A PowerShell array list is a dynamic collection that allows you to store and manipulate a list of items efficiently, and you can create one using the following code snippet:
$arrayList = New-Object System.Collections.ArrayList
$arrayList.Add("Item1")
$arrayList.Add("Item2")
$arrayList
Understanding Array Lists in PowerShell
What is an Array List?
An array list is a flexible, dynamic data structure provided by PowerShell through the .NET Framework. Unlike traditional arrays, which have a fixed size, array lists can grow and shrink as needed. This inherent flexibility makes them particularly useful for scenarios where the number of elements fluctuates.
Key features of array lists include:
- Dynamic Resizing: You can add or remove elements without needing to define the size beforehand.
- Versatility: Array lists can store different data types within a single list, unlike standard arrays, which are typically homogeneous.
Why Use Array Lists in PowerShell?
Using array lists in PowerShell offers several advantages:
- Dynamic Resizing: As mentioned, array lists adjust their size dynamically, meaning you’re not restricted by a predetermined number of elements.
- Performance Benefits: For large datasets, array lists outperform traditional arrays when it comes to adding and removing elements, making them a practical choice for many PowerShell scripts.
- Real-World Use Cases: Imagine needing to store temporary results from commands where the number of results can vary; array lists can handle this variability effectively.
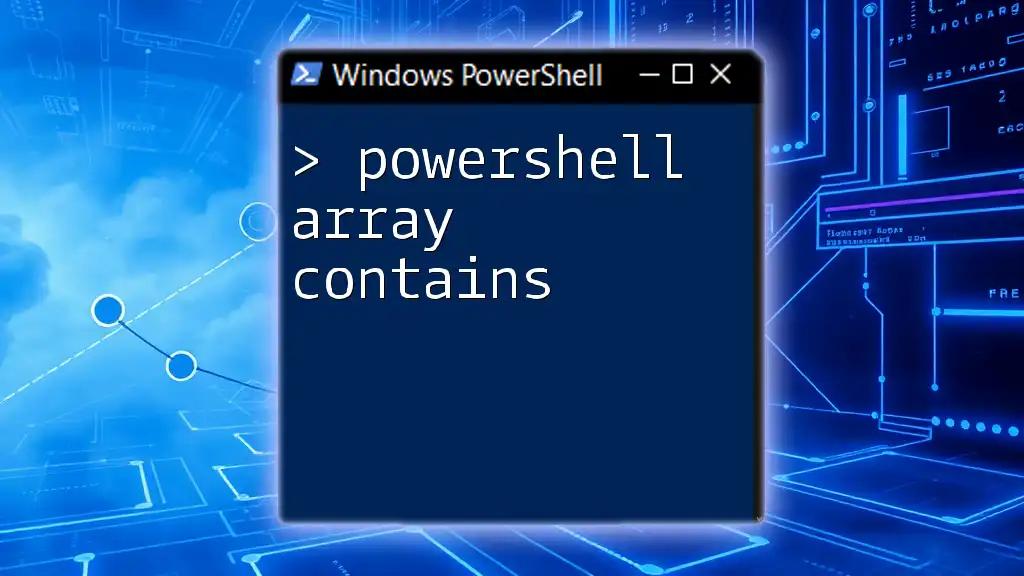
Getting Started with Array Lists
Creating an Array List
To create an array list in PowerShell, you utilize the `System.Collections.ArrayList` class. Here’s how you do it:
$arrayList = New-Object System.Collections.ArrayList
This line initializes a new instance of an array list, ready for you to add items.
Adding Elements to an Array List
Populating your array list is straightforward. Use the `.Add()` method to insert items:
$arrayList.Add("First Item")
$arrayList.Add("Second Item")
In this case, the array list now contains two elements, "First Item" and "Second Item."
Accessing Elements in an Array List
Accessing elements in an array list is similar to accessing them in an array, utilizing an index. For example:
$firstItem = $arrayList[0]
Here, `$firstItem` will hold the string "First Item."
Removing Elements from an Array List
Removing elements can be done using either the `.Remove()` or `.RemoveAt()` methods. For instance:
$arrayList.Remove("First Item")
$arrayList.RemoveAt(0)
The first line removes "First Item", while the second removes the first item in the list which may be "Second Item" if it hasn't changed.
