You can easily count the number of elements in a PowerShell array by using the `.Count` property, as shown in the following code snippet:
$array = @(1, 2, 3, 4, 5)
$count = $array.Count
Write-Host "The array contains $count elements."
Understanding PowerShell Arrays
What is an Array?
An array is a fundamental data structure used in programming to store a collection of variables. With arrays, you can group multiple items together, allowing for efficient management and manipulation of these items in scripts. In PowerShell, arrays provide a convenient way to handle grouped data and enable automation for various tasks.
Creating a PowerShell Array
To create an array in PowerShell, you can leverage a simple syntax that allows you to define multiple values at once. For instance, the following code initializes an array containing several integers:
$myArray = 1, 2, 3, 4
In this example, `$myArray` contains four elements: 1, 2, 3, and 4. Each element can be of varying data types, such as strings, integers, or even objects.
Types of Arrays in PowerShell
PowerShell supports different types of arrays for various use cases:
Single-Dimensional Arrays are the most common type and hold a linear collection of elements, suitable for tasks where you need a simple list of items.
Multi-Dimensional Arrays, on the other hand, allow you to store data in a tabular format (rows and columns). You can create a multi-dimensional array like this:
$multiArray = @( @(1, 2), @(3, 4) )
In this case, `$multiArray` contains two rows of two columns, which can be especially useful for complex datasets.
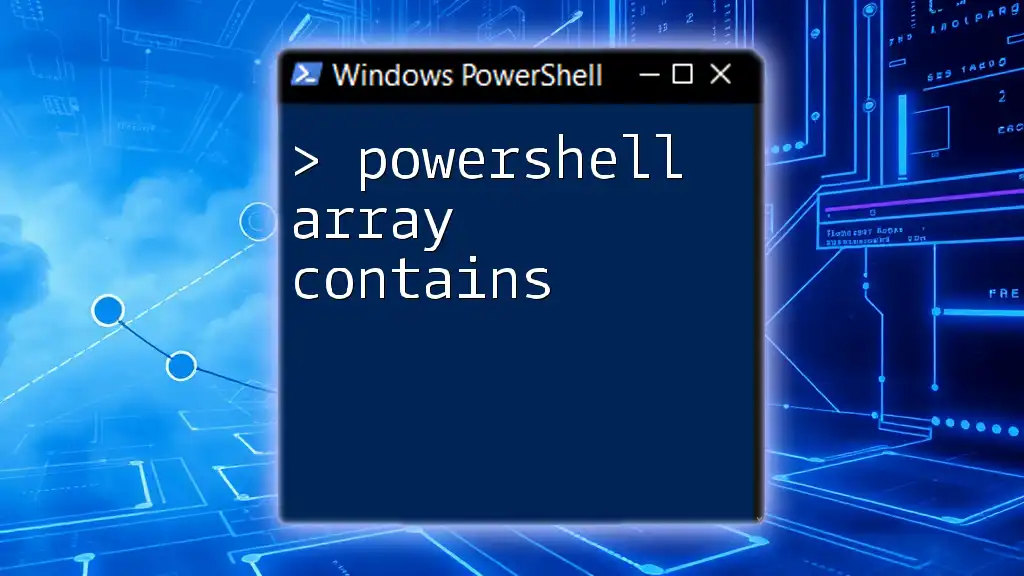
Counting Elements in a PowerShell Array
Using the .Length Property
Counting the elements in a PowerShell array can be achieved using the `.Length` property. This property returns the total number of elements in the array. Here's how you can implement it:
$myArray = 1, 2, 3, 4
$count = $myArray.Length
Write-Output "Count of elements: $count"
When executed, this script will output:
Count of elements: 4
This method is straightforward and widely used in various scripting scenarios.
Using the Count Property for Collections
For collections like `ArrayList`, you can utilize the `.Count` property, which functions similarly to `.Length` but is specific to those collection types. An example is shown below:
$myArrayList = New-Object System.Collections.ArrayList
$myArrayList.Add(1)
$myArrayList.Add(2)
$count = $myArrayList.Count
Write-Output "Count of elements in ArrayList: $count"
This will yield:
Count of elements in ArrayList: 2
Understanding both properties enables you to manage your data structures effectively in scripts.
