In PowerShell, you can play a sound file using the `System.Media.SoundPlayer` class, as shown in the snippet below:
$player = New-Object System.Media.SoundPlayer 'C:\path\to\your\soundfile.wav'; $player.PlaySync();
Understanding Sound in PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administrators and power users. It allows users to automate tasks and manage system configurations efficiently. One of the many capabilities of PowerShell is the ability to interact with system-level components, such as sound.
Why Use Sound in Scripts?
Incorporating sound notifications into your scripts can significantly enhance the user experience. Whether you need alerts for completed processes or warnings for errors, audio cues can draw attention effectively. Imagine a script that performs automated tasks throughout the day; a sound alert can notify you when specific conditions arise, making your workflow smoother and more efficient.
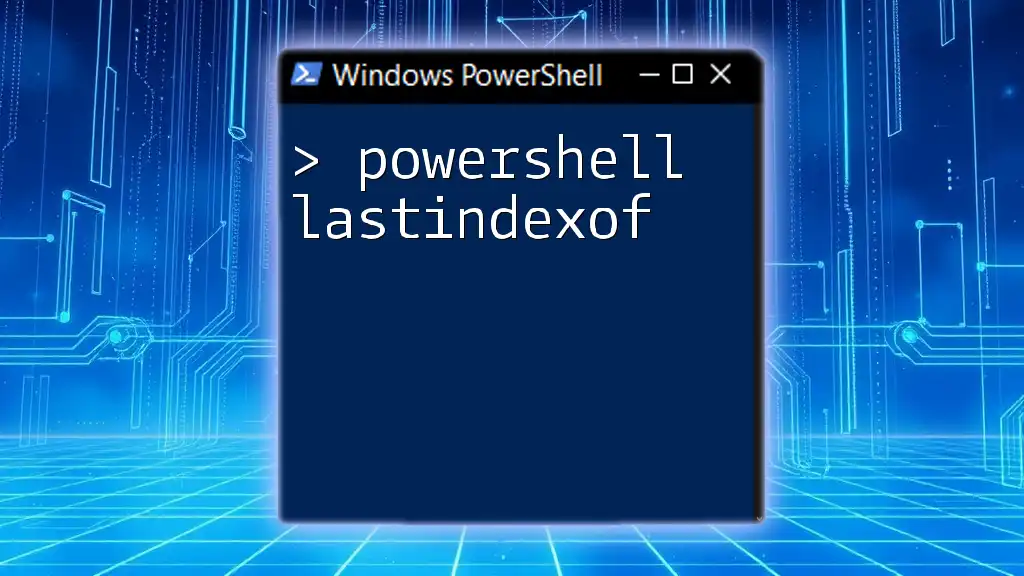
Playing Sounds with PowerShell
Overview of Sound File Formats
When dealing with audio in PowerShell, it’s essential to understand compatible sound file formats. The most commonly used format, .wav, is recommended because it is natively supported and offers fast playback without conversion delays. While .mp3 files can be used, they may require additional handling and libraries.
Built-in Methods to Play Sounds in PowerShell
Using the `System.Media.SoundPlayer`
The `System.Media.SoundPlayer` class provides a straightforward way to play sound files. It is primarily designed for `.wav` files and offers two main methods for playback: Play() (asynchronous) and PlaySync() (synchronous).
Example:
$player = New-Object System.Media.SoundPlayer
$player.SoundLocation = "C:\Path\To\Your\Sound.wav"
$player.PlaySync() # Plays the sound synchronously
In this example, the sound file specified by SoundLocation will play until it completes before the script continues execution. If you want the script to continue running while the sound plays, use Play() instead.
Utilizing Windows Media Player COM Object
Another method for playing sounds in PowerShell is by using the Windows Media Player COM object. This is versatile because it can handle multiple audio formats, including `.mp3`.
Example:
$wmp = New-Object -ComObject WMPlayer.OCX
$wmp.URL = "C:\Path\To\Your\Sound.mp3"
$wmp.controls.play()
In this case, we've created an instance of Windows Media Player and specified the URL of the sound file. $wmp.controls.play() allows for additional controls over playback, such as pause, stop, and volume adjustments.
