, and `False` otherwise.
Here’s an example:
if ($fruits -contains 'Banana') {
Write-Host "The array contains Banana."
}
In this case, "The array contains Banana." will be printed because Banana is indeed one of the elements in the `$fruits` array.
Understanding Case Sensitivity
It is crucial to note that the `-contains` operator is case-sensitive. This means that a check for a lowercase string will not match its uppercase counterpart. Consider this example:
if ($fruits -contains 'banana') {
Write-Host "The array contains Banana."
} else {
Write-Host "The check is case-sensitive: it does not contain 'banana'."
}
The output will communicate that the array does not contain 'banana', as PowerShell distinguishes between Banana and banana.
Using `-like` Operator for Partial Matches
For scenarios where you want to check for partial matches or use wildcards, the `-like` operator can be a suitable alternative. This operator allows you to find strings that match a specified pattern. Here’s an example of how it works:
if ($fruits -like '*nana*') {
Write-Host "There is a fruit that contains 'nana'."
}
In this instance, the output will confirm that there is a fruit with 'nana', which is Banana.
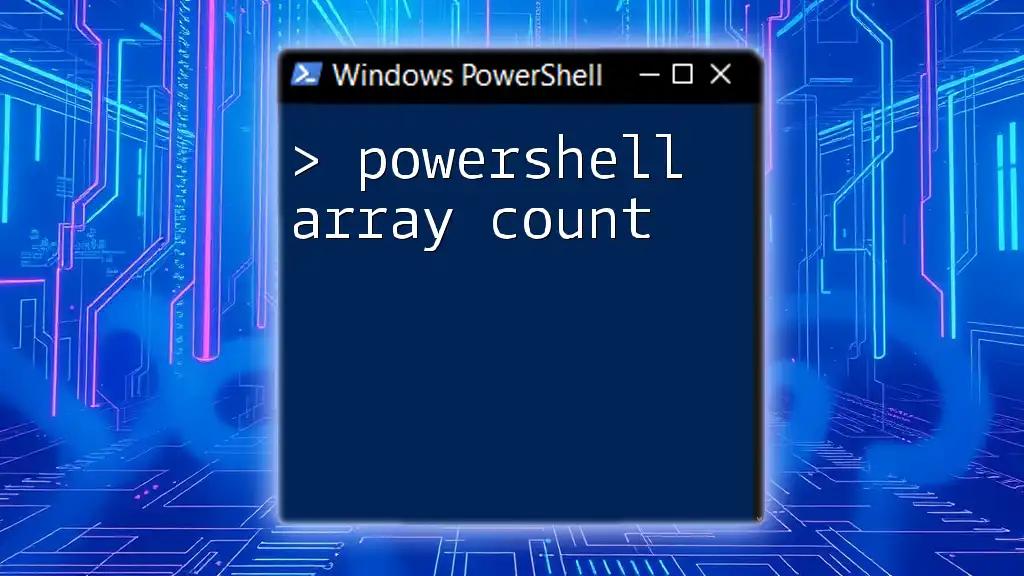
PowerShell If in Array
Simplified Conditional Statements
The integration of `if` statements with array checks enables logical controls in your scripts. Here's how you can implement a check for a fruit:
$checkFruit = 'Grape'
if ($fruits -contains $checkFruit) {
Write-Host "$checkFruit is found in the array."
} else {
Write-Host "$checkFruit is not found in the array."
}
If Grape is not present in the `$fruits` array, the output will state "Grape is not found in the array."
Looping Through Array for More Complex Checks
In cases where your logic requires evaluating multiple elements, a loop can be beneficial. The following example uses `foreach` to iterate through each item in the array:
foreach ($fruit in $fruits) {
if ($fruit -eq 'Cherry') {
Write-Host "Cherry is in the array."
}
}
This loop will print "Cherry is in the array." if Cherry exists in the array.
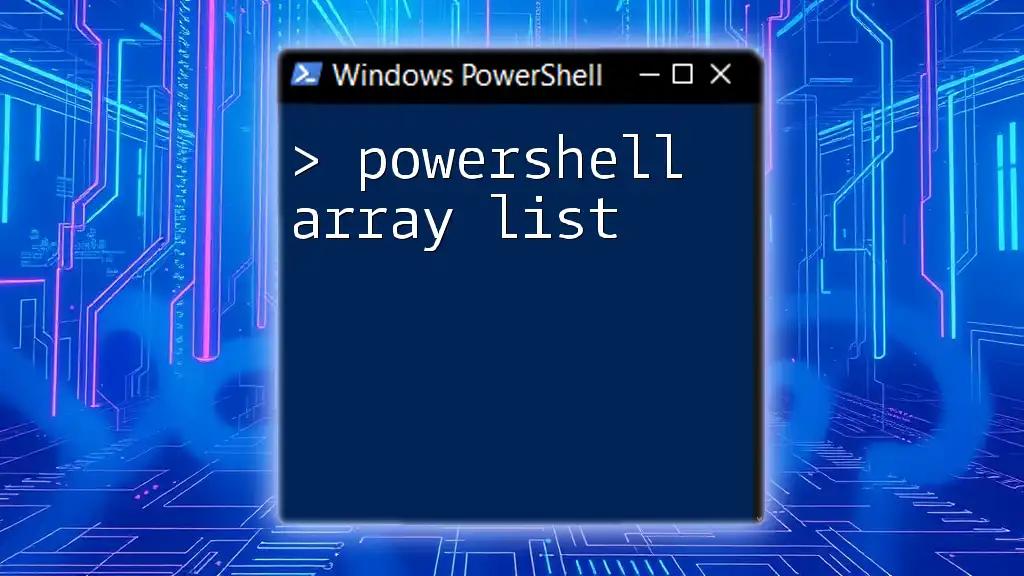
PowerShell If Array Contains
Using the `Where-Object` Cmdlet
The `Where-Object` cmdlet can provide a flexible approach for filtering based on conditions. If you want to verify the presence of an element, utilize it as shown below:
$containsCherry = $fruits | Where-Object { $_ -eq 'Cherry' }
if ($containsCherry) {
Write-Host "Cherry found in the array using Where-Object."
}
Here, the code checks if Cherry exists. If it does, it outputs a confirmation message.
Combining with Multiple Conditions
There may be times when you need to check for multiple elements at the same time. Logical operators such as `-and` or `-or` can facilitate these checks. For instance:
if (($fruits -contains 'Apple') -and ($fruits -contains 'Banana')) {
Write-Host "The array contains both Apple and Banana."
}
This condition will print the statement if both Apple and Banana are indeed present in the array.