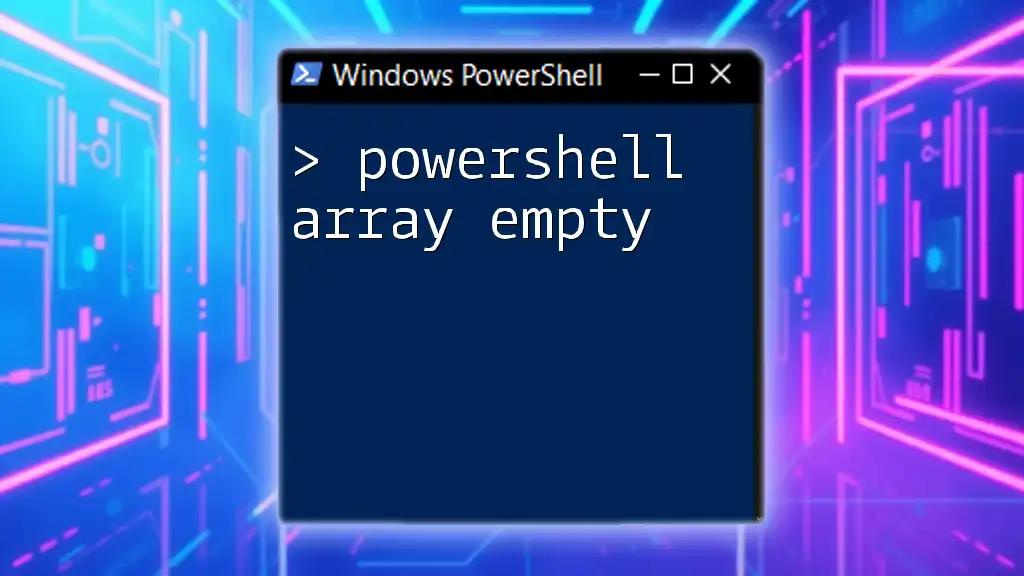
Manipulating Array Lists
Iterating Over an Array List
You can easily loop through each item in your array list using a `foreach` loop. Here’s how:
foreach ($item in $arrayList) {
Write-Host $item
}
This code snippet prints each item in the array list to the console.
Sorting an Array List
Array lists can be sorted using the `.Sort()` method, which arranges the items in a natural order:
$arrayList.Sort()
After executing this, your array list will be ordered accordingly.
Clearing an Array List
If you need to wipe the list clean, you can use the `.Clear()` method. This effectively empties the array list:
$arrayList.Clear()
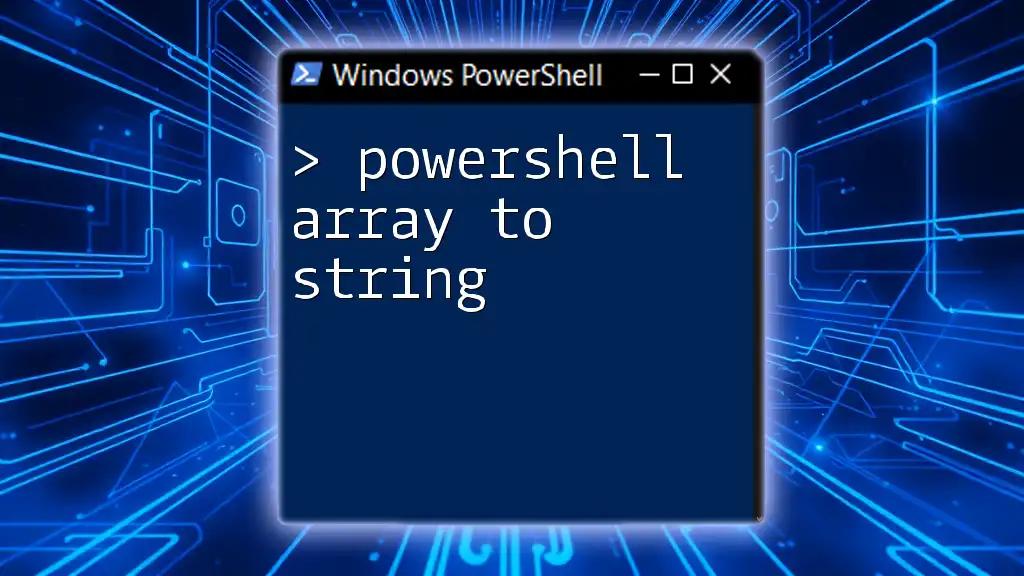
Advanced Array List Techniques
Searching for Elements in an Array List
Finding specific elements is easy with methods like `.Contains()` and `.IndexOf()`. Here is an example using `.Contains()`:
if ($arrayList.Contains("Second Item")) {
Write-Host "Item found!"
}
This checks if "Second Item" exists in the list and prints a message accordingly.
Converting an Array List to a Regular Array
If you need a regular array instead of an array list, PowerShell allows you to convert it seamlessly:
$array = @($arrayList)
This casts the array list into a standard array for you to utilize as needed.
Nesting Array Lists
You can even nest array lists for more complex data structures. Here’s how you can create a multi-dimensional array list:
$nestedList = New-Object System.Collections.ArrayList
$nestedList.Add((New-Object System.Collections.ArrayList))
This sets the stage for managing collections that contain other collections.
