PowerShell SFTP allows users to transfer files securely over SSH using the SFTP protocol, enabling efficient file management and automation in a streamlined manner.
Here's a simple code snippet to establish an SFTP session and upload a file:
# Create a new SFTP session and upload a file
$SftpSession = New-Object WinSCP.SessionOptions -Property @{
Protocol = [WinSCP.Protocol]::Sftp
HostName = "example.com"
UserName = "username"
Password = "password"
SshHostKey = "ssh-rsa 2048 xxxxxxxxxxx...="
}
$Session = New-Object WinSCP.Session
$Session.Open($SftpSession)
$TransferOptions = New-Object WinSCP.TransferOptions
$TransferOptions.TransferMode = [WinSCP.TransferMode]::Binary
$Session.PutFiles("C:\local\path\to\file.txt", "/remote/path/to/").Check()
$Session.Dispose()
Make sure to replace `"example.com"`, `"username"`, `"password"`, and file paths with your actual SFTP server details and file locations.
Understanding SFTP
What is SFTP?
SFTP, or Secure File Transfer Protocol, is a network protocol that provides secure and encrypted file transfer capabilities over a reliable data stream. Unlike FTP (File Transfer Protocol), SFTP not only encrypts the data during transmission but also encrypts the commands exchanged between the client and server. This ensures that sensitive information such as usernames, passwords, and files being transferred remain confidential and secure from eavesdropping attacks.
When to Use SFTP
SFTP should be your go-to method for file transfers whenever security is a priority. This is particularly important for scenarios involving sensitive data, such as financial transactions, personal information, or any confidential corporate data. If you need to manage files across servers or ensure secure upload/download for applications, SFTP is the ideal choice.

Why Use PowerShell for SFTP?
Benefits of Using PowerShell
Using PowerShell for SFTP provides numerous benefits:
- Automation: Automating repetitive file transfer tasks via scripts reduces human error and saves time.
- Integration: PowerShell seamlessly integrates with other Microsoft tools and services, making it a versatile option for system administrators.
- Scripting: Custom commands and scripts can be created for specific needs, offering reusable and targeted solutions.
Use Cases for SFTP in PowerShell
Many organizations use PowerShell SFTP for:
- Automating scheduled data backups to remote servers.
- Securely exchanging files with partners or clients.
- Syncing files between local and remote systems.

Setting Up Your Environment
Prerequisites for Using SFTP with PowerShell
Before you can begin using SFTP in PowerShell, ensure you have the necessary tools:
- OpenSSH: Install OpenSSH for Windows to get access to SFTP commands. This is often included in Windows 10 and later versions.
Configuring PowerShell for SFTP
To prepare your PowerShell environment, you should import any necessary modules. If you're using the Posh-SSH module, for example, you can install it using:
Install-Module -Name Posh-SSH -Force
After installing, import the module into your session with:
Import-Module Posh-SSH
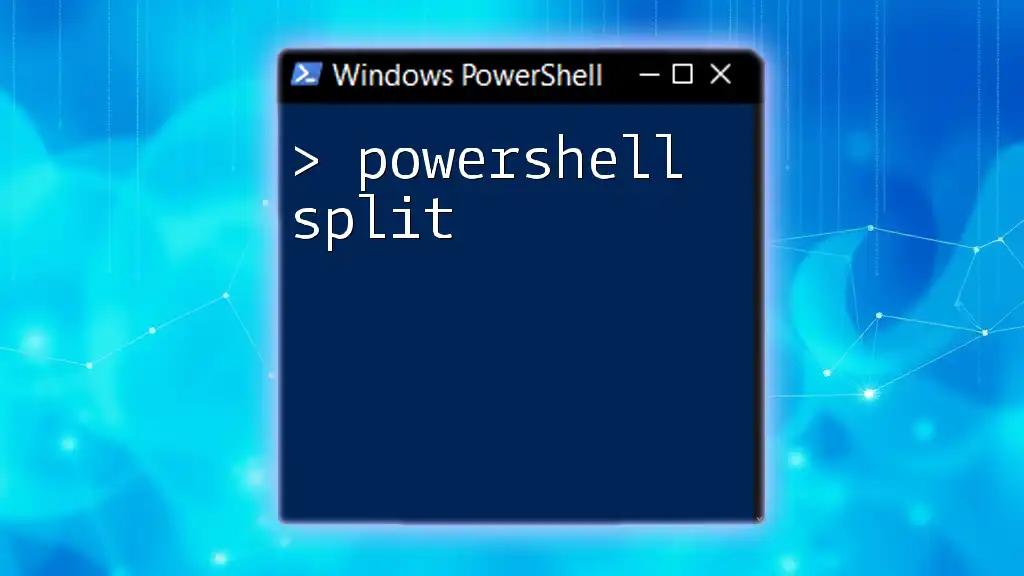
Basic SFTP Commands in PowerShell
Establishing an SFTP Connection
Initiating an SFTP session begins with creating a connection to your SFTP server. Here’s how you can do it:
$session = New-SFTPSession -ComputerName "sftp.example.com" -Credential (Get-Credential)
You will be prompted for your username and password here. This creates a session object that can be used for subsequent commands.