PowerShell Check if Array Contains String
Checks on Multi-dimensional Arrays
PowerShell allows you to create multi-dimensional arrays, which can hold more intricate data structures. When checking values within these arrays, utilize similar methods as before. For example:
$multiArray = @(@('A', 'B'), @('C', 'D'))
if ($multiArray -contains 'C') {
Write-Host "C is in the multi-dimensional array."
}
The code above confirms that C exists in the `$multiArray`.
Using Custom Functions for Array Checks
To streamline your checks and make your code cleaner, consider creating custom functions. This approach enhances reusability across your scripts. Here’s a simple function you can write:
function Contains-Item {
param (
[string[]]$array,
[string]$item
)
return $array -contains $item
}
Using the function:
if (Contains-Item $fruits 'Apple') {
Write-Host "Apple is in the array."
}
This structure allows you to quickly verify the presence of an item in any array with just one function call.

PowerShell Contains Array
Creating and Checking Against Custom Arrays
Creating dynamic arrays can be a versatile approach when the data is generated or modified during execution. You can add items to arrays flexibly, as shown here:
$dynamicFruits = @()
$dynamicFruits += 'Apple'
$dynamicFruits += 'Banana'
As you can observe, `$dynamicFruits` now contains Apple and Banana. You can then apply the checks discussed previously.
Summary of Methods for Checking Array Contents
Throughout this article, we’ve explored numerous methods to check if a PowerShell array contains a specific value. With the `-contains` operator for direct checks, `Where-Object` for filtered conditions, and custom functions for reusability, you're equipped to handle various scenarios efficiently.

Conclusion
Understanding how to check if an array contains specific values is fundamental for effective scripting and data management in PowerShell. By mastering these techniques, you can enhance your script's logic and data handling capabilities, ensuring better performance and accuracy. Practice utilizing different methods discussed here to deepen your grasp of PowerShell array operations.