The `PadLeft` method in PowerShell is used to align strings to the right by padding them with a specified character, allowing you to format output cleanly.
Here's a simple example of using `PadLeft` in PowerShell:
$number = "5"
$paddedNumber = $number.PadLeft(3, '0')
Write-Host $paddedNumber # Output: 005
Understanding `PadLeft`
What is `PadLeft`?
The `PadLeft` method in PowerShell is used to format strings by adding padding to the left side. This method is particularly useful when you need to ensure that strings align properly in outputs, creating a more organized and readable presentation, especially when dealing with tabular data or logs.
Syntax of `PadLeft`
The syntax for the `PadLeft` method is straightforward:
$string.PadLeft(length, char)
- `length`: Specifies the total length of the resulting string after padding.
- `char`: (Optional) Defines the character to use for padding. If not provided, spaces are used by default.
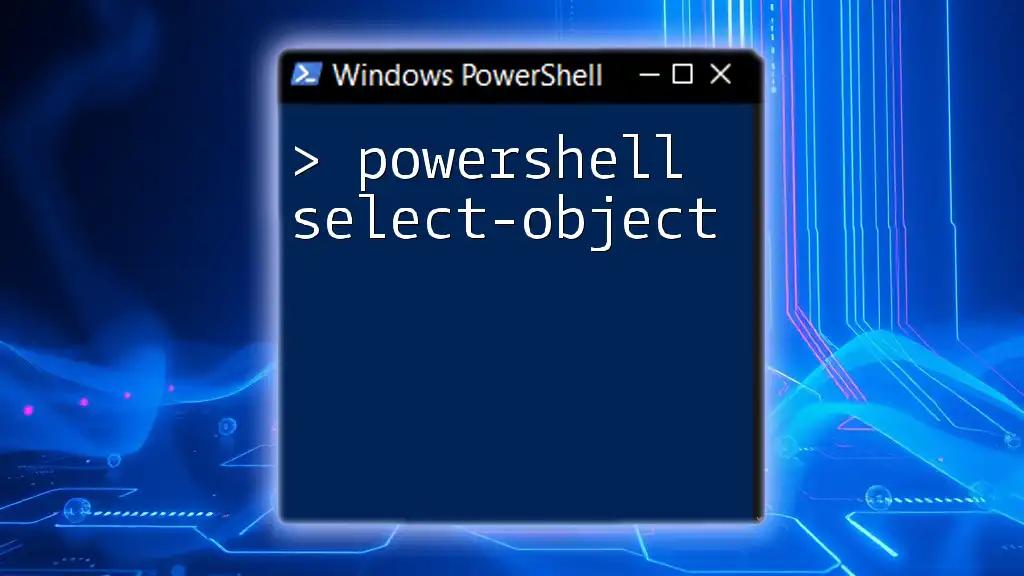
How `PadLeft` Works
Basic Functionality
The essential function of `PadLeft` is to add spaces or a specified character to the left side of a string until it reaches the desired total length.
Example 1: Basic usage of `PadLeft`
$text = "Hello"
$paddedText = $text.PadLeft(10)
Write-Output $paddedText
In this example, the output would be:
Hello
The string "Hello" is padded with spaces so that its total length becomes 10 characters.
Padding with Custom Characters
You can also specify a character to pad the string. This can be useful for creating visually distinct outputs in reports or logs.
Example 2: Padding with asterisks
$text = "Hello"
$paddedText = $text.PadLeft(10, '*')
Write-Output $paddedText
This example produces:
*****Hello
Here, the string "Hello" is padded with asterisks on the left.
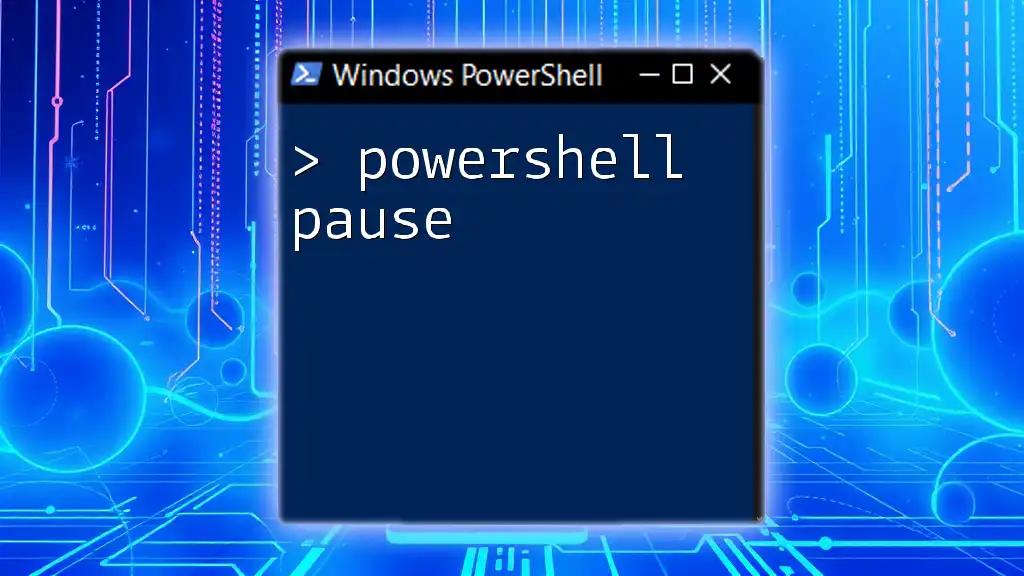
Common Use Cases for `PadLeft`
Formatting Output
The `PadLeft` method is particularly valuable for producing well-formatted output. Often, when presenting data, especially multiple values, padding ensures that the elements are aligned, which enhances readability.