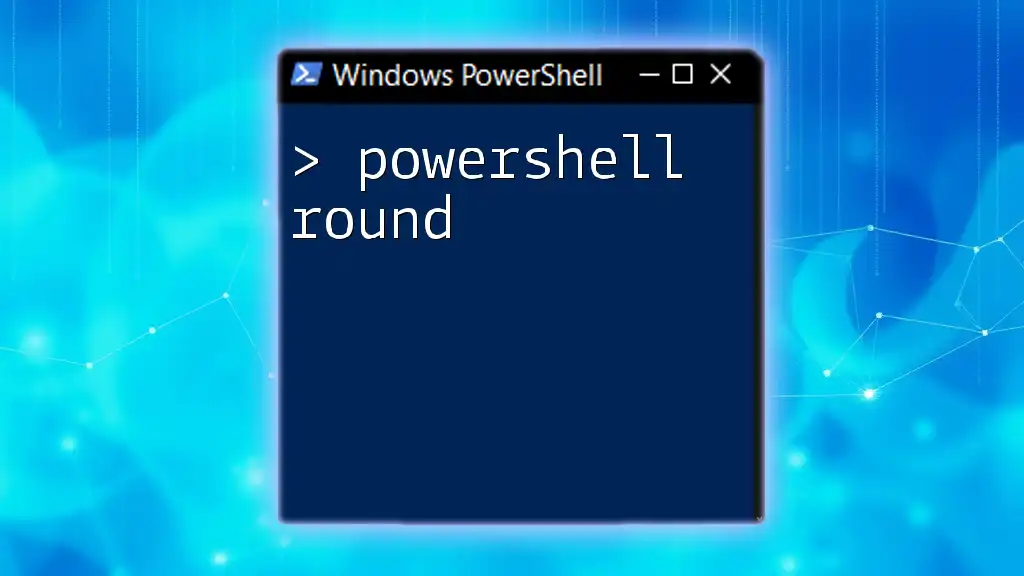
Advanced Sound Playback Techniques
Creating a Sound Notification System
Incorporating a sound notification system adds significant functionality to your scripts, particularly for monitoring tasks. For example, imagine a PowerShell script that continuously checks for a running process and alerts you with sound when it finds it.
Example Implementation:
if (Get-Process "notepad" -ErrorAction SilentlyContinue) {
$player.PlaySync()
}
In this snippet, the script checks if Notepad is running. If it is, the sound will play, effectively notifying you without needing constant checks visually.
Scheduling Sound Alerts with Task Scheduler
You can enhance your automation further by scheduling sound alerts with Windows Task Scheduler. By creating a task that triggers the playback of a sound file at specific intervals or based on system events, you can ensure that you receive notifications aligned with your workflow.
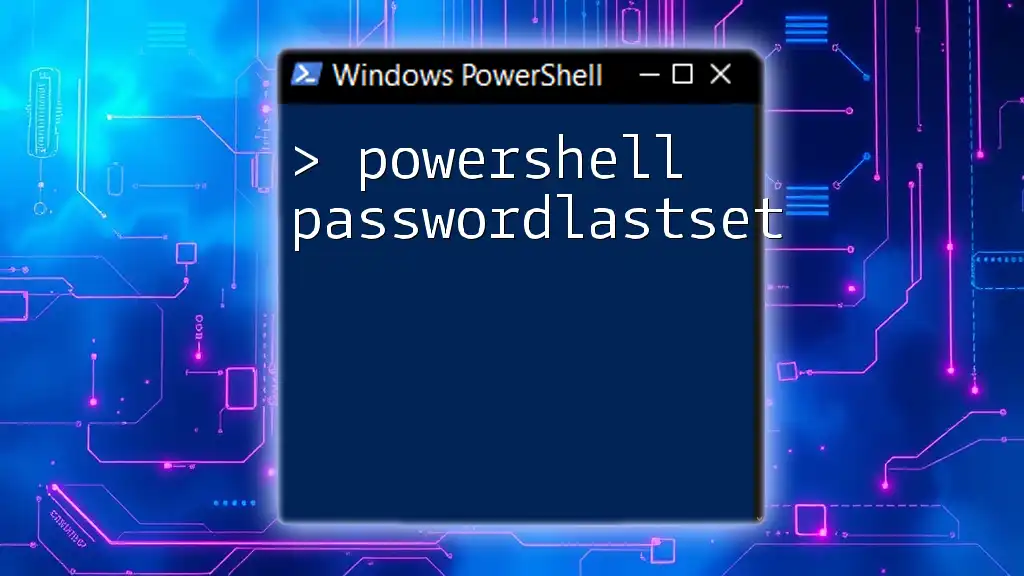
Troubleshooting Sound Playback Issues
Common Problems and Solutions
When implementing sound playback in PowerShell scripts, several common issues may arise:
- No sound output: This often occurs due to incorrect path specifications or volume settings. Ensure the file path is correct and check your system's volume settings.