Counting Specific Types of Elements
If you need to count specific types of elements within an array, you can utilize PowerShell's pipeline and filtering capabilities. For example, if you wish to count how many times "apple" appears in an array, you can do so as follows:
$mixedArray = "apple", "banana", 34, "apple", "pear"
$appleCount = ($mixedArray | Where-Object {$_ -eq "apple"}).Count
Write-Output "Count of apple: $appleCount"
The output will display:
Count of apple: 2
This approach demonstrates how to filter elements dynamically and count them in real-time.
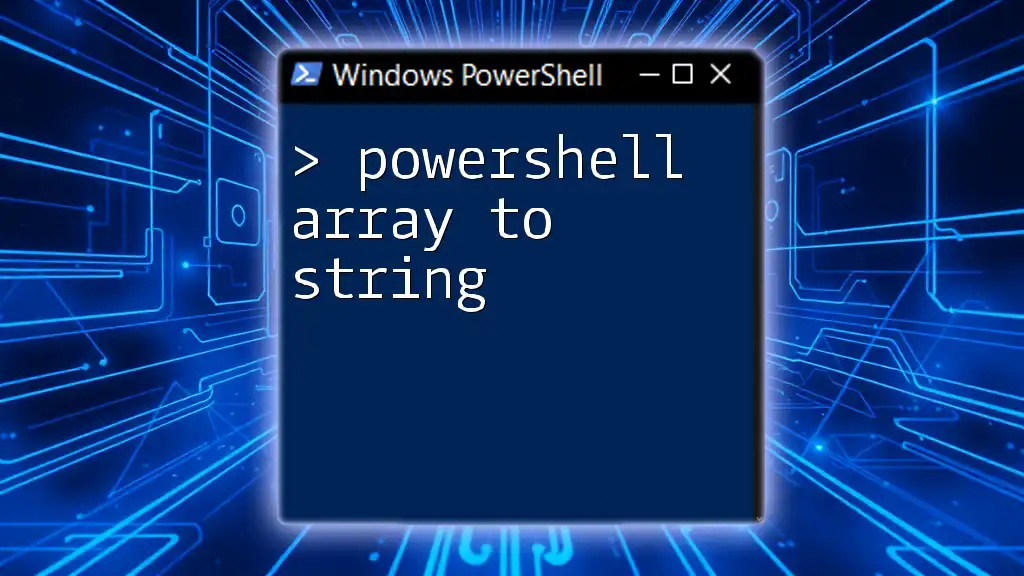
Practical Applications and Use Cases
Automating Tasks with Array Counts
Utilizing PowerShell array count can dramatically simplify the automation of repetitive tasks. For instance, you might use an array to hold a list of user accounts for processing, and knowing how many accounts you have can help you better control your automation workflow.
Consider a scenario where you want to inform an administrator about the number of users needing updates. Instead of manually counting, you can leverage the previously discussed methods to automate such notifications.
Performance Considerations
When managing large arrays, performance can become an issue. The method you choose for counting elements may affect execution time. For larger datasets, prefer properties like `.Length` over counting via filtering, as the latter may slow down the script.