Example 3: Creating a formatted table
$data = @("One", "Two", "Three")
foreach ($item in $data) {
$formattedItem = $item.PadLeft(5)
Write-Output $formattedItem
}
The output from this code would align all items:
One
Two
Three
The text is aligned neatly to the right, providing a cleaner appearance.
Data Processing
`PadLeft` is useful in string manipulations, especially in data processing scenarios such as reading logs or CSV files where consistent formats are crucial.
Example 4: Aligning usernames in a log report
$usernames = @("Alice", "Bob", "Charlie")
foreach ($username in $usernames) {
Write-Output "$($username.PadLeft(10)) - Action logged."
}
The resulting output will look like:
Alice - Action logged.
Bob - Action logged.
Charlie - Action logged.
This example demonstrates how `PadLeft` can help maintain a uniform log format, making it easier to read.
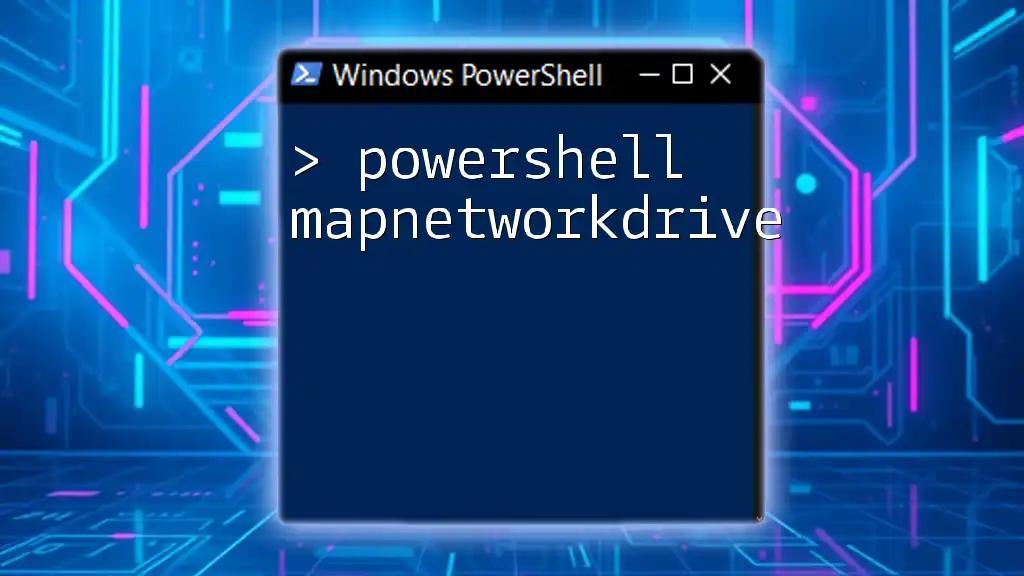
Performance Considerations
Efficiency
While `PadLeft` is efficient in most scenarios, using it on large strings or arrays may affect performance. PowerShell handles string operations relatively well, but when dealing with massive datasets, it’s wise to benchmark and profile your scripts to ensure they perform adequately.
Limitations
Though `PadLeft` is powerful, there are limitations to be aware of. For instance, if the specified length is less than the original string, the method returns the original string without padding. Additionally, you need to consider the execution context; excessive padding could lead to truncated outputs in some instances.