Uploading Files via SFTP
Uploading files is straightforward once the session is established. Use the `Put-SFTPItem` command to transfer files from your local machine to the server:
Put-SFTPItem -SessionId $session.SessionId -Path "localFile.txt" -Destination "/remote/path/localFile.txt"
Downloading Files via SFTP
To download files, the `Get-SFTPItem` command is your friend. Here’s how you can retrieve a file from a remote server:
Get-SFTPItem -SessionId $session.SessionId -Path "/remote/path/remoteFile.txt" -Destination "localPath/remoteFile.txt"
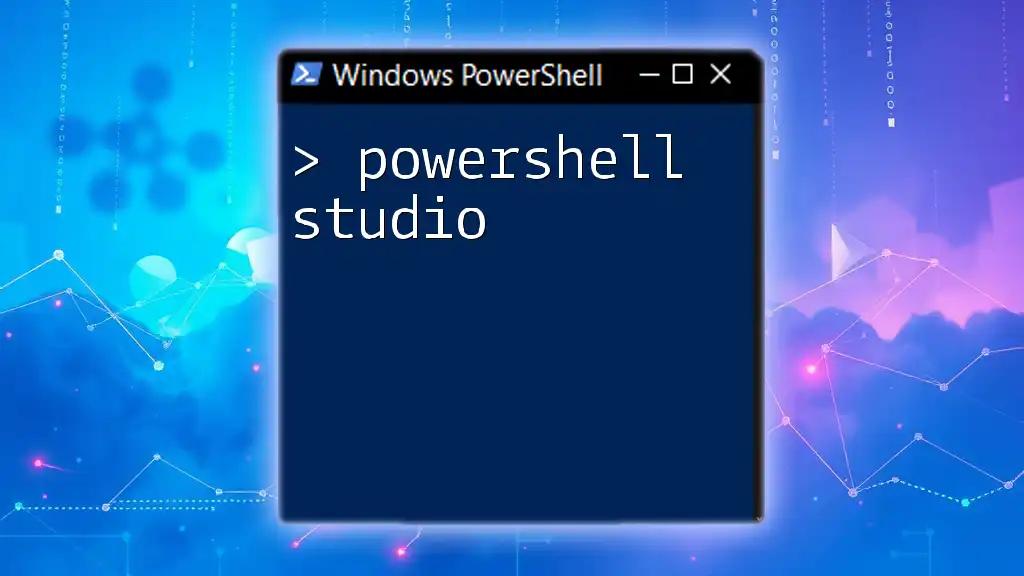
Advanced SFTP Operations
Managing Directories with PowerShell
Directory operations can be handled with ease in PowerShell. Create a new directory using:
New-SFTPDirectory -SessionId $session.SessionId -Path "/remote/path/newDirectory"
To remove an existing directory:
Remove-SFTPDirectory -SessionId $session.SessionId -Path "/remote/path/removeDirectory"
Handling Errors in SFTP Commands
Errors during SFTP operations can be managed using `try-catch` blocks. This helps ensure that your script doesn’t crash unexpectedly. Here’s an example:
try {
# Your SFTP command, e.g., uploading a file
} catch {
Write-Host "An error occurred: $_"
}
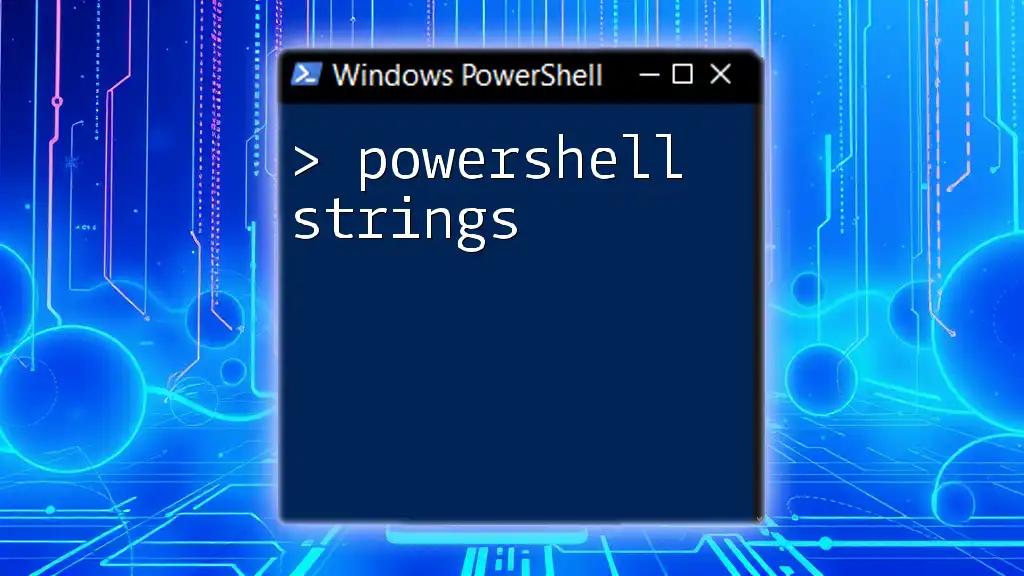
Automating SFTP Tasks with PowerShell Scripts
Writing Your First SFTP Script
Writing a simple PowerShell script to automate SFTP tasks can greatly enhance efficiency. Here’s an example of a script to upload a file and log the operations:
$session = New-SFTPSession -ComputerName "sftp.example.com" -Credential (Get-Credential)
Put-SFTPItem -SessionId $session.SessionId -Path "localFile.txt" -Destination "/remote/path/localFile.txt"
Write-Host "File uploaded successfully."
Remove-SFTPSession -SessionId $session.SessionId
Scheduling the SFTP Script
You can automate your SFTP script to run at specified intervals using Windows Task Scheduler. Create a new task, set triggers, and point the action to your PowerShell script. This setup allows for seamless automated file transfers.

Security Best Practices for SFTP in PowerShell
Using SSH Keys for Authentication
For enhanced security, consider using SSH keys instead of passwords. Generate a key pair using:
ssh-keygen -t rsa -b 2048
Add the generated public key to your SFTP server, enabling more secure authentication without needing passwords.
Ensuring Secure Connections
Always ensure your SFTP sessions use up-to-date versions of encryption protocols. Regularly monitor systems for unauthorized access attempts, and utilize logging features available in PowerShell to track all SFTP activities.
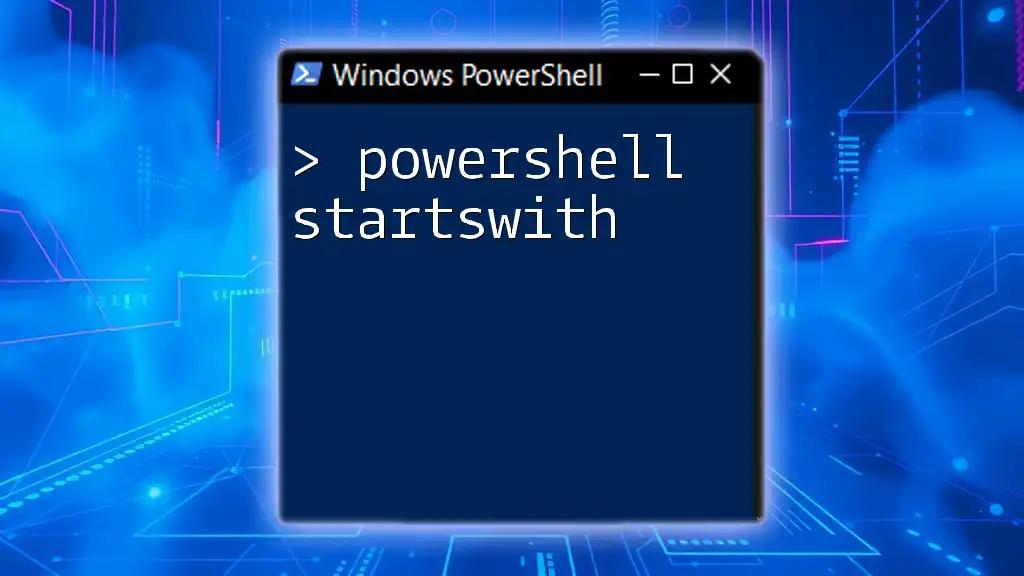
Conclusion
In conclusion, utilizing PowerShell SFTP can significantly streamline secure file transfer processes within your organization. The combination of robust scripting capabilities with the safety of SFTP provides a powerful tool for any administrator. It’s time to leverage these tools for more efficient and secure data management.