- File type not supported: PowerShell typically supports `.wav` files natively. If you're using another format, it’s advisable to convert them to `.wav`.
Debugging Sound Playback with PowerShell
Effective debugging can save a lot of time during development. Implement Try-Catch blocks in your scripts to handle any errors gracefully:
Example:
try {
$player = New-Object System.Media.SoundPlayer
$player.SoundLocation = "C:\Path\To\Your\Sound.wav"
$player.PlaySync()
} catch {
Write-Host "An error occurred: $_"
}
This snippet captures any exceptions and outputs them to the console, helping you identify issues quickly.
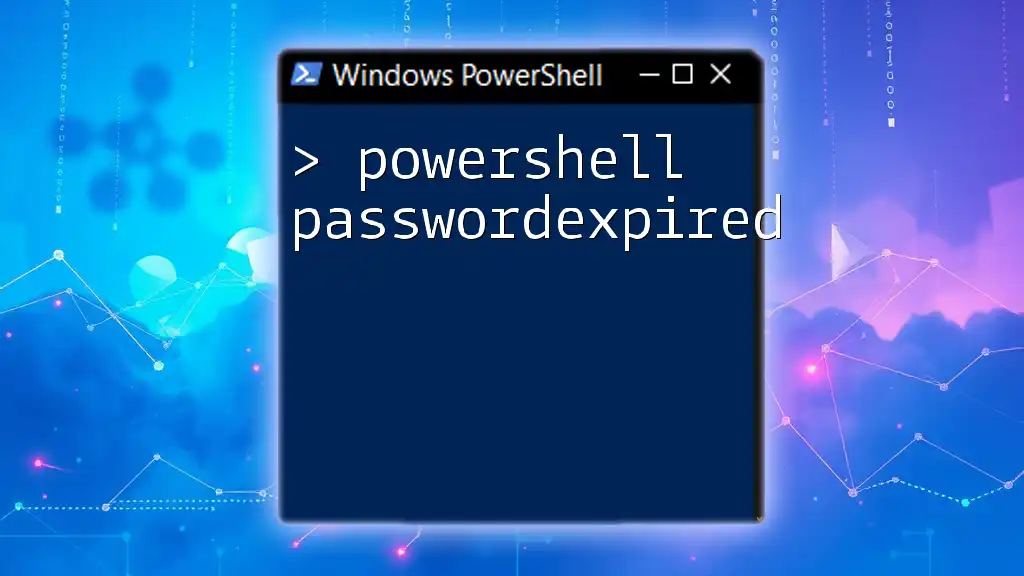
Best Practices for Sound in PowerShell Scripts
Choosing the Right Sound Files
When integrating sound, it’s crucial to choose files that are appropriate for the context. Sound files should be short and clear—long or annoying sounds can disrupt productivity. Aim for audio cues that are noticeable but not intrusive.
Documenting Sound Usage in Scripts
Proper documentation enhances the maintainability of your scripts. Including comments that explain why a sound is played or what event triggers it is beneficial for anyone who may work with your code in the future.