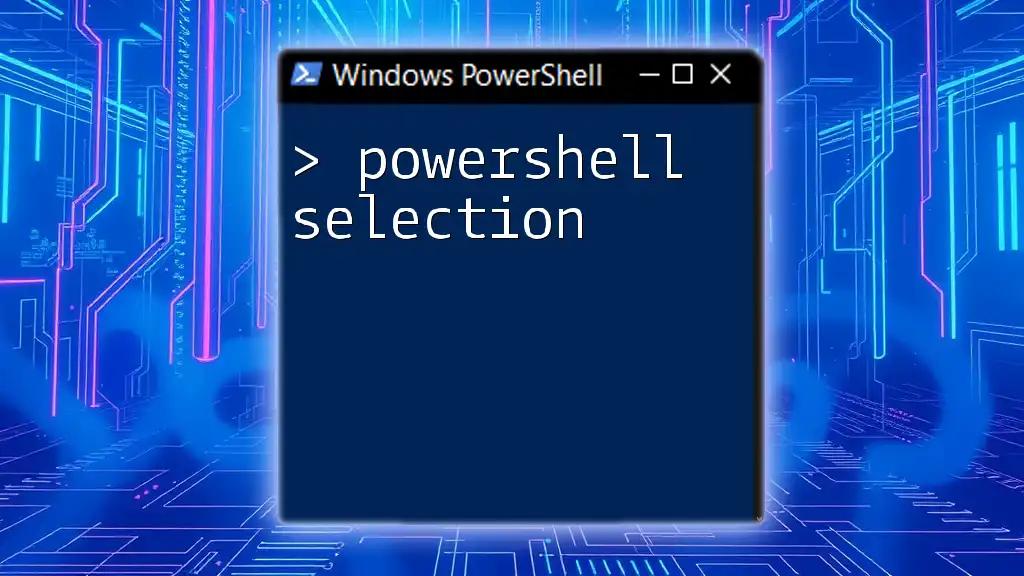
Best Practices
Keeping it Readable
When using `PadLeft`, readability should always be a consideration. Code that is easy to read and maintain ensures that future modifications are trouble-free. Strive to avoid overly complex combinations that might confuse readers.
Combining `PadLeft` with other string methods can yield improved outcomes.
Example 5: Combining with `ToUpper`
$text = "test"
$formattedText = $text.ToUpper().PadLeft(10)
Write-Output $formattedText
This results in:
TEST
Here, the string is converted to uppercase and then padded, illustrating how `PadLeft` can be used alongside other string methods.
Use in Scripts
It's essential to integrate `PadLeft` meaningfully in your PowerShell scripts. When handling user input or dynamic content, adjusting the padding dynamically ensures output remains consistent and clear.

Troubleshooting Common Issues
Common Errors with `PadLeft`
Like any coding technique, mistakes can happen. Common issues include specifying characters that aren't valid for padding or using incorrect lengths, which may lead to unintended outputs.
Debugging
When debugging any problems linked to `PadLeft`, you can employ write-debug techniques or utilize PowerShell's `Test-Path` cmdlet to verify string properties. Trusting in PowerShell's capabilities to handle exceptions and errors will also make troubleshooting easier.
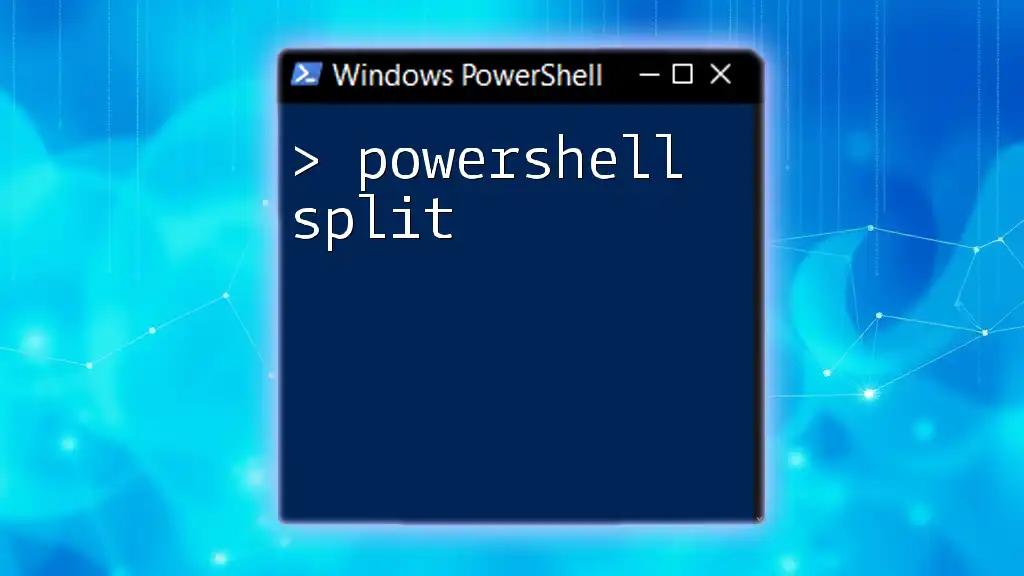
Conclusion
In summary, the `PadLeft` method is an invaluable tool in PowerShell for formatting strings. Its ability to align and present data clearly is essential for many scripting scenarios. By practicing basic examples and exploring its functionalities, you can enhance your PowerShell skills and create more organized outputs.
Additional Resources
For those eager to dive deeper, numerous tutorials, articles, and courses are available to further your understanding of PowerShell's string manipulation capabilities and beyond. Engaging with these resources will facilitate continuous learning and mastery in PowerShell scripting.