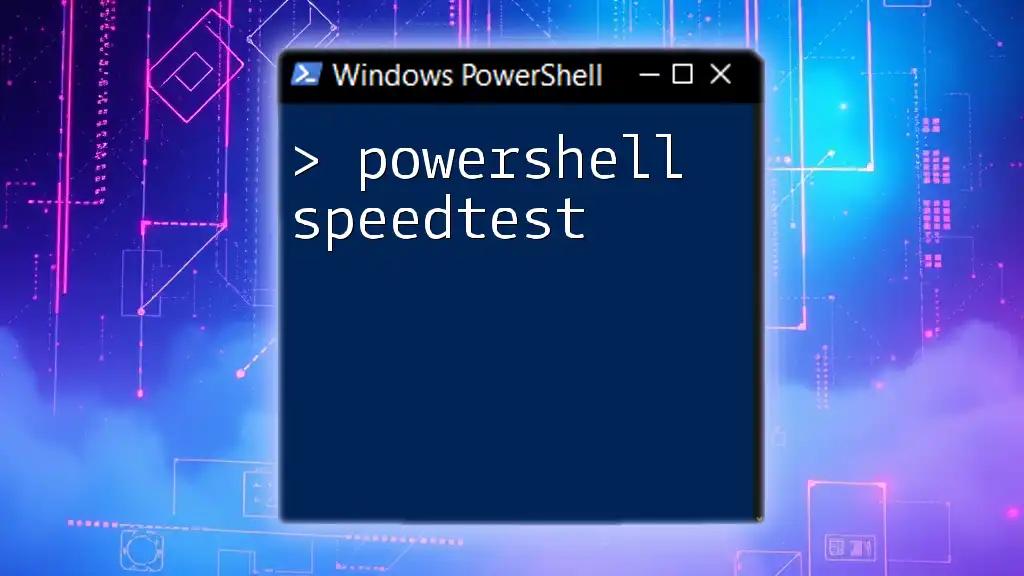
Additional Resources
For further reading and exploration of PowerShell SFTP, consider reviewing the official PowerShell documentation and various modules available to extend functionality. Engage with the community through forums and attend workshops to deepen your knowledge.
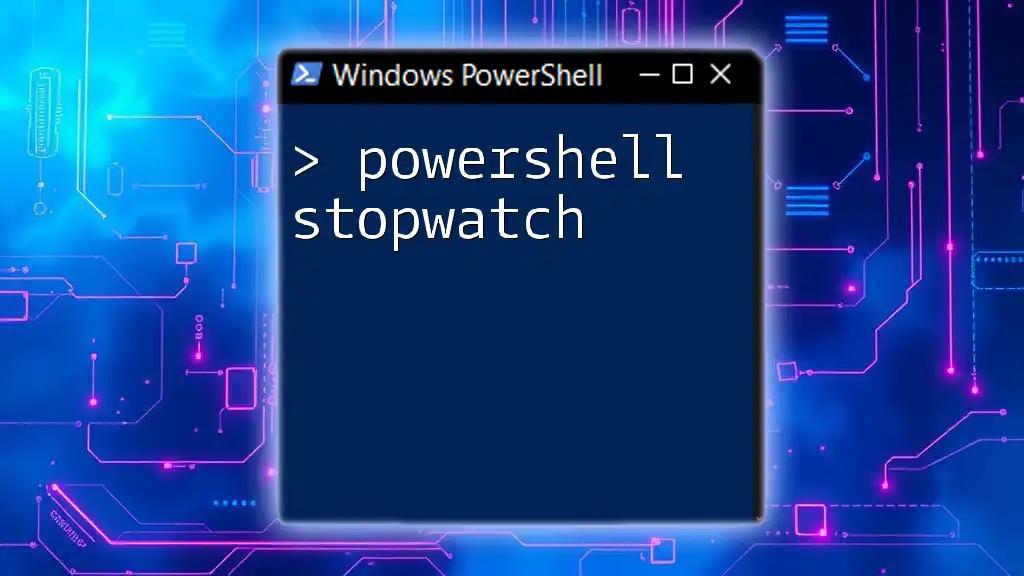
Call to Action
Join our mailing list to get more tips on maximizing your PowerShell experience and take part in upcoming workshops for hands-on training in PowerShell and its applications in secure file transfers.