Example of well-documented sound playback code:
# Check for Notepad process and play alert sound if found
if (Get-Process "notepad" -ErrorAction SilentlyContinue) {
$player.SoundLocation = "C:\Path\To\Your\AlertSound.wav"
$player.PlaySync() # Alert sound for user notification
}
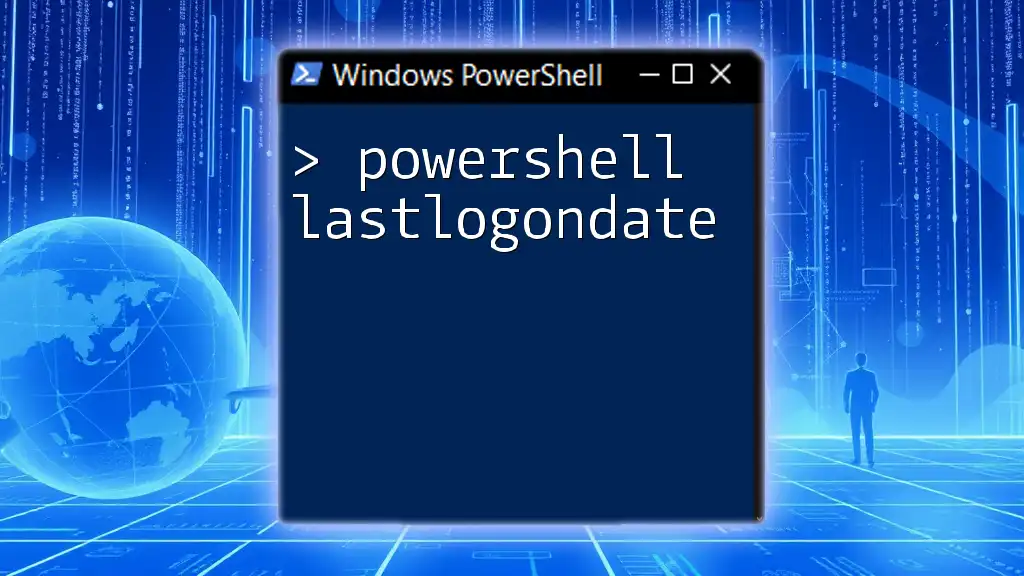
Conclusion
Integrating sound playback into your PowerShell scripts can elevate functionality and provide essential notifications in your automated workflows. From alerting you to system changes to enhancing the interactivity of your scripts, using PowerShell play sound features effectively can lead to a more efficient user experience.
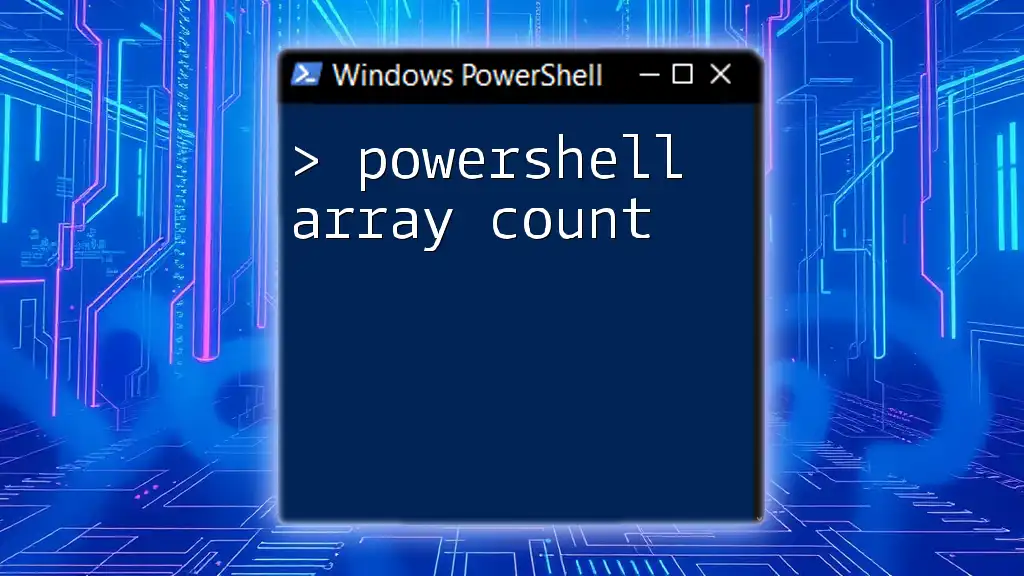
Call to Action
Experiment with your own scripts to incorporate sound notifications. Don’t hesitate to share your scripts or ask questions! For further resources, tutorials, or specialized training in PowerShell, explore our offerings designed to empower your scripting capabilities.