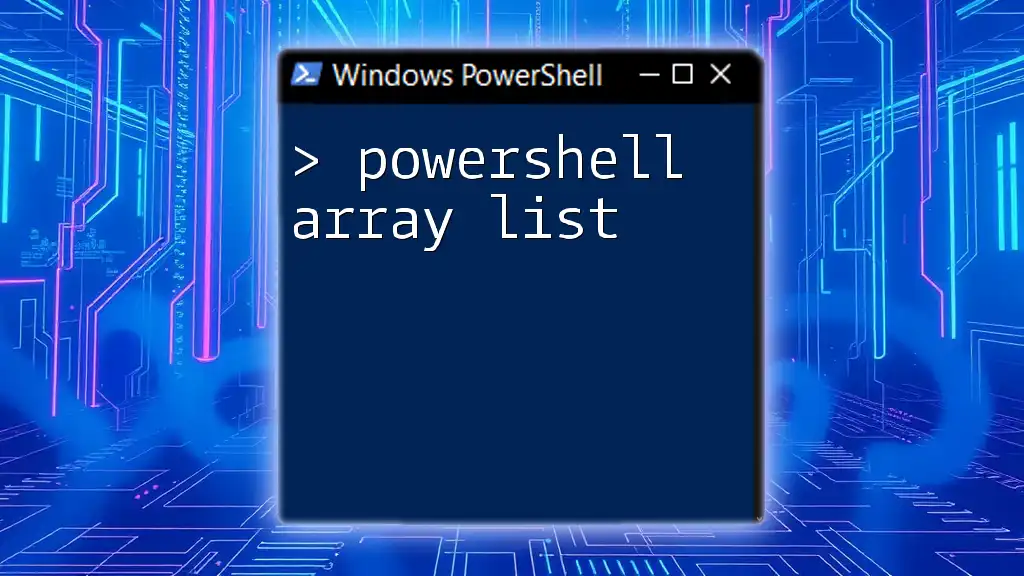
Common Issues and Troubleshooting
Handling Null or Empty Arrays
When dealing with arrays, you might encounter null or empty scenarios that can lead to errors during execution. It's vital to check for these conditions before attempting to count elements. Here's how you can check:
if ($null -eq $myArray -or $myArray.Count -eq 0) {
Write-Output "Array is either null or empty."
}
This code snippet ensures that you won't face unexpected errors when executing subsequent operations.
Performance Impact of Large Arrays
Large arrays can cause performance bottlenecks if not properly managed. When working with such datasets, always consider memory allocation and execution time. Using optimized methods and avoiding complex operations can significantly improve performance.
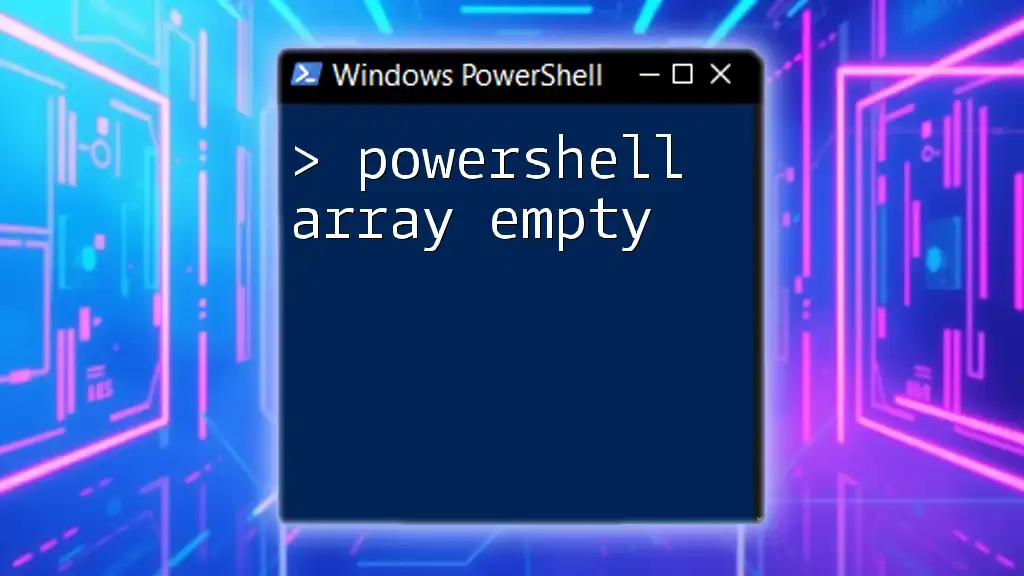
Additional Resources
Online Documentation
To deepen your understanding of PowerShell arrays and the various ways to manipulate them, refer to the official Microsoft PowerShell documentation. This resource contains detailed explanations and examples that can enhance your skills.
Useful Cmdlets
Familiarizing yourself with additional cmdlets that work well with arrays, such as `ForEach-Object` and `Where-Object`, can expand your scripting capabilities. These tools provide added flexibility in processing data, allowing for more streamlined automation.