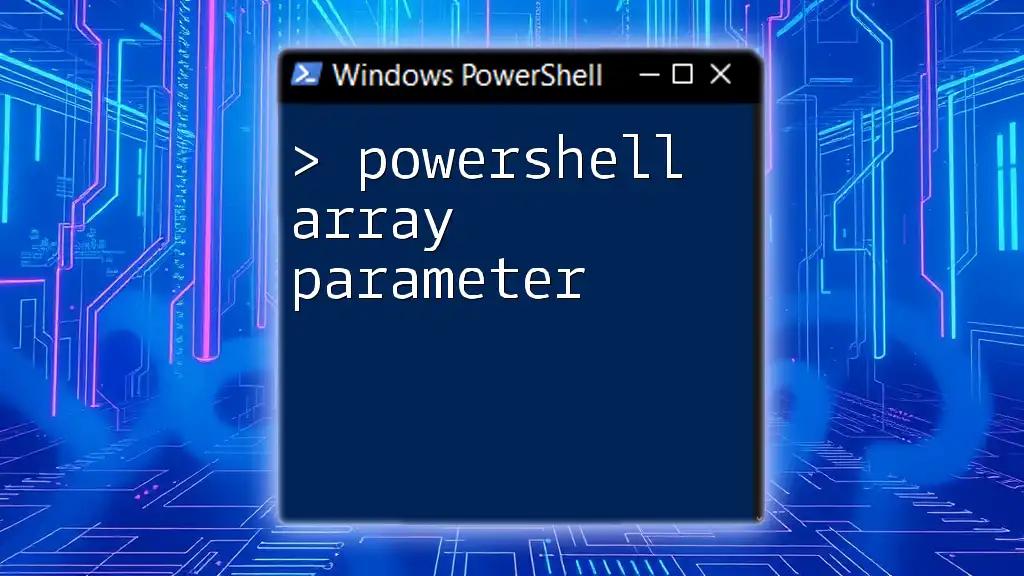
Best Practices for Using Array Lists in PowerShell
When to Use Array Lists Over Arrays
Deciding between using an array or an array list may depend on your specific needs:
- If you expect a fluctuating number of elements, opt for an array list.
- For stable datasets with a fixed size, using a standard array might improve efficiency and clarity.
Common Pitfalls to Avoid
Be mindful of index out of range errors, which can occur if you try to access or remove an item that doesn’t exist. Additionally, always consider memory management; shrinking large array lists may lead to fragmentation. Use the `.TrimToSize()` method if necessary.
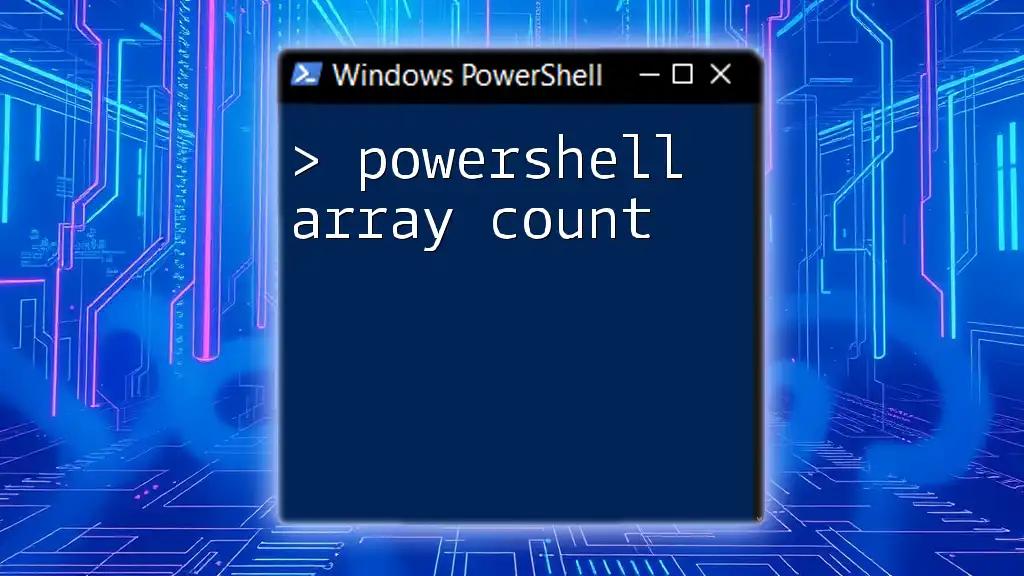
Conclusion
Recap of Key Points
In summary, understanding the capabilities of the PowerShell array list is essential for effective scripting. The dynamic nature of array lists enhances script performance and flexibility, particularly when handling varying amounts of data.
Further Learning Resources
To deepen your knowledge of PowerShell array lists, consider exploring official Microsoft documentation, online tutorials, and engaging with community forums. Continuous learning will help you harness the full potential of PowerShell in your projects.