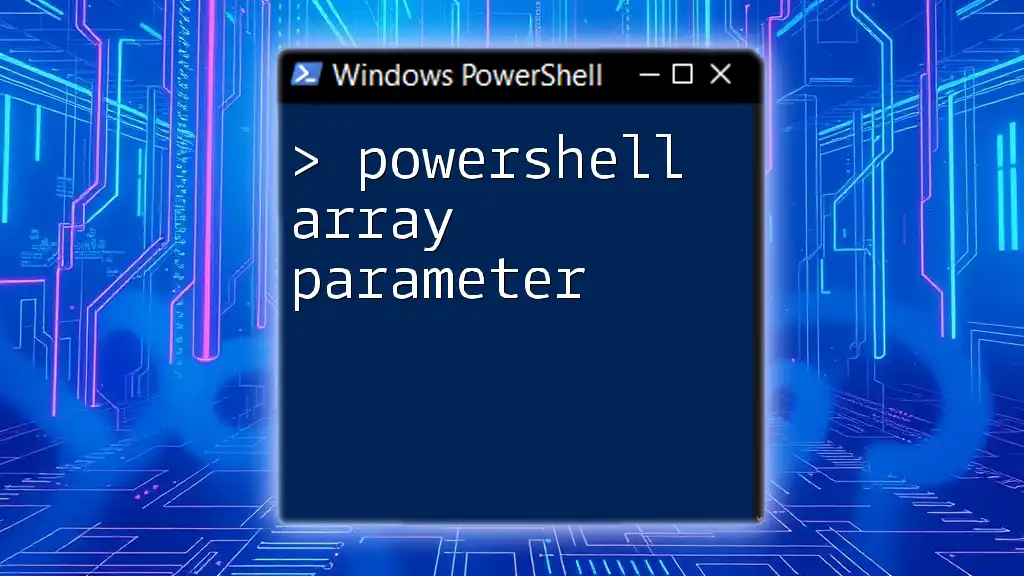
Conclusion
PowerShell's array count functionality is an essential skill for anyone looking to harness the full power of PowerShell scripting. By understanding how to create, manipulate, and count elements in arrays, you can develop scripts that are both efficient and effective.
Through practice and exploration of these concepts, you will become more proficient in automating tasks and managing larger datasets. Keep experimenting with what you've learned today, and you'll become an expert in PowerShell in no time!
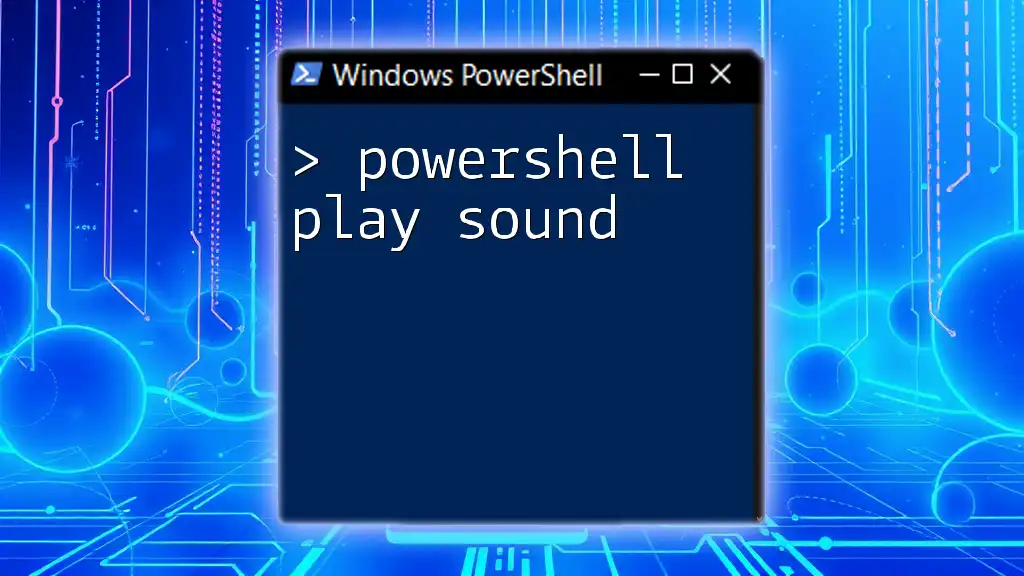
Call to Action
To further enhance your PowerShell skills, consider subscribing for additional tutorials or exploring our free e-book that delves into best practices for effective PowerShell scripting. Embrace this opportunity to elevate your automation capabilities!